Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebControls / HiddenField.cs / 1 / HiddenField.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System.ComponentModel; using System.Security.Permissions; using System.Collections.Specialized; ////// Inserts a hidden field into the web form. /// [ ControlValueProperty("Value"), DefaultEvent("ValueChanged"), DefaultProperty("Value"), Designer("System.Web.UI.Design.WebControls.HiddenFieldDesigner, " + AssemblyRef.SystemDesign), ParseChildren(true), PersistChildren(false), NonVisualControl(), SupportsEventValidation, ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class HiddenField : Control, IPostBackDataHandler { private static readonly object EventValueChanged = new object(); [ DefaultValue(false), EditorBrowsable(EditorBrowsableState.Never), ] public override bool EnableTheming { get { return false; } set { throw new NotSupportedException(SR.GetString(SR.NoThemingSupport, this.GetType().Name)); } } [ DefaultValue(""), EditorBrowsable(EditorBrowsableState.Never), ] public override string SkinID { get { return String.Empty; } set { throw new NotSupportedException(SR.GetString(SR.NoThemingSupport, this.GetType().Name)); } } ////// Gets or sets the value of the hidden field. /// [ Bindable(true), WebCategory("Behavior"), DefaultValue(""), WebSysDescription(SR.HiddenField_Value) ] public virtual string Value { get { string s = (string)ViewState["Value"]; return (s != null) ? s : String.Empty; } set { ViewState["Value"] = value; } } ////// Raised when the value of the hidden field is changed on the client. /// [ WebCategory("Action"), WebSysDescription(SR.HiddenField_OnValueChanged) ] public event EventHandler ValueChanged { add { Events.AddHandler(EventValueChanged, value); } remove { Events.RemoveHandler(EventValueChanged, value); } } protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } [ EditorBrowsable(EditorBrowsableState.Never), ] public override void Focus() { throw new NotSupportedException(SR.GetString(SR.NoFocusSupport, this.GetType().Name)); } protected virtual bool LoadPostData(string postDataKey, NameValueCollection postCollection) { ValidateEvent(UniqueID); string current = Value; string postData = postCollection[postDataKey]; if (!current.Equals(postData, StringComparison.Ordinal)) { Value = postData; return true; } return false; } protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); if (SaveValueViewState == false) { ViewState.SetItemDirty("Value", false); } } protected virtual void OnValueChanged(EventArgs e) { EventHandler handler = (EventHandler)Events[EventValueChanged]; if (handler != null) { handler(this, e); } } protected virtual void RaisePostDataChangedEvent() { OnValueChanged(EventArgs.Empty); } protected internal override void Render(HtmlTextWriter writer) { string uniqueID = UniqueID; // Make sure we are in a form tag with runat=server. if (Page != null) { Page.VerifyRenderingInServerForm(this); Page.ClientScript.RegisterForEventValidation(uniqueID); } writer.AddAttribute(HtmlTextWriterAttribute.Type, "hidden"); if (uniqueID != null) { writer.AddAttribute(HtmlTextWriterAttribute.Name, uniqueID); } if (ID != null) { writer.AddAttribute(HtmlTextWriterAttribute.Id, ClientID); } string s; s = Value; if (s.Length > 0) { writer.AddAttribute(HtmlTextWriterAttribute.Value, s); } writer.RenderBeginTag(HtmlTextWriterTag.Input); writer.RenderEndTag(); } ////// Determines whether the Value must be stored in view state, to /// optimize the size of the saved state. /// private bool SaveValueViewState { get { // Must be saved when // 1. There is a registered event handler for EventValueChanged // 2. Control is not visible, because the browser's post data will not include this control // 3. The instance is a derived instance, which might be overriding the OnValueChanged method if ((Events[EventValueChanged] != null) || (Visible == false) || (this.GetType() != typeof(HiddenField))) { return true; } return false; } } #region Implementation of IPostBackDataHandler bool IPostBackDataHandler.LoadPostData(string postDataKey, NameValueCollection postCollection) { return LoadPostData(postDataKey, postCollection); } void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } #endregion } }
Link Menu
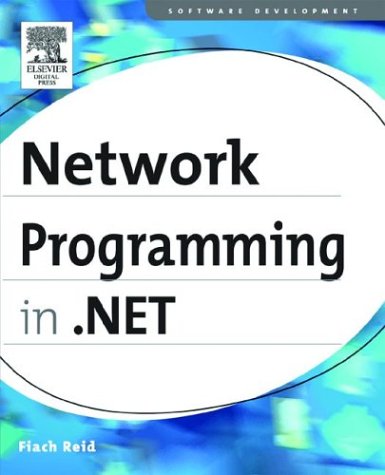
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListViewGroupConverter.cs
- Matrix3DConverter.cs
- PagedDataSource.cs
- SessionState.cs
- ProxyWebPartManager.cs
- PropertyGeneratedEventArgs.cs
- AutomationProperty.cs
- ByteStorage.cs
- DataGridViewComboBoxColumnDesigner.cs
- DefaultTypeArgumentAttribute.cs
- GACIdentityPermission.cs
- DesignerValidationSummaryAdapter.cs
- X509IssuerSerialKeyIdentifierClause.cs
- GeneralTransform3DTo2D.cs
- MergeFilterQuery.cs
- CrossAppDomainChannel.cs
- InternalEnumValidator.cs
- PropertyState.cs
- externdll.cs
- DataColumnSelectionConverter.cs
- CodeDomComponentSerializationService.cs
- MailBnfHelper.cs
- EventTrigger.cs
- MiniModule.cs
- TextFormattingConverter.cs
- MessageHeader.cs
- DataServiceBuildProvider.cs
- ClickablePoint.cs
- SparseMemoryStream.cs
- BackgroundFormatInfo.cs
- InvalidFilterCriteriaException.cs
- ToolStripContainer.cs
- QueueProcessor.cs
- IDQuery.cs
- FunctionImportElement.cs
- ObjectTag.cs
- StrokeDescriptor.cs
- BehaviorEditorPart.cs
- SelectionRangeConverter.cs
- ZipIOLocalFileHeader.cs
- WinFormsSecurity.cs
- Literal.cs
- ExpressionBuilder.cs
- ChtmlSelectionListAdapter.cs
- basenumberconverter.cs
- SecurityStandardsManager.cs
- TreeNodeBindingCollection.cs
- PasswordTextNavigator.cs
- EntityDataSourceStatementEditor.cs
- DrawingBrush.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- CharacterMetricsDictionary.cs
- MsmqUri.cs
- ToolStripOverflow.cs
- SemaphoreSecurity.cs
- ProfileSettings.cs
- HybridDictionary.cs
- PreviewKeyDownEventArgs.cs
- CodeDirectoryCompiler.cs
- DirectionalLight.cs
- HttpUnhandledOperationInvoker.cs
- RecipientInfo.cs
- BitConverter.cs
- InkPresenter.cs
- OdbcConnection.cs
- SqlBulkCopyColumnMappingCollection.cs
- DropShadowEffect.cs
- BitmapPalettes.cs
- InstalledFontCollection.cs
- AppSettingsSection.cs
- GridViewSortEventArgs.cs
- ScrollProperties.cs
- AttributeTableBuilder.cs
- Point3DCollection.cs
- SkewTransform.cs
- WindowsTab.cs
- TypeReference.cs
- TextServicesPropertyRanges.cs
- IItemContainerGenerator.cs
- ImplicitInputBrush.cs
- FileDialogCustomPlace.cs
- ResolvedKeyFrameEntry.cs
- SequentialUshortCollection.cs
- SqlDataSourceCustomCommandPanel.cs
- GenericTypeParameterBuilder.cs
- StylusPoint.cs
- Calendar.cs
- MetadataArtifactLoaderFile.cs
- AmbientValueAttribute.cs
- CancelAsyncOperationRequest.cs
- securestring.cs
- SessionState.cs
- ArrayConverter.cs
- Bits.cs
- ToolStripItemTextRenderEventArgs.cs
- MSAAEventDispatcher.cs
- WindowsTreeView.cs
- MarkerProperties.cs
- ScrollEvent.cs