Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / Serialization / manager / ReachIDocumentPaginatorSerializer.cs / 1 / ReachIDocumentPaginatorSerializer.cs
/*++ Copyright (C) 2004- 2005 Microsoft Corporation All rights reserved. Module Name: ReachIDocumentPaginatorSerializer.cs Abstract: Author: [....] ([....]) January 2005 Revision History: --*/ using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Xml; using System.IO; using System.Security; using System.Security.Permissions; using System.ComponentModel.Design.Serialization; using System.Windows.Xps.Packaging; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Markup; namespace System.Windows.Xps.Serialization { ////// /// internal class DocumentPaginatorSerializer : ReachSerializer { ////// /// public DocumentPaginatorSerializer( PackageSerializationManager manager ) : base(manager) { } public override void SerializeObject( Object serializedObject ) { // // Create the ImageTable required by the Type Converters // The Image table at this time is shared / document // ((XpsSerializationManager)SerializationManager).ResourcePolicy.ImageCrcTable = new Dictionary(); ((XpsSerializationManager)SerializationManager).ResourcePolicy.ImageUriHashTable = new Dictionary (); // // Create the ColorContextTable required by the Type Converters // The ColorContext table at this time is shared / document // ((XpsSerializationManager)SerializationManager).ResourcePolicy.ColorContextTable = new Dictionary (); SerializableObjectContext serializableObjectContext = new SerializableObjectContext(serializedObject, null); PersistObjectData(serializableObjectContext); } /// /// /// internal override void PersistObjectData( SerializableObjectContext serializableObjectContext ) { String xmlnsForType = SerializationManager.GetXmlNSForType(typeof(FixedDocument)); String nameForType = XpsS0Markup.FixedDocument; if( SerializationManager is XpsSerializationManager) { (SerializationManager as XpsSerializationManager).RegisterDocumentStart(); } if (xmlnsForType == null) { XmlWriter.WriteStartElement(nameForType); } else { XmlWriter.WriteStartElement(nameForType, xmlnsForType); } { XpsSerializationPrintTicketRequiredEventArgs e = new XpsSerializationPrintTicketRequiredEventArgs(PrintTicketLevel.FixedDocumentPrintTicket, 0); ((XpsSerializationManager)SerializationManager).OnXPSSerializationPrintTicketRequired(e); // // Serialize the data for the PrintTicket // if(e.Modified) { if(e.PrintTicket != null) { PrintTicketSerializer serializer = new PrintTicketSerializer(SerializationManager); serializer.SerializeObject(e.PrintTicket); } } DocumentPaginator paginator = (DocumentPaginator)serializableObjectContext.TargetObject; XmlLanguage language = null; DependencyObject dependencyObject = paginator.Source as DependencyObject; if (dependencyObject != null) { language = (XmlLanguage)dependencyObject.GetValue(FrameworkContentElement.LanguageProperty); } if (language == null) { //If the language property is null, assign the language to the default language = XmlLanguage.GetLanguage(XpsS0Markup.XmlLangValue); } SerializationManager.Language = language; for (int i = 0; !paginator.IsPageCountValid || (i < paginator.PageCount); i++) { DocumentPage page = Toolbox.GetPage(paginator, i); ReachSerializer serializer = SerializationManager.GetSerializer(page); if (serializer != null) { serializer.SerializeObject(page); } } } XmlWriter.WriteEndElement(); XmlWriter = null; // // Clear off the table from the resource policy // ((XpsSerializationManager)SerializationManager).ResourcePolicy.ImageCrcTable = null; ((XpsSerializationManager)SerializationManager).ResourcePolicy.ImageUriHashTable = null; // // Clear off the table from the resource policy // ((XpsSerializationManager)SerializationManager).ResourcePolicy.ColorContextTable = null; // // Signal to any registered callers that the Document has been serialized // XpsSerializationProgressChangedEventArgs progressEvent = new XpsSerializationProgressChangedEventArgs(XpsWritingProgressChangeLevel.FixedDocumentWritingProgress, 0, 0, null); if( SerializationManager is XpsSerializationManager) { (SerializationManager as XpsSerializationManager).RegisterDocumentEnd(); } ((XpsSerializationManager)SerializationManager).OnXPSSerializationProgressChanged(progressEvent); } ////// /// public override XmlWriter XmlWriter { get { if (base.XmlWriter == null) { base.XmlWriter = SerializationManager.AcquireXmlWriter(typeof(FixedDocument)); } return base.XmlWriter; } set { base.XmlWriter = null; SerializationManager.ReleaseXmlWriter(typeof(FixedDocument)); } } }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
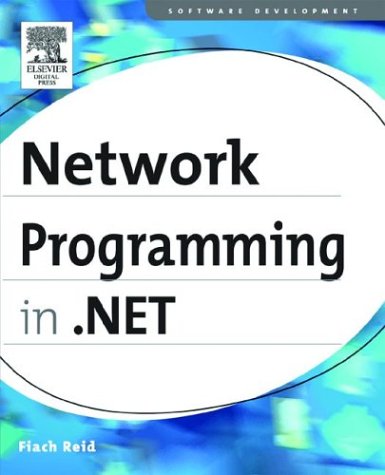
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XPathNodeList.cs
- DeadCharTextComposition.cs
- SchemaAttDef.cs
- OdbcException.cs
- dataSvcMapFileLoader.cs
- WinEventTracker.cs
- PropertyInfoSet.cs
- TagPrefixAttribute.cs
- PageTheme.cs
- CapacityStreamGeometryContext.cs
- OSFeature.cs
- ObjectStorage.cs
- EnumerationRangeValidationUtil.cs
- BrowserPolicyValidator.cs
- CommandValueSerializer.cs
- DllNotFoundException.cs
- XmlValueConverter.cs
- OLEDB_Enum.cs
- Rotation3D.cs
- RequestCachingSection.cs
- MouseBinding.cs
- StrokeNodeEnumerator.cs
- SystemIPAddressInformation.cs
- AccessibleObject.cs
- X509Extension.cs
- MappingSource.cs
- PolicyImporterElementCollection.cs
- AnnotationDocumentPaginator.cs
- ParallelDesigner.xaml.cs
- ConsumerConnectionPointCollection.cs
- WebMessageEncoderFactory.cs
- CodeCommentStatement.cs
- EventlogProvider.cs
- AmbientValueAttribute.cs
- HostingEnvironmentException.cs
- PointAnimation.cs
- PrintDocument.cs
- SourceCollection.cs
- RemotingServices.cs
- ResourceSet.cs
- Helpers.cs
- ResourceAttributes.cs
- DataTemplateKey.cs
- SrgsOneOf.cs
- ByeMessage11.cs
- FlowNode.cs
- TextTreeNode.cs
- PropertiesTab.cs
- BinaryObjectInfo.cs
- PropertyPanel.cs
- XmlReflectionMember.cs
- ApplicationActivator.cs
- GiveFeedbackEvent.cs
- ListItemCollection.cs
- MenuItemCollectionEditor.cs
- EmptyImpersonationContext.cs
- FormatPage.cs
- OLEDB_Util.cs
- Table.cs
- ValidationErrorInfo.cs
- TreeNodeSelectionProcessor.cs
- FunctionDetailsReader.cs
- RecordManager.cs
- PeerObject.cs
- Vector3DIndependentAnimationStorage.cs
- PresentationAppDomainManager.cs
- PageStatePersister.cs
- BatchWriter.cs
- DllHostedComPlusServiceHost.cs
- DbParameterHelper.cs
- TextEditorDragDrop.cs
- RsaEndpointIdentity.cs
- TraceSection.cs
- FixedPageProcessor.cs
- TextContainerChangeEventArgs.cs
- DataSet.cs
- UrlPath.cs
- GifBitmapDecoder.cs
- ConstantSlot.cs
- EditorResources.cs
- QilBinary.cs
- MsmqIntegrationProcessProtocolHandler.cs
- SHA256.cs
- HashCodeCombiner.cs
- SafeNativeMethods.cs
- CodeLabeledStatement.cs
- Padding.cs
- ColumnProvider.cs
- TextParaClient.cs
- EpmCustomContentSerializer.cs
- Marshal.cs
- Scripts.cs
- TraceSection.cs
- AnimationLayer.cs
- ClientRuntimeConfig.cs
- ManagementPath.cs
- BuildManager.cs
- CodeSnippetCompileUnit.cs
- DataServiceQuery.cs
- SafeRegistryKey.cs