Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / Cryptography / RSAPKCS1SignatureFormatter.cs / 1305376 / RSAPKCS1SignatureFormatter.cs
using System.Diagnostics.Contracts; // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // RSAPKCS1SignatureFormatter.cs // namespace System.Security.Cryptography { [System.Runtime.InteropServices.ComVisible(true)] public class RSAPKCS1SignatureFormatter : AsymmetricSignatureFormatter { private RSA _rsaKey; private String _strOID; // // public constructors // public RSAPKCS1SignatureFormatter() {} public RSAPKCS1SignatureFormatter(AsymmetricAlgorithm key) { if (key == null) throw new ArgumentNullException("key"); Contract.EndContractBlock(); _rsaKey = (RSA) key; } // // public methods // public override void SetKey(AsymmetricAlgorithm key) { if (key == null) throw new ArgumentNullException("key"); Contract.EndContractBlock(); _rsaKey = (RSA) key; } public override void SetHashAlgorithm(String strName) { _strOID = CryptoConfig.MapNameToOID(strName); } [System.Security.SecuritySafeCritical] // auto-generated public override byte[] CreateSignature(byte[] rgbHash) { if (rgbHash == null) throw new ArgumentNullException("rgbHash"); Contract.EndContractBlock(); if (_strOID == null) throw new CryptographicUnexpectedOperationException(Environment.GetResourceString("Cryptography_MissingOID")); if (_rsaKey == null) throw new CryptographicUnexpectedOperationException(Environment.GetResourceString("Cryptography_MissingKey")); // Two cases here -- if we are talking to the CSP version or if we are talking to some other RSA provider. if (_rsaKey is RSACryptoServiceProvider) { return ((RSACryptoServiceProvider) _rsaKey).SignHash(rgbHash, _strOID); } else { byte[] pad = Utils.RsaPkcs1Padding(_rsaKey, CryptoConfig.EncodeOID(_strOID), rgbHash); // Create the signature by applying the private key to the padded buffer we just created. return _rsaKey.DecryptValue(pad); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
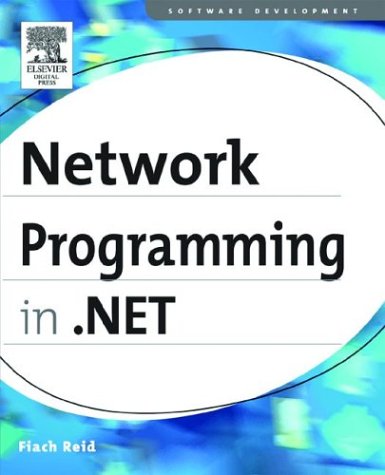
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextControl.cs
- HuffCodec.cs
- Walker.cs
- DesignSurfaceEvent.cs
- Pkcs9Attribute.cs
- BoundPropertyEntry.cs
- ObjectListItem.cs
- PrimitiveSchema.cs
- ConfigurationErrorsException.cs
- ImageConverter.cs
- Stackframe.cs
- DefaultPropertiesToSend.cs
- URLString.cs
- Faults.cs
- XmlSerializationReader.cs
- ManagementObjectSearcher.cs
- MachineKeyConverter.cs
- QilPatternVisitor.cs
- EmissiveMaterial.cs
- VerificationAttribute.cs
- SpotLight.cs
- InputScope.cs
- OutArgument.cs
- Cell.cs
- TdsParserSafeHandles.cs
- DataGridViewColumnDesignTimeVisibleAttribute.cs
- InheritedPropertyChangedEventArgs.cs
- documentsequencetextcontainer.cs
- GridItemProviderWrapper.cs
- Directory.cs
- SiteMapNodeItem.cs
- Debugger.cs
- DataFormats.cs
- CompilerScopeManager.cs
- SHA1.cs
- TraceProvider.cs
- LineMetrics.cs
- RowParagraph.cs
- RequestQueue.cs
- TextFormatterContext.cs
- ISFTagAndGuidCache.cs
- LambdaCompiler.Unary.cs
- IconBitmapDecoder.cs
- Mouse.cs
- DataService.cs
- SimpleWebHandlerParser.cs
- ProtocolsConfiguration.cs
- PopupControlService.cs
- Assert.cs
- XmlElementAttributes.cs
- XmlSortKey.cs
- TemplatePropertyEntry.cs
- DataGridViewMethods.cs
- ComIntegrationManifestGenerator.cs
- DbException.cs
- HtmlElement.cs
- TypeDelegator.cs
- DataGridViewRowsAddedEventArgs.cs
- ObjectManager.cs
- DayRenderEvent.cs
- CodeAttributeArgumentCollection.cs
- MetadataItemCollectionFactory.cs
- BooleanAnimationUsingKeyFrames.cs
- CodeDomDesignerLoader.cs
- DesigntimeLicenseContext.cs
- DocumentReferenceCollection.cs
- HttpModuleActionCollection.cs
- ImageSourceValueSerializer.cs
- DrawListViewSubItemEventArgs.cs
- RoutedEventHandlerInfo.cs
- GraphicsContext.cs
- DataRelationPropertyDescriptor.cs
- HtmlImage.cs
- WebEncodingValidator.cs
- TargetException.cs
- ColumnMap.cs
- XmlParserContext.cs
- XmlElementAttribute.cs
- DeclaredTypeElement.cs
- Keyboard.cs
- ToolStripStatusLabel.cs
- Relationship.cs
- ToolStripManager.cs
- SystemIPGlobalStatistics.cs
- dbenumerator.cs
- SamlSubject.cs
- _AuthenticationState.cs
- ExeConfigurationFileMap.cs
- SecurityTokenParametersEnumerable.cs
- KeyToListMap.cs
- XmlDownloadManager.cs
- ImageField.cs
- HWStack.cs
- AdornerDecorator.cs
- LongTypeConverter.cs
- DataGridViewColumnEventArgs.cs
- GeometryGroup.cs
- DataGridViewColumnEventArgs.cs
- PositiveTimeSpanValidatorAttribute.cs
- StreamGeometryContext.cs