Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / SMDiagnostics / System / ServiceModel / Diagnostics / ExceptionUtility.cs / 1 / ExceptionUtility.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Diagnostics { using System; using System.Diagnostics; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; using System.Threading; using System.Runtime.Serialization; using System.Reflection; using System.Runtime.CompilerServices; using System.Collections; class ExceptionUtility { const string ExceptionStackAsStringKey = "System.ServiceModel.Diagnostics.ExceptionUtility.ExceptionStackAsString"; #if DEBUG internal static ExceptionUtility mainInstance; #endif DiagnosticTrace diagnosticTrace; string name; string eventSourceName; [ThreadStatic] static Guid activityId; [ThreadStatic] static bool useStaticActivityId; [Obsolete("For SMDiagnostics.dll use only. Call DiagnosticUtility.ExceptionUtility instead")] internal ExceptionUtility(string name, string eventSourceName, object diagnosticTrace) { this.diagnosticTrace = (DiagnosticTrace)diagnosticTrace; this.name = name; this.eventSourceName = eventSourceName; } [Obsolete("For SMDiagnostics.dll use only. Call DiagnosticUtility.ExceptionUtility instead")] [MethodImpl(MethodImplOptions.NoInlining)] #pragma warning disable 56500 internal void TraceFailFast(string message) { EventLogger logger = null; try { #pragma warning disable 618 logger = new EventLogger(this.eventSourceName, this.diagnosticTrace); #pragma warning restore 618 } finally { #pragma warning disable 618 TraceFailFast(message, logger); #pragma warning restore 618 } } // Fail-- Event Log entry will be generated. // To force a Watson on a dev machine, do the following: // 1. Set \HKLM\SOFTWARE\Microsoft\PCHealth\ErrorReporting ForceQueueMode = 0 // 2. In the command environment, set COMPLUS_DbgJitDebugLaunchSetting=0 [Obsolete("For SMDiagnostics.dll use only. Call DiagnosticUtility.ExceptionUtility instead")] [MethodImpl(MethodImplOptions.NoInlining)] internal static void TraceFailFast(string message, EventLogger logger) { try { if (logger != null) { string stackTrace = null; try { stackTrace = new StackTrace().ToString(); } catch (Exception exception) { stackTrace = exception.Message; } finally { logger.LogEvent(TraceEventType.Critical, EventLogCategory.FailFast, EventLogEventId.FailFast, message, stackTrace); } } } catch (Exception e) { if (logger != null) { logger.LogEvent(TraceEventType.Critical, EventLogCategory.FailFast, EventLogEventId.FailFastException, e.ToString()); } throw; } } #pragma warning restore 56500 [Obsolete("For SMDiagnostics.dll use only. Call DiagnosticUtility.ExceptionUtility instead")] internal void TraceFailFastException(Exception exception) { TraceFailFast(exception == null ? null : exception.ToString()); } internal Exception ThrowHelper(Exception exception, TraceEventType eventType, TraceRecord extendedData) { if (this.diagnosticTrace != null) { using (ExceptionUtility.useStaticActivityId ? Activity.CreateActivity(ExceptionUtility.activityId) : null) { this.diagnosticTrace.TraceEvent(eventType, TraceCode.ThrowingException, TraceSR.GetString(TraceSR.ThrowingException), extendedData, exception, null); } } #if DEBUG string stack = exception.StackTrace; #else // Avoid doing this for perf reasons unless we're already taking the hit for tracing. #pragma warning disable 618 string stack = (this.diagnosticTrace != null && this.diagnosticTrace.ShouldTrace(eventType)) ? exception.StackTrace : null; #pragma warning restore 618 #endif if (!string.IsNullOrEmpty(stack)) { IDictionary data = exception.Data; if (data != null && !data.IsReadOnly && !data.IsFixedSize) { object existingString = data[ExceptionStackAsStringKey]; string stackString = existingString == null ? "" : existingString as string; if (stackString != null) { stackString = string.Concat(stackString, stackString.Length == 0 ? "" : Environment.NewLine, "throw", Environment.NewLine, stack, Environment.NewLine, "catch", Environment.NewLine); data[ExceptionStackAsStringKey] = stackString; } } } return exception; } internal Exception ThrowHelper(Exception exception, TraceEventType eventType) { return this.ThrowHelper(exception, eventType, null); } internal ArgumentException ThrowHelperArgument(string message) { return (ArgumentException)this.ThrowHelperError(new ArgumentException(message)); } internal ArgumentException ThrowHelperArgument(string paramName, string message) { return (ArgumentException)this.ThrowHelperError(new ArgumentException(message, paramName)); } internal ArgumentNullException ThrowHelperArgumentNull(string paramName) { return (ArgumentNullException)this.ThrowHelperError(new ArgumentNullException(paramName)); } internal ArgumentNullException ThrowHelperArgumentNull(string paramName, string message) { return (ArgumentNullException)this.ThrowHelperError(new ArgumentNullException(paramName, message)); } internal Exception ThrowHelperFatal(string message, Exception innerException) { return this.ThrowHelperError(new FatalException(message, innerException)); } internal Exception ThrowHelperInternal(bool fatal) { #pragma warning disable 618 AssertUtility.DebugAssert("InternalException should never be thrown."); #pragma warning restore 618 return this.ThrowHelperError(new InternalException(fatal)); } internal Exception ThrowHelperCallback(string message, Exception innerException) { return this.ThrowHelperCritical(new CallbackException(message, innerException)); } internal Exception ThrowHelperCallback(Exception innerException) { return this.ThrowHelperCallback(TraceSR.GetString(TraceSR.GenericCallbackException), innerException); } internal Exception ThrowHelperCritical(Exception exception) { return this.ThrowHelper(exception, TraceEventType.Critical); } internal Exception ThrowHelperError(Exception exception) { return this.ThrowHelper(exception, TraceEventType.Error); } internal Exception ThrowHelperWarning(Exception exception) { return this.ThrowHelper(exception, TraceEventType.Warning); } internal void TraceHandledException(Exception exception, TraceEventType eventType) { if (this.diagnosticTrace != null) { using (ExceptionUtility.useStaticActivityId ? Activity.CreateActivity(ExceptionUtility.activityId) : null) { this.diagnosticTrace.TraceEvent(eventType, TraceCode.TraceHandledException, TraceSR.GetString(TraceSR.TraceHandledException), null, exception, null); } } } // On a single thread, these functions will complete just fine // and don't need to worry about locking issues because the effected // variables are ThreadStatic. internal static void UseActivityId(Guid activityId) { ExceptionUtility.activityId = activityId; ExceptionUtility.useStaticActivityId = true; } internal static void ClearActivityId() { ExceptionUtility.useStaticActivityId = false; ExceptionUtility.activityId = Guid.Empty; } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static bool IsInfrastructureException(Exception exception) { return exception != null && (exception is ThreadAbortException || exception is AppDomainUnloadedException); } internal static bool IsFatal(Exception exception) { InternalException internalException; while (exception != null) { if (exception is FatalException || ((internalException = exception as InternalException) != null && internalException.IsFatal) || (exception is OutOfMemoryException && !(exception is InsufficientMemoryException)) || exception is ThreadAbortException || exception is AccessViolationException || exception is SEHException) { return true; } // These exceptions aren't themselves fatal, but since the CLR uses them to wrap other exceptions, // we want to check to see whether they've been used to wrap a fatal exception. If so, then they // count as fatal. if (exception is TypeInitializationException || exception is TargetInvocationException) { exception = exception.InnerException; } else { break; } } return false; } [Serializable] private class InternalException : SystemException { private bool fatal; public InternalException() { } protected InternalException(SerializationInfo info, StreamingContext context) : base(info, context) { } public InternalException(bool fatal) : base(TraceSR.GetString(TraceSR.InternalException)) { this.fatal = fatal; } public bool IsFatal { get { return this.fatal; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
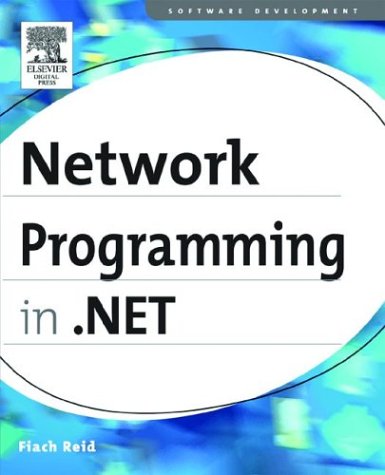
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EnumValidator.cs
- Panel.cs
- TextDecoration.cs
- XhtmlBasicPageAdapter.cs
- ClientTargetSection.cs
- EmptyEnumerable.cs
- ObjectDisposedException.cs
- KoreanLunisolarCalendar.cs
- DataBoundControlAdapter.cs
- SchemaCompiler.cs
- EdmValidator.cs
- ArglessEventHandlerProxy.cs
- BooleanExpr.cs
- WebMessageBodyStyleHelper.cs
- ErrorTableItemStyle.cs
- PrinterUnitConvert.cs
- RectIndependentAnimationStorage.cs
- webeventbuffer.cs
- AdPostCacheSubstitution.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- DoubleLinkList.cs
- CatchBlock.cs
- FontFaceLayoutInfo.cs
- SqlSelectStatement.cs
- SafeFileMappingHandle.cs
- DesignerValidationSummaryAdapter.cs
- RectIndependentAnimationStorage.cs
- CodeExporter.cs
- SplitterCancelEvent.cs
- PolyLineSegmentFigureLogic.cs
- ListControlConvertEventArgs.cs
- OdbcError.cs
- ObjectDataSourceMethodEventArgs.cs
- XamlTreeBuilderBamlRecordWriter.cs
- DirectoryGroupQuery.cs
- WebPartChrome.cs
- DataGridViewRowCollection.cs
- SQLDoubleStorage.cs
- ToolStripSystemRenderer.cs
- SqlResolver.cs
- FontSource.cs
- ApplicationSecurityInfo.cs
- SqlConnectionStringBuilder.cs
- FileRecordSequenceCompletedAsyncResult.cs
- ObjectRef.cs
- SelectionEditingBehavior.cs
- PerformanceCounter.cs
- WindowsRichEdit.cs
- ConfigXmlReader.cs
- RSAPKCS1SignatureDeformatter.cs
- CodeParameterDeclarationExpression.cs
- BatchParser.cs
- coordinatorfactory.cs
- TextTreeFixupNode.cs
- VisualStyleTypesAndProperties.cs
- DataExpression.cs
- Char.cs
- MonikerUtility.cs
- SemaphoreSecurity.cs
- Encoder.cs
- HostVisual.cs
- PerfProviderCollection.cs
- AppDomainEvidenceFactory.cs
- EncoderExceptionFallback.cs
- XsdBuildProvider.cs
- TypeGeneratedEventArgs.cs
- UrlAuthFailedErrorFormatter.cs
- RTLAwareMessageBox.cs
- ToolStripLocationCancelEventArgs.cs
- ColorAnimation.cs
- DirectoryObjectSecurity.cs
- CommandPlan.cs
- OrderPreservingPipeliningMergeHelper.cs
- ColumnWidthChangingEvent.cs
- DataException.cs
- HMACSHA512.cs
- TextAnchor.cs
- SmtpReplyReader.cs
- RectangleHotSpot.cs
- DateTimeFormatInfoScanner.cs
- BamlBinaryWriter.cs
- ZipFileInfoCollection.cs
- ExtenderProviderService.cs
- ImportCatalogPart.cs
- WorkflowWebService.cs
- Image.cs
- SmiTypedGetterSetter.cs
- CreateRefExpr.cs
- SqlProfileProvider.cs
- ImageButton.cs
- TypedRowGenerator.cs
- DesignerCommandAdapter.cs
- ReadOnlyCollectionBase.cs
- MenuItemStyleCollection.cs
- RowToParametersTransformer.cs
- ProfilePropertySettingsCollection.cs
- StateManagedCollection.cs
- MappableObjectManager.cs
- MultipleViewProviderWrapper.cs
- GridViewItemAutomationPeer.cs