Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / MS / Internal / Ink / StrokeIntersection.cs / 1 / StrokeIntersection.cs
//------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using MS.Internal; using MS.Internal.Ink; using MS.Utility; using System; using System.Windows; using System.Collections.Generic; using System.Globalization; namespace System.Windows.Ink { ////// A helper struct that represents a fragment of a stroke spine. /// internal struct StrokeIntersection { #region Private statics private static StrokeIntersection s_empty = new StrokeIntersection(AfterLast, AfterLast, BeforeFirst, BeforeFirst); private static StrokeIntersection s_full = new StrokeIntersection(BeforeFirst, BeforeFirst, AfterLast, AfterLast); #endregion #region Public API ////// BeforeFirst /// ///internal static double BeforeFirst { get { return StrokeFIndices.BeforeFirst; } } /// /// AfterLast /// ///internal static double AfterLast { get { return StrokeFIndices.AfterLast; } } /// /// Constructor /// /// /// /// /// internal StrokeIntersection(double hitBegin, double inBegin, double inEnd, double hitEnd) { //ISSUE-2004/12/06-XiaoTu: should we validate the input? _hitSegment = new StrokeFIndices(hitBegin, hitEnd); _inSegment = new StrokeFIndices(inBegin, inEnd); } ////// hitBeginFIndex /// ///internal double HitBegin { set { _hitSegment.BeginFIndex = value; } } /// /// hitEndFIndex /// ///internal double HitEnd { get { return _hitSegment.EndFIndex; } set { _hitSegment.EndFIndex = value; } } /// /// InBegin /// ///internal double InBegin { get { return _inSegment.BeginFIndex; } set { _inSegment.BeginFIndex = value; } } /// /// InEnd /// ///internal double InEnd { get { return _inSegment.EndFIndex; } set { _inSegment.EndFIndex = value; } } /// /// ToString /// public override string ToString() { return "{" + StrokeFIndices.GetStringRepresentation(_hitSegment.BeginFIndex) + "," + StrokeFIndices.GetStringRepresentation(_inSegment.BeginFIndex) + "," + StrokeFIndices.GetStringRepresentation(_inSegment.EndFIndex) + "," + StrokeFIndices.GetStringRepresentation(_hitSegment.EndFIndex) + "}"; } ////// Equals /// /// ///public override bool Equals(Object obj) { // Check for null and compare run-time types if (obj == null || GetType() != obj.GetType()) return false; return ((StrokeIntersection)obj == this); } /// /// GetHashCode /// ///public override int GetHashCode() { return _hitSegment.GetHashCode() ^ _inSegment.GetHashCode(); } /// /// operator == /// /// /// ///public static bool operator ==(StrokeIntersection left, StrokeIntersection right) { return (left._hitSegment == right._hitSegment && left._inSegment == right._inSegment); } /// /// operator != /// /// /// ///public static bool operator !=(StrokeIntersection left, StrokeIntersection right) { return !(left == right); } #endregion #region Internal API /// /// /// internal static StrokeIntersection Full { get { return s_full; } } ////// /// internal bool IsEmpty { get { return _hitSegment.IsEmpty; } } ////// /// internal StrokeFIndices HitSegment { get { return _hitSegment; } } ////// /// internal StrokeFIndices InSegment { get { return _inSegment; } } #endregion #region Internal static methods ////// Get the "in-segments" of the intersections. /// internal static StrokeFIndices[] GetInSegments(StrokeIntersection[] intersections) { System.Diagnostics.Debug.Assert(intersections != null); System.Diagnostics.Debug.Assert(intersections.Length > 0); ListinFIndices = new List (intersections.Length); for (int j = 0; j < intersections.Length; j++) { System.Diagnostics.Debug.Assert(!intersections[j].IsEmpty); if (!intersections[j].InSegment.IsEmpty) { if (inFIndices.Count > 0 && inFIndices[inFIndices.Count - 1].EndFIndex >= intersections[j].InSegment.BeginFIndex) { //merge StrokeFIndices sfiPrevious = inFIndices[inFIndices.Count - 1]; sfiPrevious.EndFIndex = intersections[j].InSegment.EndFIndex; inFIndices[inFIndices.Count - 1] = sfiPrevious; } else { inFIndices.Add(intersections[j].InSegment); } } } return inFIndices.ToArray(); } /// /// Get the "hit-segments" /// internal static StrokeFIndices[] GetHitSegments(StrokeIntersection[] intersections) { System.Diagnostics.Debug.Assert(intersections != null); System.Diagnostics.Debug.Assert(intersections.Length > 0); ListhitFIndices = new List (intersections.Length); for (int j = 0; j < intersections.Length; j++) { System.Diagnostics.Debug.Assert(!intersections[j].IsEmpty); if (!intersections[j].HitSegment.IsEmpty) { if (hitFIndices.Count > 0 && hitFIndices[hitFIndices.Count - 1].EndFIndex >= intersections[j].HitSegment.BeginFIndex) { //merge StrokeFIndices sfiPrevious = hitFIndices[hitFIndices.Count - 1]; sfiPrevious.EndFIndex = intersections[j].HitSegment.EndFIndex; hitFIndices[hitFIndices.Count - 1] = sfiPrevious; } else { hitFIndices.Add(intersections[j].HitSegment); } } } return hitFIndices.ToArray(); } #endregion #region Fields private StrokeFIndices _hitSegment; private StrokeFIndices _inSegment; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using MS.Internal; using MS.Internal.Ink; using MS.Utility; using System; using System.Windows; using System.Collections.Generic; using System.Globalization; namespace System.Windows.Ink { ////// A helper struct that represents a fragment of a stroke spine. /// internal struct StrokeIntersection { #region Private statics private static StrokeIntersection s_empty = new StrokeIntersection(AfterLast, AfterLast, BeforeFirst, BeforeFirst); private static StrokeIntersection s_full = new StrokeIntersection(BeforeFirst, BeforeFirst, AfterLast, AfterLast); #endregion #region Public API ////// BeforeFirst /// ///internal static double BeforeFirst { get { return StrokeFIndices.BeforeFirst; } } /// /// AfterLast /// ///internal static double AfterLast { get { return StrokeFIndices.AfterLast; } } /// /// Constructor /// /// /// /// /// internal StrokeIntersection(double hitBegin, double inBegin, double inEnd, double hitEnd) { //ISSUE-2004/12/06-XiaoTu: should we validate the input? _hitSegment = new StrokeFIndices(hitBegin, hitEnd); _inSegment = new StrokeFIndices(inBegin, inEnd); } ////// hitBeginFIndex /// ///internal double HitBegin { set { _hitSegment.BeginFIndex = value; } } /// /// hitEndFIndex /// ///internal double HitEnd { get { return _hitSegment.EndFIndex; } set { _hitSegment.EndFIndex = value; } } /// /// InBegin /// ///internal double InBegin { get { return _inSegment.BeginFIndex; } set { _inSegment.BeginFIndex = value; } } /// /// InEnd /// ///internal double InEnd { get { return _inSegment.EndFIndex; } set { _inSegment.EndFIndex = value; } } /// /// ToString /// public override string ToString() { return "{" + StrokeFIndices.GetStringRepresentation(_hitSegment.BeginFIndex) + "," + StrokeFIndices.GetStringRepresentation(_inSegment.BeginFIndex) + "," + StrokeFIndices.GetStringRepresentation(_inSegment.EndFIndex) + "," + StrokeFIndices.GetStringRepresentation(_hitSegment.EndFIndex) + "}"; } ////// Equals /// /// ///public override bool Equals(Object obj) { // Check for null and compare run-time types if (obj == null || GetType() != obj.GetType()) return false; return ((StrokeIntersection)obj == this); } /// /// GetHashCode /// ///public override int GetHashCode() { return _hitSegment.GetHashCode() ^ _inSegment.GetHashCode(); } /// /// operator == /// /// /// ///public static bool operator ==(StrokeIntersection left, StrokeIntersection right) { return (left._hitSegment == right._hitSegment && left._inSegment == right._inSegment); } /// /// operator != /// /// /// ///public static bool operator !=(StrokeIntersection left, StrokeIntersection right) { return !(left == right); } #endregion #region Internal API /// /// /// internal static StrokeIntersection Full { get { return s_full; } } ////// /// internal bool IsEmpty { get { return _hitSegment.IsEmpty; } } ////// /// internal StrokeFIndices HitSegment { get { return _hitSegment; } } ////// /// internal StrokeFIndices InSegment { get { return _inSegment; } } #endregion #region Internal static methods ////// Get the "in-segments" of the intersections. /// internal static StrokeFIndices[] GetInSegments(StrokeIntersection[] intersections) { System.Diagnostics.Debug.Assert(intersections != null); System.Diagnostics.Debug.Assert(intersections.Length > 0); ListinFIndices = new List (intersections.Length); for (int j = 0; j < intersections.Length; j++) { System.Diagnostics.Debug.Assert(!intersections[j].IsEmpty); if (!intersections[j].InSegment.IsEmpty) { if (inFIndices.Count > 0 && inFIndices[inFIndices.Count - 1].EndFIndex >= intersections[j].InSegment.BeginFIndex) { //merge StrokeFIndices sfiPrevious = inFIndices[inFIndices.Count - 1]; sfiPrevious.EndFIndex = intersections[j].InSegment.EndFIndex; inFIndices[inFIndices.Count - 1] = sfiPrevious; } else { inFIndices.Add(intersections[j].InSegment); } } } return inFIndices.ToArray(); } /// /// Get the "hit-segments" /// internal static StrokeFIndices[] GetHitSegments(StrokeIntersection[] intersections) { System.Diagnostics.Debug.Assert(intersections != null); System.Diagnostics.Debug.Assert(intersections.Length > 0); ListhitFIndices = new List (intersections.Length); for (int j = 0; j < intersections.Length; j++) { System.Diagnostics.Debug.Assert(!intersections[j].IsEmpty); if (!intersections[j].HitSegment.IsEmpty) { if (hitFIndices.Count > 0 && hitFIndices[hitFIndices.Count - 1].EndFIndex >= intersections[j].HitSegment.BeginFIndex) { //merge StrokeFIndices sfiPrevious = hitFIndices[hitFIndices.Count - 1]; sfiPrevious.EndFIndex = intersections[j].HitSegment.EndFIndex; hitFIndices[hitFIndices.Count - 1] = sfiPrevious; } else { hitFIndices.Add(intersections[j].HitSegment); } } } return hitFIndices.ToArray(); } #endregion #region Fields private StrokeFIndices _hitSegment; private StrokeFIndices _inSegment; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
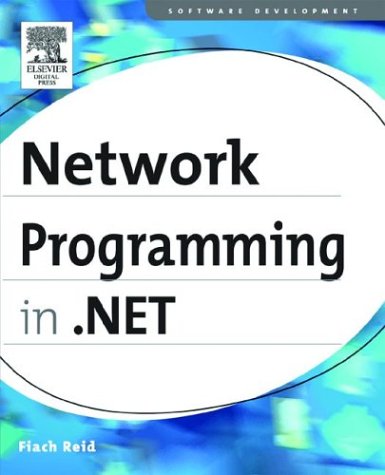
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FileVersionInfo.cs
- ToolStripButton.cs
- HttpPostedFileBase.cs
- StandardBindingReliableSessionElement.cs
- SocketException.cs
- TextRangeBase.cs
- DesignerActionUIService.cs
- InsufficientMemoryException.cs
- Authorization.cs
- FixedMaxHeap.cs
- OracleFactory.cs
- EventWaitHandle.cs
- New.cs
- DataContractSerializer.cs
- ProxyWebPart.cs
- UInt32Converter.cs
- RegexInterpreter.cs
- Queue.cs
- StringValidatorAttribute.cs
- Geometry3D.cs
- RequestCacheValidator.cs
- StickyNoteAnnotations.cs
- PowerStatus.cs
- SQLByte.cs
- AuthenticationModulesSection.cs
- bindurihelper.cs
- baseaxisquery.cs
- AdornedElementPlaceholder.cs
- BindingMAnagerBase.cs
- BindUriHelper.cs
- WorkflowElementDialog.cs
- NetworkCredential.cs
- LightweightEntityWrapper.cs
- CacheVirtualItemsEvent.cs
- Sorting.cs
- AsymmetricSecurityBindingElement.cs
- HtmlToClrEventProxy.cs
- CompilerError.cs
- Compiler.cs
- BamlStream.cs
- ToolBarButtonClickEvent.cs
- OleDbStruct.cs
- BrowserTree.cs
- ImmutableCollection.cs
- DbCommandTree.cs
- DataColumnPropertyDescriptor.cs
- AnnotationObservableCollection.cs
- DeadCharTextComposition.cs
- InternalConfigHost.cs
- NativeMethods.cs
- XPathSelfQuery.cs
- TextTreeObjectNode.cs
- XPathNodeList.cs
- EntryPointNotFoundException.cs
- RijndaelManaged.cs
- OutputCacheModule.cs
- CompositionCommandSet.cs
- DateTimeFormat.cs
- NumberSubstitution.cs
- RootBrowserWindow.cs
- BamlResourceDeserializer.cs
- RTTrackingProfile.cs
- ZipPackagePart.cs
- ResXDataNode.cs
- HostedTcpTransportManager.cs
- PatternMatcher.cs
- AdvancedBindingPropertyDescriptor.cs
- GroupItemAutomationPeer.cs
- DesignerActionList.cs
- HostingEnvironmentSection.cs
- WebPartConnectionsDisconnectVerb.cs
- CompositeTypefaceMetrics.cs
- EditorPartChrome.cs
- PixelFormats.cs
- DrawingContext.cs
- HasActivatableWorkflowEvent.cs
- HotSpot.cs
- SqlClientPermission.cs
- SqlConnectionPoolProviderInfo.cs
- HebrewCalendar.cs
- CustomBindingElement.cs
- BaseUriHelper.cs
- InputProviderSite.cs
- ScaleTransform3D.cs
- NavigationProperty.cs
- PngBitmapDecoder.cs
- HostProtectionException.cs
- SystemWebExtensionsSectionGroup.cs
- TypeDescriptorFilterService.cs
- GenericAuthenticationEventArgs.cs
- EntryWrittenEventArgs.cs
- KnownTypes.cs
- InstanceKeyView.cs
- RepeaterCommandEventArgs.cs
- WebPartCollection.cs
- DefaultValidator.cs
- ToolStripMenuItemDesigner.cs
- XmlNullResolver.cs
- FormViewInsertedEventArgs.cs
- SchemaTableOptionalColumn.cs