Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / XmlUtils / System / Xml / Xsl / QIL / WhitespaceRule.cs / 5 / WhitespaceRule.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System.Diagnostics; using System.IO; using System.Xml.Xsl.Runtime; namespace System.Xml.Xsl.Qil { ////// Data structure for storing whitespace rules generated by xsl:strip-space and xsl:preserve-space /// internal class WhitespaceRule { private string localName; private string namespaceName; private bool preserveSpace; ////// Allow derived classes to construct empty whitespace rule. /// protected WhitespaceRule() { } ////// Construct new whitespace rule. /// public WhitespaceRule(string localName, string namespaceName, bool preserveSpace) { Init(localName, namespaceName, preserveSpace); } ////// Initialize whitespace rule after it's been constructed. /// protected void Init(string localName, string namespaceName, bool preserveSpace) { this.localName = localName; this.namespaceName = namespaceName; this.preserveSpace = preserveSpace; } ////// Local name of the element. /// public string LocalName { get { return localName; } set { localName = value; } } ////// Namespace name (uri) of the element. /// public string NamespaceName { get { return namespaceName; } set { namespaceName = value; } } ////// True, if this element is whitespace-preserving. /// False, if this element is whitespace-stripping. /// public bool PreserveSpace { get { return preserveSpace; } } ////// Serialize the object to BinaryWriter. /// public void GetObjectData(XmlQueryDataWriter writer) { Debug.Assert(this.GetType() == typeof(WhitespaceRule), "Serialization of WhitespaceRule subclasses is not implemented"); // string localName; writer.WriteStringQ(localName); // string namespaceName; writer.WriteStringQ(namespaceName); // bool preserveSpace; writer.Write(preserveSpace); } ////// Deserialize the object from BinaryReader. /// public WhitespaceRule(XmlQueryDataReader reader) { // string localName; this.localName = reader.ReadStringQ(); // string namespaceName; this.namespaceName = reader.ReadStringQ(); // bool preserveSpace; this.preserveSpace = reader.ReadBoolean(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System.Diagnostics; using System.IO; using System.Xml.Xsl.Runtime; namespace System.Xml.Xsl.Qil { ////// Data structure for storing whitespace rules generated by xsl:strip-space and xsl:preserve-space /// internal class WhitespaceRule { private string localName; private string namespaceName; private bool preserveSpace; ////// Allow derived classes to construct empty whitespace rule. /// protected WhitespaceRule() { } ////// Construct new whitespace rule. /// public WhitespaceRule(string localName, string namespaceName, bool preserveSpace) { Init(localName, namespaceName, preserveSpace); } ////// Initialize whitespace rule after it's been constructed. /// protected void Init(string localName, string namespaceName, bool preserveSpace) { this.localName = localName; this.namespaceName = namespaceName; this.preserveSpace = preserveSpace; } ////// Local name of the element. /// public string LocalName { get { return localName; } set { localName = value; } } ////// Namespace name (uri) of the element. /// public string NamespaceName { get { return namespaceName; } set { namespaceName = value; } } ////// True, if this element is whitespace-preserving. /// False, if this element is whitespace-stripping. /// public bool PreserveSpace { get { return preserveSpace; } } ////// Serialize the object to BinaryWriter. /// public void GetObjectData(XmlQueryDataWriter writer) { Debug.Assert(this.GetType() == typeof(WhitespaceRule), "Serialization of WhitespaceRule subclasses is not implemented"); // string localName; writer.WriteStringQ(localName); // string namespaceName; writer.WriteStringQ(namespaceName); // bool preserveSpace; writer.Write(preserveSpace); } ////// Deserialize the object from BinaryReader. /// public WhitespaceRule(XmlQueryDataReader reader) { // string localName; this.localName = reader.ReadStringQ(); // string namespaceName; this.namespaceName = reader.ReadStringQ(); // bool preserveSpace; this.preserveSpace = reader.ReadBoolean(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
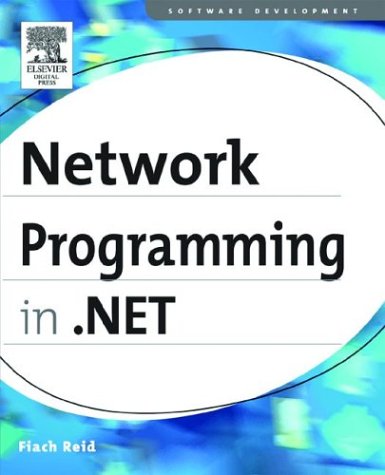
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConnectionManagementSection.cs
- Rijndael.cs
- PreDigestedSignedInfo.cs
- RepeaterItemEventArgs.cs
- SuppressMessageAttribute.cs
- AppSettingsExpressionBuilder.cs
- DrawingState.cs
- DataGridItemEventArgs.cs
- ExpressionVisitor.cs
- SqlNamer.cs
- StrokeIntersection.cs
- COM2ExtendedBrowsingHandler.cs
- ByteStorage.cs
- OneWayChannelListener.cs
- AssociatedControlConverter.cs
- AsyncResult.cs
- XmlSchemaComplexContentRestriction.cs
- SecurityCriticalDataForSet.cs
- DateTimeOffsetAdapter.cs
- PropertyOverridesDialog.cs
- Substitution.cs
- ClientTargetSection.cs
- PersonalizationStateInfo.cs
- WmlSelectionListAdapter.cs
- DataGridViewComboBoxEditingControl.cs
- AdornerPresentationContext.cs
- _ConnectOverlappedAsyncResult.cs
- VerticalAlignConverter.cs
- HtmlShim.cs
- TextServicesCompartmentContext.cs
- EncodingInfo.cs
- ProfileProvider.cs
- UIElement.cs
- WindowsProgressbar.cs
- SchemaLookupTable.cs
- MarkedHighlightComponent.cs
- LinqDataSourceValidationException.cs
- WpfKnownMember.cs
- SemanticBasicElement.cs
- TraceSwitch.cs
- AutoResizedEvent.cs
- GeneralTransform3DTo2D.cs
- SQLBytes.cs
- PopupControlService.cs
- MailMessageEventArgs.cs
- StringConverter.cs
- MailWebEventProvider.cs
- SpotLight.cs
- ListViewUpdateEventArgs.cs
- ActiveDesignSurfaceEvent.cs
- ProgressPage.cs
- ContextBase.cs
- InkCanvasFeedbackAdorner.cs
- SemanticBasicElement.cs
- TextRunCache.cs
- Enumerable.cs
- DataViewSetting.cs
- Buffer.cs
- PerfCounters.cs
- DeliveryRequirementsAttribute.cs
- XPathMessageFilterElement.cs
- SerializationTrace.cs
- TypeLibConverter.cs
- PropertyDescriptor.cs
- Zone.cs
- ControlBindingsConverter.cs
- ExtensionMethods.cs
- FilterableAttribute.cs
- ProcessProtocolHandler.cs
- UrlPropertyAttribute.cs
- ToolTipService.cs
- CAGDesigner.cs
- CommonProperties.cs
- EntityContainerEntitySet.cs
- OperatingSystem.cs
- FormsAuthentication.cs
- HttpRuntime.cs
- DataSourceConverter.cs
- TextDecorations.cs
- LicenseContext.cs
- DataListItemEventArgs.cs
- ProfileEventArgs.cs
- InputBinder.cs
- DependencyObject.cs
- WindowInteractionStateTracker.cs
- ExpressionEditorSheet.cs
- TextServicesLoader.cs
- ComplexPropertyEntry.cs
- RandomNumberGenerator.cs
- ChannelBuilder.cs
- DataColumnMappingCollection.cs
- HiddenField.cs
- TerminatorSinks.cs
- EasingFunctionBase.cs
- MultipleViewPatternIdentifiers.cs
- ScriptIgnoreAttribute.cs
- FirstMatchCodeGroup.cs
- ProgressBarRenderer.cs
- LinkLabelLinkClickedEvent.cs
- MeasurementDCInfo.cs