Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Input / TextServicesCompartmentContext.cs / 1 / TextServicesCompartmentContext.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Manages Text Services Compartment. // // History: // 07/30/2003 : yutakas - Ported from .net tree. // //--------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Collections; using MS.Internal; using MS.Utility; using MS.Win32; namespace System.Windows.Input { //----------------------------------------------------- // // TextServicesCompartmentContext class // //----------------------------------------------------- internal class TextServicesCompartmentContext { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- ////// private constructer to avoid from creating instance outside. /// private TextServicesCompartmentContext() { } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Get the compartment of the given input method state. /// ////// Critical - retrieves message pump/input manager wrapper class /// TreatAsSafe - returns safe wrapper for property request /// [SecurityCritical, SecurityTreatAsSafe] internal TextServicesCompartment GetCompartment(InputMethodStateType statetype) { for (int i = 0; i < InputMethodEventTypeInfo.InfoList.Length; i++) { InputMethodEventTypeInfo iminfo = InputMethodEventTypeInfo.InfoList[i]; if (iminfo.Type == statetype) { if (iminfo.Scope == CompartmentScope.Thread) return GetThreadCompartment(iminfo.Guid); else if (iminfo.Scope == CompartmentScope.Global) return GetGlobalCompartment(iminfo.Guid); } } return null; } ////// Get the thread compartment of the Guid. /// ////// Critical - manipulates input manager/message pump /// [SecurityCritical] internal TextServicesCompartment GetThreadCompartment(Guid guid) { // No TextServices are installed so that the compartment won't work. if (!TextServicesLoader.ServicesInstalled || TextServicesContext.DispatcherCurrent == null) return null; UnsafeNativeMethods.ITfThreadMgr threadmgr = TextServicesContext.DispatcherCurrent.ThreadManager; if (threadmgr == null) return null; if (_compartmentTable == null) _compartmentTable = new Hashtable(); TextServicesCompartment compartment; compartment = _compartmentTable[guid] as TextServicesCompartment; if (compartment == null) { compartment = new TextServicesCompartment(guid, threadmgr as UnsafeNativeMethods.ITfCompartmentMgr); _compartmentTable[guid] = compartment; } return compartment; } ////// Get the global compartment of the Guid. /// ////// Critical - access input manager directly /// [SecurityCritical] internal TextServicesCompartment GetGlobalCompartment(Guid guid) { // No TextServices are installed so that the compartment won't work. if (!TextServicesLoader.ServicesInstalled || TextServicesContext.DispatcherCurrent == null) return null; if (_globalcompartmentTable == null) _globalcompartmentTable = new Hashtable(); if (_globalcompartmentmanager == null) { UnsafeNativeMethods.ITfThreadMgr threadmgr = TextServicesContext.DispatcherCurrent.ThreadManager; if (threadmgr == null) return null; threadmgr.GetGlobalCompartment(out _globalcompartmentmanager); } TextServicesCompartment compartment = null; compartment = _globalcompartmentTable[guid] as TextServicesCompartment; if (compartment == null) { compartment = new TextServicesCompartment(guid, _globalcompartmentmanager); _globalcompartmentTable[guid] = compartment; } return compartment; } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ ////// Create and get thread local compartment context. /// internal static TextServicesCompartmentContext Current { get { // TextServicesCompartmentContext for the current Dispatcher is stored in InputMethod of // the current Dispatcher. if (InputMethod.Current.TextServicesCompartmentContext == null) InputMethod.Current.TextServicesCompartmentContext = new TextServicesCompartmentContext(); return InputMethod.Current.TextServicesCompartmentContext; } } //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- // cache of ITfCompartments private Hashtable _compartmentTable; private Hashtable _globalcompartmentTable; // cache of the global compartment manager private UnsafeNativeMethods.ITfCompartmentMgr _globalcompartmentmanager; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Manages Text Services Compartment. // // History: // 07/30/2003 : yutakas - Ported from .net tree. // //--------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Collections; using MS.Internal; using MS.Utility; using MS.Win32; namespace System.Windows.Input { //----------------------------------------------------- // // TextServicesCompartmentContext class // //----------------------------------------------------- internal class TextServicesCompartmentContext { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- ////// private constructer to avoid from creating instance outside. /// private TextServicesCompartmentContext() { } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Get the compartment of the given input method state. /// ////// Critical - retrieves message pump/input manager wrapper class /// TreatAsSafe - returns safe wrapper for property request /// [SecurityCritical, SecurityTreatAsSafe] internal TextServicesCompartment GetCompartment(InputMethodStateType statetype) { for (int i = 0; i < InputMethodEventTypeInfo.InfoList.Length; i++) { InputMethodEventTypeInfo iminfo = InputMethodEventTypeInfo.InfoList[i]; if (iminfo.Type == statetype) { if (iminfo.Scope == CompartmentScope.Thread) return GetThreadCompartment(iminfo.Guid); else if (iminfo.Scope == CompartmentScope.Global) return GetGlobalCompartment(iminfo.Guid); } } return null; } ////// Get the thread compartment of the Guid. /// ////// Critical - manipulates input manager/message pump /// [SecurityCritical] internal TextServicesCompartment GetThreadCompartment(Guid guid) { // No TextServices are installed so that the compartment won't work. if (!TextServicesLoader.ServicesInstalled || TextServicesContext.DispatcherCurrent == null) return null; UnsafeNativeMethods.ITfThreadMgr threadmgr = TextServicesContext.DispatcherCurrent.ThreadManager; if (threadmgr == null) return null; if (_compartmentTable == null) _compartmentTable = new Hashtable(); TextServicesCompartment compartment; compartment = _compartmentTable[guid] as TextServicesCompartment; if (compartment == null) { compartment = new TextServicesCompartment(guid, threadmgr as UnsafeNativeMethods.ITfCompartmentMgr); _compartmentTable[guid] = compartment; } return compartment; } ////// Get the global compartment of the Guid. /// ////// Critical - access input manager directly /// [SecurityCritical] internal TextServicesCompartment GetGlobalCompartment(Guid guid) { // No TextServices are installed so that the compartment won't work. if (!TextServicesLoader.ServicesInstalled || TextServicesContext.DispatcherCurrent == null) return null; if (_globalcompartmentTable == null) _globalcompartmentTable = new Hashtable(); if (_globalcompartmentmanager == null) { UnsafeNativeMethods.ITfThreadMgr threadmgr = TextServicesContext.DispatcherCurrent.ThreadManager; if (threadmgr == null) return null; threadmgr.GetGlobalCompartment(out _globalcompartmentmanager); } TextServicesCompartment compartment = null; compartment = _globalcompartmentTable[guid] as TextServicesCompartment; if (compartment == null) { compartment = new TextServicesCompartment(guid, _globalcompartmentmanager); _globalcompartmentTable[guid] = compartment; } return compartment; } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ ////// Create and get thread local compartment context. /// internal static TextServicesCompartmentContext Current { get { // TextServicesCompartmentContext for the current Dispatcher is stored in InputMethod of // the current Dispatcher. if (InputMethod.Current.TextServicesCompartmentContext == null) InputMethod.Current.TextServicesCompartmentContext = new TextServicesCompartmentContext(); return InputMethod.Current.TextServicesCompartmentContext; } } //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- // cache of ITfCompartments private Hashtable _compartmentTable; private Hashtable _globalcompartmentTable; // cache of the global compartment manager private UnsafeNativeMethods.ITfCompartmentMgr _globalcompartmentmanager; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
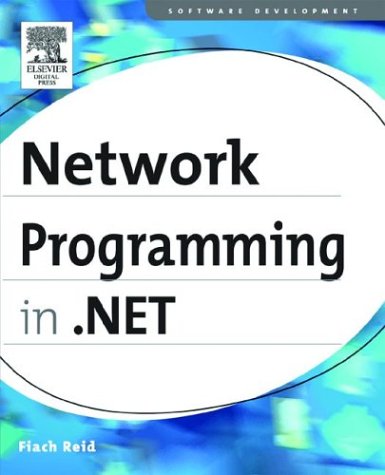
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataSourceXmlAttributeAttribute.cs
- PriorityItem.cs
- Module.cs
- ObsoleteAttribute.cs
- PlatformCulture.cs
- AssemblyResourceLoader.cs
- EntityCollection.cs
- MethodCallTranslator.cs
- EventToken.cs
- SamlAdvice.cs
- TreeNodeConverter.cs
- Comparer.cs
- DebugHandleTracker.cs
- TaskExtensions.cs
- BaseValidator.cs
- EntityDataSourceQueryBuilder.cs
- Guid.cs
- WindowsContainer.cs
- QilIterator.cs
- ValidationRule.cs
- BuildResult.cs
- XmlMembersMapping.cs
- MLangCodePageEncoding.cs
- HttpModule.cs
- TreeViewCancelEvent.cs
- RemotingSurrogateSelector.cs
- UInt64Converter.cs
- Parser.cs
- VisualProxy.cs
- NamedPipeActivation.cs
- TextFormatterHost.cs
- TagNameToTypeMapper.cs
- sqlpipe.cs
- GlyphRunDrawing.cs
- HandledEventArgs.cs
- Stroke.cs
- CommandHelpers.cs
- UnsafeNativeMethods.cs
- EntityDataSource.cs
- ConnectionProviderAttribute.cs
- BrushConverter.cs
- CodeAssignStatement.cs
- MenuScrollingVisibilityConverter.cs
- BindingOperations.cs
- CertificateReferenceElement.cs
- TemplatePropertyEntry.cs
- DrawToolTipEventArgs.cs
- OperationResponse.cs
- TimeoutException.cs
- MessageSmuggler.cs
- RunClient.cs
- _NegotiateClient.cs
- RowToParametersTransformer.cs
- DEREncoding.cs
- _FtpDataStream.cs
- Int32Collection.cs
- CodeTypeDeclaration.cs
- DataControlLinkButton.cs
- PictureBoxDesigner.cs
- FileChangeNotifier.cs
- Automation.cs
- HtmlInputPassword.cs
- WindowsListViewItemCheckBox.cs
- ApplySecurityAndSendAsyncResult.cs
- DbParameterCollectionHelper.cs
- WmfPlaceableFileHeader.cs
- SoapAttributeOverrides.cs
- TableCellsCollectionEditor.cs
- XamlToRtfParser.cs
- Renderer.cs
- DispatcherSynchronizationContext.cs
- WebAdminConfigurationHelper.cs
- Ipv6Element.cs
- EffectiveValueEntry.cs
- PrinterSettings.cs
- UIHelper.cs
- LoginStatusDesigner.cs
- ColorMatrix.cs
- AutoGeneratedFieldProperties.cs
- XmlIlGenerator.cs
- Helpers.cs
- COM2ExtendedUITypeEditor.cs
- PathSegment.cs
- PropertyIDSet.cs
- GroupBox.cs
- ObjectDataSourceMethodEventArgs.cs
- HttpListenerResponse.cs
- CollectionViewProxy.cs
- RelationshipEndMember.cs
- DataGridViewDataConnection.cs
- Image.cs
- ConnectionStringSettingsCollection.cs
- ImportedNamespaceContextItem.cs
- XslVisitor.cs
- unsafenativemethodsother.cs
- NotifyParentPropertyAttribute.cs
- JavaScriptString.cs
- CryptoProvider.cs
- DeviceContext2.cs
- Point.cs