Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / DbCommandTree.cs / 2 / DbCommandTree.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Common; using System.Globalization; using System.IO; using System.Diagnostics; using System.Text; using System.Text.RegularExpressions; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; namespace System.Data.Common.CommandTrees { ////// DbCommandTree is the abstract base type for the Delete, Query, Insert and Update DbCommandTree types. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbCommandTree { #region Private Instance Fields // Metadata collection private MetadataWorkspace _metadata; private DataSpace _dataSpace; // Commonly used types private CommandTreeTypeHelper _typeHelp; // CreateParameter Support private Dictionary> _paramMappings = new Dictionary >(StringComparer.OrdinalIgnoreCase); // Pre-built Expressions DbConstantExpression _trueExpr; DbConstantExpression _falseExpr; // Validation support private int _currentVersion = 1; private int _validatedVersion; // Referenced Container Tracking private List _containers = new List (); // Binding alias generation private AliasGenerator _bindingAliases; internal AliasGenerator BindingAliases { get { if (null == _bindingAliases) { _bindingAliases = new AliasGenerator(string.Format(CultureInfo.InvariantCulture, "Var_{0}_", this.ObjectId), 0); } return _bindingAliases; } } private static int s_instanceCount; // Bid counter internal readonly int ObjectId = System.Threading.Interlocked.Increment(ref s_instanceCount); #endregion #region Constructors /// /// Initializes a new command tree with a given metadata workspace. /// /// The metadata workspace against which the command tree should operate. /// The logical 'space' that metadata in the expressions used in this command tree must belong to. ////// or is null internal DbCommandTree(MetadataWorkspace metadata, DataSpace dataSpace) { using (new EntityBid.ScopeAuto(" does not represent a valid data space %d#", this.ObjectId)) { if (null == metadata) { throw EntityUtil.ArgumentNull("metadata"); } // // Ensure that this is a valid value, in addition to non-null // if (!CommandTreeUtils.IsValidDataSpace(dataSpace)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_CommandTree_InvalidDataSpace, "dataSpace"); } // // Assign the metadata workspace and initalize commonly used types. // _metadata = new MetadataWorkspace(); ItemCollection objectItemCollection; //While EdmItemCollection and StorageitemCollections are required //ObjectItemCollection may or may not be registered on the workspace yet. //So register the ObjectItemCollection if it exists. if (metadata.TryGetItemCollection(DataSpace.OSpace, out objectItemCollection)) { _metadata.RegisterItemCollection(objectItemCollection); } _metadata.RegisterItemCollection(metadata.GetItemCollection(DataSpace.CSpace)); _metadata.RegisterItemCollection(metadata.GetItemCollection(DataSpace.CSSpace)); _metadata.RegisterItemCollection(metadata.GetItemCollection(DataSpace.SSpace)); _dataSpace = dataSpace; _typeHelp = new CommandTreeTypeHelper(this); } } #endregion #region Public API /// /// Clones the specified /// The expression to import ///in the context of this command tree, returning a copy of the expression that is owned by this command tree. If the expression is the root of an expression subtree, the entire subtree is cloned. If the expression is already owned by this command tree the original expression is returned. /// If the expression is already owned by this command tree, the original expression. Otherwise, a copy of the expression that is owned by this command tree ////// is null /*CQT_PUBLIC_API(*/internal/*)*/ DbExpression Import(DbExpression source) { if (null == source) { throw EntityUtil.ArgumentNull("source"); } // #433613: PreSharp warning 56506: Parameter 'source.CommandTree' to this public method must be validated: A null-dereference can occur here. if (null == source.CommandTree) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_CommandTree_Import_NullCommandTreeInvalid, "source"); } using(new EntityBid.ScopeAuto(" refers to metadata that is not present in the metadata workspace of this command tree %d#", this.ObjectId)) { EntityBid.Trace(" %d#, source=%d#, %d{cqt.DbExpressionKind}\n", this.ObjectId, DbExpression.GetObjectId(source), DbExpression.GetExpressionKind(source)); if (this == source.CommandTree) { return source; } // Command Trees should only ever exist in one Data Space, the C-Space. //Debug.Assert(source.CommandTree.DataSpace == this.DataSpace, "DbCommandTree DataSpace mismatch"); return ExpressionCopier.Copy(this, source); } } /// /// Verifies that the command tree is valid, including the validity of variable and parameter references. /// ///If the command tree is invalid /*CQT_PUBLIC_API(*/internal/*)*/ void Validate() { using(new EntityBid.ScopeAuto("%d#", this.ObjectId)) { if (!this.ValidationRequired) { return; } this.Validate(new System.Data.Common.CommandTrees.Internal.Validator()); this.SetValid(); } } /// /// Adds a parameter to the set of parameters that may be referenced within this command tree. If a parameter with the same name but a different type already exists on this command tree then an exception will be thrown. /// /// The name of the new parameter /// A description of the type of the new parameter ////// or is null A parameter with the same name but a different type already exists on this command tree. /*CQT_PUBLIC_API(*/internal/*)*/ void AddParameter(string name, TypeUsage type) { EntityUtil.CheckArgumentNull(name, "name"); this.TypeHelper.CheckType(type); using(new EntityBid.ScopeAuto("%d#", this.ObjectId)) { EntityBid.Trace(" %d#, name='%ls'\n", this.ObjectId, name); EntityBid.Trace(" %d#, type='%ls'\n", this.ObjectId, TypeHelpers.GetStrongName(type)); if (!DbCommandTree.IsValidParameterName(name)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_CommandTree_InvalidParameterName(name), "name"); } KeyValuePair foundParam; if (_paramMappings.TryGetValue(name, out foundParam)) { // SQLBUDT#545720: Equivalence is not a sufficient check (consider row types for TVPs) - equality is required. if (!TypeSemantics.IsStructurallyEqualTo(type, foundParam.Value)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_CommandTree_ParameterExists, "type"); } } else { _paramMappings.Add(name, new KeyValuePair (name, type)); } } } /// /// Gets the name and corresponding type of each parameter that can be referenced within this command tree. /// public IEnumerable> Parameters { get { using(new EntityBid.ScopeAuto(" %d#", this.ObjectId)) { Dictionary retParams = new Dictionary (); foreach (KeyValuePair definedParam in _paramMappings.Values) { EntityBid.Trace(" %d#, name='%ls'\n", this.ObjectId, definedParam.Key); EntityBid.Trace(" %d#, type='%ls'\n", this.ObjectId, TypeHelpers.GetStrongName(definedParam.Value)); retParams.Add(definedParam.Key, definedParam.Value); } return retParams; } } } // VersionNumber need only be public when Command Trees are mutable. #if PUBLIC_COMMANDTREE_VERSIONNUMBER /// /// Gets the current version of this DbCommandTree. /// public int VersionNumber { get { return _currentVersion; } } #endif #region Non-DbExpression Factory Methods ////// Creates a new /// The expression to bind /// The variable name that should be used for the binding ///that uses the specified variable name to bind the given expression /// A new expression binding with the specified expression and variable name ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbExpressionBinding CreateExpressionBinding(DbExpression input, string varName) { return new DbExpressionBinding(this, input, varName); } ///is associated with a different command tree /// or does not have a collection result type /// /// Creates a new /// The expression to bind ///that uses a generated variable name to bind the given expression /// A new expression binding with the specified expression and a generated variable name ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbExpressionBinding CreateExpressionBinding(DbExpression input) { return new DbExpressionBinding(this, input, this.BindingAliases.Next()); } ///is associated with a different command tree /// or does not have a collection result type /// /// Creates a new /// The expression to bind /// The variable name that should be used for the binding /// The variable name that should be used to refer to the group when the new group expression binding is used in a group-by expression ///that uses the specified variable name and group variable names to bind the given expression /// A new group expression binding with the specified expression, variable name and group variable name ////// , or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbGroupExpressionBinding CreateGroupExpressionBinding(DbExpression input, string varName, string groupVarName) { return new DbGroupExpressionBinding(this, input, varName, groupVarName); } ///is associated with a different command tree /// or does not have a collection result type /// /// Creates a new group expression binding that uses generated variable and group variable names to bind the given expression /// /// The expression to bind ///A new group expression binding with the specified expression and a generated variable name and group variable name ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbGroupExpressionBinding CreateGroupExpressionBinding(DbExpression input) { string alias = this.BindingAliases.Next(); return new DbGroupExpressionBinding(this, input, alias, string.Format(CultureInfo.InvariantCulture, "Group{0}", alias)); } ///is associated with a different command tree /// or does not have a collection result type /// /// Creates a new /// The expression that defines the sort key /// Specifies whether the sort order is ascending (true) or descending (false) ////// A new sort clause with the given sort key and sort order ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbSortClause CreateSortClause(DbExpression key, bool ascending) { return new DbSortClause(key, ascending, String.Empty); } ///is associated with a different command tree /// or does not have an order-comparable result type /// /// Creates a new /// The expression that defines the sort key /// Specifies whether the sort order is ascending (true) or descending (false) /// The collation to sort under ////// A new sort clause with the given sort key, sort order and collation ////// or is null /// is empty or contains only space characters /// /*CQT_PUBLIC_API(*/internal/*)*/ DbSortClause CreateSortClause(DbExpression key, bool ascending, string collation) { EntityUtil.CheckArgumentNull(collation, "collation"); if (StringUtil.IsNullOrEmptyOrWhiteSpace(collation)) { throw EntityUtil.ArgumentOutOfRange(System.Data.Entity.Strings.Cqt_Sort_EmptyCollationInvalid, "collation"); } return new DbSortClause(key, ascending, collation); } ///is associated with a different command tree or does not have a string result type /// /// Creates a new /// The function that defines the aggregate operation. /// The argument over which the aggregate function should be calculated. ///. /// A new function aggregate with a reference to the given function and argument. The function aggregate's Distinct property will have the value false ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbFunctionAggregate CreateFunctionAggregate(EdmFunction function, DbExpression argument) { return new DbFunctionAggregate(this, function, argument, false); } ///is not associated with this command tree's metadata workspace /// or has more than one argument, /// is associated with a different command tree, or /// the result type of is not equal or promotable to /// the parameter type of /// /// Creates a new /// The function that defines the aggregate operation. /// The argument over which the aggregate function should be calculated. ///that is applied in a distinct fashion. /// A new function aggregate with a reference to the given function and argument. The function aggregate's Distinct property will have the value true ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbFunctionAggregate CreateDistinctFunctionAggregate(EdmFunction function, DbExpression argument) { return new DbFunctionAggregate(this, function, argument, true); } #if ENABLE_NESTAGGREGATE ///is not associated with this command tree's metadata workspace /// or has more than one argument, /// is associated with a different command tree, or /// the result type of is not equal or promotable to /// the parameter type of /// /// Creates a new /// The argument over which to perform the nest operation ///over the specified argument /// A new nest aggregate with a reference to the given argument. ////// is null /*CQT_PUBLIC_API(*/internal/*)*/ NestAggregate CreateNestAggregate(Expression argument) { return new NestAggregate(this, argument); } #endif /// is associated with a different command tree /// Creates a new /// The relationship end from which navigation takes place ///The relationship end to which navigation may be satisifed using the target entity ref ///An expression that produces a reference to the target entity (and must therefore have a Ref result type) internal DbRelatedEntityRef CreateRelatedEntityRef(RelationshipEndMember sourceEnd, RelationshipEndMember targetEnd, DbExpression targetEntity) { return new DbRelatedEntityRef(this, sourceEnd, targetEnd, targetEntity); } #endregion #region DbExpression Factory Methods #region Constants ///that describes how to satisfy the relationship /// navigation operation from to , which /// must be declared by the same relationship type. /// DbRelatedEntityRefs are used in conjuction with /// to construct Entity instances that are capable of resolving relationship navigation operations based on /// the provided DbRelatedEntityRefs without the need for additional navigation operations. /// Note also that this factory method is not intended to be part of the public Command Tree API /// since its intent is to support Entity constructors in view definitions that express information about /// related Entities using the 'WITH RELATIONSHIPS' clause in eSQL. /// /// Creates a new /// The constant value to represent. ///with the given constant value. /// A new DbConstantExpression with the given value. ////// is null /*CQT_PUBLIC_API(*/internal/*)*/ DbConstantExpression CreateConstantExpression(object value) { return new DbConstantExpression(this, value); } /// is not an instance of a valid constant type /// Creates a new /// The constant value to represent. /// The type of the constant value. ///of the specified primitive type with the given constant value. /// A new DbConstantExpression with the given value and a result type of ///. /// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbConstantExpression CreateConstantExpression(object value, TypeUsage constantType) { return new DbConstantExpression(this, value, constantType); } ///is not an instance of a valid constant type, /// does not represent a primitive type, or /// is of a different primitive type than that represented by /// /// Creates a ///with the Boolean value false
. ///A DbConstantExpression with the Boolean value false. /*CQT_PUBLIC_API(*/internal/*)*/ DbConstantExpression CreateFalseExpression() { if (null == _falseExpr) { _falseExpr = this.CreateConstantExpression(false, this.TypeHelper.CreateBooleanResultType()); } return _falseExpr; } ////// Creates a new /// The type of the null value. ///, which represents a typed null value. /// An instance of DbNullExpression ////// is null /*CQT_PUBLIC_API(*/internal/*)*/ DbNullExpression CreateNullExpression(TypeUsage type) { return new DbNullExpression(this, type); } /// is not associated with this command tree's metadata workspace /// Creates a ///with the Boolean value true. /// A DbConstantExpression with the Boolean value /*CQT_PUBLIC_API(*/internal/*)*/ DbConstantExpression CreateTrueExpression() { if (null == _trueExpr) { _trueExpr = this.CreateConstantExpression(true, this.TypeHelper.CreateBooleanResultType()); } return _trueExpr; } #endregion #region Variables ///true
./// Creates a /// The name of the parameter to reference. ///that references the parameter with the given name. /// The parameter must already exist on the command tree. /// A new DbParameterReferenceExpression that references the given parameter. ////// is null If no parameter with the given name exists on the command tree. Parameters can be added to the command tree using the AddParameter method /*CQT_PUBLIC_API(*/internal/*)*/ DbParameterReferenceExpression CreateParameterReferenceExpression(string name) { if (null == name) { throw EntityUtil.ArgumentNull("name"); } // // Attempt to find a parameter with the specified name in the parameters dictionary. // If the name is not valid the dictionary is not consulted since no parameter with // an invalid name should ever be added to the dictionary. // Note that when the ParameterRefExpression is created the name that is used is the // exact name that the parameter was originally created with (from the dictionary) // since this is the name the Validator will use (in an exact match) to determine // whether a given ParameterRefExpression is valid or not. // KeyValuePairfoundParam; if (DbCommandTree.IsValidParameterName(name) && _paramMappings.TryGetValue(name, out foundParam)) { return new DbParameterReferenceExpression(this, foundParam.Value, foundParam.Key); } throw EntityUtil.ArgumentOutOfRange(System.Data.Entity.Strings.Cqt_CommandTree_NoParameterExists, "name"); } /// /// Creates a /// The name of the variable to reference. /// The type of the variable to reference. ///that references a variable with the given name and type. /// A new DbVariableReferenceExpression that references the specified variable. ////// or is null /*CQT_PUBLIC_API(*/internal/*)*/ DbVariableReferenceExpression CreateVariableReferenceExpression(string varName, TypeUsage varType) { return new DbVariableReferenceExpression(this, varType, varName); } #endregion #region Member Access #if METHOD_EXPRESSION /// is not associated with this command tree's metadata workspace /// Creates a new /// The metadata for the method to invoke. /// The invocation target. /// The arguments to the method. ///representing the invocation of the specified method on the given instance with the given arguments. /// A new MethodExpression that represents the method invocation. ////// ///or is null, /// or is null or contains null /// /// /*CQT_PUBLIC_API(*/internal/*)*/ MethodExpression CreateInstanceMethodExpression(MethodMetadata methodInfo, Expression instance, IListis not associated with this command tree's metadata workspace, /// is associated with a different command tree /// or has a result type that is not equal or promotable to the declaring type of the method, /// or contains an incorrect number of expressions, /// an expression with a result type that is not equal or promotable to the type of the corresponding /// method parameter, or an expression that is associated with a different command tree. /// args) { if (methodInfo != null && methodInfo.IsStatic) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Factory_InstanceMethodRequired, "methodInfo"); } return new MethodExpression(this, methodInfo, args, instance); } /// /// Creates a new /// The metadata for the method to invoke. /// The arguments to the method. ///representing the invocation of the specified method with the given arguments. /// A new MethodExpression that represents the method invocation. ////// is null, or is null or contains null /// /*CQT_PUBLIC_API(*/internal/*)*/ MethodExpression CreateStaticMethodExpression(MethodMetadata methodInfo, IListis not associated with this command tree's metadata workspace, /// or contains an incorrect number of expressions, /// an expression with a result type that is not equal or promotable to the type of the corresponding /// method parameter, or an expression that is associated with a different command tree. /// args) { if (methodInfo != null && !methodInfo.IsStatic) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Factory_StaticMethodRequired, "methodInfo"); } return new MethodExpression(this, methodInfo, args, null); } #endif /// /// Creates a new /// The name of the property to retrieve. /// The instance from which to retrieve the property. ///representing the retrival of the instance property with the specified name from the given instance. /// A new DbPropertyExpression that represents the property retrieval ////// is null or is null and the property is not static. No property with the specified name is declared by the type of ///. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbPropertyExpression CreatePropertyExpression(string propertyName, DbExpression instance) { return CreatePropertyExpression(propertyName, false, instance); } ///is associated with a different command tree, or is non-null and the property is static. /// /// Creates a new /// The name of the property to retrieve. /// Ifrepresenting the retrival of the instance property with the specified name from the given instance. /// true , a case-insensitive member lookup ofis performed; otherwise a member with a name that is an exact match must be declared by the type of . /// The instance from which to retrieve the property. /// A new DbPropertyExpression that represents the property retrieval ////// is null or is null and the property is not static. No property with the specified name (determined with respect to ///) is declared by the type of . /// /*CQT_PUBLIC_API(*/internal/*)*/ DbPropertyExpression CreatePropertyExpression(string propertyName, bool ignoreCase, DbExpression instance) { EntityUtil.CheckArgumentNull(propertyName, "propertyName"); EntityUtil.CheckArgumentNull(instance, "instance"); // // EdmProperty, NavigationProperty and RelationshipEndMember are the only valid members for DbPropertyExpression. // Since these all derive from EdmMember they are declared by subtypes of StructuralType, // so a non-StructuralType instance is invalid. // StructuralType structType; if(TypeHelpers.TryGetEdmTypeis associated with a different command tree, or is non-null and the property is static. /// (instance.ResultType, out structType)) { // // Does the type declare a member with the given name? // EdmMember foundMember; if(structType.Members.TryGetValue(propertyName, ignoreCase, out foundMember)) { // // If the member is a RelationshipEndMember, call the corresponding overload. // if (Helper.IsRelationshipEndMember(foundMember)) { return this.CreatePropertyExpression((RelationshipEndMember)foundMember, instance); } // // Otherwise if the member is an EdmProperty, call that overload instead. // if (Helper.IsEdmProperty(foundMember)) { return this.CreatePropertyExpression((EdmProperty)foundMember, instance); } // // The only remaining valid option is NavigationProperty. // if (Helper.IsNavigationProperty(foundMember)) { return this.CreatePropertyExpression((NavigationProperty)foundMember, instance); } } } throw EntityUtil.ArgumentOutOfRange(System.Data.Entity.Strings.Cqt_Factory_NoSuchProperty, "propertyName"); } /// /// Creates a new /// Metadata for the property to retrieve. /// The instance from which to retrieve the property. May be null if the property is static. ///representing the retrival of the specified property. /// A new DbPropertyExpression representing the property retrieval. ////// is null or is null and the property is not static. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbPropertyExpression CreatePropertyExpression(EdmProperty propertyInfo, DbExpression instance) { return new DbPropertyExpression(this, propertyInfo, instance); } ///is not associated with this command tree's metadata workspace, /// or is associated with a different command tree, or is non-null and the property is static. /// /// Creates a new /// Metadata for the relationship end member to retrieve. /// The instance from which to retrieve the relationship end member. ///representing the retrival of the specified relationship end member. /// A new DbPropertyExpression representing the relationship end member retrieval. ////// is null or is null and the property is not static. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbPropertyExpression CreatePropertyExpression(RelationshipEndMember memberInfo, DbExpression instance) { return new DbPropertyExpression(this, memberInfo, instance); } ///is not associated with this command tree's metadata workspace, /// or is associated with a different command tree. /// /// Creates a new /// Metadata for the navigation property to retrieve. /// The instance from which to retrieve the navigation property. ///representing the retrival of the specified navigation property. /// A new DbPropertyExpression representing the navigation property retrieval. ////// is null or is null. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbPropertyExpression CreatePropertyExpression(NavigationProperty propertyInfo, DbExpression instance) { return new DbPropertyExpression(this, propertyInfo, instance); } ///is not associated with this command tree's metadata workspace, /// or is associated with a different command tree. /// /// Creates a new /// Metadata for the property that represents the end of the relationship from which navigation should occur /// Metadata for the property that represents the end of the relationship to which navigation should occur /// An expression the specifies the instance from which naviagtion should occur ///representing the navigation of a composition or association relationship. /// A new DbRelationshipNavigationExpression representing the navigation of the specified from and to relation ends of the specified relation type from the specified navigation source instance ////// , or is null /// ///and are not declared by the same relationship type /// or are not associated with this command tree's metadata workspace, or is associated /// with a different command tree or has a result type that is not compatible with the property type of . /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbRelationshipNavigationExpression CreateRelationshipNavigationExpression(RelationshipEndMember fromEnd, RelationshipEndMember toEnd, DbExpression from) { return new DbRelationshipNavigationExpression(this, fromEnd, toEnd, from); } ///requires that navigation always occur from a reference, and so must always have a reference result type. /// /// Creates a new /// Metadata for the relation type that represents the relationship /// The name of the property of the relation type that represents the end of the relationship from which navigation should occur /// The name of the property of the relation type that represents the end of the relationship to which navigation should occur /// An expression the specifies the instance from which naviagtion should occur ///representing the navigation of a composition or association relationship. /// A new DbRelationshipNavigationExpression representing the navigation of the specified from and to relation ends of the specified relation type from the specified navigation source instance ////// ///, , or is null. /// /// ///is not associated with this command tree's metadata workspace or is associated with a different command tree, /// or does not declare a relation end property with name or , /// or has a result type that is not compatible with the property type of the relation end property with name . /// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] /*CQT_PUBLIC_API(*/internal/*)*/ DbRelationshipNavigationExpression CreateRelationshipNavigationExpression(RelationshipType type, string fromEndName, string toEndName, DbExpression from) { return new DbRelationshipNavigationExpression(this, type, fromEndName, toEndName, from); } #endregion #region EdmFunction Invocation (including Lambda) ///requires that navigation always occur from a reference, and so must always have a reference result type. /// /// Creates a new /// Metadata for the function to invoke. /// A list of expressions that provide the arguments to the function. ///representing the invocation of the specified function with the given arguments. /// A new DbFunctionExpression representing the function invocation. ////// ///is null, or is null or contains null. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbFunctionExpression CreateFunctionExpression(EdmFunction function, IListis not associated with this command tree's metadata workspace, /// the count of does not equal the number of parameters declared by , /// or contains an expression that is associated with a different command tree or has /// a result type that is not equal or promotable to the corresponding function parameter type. /// args) { return new DbFunctionExpression(this, function, null, args); } /// /// Creates a /// The names and types of the formal arguments to the Lambda function /// An expression that defines the logic of the Lambda function /// A list of expressions that provides the values on which the function is invoked. ///that represents the invocation of the inline (Lambda) function described by the given formal parameters and body expression. /// A new DbFunctionExpression that invokes the specified Lambda function with the given arguments ////// , oris null or contains an element with a null name or type, /// is null is null or contains null. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbFunctionExpression CreateLambdaFunctionExpression(IEnumerablecontains an element with a type associated with a different metadata workspace, /// is associated with a different command tree, or constains /// an expression that is associated with a different command tree. /// > formals, DbExpression body, IList args) { EntityUtil.CheckArgumentNull(formals, "formals"); EntityUtil.CheckArgumentNull(body, "body"); List funcParams = new List (); List > formalArgs = new List >(formals); int idx = 0; CommandTreeUtils.CheckNamedList ( "formals", formalArgs, true, delegate(KeyValuePair formal, int index) { this.TypeHelper.CheckType(formal.Value, CommandTreeUtils.FormatIndex("formals", idx)); idx++; funcParams.Add(new FunctionParameter(formal.Key, formal.Value, ParameterMode.In)); } ); FunctionParameter retParam = new FunctionParameter("Return", body.ResultType, ParameterMode.ReturnValue); EdmFunction lambdaFunction = new EdmFunction(DbFunctionExpression.LambdaFunctionName, DbFunctionExpression.LambdaFunctionNameSpace, retParam.TypeUsage.EdmType.DataSpace, new EdmFunctionPayload { ReturnParameter = retParam, Parameters = funcParams.ToArray(), }); lambdaFunction.SetReadOnly(); return new DbFunctionExpression(this, lambdaFunction, body, args); } #endregion #region Arithmetic Operators /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that divides the left argument by the right argument. /// A new DbArithmeticExpression representing the division operation. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbArithmeticExpression CreateDivideExpression(DbExpression left, DbExpression right) { return new DbArithmeticExpression(this, DbExpressionKind.Divide, CommandTreeUtils.CreateListor is associated with a different command tree, /// or no common numeric result type exists between them. /// (left, right)); } /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that subtracts the right argument from the left argument. /// A new DbArithmeticExpression representing the subtraction operation. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbArithmeticExpression CreateMinusExpression(DbExpression left, DbExpression right) { return new DbArithmeticExpression(this, DbExpressionKind.Minus, CommandTreeUtils.CreateListor is associated with a different command tree, /// or no common numeric result type exists between them. /// (left, right)); } /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that computes the remainder of the left argument divided by the right argument. /// A new DbArithmeticExpression representing the modulo operation. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbArithmeticExpression CreateModuloExpression(DbExpression left, DbExpression right) { return new DbArithmeticExpression(this, DbExpressionKind.Modulo, CommandTreeUtils.CreateListor is associated with a different command tree, /// or no common numeric result type exists between them. /// (left, right)); } /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that multiplies the left argument by the right argument. /// A new DbArithmeticExpression representing the multiplication operation. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbArithmeticExpression CreateMultiplyExpression(DbExpression left, DbExpression right) { return new DbArithmeticExpression(this, DbExpressionKind.Multiply, CommandTreeUtils.CreateListor is associated with a different command tree, /// or no common numeric result type exists between them. /// (left, right)); } /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that adds the left argument to the right argument. /// A new DbArithmeticExpression representing the addition operation. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbArithmeticExpression CreatePlusExpression(DbExpression left, DbExpression right) { return new DbArithmeticExpression(this, DbExpressionKind.Plus, CommandTreeUtils.CreateListor is associated with a different command tree, /// or no common numeric result type exists between them. /// (left, right)); } /// /// Creates a new /// An expression that specifies the argument. ///that negates the value of the argument. /// A new DbArithmeticExpression representing the negation operation. ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbArithmeticExpression CreateUnaryMinusExpression(DbExpression argument) { return new DbArithmeticExpression(this, DbExpressionKind.UnaryMinus, CommandTreeUtils.CreateListis associated with a different command tree, /// or has a non-numeric result type. /// (argument)); } #endregion #region Comparison Operators /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that compares the left and right arguments for equality. /// A new DbComparisonExpression representing the equality comparison. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbComparisonExpression CreateEqualsExpression(DbExpression left, DbExpression right) { return new DbComparisonExpression(this, DbExpressionKind.Equals, left, right); } ///or is associated with a different command tree, /// or no common equality-comparable result type exists between them. /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that determines whether the left argument is greater than the right argument. /// A new DbComparisonExpression representing the greater-than comparison. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbComparisonExpression CreateGreaterThanExpression(DbExpression left, DbExpression right) { return new DbComparisonExpression(this, DbExpressionKind.GreaterThan, left, right); } ///or is associated with a different command tree, /// or no common order-comparable result type exists between them. /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that determines whether the left argument is greater than or equal to the right argument. /// A new DbComparisonExpression representing the greater-than-or-equal-to comparison. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbComparisonExpression CreateGreaterThanOrEqualsExpression(DbExpression left, DbExpression right) { return new DbComparisonExpression(this, DbExpressionKind.GreaterThanOrEquals, left, right); } ///or is associated with a different command tree, /// or no common result type that is both equality- and order-comparable exists between them. /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that determines whether the left argument is less than the right argument. /// A new DbComparisonExpression representing the less-than comparison. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbComparisonExpression CreateLessThanExpression(DbExpression left, DbExpression right) { return new DbComparisonExpression(this, DbExpressionKind.LessThan, left, right); } ///or is associated with a different command tree, /// or no common order-comparable result type exists between them. /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that determines whether the left argument is less than or equal to the right argument. /// A new DbComparisonExpression representing the less-than-or-equal-to comparison. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbComparisonExpression CreateLessThanOrEqualsExpression(DbExpression left, DbExpression right) { return new DbComparisonExpression(this, DbExpressionKind.LessThanOrEquals, left, right); } ///or is associated with a different command tree, /// or no common result type that is both equality- and order-comparable exists between them. /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that compares the left and right arguments for inequality. /// A new DbComparisonExpression representing the inequality comparison. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbComparisonExpression CreateNotEqualsExpression(DbExpression left, DbExpression right) { return new DbComparisonExpression(this, DbExpressionKind.NotEquals, left, right); } #endregion #region Boolean Connectors ///or is associated with a different command tree, /// or no common equality-comparable result type exists between them. /// /// Creates an /// A Boolean expression that specifies the left argument. /// A Boolean expression that specifies the right argument. ///that performs the logical And of the left and right arguments. /// A new DbAndExpression with the specified arguments. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbAndExpression CreateAndExpression(DbExpression left, DbExpression right) { return new DbAndExpression(this, left, right); } ///or is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates an /// A Boolean expression that specifies the left argument. /// A Boolean expression that specifies the right argument. ///that performs the logical Or of the left and right arguments. /// A new DbOrExpression with the specified arguments. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbOrExpression CreateOrExpression(DbExpression left, DbExpression right) { return new DbOrExpression(this, left, right); } ///or is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates a /// A Boolean expression that specifies the argument. ///that performs the logical negation of the given argument. /// A new DbNotExpression with the specified argument. ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbNotExpression CreateNotExpression(DbExpression argument) { return new DbNotExpression(this, argument); } #endregion #region Relational Operators ///is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates a new /// An expression binding that specifies the input set. /// An expression representing a predicate to evaluate for each member of the input set. ///that determines whether the given predicate holds for any element of the input set. /// A new DbQuantifierExpression that represents the Any operation. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbQuantifierExpression CreateAnyExpression(DbExpressionBinding input, DbExpression predicate) { return new DbQuantifierExpression(this, DbExpressionKind.Any, input, predicate); } ///or is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates a new /// An expression binding that specifies the input set. /// An expression representing a predicate to evaluate for each member of the input set. ///that determines whether the given predicate holds for all elements of the input set. /// A new DbQuantifierExpression that represents the All operation. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbQuantifierExpression CreateAllExpression(DbExpressionBinding input, DbExpression predicate) { return new DbQuantifierExpression(this, DbExpressionKind.All, input, predicate); } ///or is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates a new /// Anthat evaluates the given expression once for each element of a given input set, /// producing a collection of rows with corresponding input and apply columns. Rows for which evaluates to an empty set are not included. /// that specifies the input set. /// An that specifies logic to evaluate once for each member of the input set. /// An new DbApplyExpression with the specified input and apply bindings and an ///of CrossApply. /// or is null /*CQT_PUBLIC_API(*/internal/*)*/ DbApplyExpression CreateCrossApplyExpression(DbExpressionBinding input, DbExpressionBinding apply) { return new DbApplyExpression(this, input, apply, DbExpressionKind.CrossApply); } /// or is associated with a different command tree /// Creates a new /// Anthat evaluates the given expression once for each element of a given input set, /// producing a collection of rows with corresponding input and apply columns. Rows for which evaluates to an empty set have an apply column value of null
. ///that specifies the input set. /// An that specifies logic to evaluate once for each member of the input set. /// An new DbApplyExpression with the specified input and apply bindings and an ///of OuterApply. /// or is null /*CQT_PUBLIC_API(*/internal/*)*/ DbApplyExpression CreateOuterApplyExpression(DbExpressionBinding input, DbExpressionBinding apply) { return new DbApplyExpression(this, input, apply, DbExpressionKind.OuterApply); } /// or is associated with a different command tree /// Creates a new /// An expression that defines the set over which to perfom the distinct operation. ///that removes duplicates from the given set argument. /// A new DbDistinctExpression that represents the distinct operation applied to the specified set argument. ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbDistinctExpression CreateDistinctExpression(DbExpression argument) { return new DbDistinctExpression(this, argument); } ///is associated with a different command tree, /// or does not have a collection result type. /// /// Creates a new /// An expression that specifies the input set. ///that converts a single-member set into a singleton. /// A DbElementExpression that represents the conversion of the single-member set argument to a singleton. ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbElementExpression CreateElementExpression(DbExpression argument) { return new DbElementExpression(this, argument, false); } ///is associated with a different command tree, /// or does not have a collection result type. /// /// Creates a new /// An expression that specifies the input set. ///that converts a single-member set with a single property /// into a singleton. The result type of the created equals the result type /// of the single property of the element of the argument. /// /// This method should only be used when the argument is of a collection type with /// element of structured type with only one property. /// A DbElementExpression that represents the conversion of the single-member set argument to a singleton. ////// is null /// internal DbElementExpression CreateElementExpressionUnwrapSingleProperty(DbExpression argument) { return new DbElementExpression(this, argument, true); } ///is associated with a different command tree, /// or does not have a collection result type, or its element type is not a structured type /// with only one property /// /// Creates a new /// An expression that defines the left set argument. /// An expression that defines the right set argument. ///that computes the subtraction of the right set argument from the left set argument. /// A new DbExceptExpression that represents the difference of the left argument from the right argument. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbExceptExpression CreateExceptExpression(DbExpression left, DbExpression right) { return new DbExceptExpression(this, left, right); } ///or is associated with a different command tree, /// or no common collection result type exists between them. /// /// Creates a new /// An expression binding that specifies the input set. /// An expression representing a predicate to evaluate for each member of the input set. ///that filters the elements in the given input set using the specified predicate. /// A new DbFilterExpression that produces the filtered set. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbFilterExpression CreateFilterExpression(DbExpressionBinding input, DbExpression predicate) { return new DbFilterExpression(this, input, predicate); } ///or is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates a new /// Athat groups the elements of the input set according to the specified group keys and applies the given aggregates. /// that specifies the input set. /// A list of string-expression pairs that define the grouping columns. /// A list of expressions that specify aggregates to apply. /// A new DbGroupByExpression with the specified input set, grouping keys and aggregates. ////// ///, or is null, /// contains a null key column name or expression, or /// contains a null aggregate column name or aggregate. /// /// ///is associated with a different command tree, /// both and are empty, /// contains an expression that is associated with a different command tree, /// or contains an aggregate that is not associated with the command tree's metadata workspace, /// or an invalid or duplicate column name was specified. /// /// DbGroupByExpression allows either the list of keys or the list of aggregates to be empty, but not both. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbGroupByExpression CreateGroupByExpression(DbGroupExpressionBinding input, IList> keys, IList > aggregates) { return new DbGroupByExpression(this, input, keys, aggregates); } /// /// Creates a new /// An expression that defines the left set argument. /// An expression that defines the right set argument. ///that computes the intersection of the left and right set arguments. /// A new DbIntersectExpression that represents the intersection of the left and right arguments. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbIntersectExpression CreateIntersectExpression(DbExpression left, DbExpression right) { return new DbIntersectExpression(this, left, right); } ///or is associated with a different command tree, /// or no common collection result type exists between them. /// /// Creates a new /// A list of expression bindings that specifies the input sets. ///that unconditionally joins the sets specified by the list of input expression bindings. /// A new DbCrossJoinExpression, with an ///of CrossJoin, that represents the unconditional join of the input sets. /// is null or contains null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbCrossJoinExpression CreateCrossJoinExpression(IListcontains fewer than 2 expression bindings, /// or contains an expression binding that is associated with a different command tree. /// inputs) { return new DbCrossJoinExpression(this, inputs); } /// /// Creates a new /// Anthat joins the sets specified by the left and right /// expression bindings, on the specified join condition, using InnerJoin as the . /// that specifies the left set argument. /// An that specifies the right set argument. /// An expression that specifies the condition on which to join. /// /// A new DbJoinExpression, with an ///of InnerJoin, that represents the inner join operation applied to the left and right /// input sets under the given join condition. /// /// ///, or is null. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbJoinExpression CreateInnerJoinExpression(DbExpressionBinding left, DbExpressionBinding right, DbExpression joinCondition) { return new DbJoinExpression(this, DbExpressionKind.InnerJoin, left, right, joinCondition); } ///, or is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates a new /// Anthat joins the sets specified by the left and right /// expression bindings, on the specified join condition, using LeftOuterJoin as the . /// that specifies the left set argument. /// An that specifies the right set argument. /// An expression that specifies the condition on which to join. /// /// A new DbJoinExpression, with an ///of LeftOuterJoin, that represents the left outer join operation applied to the left and right /// input sets under the given join condition. /// /// ///, or is null. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbJoinExpression CreateLeftOuterJoinExpression(DbExpressionBinding left, DbExpressionBinding right, DbExpression joinCondition) { return new DbJoinExpression(this, DbExpressionKind.LeftOuterJoin, left, right, joinCondition); } ///, or is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates a new /// Anthat joins the sets specified by the left and right /// expression bindings, on the specified join condition, using FullOuterJoin as the . /// that specifies the left set argument. /// An that specifies the right set argument. /// An expression that specifies the condition on which to join. /// /// A new DbJoinExpression, with an ///of FullOuterJoin, that represents the full outer join operation applied to the left and right /// input sets under the given join condition. /// /// ///, or is null. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbJoinExpression CreateFullOuterJoinExpression(DbExpressionBinding left, DbExpressionBinding right, DbExpression joinCondition) { return new DbJoinExpression(this, DbExpressionKind.FullOuterJoin, left, right, joinCondition); } ///, or is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates a new /// An expression binding that specifies the input set. /// An expression to project over the set. ///that projects the specified expression over the given input set. /// A new DbProjectExpression that represents the projection operation. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbProjectExpression CreateProjectExpression(DbExpressionBinding input, DbExpression projection) { return new DbProjectExpression(this, input, projection); } ///or is associated with a different command tree. /// /// Creates a new /// An expression binding that specifies the input set. /// A list of sort specifications that determine how the elements of the input set should be sorted. /// An expression the specifies how many elements of the ordered set to skip. ///that sorts the given input set by the given sort specifications before skipping the specified number of elements. /// A new DbSkipExpression that represents the skip operation. ////// ///, or is null, /// or contains null. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbSkipExpression CreateSkipExpression(DbExpressionBinding input, IListor is associated with a different command tree, /// is empty or contains a that is associated with a different command tree, /// or is not or or has a /// result type that is not equal or promotable to a 64-bit integer type. /// sortOrder, DbExpression count) { return new DbSkipExpression(this, input, sortOrder, count); } /// /// Creates a new /// An expression binding that specifies the input set. /// A list of sort specifications that determine how the elements of the input set should be sorted. ///that sorts the given input set by the specified sort specifications. /// A new DbSortExpression that represents the sort operation. ////// ///or is null, /// or contains null. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbSortExpression CreateSortExpression(DbExpressionBinding input, IListis associated with a different command tree, /// or is empty or contains a that is associated with a different command tree. /// sortOrder) { return new DbSortExpression(this, input, sortOrder); } /// /// Creates a new /// An expression that defines the left set argument. /// An expression that defines the right set argument. ///that computes the union of the left and right set arguments and does not remove duplicates. /// A new DbUnionAllExpression that union, including duplicates, of the the left and right arguments. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbUnionAllExpression CreateUnionAllExpression(DbExpression left, DbExpression right) { return new DbUnionAllExpression(this, left, right); } #endregion #region General Operators ///or is associated with a different command tree, /// or no common collection result type exists between them. /// /// Creates a new /// A list of expressions that provide the conditional for of each case. /// A list of expressions that provide the result of each case. /// An expression that defines the result when no case is matched. ///. /// A new DbCaseExpression with the specified cases and default result. ////// ///or is null or contains null, /// or is null. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbCaseExpression CreateCaseExpression(IListor is empty or contains an expression associated with a different command tree, /// is associated with a different command tree, or no common result type exists for all expressions in /// and . /// whenExpressions, IList thenExpressions, DbExpression elseExpression) { return new DbCaseExpression(this, whenExpressions, thenExpressions, elseExpression); } /// /// Creates a new /// The argument to which the cast should be applied. /// Type metadata that specifies the type to cast to. ///that applies a cast operation to a polymorphic argument. /// A new DbCastExpression with the specified argument and target type. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbCastExpression CreateCastExpression(DbExpression argument, TypeUsage toType) { return new DbCastExpression(this, toType, argument); } ///is associated with a different command tree, /// is not associated with this command tree's metadata workspace, /// or the specified cast is not valid. /// /// Creates a new /// Metadata for the entity or relationship set to reference. ///that references the specified entity or relationship set. /// A new DbScanExpression based on the specified entity or relationship set. ////// is null /*CQT_PUBLIC_API(*/internal/*)*/ DbScanExpression CreateScanExpression(EntitySetBase target) { return new DbScanExpression(this, target); } /// is not associated with the metadata workspace of this command tree /// Creates a new /// An expression that specifies the input set ///that determines whether the specified set argument is an empty set. /// A new DbIsEmptyExpression with the specified argument. ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbIsEmptyExpression CreateIsEmptyExpression(DbExpression argument) { return new DbIsEmptyExpression(this, argument); } ///is associated with a different command tree, /// or does not have a collection result type. /// /// Creates a new /// An expression that specifies the argument. ///that determines whether the specified argument is null. /// A new DbIsNullExpression with the specified argument. ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbIsNullExpression CreateIsNullExpression(DbExpression argument) { return new DbIsNullExpression(this, argument, false); } ///is associated with a different command tree, or /// has a collection result type. /// /// Creates a new /// An expression that specifies the argument. ///that determines whether the specified argument is null. /// Used only by span rewriter, when a row could is specified as an argument /// A new DbIsNullExpression with the specified argument. ////// is null /// internal DbIsNullExpression CreateIsNullExpressionAllowingRowTypeArgument(DbExpression argument) { return new DbIsNullExpression(this, argument, true); } ///is associated with a different command tree, or /// has a collection result type. /// /// Creates a new /// An expression that specifies the instance. /// Type metadata that specifies the type that the instance's result type should be compared to. ///that determines whether the given argument is of the specified type or a subtype. /// A new DbIsOfExpression with the specified instance and type and DbExpressionKind IsOf. ////// or is null /// ///is associated with a different command tree, /// is not associated with this command tree's metadata workspace, or /// is not in the same type hierarchy as the result type of . /// /// DbIsOfExpression requires that /*CQT_PUBLIC_API(*/internal/*)*/ DbIsOfExpression CreateIsOfExpression(DbExpression argument, TypeUsage type) { return new DbIsOfExpression(this, DbExpressionKind.IsOf, type, argument); } ///has a polymorphic result type, /// and that is a type from the same type hierarchy as that result type. /// /// Creates a new /// An expression that specifies the instance. /// Type metadata that specifies the type that the instance's result type should be compared to. ///expression that determines whether the given argument is of the specified type, and only that type (not a subtype). /// A new DbIsOfExpression with the specified instance and type and DbExpressionKind IsOfOnly. ////// or is null /// ///is associated with a different command tree, /// is not associated with this command tree's metadata workspace, or /// is not in the same type hierarchy as the result type of . /// /// DbIsOfExpression requires that /*CQT_PUBLIC_API(*/internal/*)*/ DbIsOfExpression CreateIsOfOnlyExpression(DbExpression argument, TypeUsage type) { return new DbIsOfExpression(this, DbExpressionKind.IsOfOnly, type, argument); } ///has a polymorphic result type, /// and that is a type from the same type hierarchy as that result type. /// /// Creates a new /// An expression that specifies the input string. /// An expression that specifies the pattern string. ///that compares the specified input string to the given pattern. /// A new DbLikeExpression with the specified input, pattern and a null escape. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbLikeExpression CreateLikeExpression(DbExpression input, DbExpression pattern) { return new DbLikeExpression(this, input, pattern, null); } ///or is associated with a different command tree, /// or does not have a string result type. /// /// Creates a new /// An expression that specifies the input string. /// An expression that specifies the pattern string. /// An optional expression that specifies the escape string. ///that compares the specified input string to the given pattern using the optional escape. /// A new DbLikeExpression with the specified input, pattern and escape. ////// , or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbLikeExpression CreateLikeExpression(DbExpression input, DbExpression pattern, DbExpression escape) { EntityUtil.CheckArgumentNull(escape, "escape"); return new DbLikeExpression(this, input, pattern, escape); } ///, or is associated with a different command tree, /// or does not have a string result type. /// /// Creates a new /// An expression that specifies the input collection. /// An expression that specifies the limit value. ///that restricts the number of elements in the Argument collection to the specified Limit value. /// Tied results are not included in the output. /// A new DbLimitExpression with the specified argument and limit values that does not include tied results. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbLimitExpression CreateLimitExpression(DbExpression argument, DbExpression limit) { return new DbLimitExpression(this, argument, limit, false); } ///is associated with a different command tree or does not have a collection result type, /// or is associated with a different command tree or does not have a result type that is equal or promotable to a 64-bit integer type. /// /// Creates a new /// An expression that specifies the input collection. /// An expression that specifies the limit value. ///that restricts the number of elements in the Argument collection to the specified Limit value, /// including tied results in the output. /// A new DbLimitExpression with the specified argument and limit values that includes tied results. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbLimitExpression CreateLimitWithTiesExpression(DbExpression argument, DbExpression limit) { return new DbLimitExpression(this, argument, limit, true); } ///is associated with a different command tree or does not have a collection result type, /// or is associated with a different command tree or does not have a result type that is equal or promotable to a 64-bit integer type. /// /// Creates a new /// The type of the new instance. /// Expressions that specify values of the new instances, interpreted according to the instance's type. ///. If the type argument is a collection type, the arguments specify the elements of the collection. Otherwise the arguments are used as property or column values in the new instance. /// A new DbNewInstanceExpression with the specified type and arguments. ////// or is null, or contains null /// ///is not associated with this command tree's metadata workspace, /// is empty or contains an expression associated with a different command tree, /// or the result types of the contained expressions do not match the requirements of (as explained in the remarks section). /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbNewInstanceExpression CreateNewInstanceExpression(TypeUsage type, IList/// if ///is a a collection type then every expression in must have a result type that is promotable to the element type of the . /// /// if ///is a row type, must contain as many expressions as there are columns in the record /// type, and the result type of each expression must be equal or promotable to the type of the corresponding column. A row type that does not declare any columns is invalid. /// /// if ///is an entity type, must contain as many expressions as there are properties defined by the type, /// and the result type of each expression must be equal or promotable to the type of the corresponding property. /// args) { return new DbNewInstanceExpression(this, type, args); } /// /// Creates a new /// A list of string-DbExpression key-value pairs that defines the structure and values of the row. ///that produces a row with the specified named columns and the given values, specified as expressions. /// A new DbNewInstanceExpression that represents the construction of the row. ////// is null or contains an element with a null column name or expression /// /*CQT_PUBLIC_API(*/internal/*)*/ DbNewInstanceExpression CreateNewRowExpression(IListis empty, contains a duplicate or invalid column name, /// or contains an expression that is associated with a different command tree. /// > recordColumns) { List args = new List (); List > colTypes = new List >(); CommandTreeUtils.CheckNamedList ( "recordColumns", recordColumns, false, // Don't allow empty list delegate(KeyValuePair exprInfo, int index) { // Use ExpressionLink for standard expression argument validation ExpressionLink testLink = new ExpressionLink(CommandTreeUtils.FormatIndex("recordColumns", index), this, exprInfo.Value); args.Add(exprInfo.Value); colTypes.Add(new KeyValuePair (exprInfo.Key, exprInfo.Value.ResultType)); } ); TypeUsage resultType = CommandTreeTypeHelper.CreateResultType(TypeHelpers.CreateRowType(colTypes)); return new DbNewInstanceExpression(this, resultType, args); } /// /// Creates a new /// A list of expressions that provide the elements of the collection ///that constructs a collection containing the specified elements. The type of the collection is based on the common type of the elements. If no common element type exists an exception is thrown. /// A new DbNewInstanceExpression with the specified collection type and arguments. ////// is null, or contains null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbNewInstanceExpression CreateNewCollectionExpression(IListis empty, contains an expression that is not associated with this command tree, /// or contains expressions for which no common result type exists. /// collectionElements) { if (null == collectionElements) { throw EntityUtil.ArgumentNull("collectionElements"); } if (collectionElements.Count < 1) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Factory_NewCollectionElementsRequired, "collectionElements"); } // // Use an ExpressionList to determine the common element type of the collection // (Note that this ExpressionList is not used by the DbNewInstanceExpression itself) // ExpressionList elements = new ExpressionList("collectionElements", this, collectionElements.Count); elements.SetElements(collectionElements); TypeUsage commonElementType = elements.GetCommonElementType(); if (TypeSemantics.IsNullOrNullType(commonElementType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Factory_NewCollectionInvalidCommonType, "collectionElements"); } TypeUsage resultType = CommandTreeTypeHelper.CreateCollectionResultType(commonElementType); return new DbNewInstanceExpression(this, resultType, elements); } /// /// Creates a new /// The type metadata for the collection to create ///that constructs an empty collection of the specified collection type. /// A new DbNewInstanceExpression with the specified collection type and an empty ///Arguments
list./// is null /*CQT_PUBLIC_API(*/internal/*)*/ DbNewInstanceExpression CreateNewEmptyCollectionExpression(TypeUsage collectionType) { EntityUtil.CheckArgumentNull(collectionType, "collectionType"); if (!TypeSemantics.IsCollectionType(collectionType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_NewInstance_CollectionTypeRequired, "collectionType"); } return new DbNewInstanceExpression(this, collectionType, null); } /// is not associated with this command tree's metadata workspace /// Creates a new /// The type of the Entity instance that is being constructed /// Values for each (non-relationship) property of the Entity /// A (possibly empty) list ofthat constructs an instance of an Entity type /// together with the specified information about Entities related to the newly constructed Entity by' /// relationship navigations where the target end has multiplicity of at most one. /// Note that this factory method is not intended to be part of the public Command Tree API since it /// its intent is to support Entity constructors in view definitions that express information about /// related Entities using the 'WITH RELATIONSHIPS' clause in eSQL. /// s that describe Entities that are related to the constructed Entity by various relationship types. /// A new DbNewInstanceExpression that represents the construction of the Entity, and includes the specified related Entity information in the see internal DbNewInstanceExpression CreateNewEntityWithRelationshipsExpression(EntityType instanceType, IListcollection. attributeValues, IList relationships) { return new DbNewInstanceExpression(this, instanceType, attributeValues, relationships); } /// /// Creates a new /// Anthat produces a set consisting of the elements of the given input set that are of the specified type. /// that specifies the input set. /// Type metadata for the type that elements of the input set must have to be included in the resulting set. /// A new DbOfTypeExpression with the specified set argument and type, and an ExpressionKind of ///. /// or is null /// ///is associated with a different command tree or does not have a collection result type, /// is not associated with this command tree's metadata workspace, or /// is not a type in the same type hierarchy as the element type of the /// collection result type of . /// /// DbOfTypeExpression requires that /*CQT_PUBLIC_API(*/internal/*)*/ DbOfTypeExpression CreateOfTypeExpression(DbExpression argument, TypeUsage ofType) { return new DbOfTypeExpression(this, DbExpressionKind.OfType, ofType, argument); } ///has a collection result type with /// a polymorphic element type, and that is a type from the same type hierarchy as that element type. /// /// Creates a new /// Anthat produces a set consisting of the elements of the given input set that are of exactly the specified type. /// that specifies the input set. /// Type metadata for the type that elements of the input set must match exactly to be included in the resulting set. /// A new DbOfTypeExpression with the specified set argument and type, and an ExpressionKind of ///. /// or is null /// ///is associated with a different command tree or does not have a collection result type, /// is not associated with this command tree's metadata workspace, or /// is not a type in the same type hierarchy as the element type of the /// collection result type of . /// /// DbOfTypeExpression requires that /*CQT_PUBLIC_API(*/internal/*)*/ DbOfTypeExpression CreateOfTypeOnlyExpression(DbExpression argument, TypeUsage ofType) { return new DbOfTypeExpression(this, DbExpressionKind.OfTypeOnly, ofType, argument); } ///has a collection result type with /// a polymorphic element type, and that is a type from the same type hierarchy as that element type. /// /// Creates a new /// The Entity set in which the referenced element resides. /// Anthat encodes a reference to a specific Entity based on key values. /// that constructs a record type with columns that match (in number and type) the Key properties of the referenced Entity type. /// A new DbRefExpression that references the element with the specified key values in the given Entity set. ////// or is null /// ///is not associated with this command tree's metadata workspace, /// or is associated with a different command tree or does not have a /// record result type that matches the key properties of the referenced entity set's entity type. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbRefExpression CreateRefExpression(EntitySet entitySet, DbExpression refKeys) { EntityUtil.CheckArgumentNull(entitySet, "entitySet"); return new DbRefExpression(this, entitySet, refKeys, entitySet.ElementType); } ///should be an expression that specifies the key values that identify the referenced entity within the given entity set. /// The result type of should contain a corresponding column for each key property defined by 's entity type. /// /// Creates a new /// The Entity set in which the referenced element resides. /// Anthat encodes a reference to a specific Entity based on key values. /// that constructs a record type with columns that match (in number and type) the Key properties of the referenced Entity type. /// The type of the Entity that the reference should refer to. /// A new DbRefExpression that references the element with the specified key values in the given Entity set. ////// , or is null /// ///or is not associated with this command tree's metadata workspace, /// or is associated with a different command tree; /// is not in the same type hierarchy as the entity set's entity type, or does not have a /// record result type that matches the key properties of the referenced entity set's entity type. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbRefExpression CreateRefExpression(EntitySet entitySet, DbExpression refKeys, EntityType entityType) { return new DbRefExpression(this, entitySet, refKeys, entityType); } ///should be an expression that specifies the key values that identify the referenced entity within the given entity set. /// The result type of should contain a corresponding column for each key property defined by 's entity type. /// /// Creates a new /// Anthat retrieves a specific Entity given a reference expression /// that provides the reference. This expression must have a reference Type /// A new DbDerefExpression that retrieves the specified Entity ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbDerefExpression CreateDerefExpression(DbExpression reference) { return new DbDerefExpression(this, reference); } ///is associated with a different command tree, /// or does not have a reference result type. /// /// Creates a new /// The expression that provides the reference. This expression must have a reference Type with an Entity element type. ///that retrieves the key values of the specifed reference in structural form. /// A new DbRefKeyExpression that retrieves the key values of the specified reference. ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbRefKeyExpression CreateRefKeyExpression(DbExpression reference) { return new DbRefKeyExpression(this, reference); } ///is associated with a different command tree, /// or does not have a reference result type. /// /// Creates a new /// The expression that provides the entity. This expression must have an entity result type. ///that retrieves the ref of the specifed entity in structural form. /// A new DbEntityRefExpression that retrieves a reference to the specified entity. ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbEntityRefExpression CreateEntityRefExpression(DbExpression entity) { return new DbEntityRefExpression(this, entity); } ///is associated with a different command tree, /// or does not have an entity result type. /// /// Creates a new /// An expression that specifies the instance. /// Type metadata for the treat-as type. ///. /// A new DbTreatExpression with the specified argument and type. ////// or is null /// ///is associated with a different command tree, /// is not associated with this command tree's metadata workspace, or /// is not in the same type hierarchy as the result type of . /// /// DbTreatExpression requires that /*CQT_PUBLIC_API(*/internal/*)*/ DbTreatExpression CreateTreatExpression(DbExpression argument, TypeUsage treatType) { return new DbTreatExpression(this, treatType, argument); } #endregion // // Additional expression builders // ... #endregion #endregion #region Internal Implementation ///has a polymorphic result type, /// and that is a type from the same type hierarchy as that result type. /// /// Gets the kind of this command tree. /// internal abstract DbCommandTreeKind CommandTreeKind { get; } ////// Gets the metadata workspace used by this command tree. /// internal MetadataWorkspace MetadataWorkspace { get { return _metadata; } } ////// Gets the data space in which metadata used by this command tree must reside. /// internal DataSpace DataSpace { get { return _dataSpace; } } internal CommandTreeTypeHelper TypeHelper { get { return _typeHelp; } } internal virtual void Validate(System.Data.Common.CommandTrees.Internal.Validator v) { } internal bool ValidationRequired { get { bool valReq = _validatedVersion < _currentVersion; EntityBid.Trace("%d#, %d{bool}\n", this.ObjectId, valReq); return valReq; } } internal void SetValid() { EntityBid.Trace(" %d#\n", this.ObjectId); _validatedVersion = _currentVersion; } internal void SetModified() { int prev = _currentVersion; if (int.MaxValue == _currentVersion) { _currentVersion = 1; _validatedVersion = 0; } else { _currentVersion++; } EntityBid.Trace(" %d# previous version=%d, new version=%d\n", this.ObjectId, prev, _currentVersion); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] // we intend for this to be public someday; called from test code for now. internal void ClearParameters() { EntityBid.Trace(" %d#\n", this.ObjectId); _paramMappings.Clear(); } internal void CopyParametersTo(DbCommandTree tree) { foreach (KeyValuePair paramInfo in _paramMappings.Values) { tree.AddParameter(paramInfo.Key, paramInfo.Value); } } internal bool HasParameter(string parameterName, TypeUsage parameterType) { Debug.Assert(!string.IsNullOrEmpty(parameterName), "Parameter name should not be null or empty"); Debug.Assert(parameterType != null, "Parameter type should not be null"); KeyValuePair foundParam; if(this._paramMappings.TryGetValue(parameterName, out foundParam)) { return (foundParam.Key.Equals(parameterName, StringComparison.Ordinal) && // SQLBUDT#545720: Equivalence is not a sufficient check (consider row types for TVPs) - equality is required. TypeSemantics.IsStructurallyEqualTo(foundParam.Value, parameterType)); } return false; } #region Dump/Print Support internal void Dump(ExpressionDumper dumper) { // // Dump information about this command tree to the specified ExpressionDumper // // First dump standard information - the DataSpace of the command tree and its parameters // Dictionary attrs = new Dictionary (); attrs.Add("DataSpace", this.DataSpace); dumper.Begin(this.GetType().Name, attrs); // // The name and type of each Parameter in turn is added to the output // dumper.Begin("Parameters", null); foreach (KeyValuePair param in this.Parameters) { Dictionary paramAttrs = new Dictionary (); paramAttrs.Add("Name", param.Key); dumper.Begin("Parameter", paramAttrs); dumper.Dump(param.Value, "ParameterType"); dumper.End("Parameter"); } dumper.End("Parameters"); // // Delegate to the derived type's implementation that dumps the structure of the command tree // this.DumpStructure(dumper); // // Matching call to End to correspond with the call to Begin above // dumper.End(this.GetType().Name); } internal abstract void DumpStructure(ExpressionDumper dumper); internal string DumpXml() { // // This is a convenience method that dumps the command tree in an XML format. // This is intended primarily as a debugging aid to allow inspection of the tree structure. // // Create a new MemoryStream that the XML dumper should write to. // MemoryStream stream = new MemoryStream(); // // Create the dumper // XmlExpressionDumper dumper = new XmlExpressionDumper(stream); // // Dump this tree and then close the XML dumper so that the end document tag is written // and the output is flushed to the stream. // this.Dump(dumper); dumper.Close(); // // Construct a string from the resulting memory stream and return it to the caller // return XmlExpressionDumper.DefaultEncoding.GetString(stream.ToArray()); } internal string Print() { return this.PrintTree(new ExpressionPrinter()); } internal abstract string PrintTree(ExpressionPrinter printer); #endregion #region DbExpression Replacement internal void Replace(ReplacerCallback callback) { using (new EntityBid.ScopeAuto(" %d#", this.ObjectId)) { ExpressionReplacer replacer = new ExpressionReplacer(callback); this.Replace(replacer); } } internal abstract void Replace(ExpressionReplacer replacer); #endregion #region Tracing internal void Trace() { if (EntityBid.AdvancedOn) { EntityBid.PutStrChunked(this.DumpXml()); EntityBid.Trace("\n"); } else if (EntityBid.TraceOn) { EntityBid.PutStrChunked(this.Print()); EntityBid.Trace("\n"); } } #endregion #region Container Tracking internal void TrackContainer(EntityContainer container) { using (new EntityBid.ScopeAuto(" %d#", this.ObjectId)) { if (!_containers.Contains(container)) { EntityBid.Trace(" %d#, Adding container to tracked containers list: '%ls'\n", this.ObjectId, container.Name); _containers.Add(container); } } } #endregion #region Internal Helper API internal DbApplyExpression CreateApplyExpressionByKind(DbExpressionKind applyKind, DbExpressionBinding input, DbExpressionBinding apply) { Debug.Assert(DbExpressionKind.CrossApply == applyKind || DbExpressionKind.OuterApply == applyKind, "Invalid ApplyType"); switch(applyKind) { case DbExpressionKind.CrossApply: return this.CreateCrossApplyExpression(input, apply); case DbExpressionKind.OuterApply: return this.CreateOuterApplyExpression(input, apply); default: throw EntityUtil.InvalidEnumerationValue(typeof(DbExpressionKind), (int)applyKind); } } internal DbExpression CreateJoinExpressionByKind(DbExpressionKind joinKind, DbExpression joinCondition, DbExpressionBinding input1, DbExpressionBinding input2) { Debug.Assert(DbExpressionKind.CrossJoin == joinKind || DbExpressionKind.FullOuterJoin == joinKind || DbExpressionKind.InnerJoin == joinKind || DbExpressionKind.LeftOuterJoin == joinKind, "Invalid DbExpressionKind for CreateJoinExpressionByKind"); if (DbExpressionKind.CrossJoin == joinKind) { Debug.Assert(null == joinCondition, "Condition should not be specified for CrossJoin"); return this.CreateCrossJoinExpression(new DbExpressionBinding[2] { input1, input2 }); } else { Debug.Assert(joinCondition != null, "Condition must be specified for non-CrossJoin"); switch (joinKind) { case DbExpressionKind.InnerJoin: return this.CreateInnerJoinExpression(input1, input2, joinCondition); case DbExpressionKind.LeftOuterJoin: return this.CreateLeftOuterJoinExpression(input1, input2, joinCondition); case DbExpressionKind.FullOuterJoin: return this.CreateFullOuterJoinExpression(input1, input2, joinCondition); default: throw EntityUtil.InvalidEnumerationValue(typeof(DbExpressionKind), (int)joinKind); } } } internal static bool IsValidParameterName(string name) { return (!StringUtil.IsNullOrEmptyOrWhiteSpace(name) && _paramNameRegex.IsMatch(name)); } private static readonly Regex _paramNameRegex = new Regex("^([A-Za-z])([A-Za-z0-9_])*$"); #endregion #endregion } /// /// Describes the different "kinds" (classes) of command trees. /// internal enum DbCommandTreeKind { Query, Update, Insert, Delete, Function, } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Common; using System.Globalization; using System.IO; using System.Diagnostics; using System.Text; using System.Text.RegularExpressions; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; namespace System.Data.Common.CommandTrees { ////// DbCommandTree is the abstract base type for the Delete, Query, Insert and Update DbCommandTree types. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbCommandTree { #region Private Instance Fields // Metadata collection private MetadataWorkspace _metadata; private DataSpace _dataSpace; // Commonly used types private CommandTreeTypeHelper _typeHelp; // CreateParameter Support private Dictionary> _paramMappings = new Dictionary >(StringComparer.OrdinalIgnoreCase); // Pre-built Expressions DbConstantExpression _trueExpr; DbConstantExpression _falseExpr; // Validation support private int _currentVersion = 1; private int _validatedVersion; // Referenced Container Tracking private List _containers = new List (); // Binding alias generation private AliasGenerator _bindingAliases; internal AliasGenerator BindingAliases { get { if (null == _bindingAliases) { _bindingAliases = new AliasGenerator(string.Format(CultureInfo.InvariantCulture, "Var_{0}_", this.ObjectId), 0); } return _bindingAliases; } } private static int s_instanceCount; // Bid counter internal readonly int ObjectId = System.Threading.Interlocked.Increment(ref s_instanceCount); #endregion #region Constructors /// /// Initializes a new command tree with a given metadata workspace. /// /// The metadata workspace against which the command tree should operate. /// The logical 'space' that metadata in the expressions used in this command tree must belong to. ////// or is null internal DbCommandTree(MetadataWorkspace metadata, DataSpace dataSpace) { using (new EntityBid.ScopeAuto(" does not represent a valid data space %d#", this.ObjectId)) { if (null == metadata) { throw EntityUtil.ArgumentNull("metadata"); } // // Ensure that this is a valid value, in addition to non-null // if (!CommandTreeUtils.IsValidDataSpace(dataSpace)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_CommandTree_InvalidDataSpace, "dataSpace"); } // // Assign the metadata workspace and initalize commonly used types. // _metadata = new MetadataWorkspace(); ItemCollection objectItemCollection; //While EdmItemCollection and StorageitemCollections are required //ObjectItemCollection may or may not be registered on the workspace yet. //So register the ObjectItemCollection if it exists. if (metadata.TryGetItemCollection(DataSpace.OSpace, out objectItemCollection)) { _metadata.RegisterItemCollection(objectItemCollection); } _metadata.RegisterItemCollection(metadata.GetItemCollection(DataSpace.CSpace)); _metadata.RegisterItemCollection(metadata.GetItemCollection(DataSpace.CSSpace)); _metadata.RegisterItemCollection(metadata.GetItemCollection(DataSpace.SSpace)); _dataSpace = dataSpace; _typeHelp = new CommandTreeTypeHelper(this); } } #endregion #region Public API /// /// Clones the specified /// The expression to import ///in the context of this command tree, returning a copy of the expression that is owned by this command tree. If the expression is the root of an expression subtree, the entire subtree is cloned. If the expression is already owned by this command tree the original expression is returned. /// If the expression is already owned by this command tree, the original expression. Otherwise, a copy of the expression that is owned by this command tree ////// is null /*CQT_PUBLIC_API(*/internal/*)*/ DbExpression Import(DbExpression source) { if (null == source) { throw EntityUtil.ArgumentNull("source"); } // #433613: PreSharp warning 56506: Parameter 'source.CommandTree' to this public method must be validated: A null-dereference can occur here. if (null == source.CommandTree) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_CommandTree_Import_NullCommandTreeInvalid, "source"); } using(new EntityBid.ScopeAuto(" refers to metadata that is not present in the metadata workspace of this command tree %d#", this.ObjectId)) { EntityBid.Trace(" %d#, source=%d#, %d{cqt.DbExpressionKind}\n", this.ObjectId, DbExpression.GetObjectId(source), DbExpression.GetExpressionKind(source)); if (this == source.CommandTree) { return source; } // Command Trees should only ever exist in one Data Space, the C-Space. //Debug.Assert(source.CommandTree.DataSpace == this.DataSpace, "DbCommandTree DataSpace mismatch"); return ExpressionCopier.Copy(this, source); } } /// /// Verifies that the command tree is valid, including the validity of variable and parameter references. /// ///If the command tree is invalid /*CQT_PUBLIC_API(*/internal/*)*/ void Validate() { using(new EntityBid.ScopeAuto("%d#", this.ObjectId)) { if (!this.ValidationRequired) { return; } this.Validate(new System.Data.Common.CommandTrees.Internal.Validator()); this.SetValid(); } } /// /// Adds a parameter to the set of parameters that may be referenced within this command tree. If a parameter with the same name but a different type already exists on this command tree then an exception will be thrown. /// /// The name of the new parameter /// A description of the type of the new parameter ////// or is null A parameter with the same name but a different type already exists on this command tree. /*CQT_PUBLIC_API(*/internal/*)*/ void AddParameter(string name, TypeUsage type) { EntityUtil.CheckArgumentNull(name, "name"); this.TypeHelper.CheckType(type); using(new EntityBid.ScopeAuto("%d#", this.ObjectId)) { EntityBid.Trace(" %d#, name='%ls'\n", this.ObjectId, name); EntityBid.Trace(" %d#, type='%ls'\n", this.ObjectId, TypeHelpers.GetStrongName(type)); if (!DbCommandTree.IsValidParameterName(name)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_CommandTree_InvalidParameterName(name), "name"); } KeyValuePair foundParam; if (_paramMappings.TryGetValue(name, out foundParam)) { // SQLBUDT#545720: Equivalence is not a sufficient check (consider row types for TVPs) - equality is required. if (!TypeSemantics.IsStructurallyEqualTo(type, foundParam.Value)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_CommandTree_ParameterExists, "type"); } } else { _paramMappings.Add(name, new KeyValuePair (name, type)); } } } /// /// Gets the name and corresponding type of each parameter that can be referenced within this command tree. /// public IEnumerable> Parameters { get { using(new EntityBid.ScopeAuto(" %d#", this.ObjectId)) { Dictionary retParams = new Dictionary (); foreach (KeyValuePair definedParam in _paramMappings.Values) { EntityBid.Trace(" %d#, name='%ls'\n", this.ObjectId, definedParam.Key); EntityBid.Trace(" %d#, type='%ls'\n", this.ObjectId, TypeHelpers.GetStrongName(definedParam.Value)); retParams.Add(definedParam.Key, definedParam.Value); } return retParams; } } } // VersionNumber need only be public when Command Trees are mutable. #if PUBLIC_COMMANDTREE_VERSIONNUMBER /// /// Gets the current version of this DbCommandTree. /// public int VersionNumber { get { return _currentVersion; } } #endif #region Non-DbExpression Factory Methods ////// Creates a new /// The expression to bind /// The variable name that should be used for the binding ///that uses the specified variable name to bind the given expression /// A new expression binding with the specified expression and variable name ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbExpressionBinding CreateExpressionBinding(DbExpression input, string varName) { return new DbExpressionBinding(this, input, varName); } ///is associated with a different command tree /// or does not have a collection result type /// /// Creates a new /// The expression to bind ///that uses a generated variable name to bind the given expression /// A new expression binding with the specified expression and a generated variable name ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbExpressionBinding CreateExpressionBinding(DbExpression input) { return new DbExpressionBinding(this, input, this.BindingAliases.Next()); } ///is associated with a different command tree /// or does not have a collection result type /// /// Creates a new /// The expression to bind /// The variable name that should be used for the binding /// The variable name that should be used to refer to the group when the new group expression binding is used in a group-by expression ///that uses the specified variable name and group variable names to bind the given expression /// A new group expression binding with the specified expression, variable name and group variable name ////// , or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbGroupExpressionBinding CreateGroupExpressionBinding(DbExpression input, string varName, string groupVarName) { return new DbGroupExpressionBinding(this, input, varName, groupVarName); } ///is associated with a different command tree /// or does not have a collection result type /// /// Creates a new group expression binding that uses generated variable and group variable names to bind the given expression /// /// The expression to bind ///A new group expression binding with the specified expression and a generated variable name and group variable name ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbGroupExpressionBinding CreateGroupExpressionBinding(DbExpression input) { string alias = this.BindingAliases.Next(); return new DbGroupExpressionBinding(this, input, alias, string.Format(CultureInfo.InvariantCulture, "Group{0}", alias)); } ///is associated with a different command tree /// or does not have a collection result type /// /// Creates a new /// The expression that defines the sort key /// Specifies whether the sort order is ascending (true) or descending (false) ////// A new sort clause with the given sort key and sort order ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbSortClause CreateSortClause(DbExpression key, bool ascending) { return new DbSortClause(key, ascending, String.Empty); } ///is associated with a different command tree /// or does not have an order-comparable result type /// /// Creates a new /// The expression that defines the sort key /// Specifies whether the sort order is ascending (true) or descending (false) /// The collation to sort under ////// A new sort clause with the given sort key, sort order and collation ////// or is null /// is empty or contains only space characters /// /*CQT_PUBLIC_API(*/internal/*)*/ DbSortClause CreateSortClause(DbExpression key, bool ascending, string collation) { EntityUtil.CheckArgumentNull(collation, "collation"); if (StringUtil.IsNullOrEmptyOrWhiteSpace(collation)) { throw EntityUtil.ArgumentOutOfRange(System.Data.Entity.Strings.Cqt_Sort_EmptyCollationInvalid, "collation"); } return new DbSortClause(key, ascending, collation); } ///is associated with a different command tree or does not have a string result type /// /// Creates a new /// The function that defines the aggregate operation. /// The argument over which the aggregate function should be calculated. ///. /// A new function aggregate with a reference to the given function and argument. The function aggregate's Distinct property will have the value false ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbFunctionAggregate CreateFunctionAggregate(EdmFunction function, DbExpression argument) { return new DbFunctionAggregate(this, function, argument, false); } ///is not associated with this command tree's metadata workspace /// or has more than one argument, /// is associated with a different command tree, or /// the result type of is not equal or promotable to /// the parameter type of /// /// Creates a new /// The function that defines the aggregate operation. /// The argument over which the aggregate function should be calculated. ///that is applied in a distinct fashion. /// A new function aggregate with a reference to the given function and argument. The function aggregate's Distinct property will have the value true ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbFunctionAggregate CreateDistinctFunctionAggregate(EdmFunction function, DbExpression argument) { return new DbFunctionAggregate(this, function, argument, true); } #if ENABLE_NESTAGGREGATE ///is not associated with this command tree's metadata workspace /// or has more than one argument, /// is associated with a different command tree, or /// the result type of is not equal or promotable to /// the parameter type of /// /// Creates a new /// The argument over which to perform the nest operation ///over the specified argument /// A new nest aggregate with a reference to the given argument. ////// is null /*CQT_PUBLIC_API(*/internal/*)*/ NestAggregate CreateNestAggregate(Expression argument) { return new NestAggregate(this, argument); } #endif /// is associated with a different command tree /// Creates a new /// The relationship end from which navigation takes place ///The relationship end to which navigation may be satisifed using the target entity ref ///An expression that produces a reference to the target entity (and must therefore have a Ref result type) internal DbRelatedEntityRef CreateRelatedEntityRef(RelationshipEndMember sourceEnd, RelationshipEndMember targetEnd, DbExpression targetEntity) { return new DbRelatedEntityRef(this, sourceEnd, targetEnd, targetEntity); } #endregion #region DbExpression Factory Methods #region Constants ///that describes how to satisfy the relationship /// navigation operation from to , which /// must be declared by the same relationship type. /// DbRelatedEntityRefs are used in conjuction with /// to construct Entity instances that are capable of resolving relationship navigation operations based on /// the provided DbRelatedEntityRefs without the need for additional navigation operations. /// Note also that this factory method is not intended to be part of the public Command Tree API /// since its intent is to support Entity constructors in view definitions that express information about /// related Entities using the 'WITH RELATIONSHIPS' clause in eSQL. /// /// Creates a new /// The constant value to represent. ///with the given constant value. /// A new DbConstantExpression with the given value. ////// is null /*CQT_PUBLIC_API(*/internal/*)*/ DbConstantExpression CreateConstantExpression(object value) { return new DbConstantExpression(this, value); } /// is not an instance of a valid constant type /// Creates a new /// The constant value to represent. /// The type of the constant value. ///of the specified primitive type with the given constant value. /// A new DbConstantExpression with the given value and a result type of ///. /// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbConstantExpression CreateConstantExpression(object value, TypeUsage constantType) { return new DbConstantExpression(this, value, constantType); } ///is not an instance of a valid constant type, /// does not represent a primitive type, or /// is of a different primitive type than that represented by /// /// Creates a ///with the Boolean value false
. ///A DbConstantExpression with the Boolean value false. /*CQT_PUBLIC_API(*/internal/*)*/ DbConstantExpression CreateFalseExpression() { if (null == _falseExpr) { _falseExpr = this.CreateConstantExpression(false, this.TypeHelper.CreateBooleanResultType()); } return _falseExpr; } ////// Creates a new /// The type of the null value. ///, which represents a typed null value. /// An instance of DbNullExpression ////// is null /*CQT_PUBLIC_API(*/internal/*)*/ DbNullExpression CreateNullExpression(TypeUsage type) { return new DbNullExpression(this, type); } /// is not associated with this command tree's metadata workspace /// Creates a ///with the Boolean value true. /// A DbConstantExpression with the Boolean value /*CQT_PUBLIC_API(*/internal/*)*/ DbConstantExpression CreateTrueExpression() { if (null == _trueExpr) { _trueExpr = this.CreateConstantExpression(true, this.TypeHelper.CreateBooleanResultType()); } return _trueExpr; } #endregion #region Variables ///true
./// Creates a /// The name of the parameter to reference. ///that references the parameter with the given name. /// The parameter must already exist on the command tree. /// A new DbParameterReferenceExpression that references the given parameter. ////// is null If no parameter with the given name exists on the command tree. Parameters can be added to the command tree using the AddParameter method /*CQT_PUBLIC_API(*/internal/*)*/ DbParameterReferenceExpression CreateParameterReferenceExpression(string name) { if (null == name) { throw EntityUtil.ArgumentNull("name"); } // // Attempt to find a parameter with the specified name in the parameters dictionary. // If the name is not valid the dictionary is not consulted since no parameter with // an invalid name should ever be added to the dictionary. // Note that when the ParameterRefExpression is created the name that is used is the // exact name that the parameter was originally created with (from the dictionary) // since this is the name the Validator will use (in an exact match) to determine // whether a given ParameterRefExpression is valid or not. // KeyValuePairfoundParam; if (DbCommandTree.IsValidParameterName(name) && _paramMappings.TryGetValue(name, out foundParam)) { return new DbParameterReferenceExpression(this, foundParam.Value, foundParam.Key); } throw EntityUtil.ArgumentOutOfRange(System.Data.Entity.Strings.Cqt_CommandTree_NoParameterExists, "name"); } /// /// Creates a /// The name of the variable to reference. /// The type of the variable to reference. ///that references a variable with the given name and type. /// A new DbVariableReferenceExpression that references the specified variable. ////// or is null /*CQT_PUBLIC_API(*/internal/*)*/ DbVariableReferenceExpression CreateVariableReferenceExpression(string varName, TypeUsage varType) { return new DbVariableReferenceExpression(this, varType, varName); } #endregion #region Member Access #if METHOD_EXPRESSION /// is not associated with this command tree's metadata workspace /// Creates a new /// The metadata for the method to invoke. /// The invocation target. /// The arguments to the method. ///representing the invocation of the specified method on the given instance with the given arguments. /// A new MethodExpression that represents the method invocation. ////// ///or is null, /// or is null or contains null /// /// /*CQT_PUBLIC_API(*/internal/*)*/ MethodExpression CreateInstanceMethodExpression(MethodMetadata methodInfo, Expression instance, IListis not associated with this command tree's metadata workspace, /// is associated with a different command tree /// or has a result type that is not equal or promotable to the declaring type of the method, /// or contains an incorrect number of expressions, /// an expression with a result type that is not equal or promotable to the type of the corresponding /// method parameter, or an expression that is associated with a different command tree. /// args) { if (methodInfo != null && methodInfo.IsStatic) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Factory_InstanceMethodRequired, "methodInfo"); } return new MethodExpression(this, methodInfo, args, instance); } /// /// Creates a new /// The metadata for the method to invoke. /// The arguments to the method. ///representing the invocation of the specified method with the given arguments. /// A new MethodExpression that represents the method invocation. ////// is null, or is null or contains null /// /*CQT_PUBLIC_API(*/internal/*)*/ MethodExpression CreateStaticMethodExpression(MethodMetadata methodInfo, IListis not associated with this command tree's metadata workspace, /// or contains an incorrect number of expressions, /// an expression with a result type that is not equal or promotable to the type of the corresponding /// method parameter, or an expression that is associated with a different command tree. /// args) { if (methodInfo != null && !methodInfo.IsStatic) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Factory_StaticMethodRequired, "methodInfo"); } return new MethodExpression(this, methodInfo, args, null); } #endif /// /// Creates a new /// The name of the property to retrieve. /// The instance from which to retrieve the property. ///representing the retrival of the instance property with the specified name from the given instance. /// A new DbPropertyExpression that represents the property retrieval ////// is null or is null and the property is not static. No property with the specified name is declared by the type of ///. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbPropertyExpression CreatePropertyExpression(string propertyName, DbExpression instance) { return CreatePropertyExpression(propertyName, false, instance); } ///is associated with a different command tree, or is non-null and the property is static. /// /// Creates a new /// The name of the property to retrieve. /// Ifrepresenting the retrival of the instance property with the specified name from the given instance. /// true , a case-insensitive member lookup ofis performed; otherwise a member with a name that is an exact match must be declared by the type of . /// The instance from which to retrieve the property. /// A new DbPropertyExpression that represents the property retrieval ////// is null or is null and the property is not static. No property with the specified name (determined with respect to ///) is declared by the type of . /// /*CQT_PUBLIC_API(*/internal/*)*/ DbPropertyExpression CreatePropertyExpression(string propertyName, bool ignoreCase, DbExpression instance) { EntityUtil.CheckArgumentNull(propertyName, "propertyName"); EntityUtil.CheckArgumentNull(instance, "instance"); // // EdmProperty, NavigationProperty and RelationshipEndMember are the only valid members for DbPropertyExpression. // Since these all derive from EdmMember they are declared by subtypes of StructuralType, // so a non-StructuralType instance is invalid. // StructuralType structType; if(TypeHelpers.TryGetEdmTypeis associated with a different command tree, or is non-null and the property is static. /// (instance.ResultType, out structType)) { // // Does the type declare a member with the given name? // EdmMember foundMember; if(structType.Members.TryGetValue(propertyName, ignoreCase, out foundMember)) { // // If the member is a RelationshipEndMember, call the corresponding overload. // if (Helper.IsRelationshipEndMember(foundMember)) { return this.CreatePropertyExpression((RelationshipEndMember)foundMember, instance); } // // Otherwise if the member is an EdmProperty, call that overload instead. // if (Helper.IsEdmProperty(foundMember)) { return this.CreatePropertyExpression((EdmProperty)foundMember, instance); } // // The only remaining valid option is NavigationProperty. // if (Helper.IsNavigationProperty(foundMember)) { return this.CreatePropertyExpression((NavigationProperty)foundMember, instance); } } } throw EntityUtil.ArgumentOutOfRange(System.Data.Entity.Strings.Cqt_Factory_NoSuchProperty, "propertyName"); } /// /// Creates a new /// Metadata for the property to retrieve. /// The instance from which to retrieve the property. May be null if the property is static. ///representing the retrival of the specified property. /// A new DbPropertyExpression representing the property retrieval. ////// is null or is null and the property is not static. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbPropertyExpression CreatePropertyExpression(EdmProperty propertyInfo, DbExpression instance) { return new DbPropertyExpression(this, propertyInfo, instance); } ///is not associated with this command tree's metadata workspace, /// or is associated with a different command tree, or is non-null and the property is static. /// /// Creates a new /// Metadata for the relationship end member to retrieve. /// The instance from which to retrieve the relationship end member. ///representing the retrival of the specified relationship end member. /// A new DbPropertyExpression representing the relationship end member retrieval. ////// is null or is null and the property is not static. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbPropertyExpression CreatePropertyExpression(RelationshipEndMember memberInfo, DbExpression instance) { return new DbPropertyExpression(this, memberInfo, instance); } ///is not associated with this command tree's metadata workspace, /// or is associated with a different command tree. /// /// Creates a new /// Metadata for the navigation property to retrieve. /// The instance from which to retrieve the navigation property. ///representing the retrival of the specified navigation property. /// A new DbPropertyExpression representing the navigation property retrieval. ////// is null or is null. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbPropertyExpression CreatePropertyExpression(NavigationProperty propertyInfo, DbExpression instance) { return new DbPropertyExpression(this, propertyInfo, instance); } ///is not associated with this command tree's metadata workspace, /// or is associated with a different command tree. /// /// Creates a new /// Metadata for the property that represents the end of the relationship from which navigation should occur /// Metadata for the property that represents the end of the relationship to which navigation should occur /// An expression the specifies the instance from which naviagtion should occur ///representing the navigation of a composition or association relationship. /// A new DbRelationshipNavigationExpression representing the navigation of the specified from and to relation ends of the specified relation type from the specified navigation source instance ////// , or is null /// ///and are not declared by the same relationship type /// or are not associated with this command tree's metadata workspace, or is associated /// with a different command tree or has a result type that is not compatible with the property type of . /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbRelationshipNavigationExpression CreateRelationshipNavigationExpression(RelationshipEndMember fromEnd, RelationshipEndMember toEnd, DbExpression from) { return new DbRelationshipNavigationExpression(this, fromEnd, toEnd, from); } ///requires that navigation always occur from a reference, and so must always have a reference result type. /// /// Creates a new /// Metadata for the relation type that represents the relationship /// The name of the property of the relation type that represents the end of the relationship from which navigation should occur /// The name of the property of the relation type that represents the end of the relationship to which navigation should occur /// An expression the specifies the instance from which naviagtion should occur ///representing the navigation of a composition or association relationship. /// A new DbRelationshipNavigationExpression representing the navigation of the specified from and to relation ends of the specified relation type from the specified navigation source instance ////// ///, , or is null. /// /// ///is not associated with this command tree's metadata workspace or is associated with a different command tree, /// or does not declare a relation end property with name or , /// or has a result type that is not compatible with the property type of the relation end property with name . /// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] /*CQT_PUBLIC_API(*/internal/*)*/ DbRelationshipNavigationExpression CreateRelationshipNavigationExpression(RelationshipType type, string fromEndName, string toEndName, DbExpression from) { return new DbRelationshipNavigationExpression(this, type, fromEndName, toEndName, from); } #endregion #region EdmFunction Invocation (including Lambda) ///requires that navigation always occur from a reference, and so must always have a reference result type. /// /// Creates a new /// Metadata for the function to invoke. /// A list of expressions that provide the arguments to the function. ///representing the invocation of the specified function with the given arguments. /// A new DbFunctionExpression representing the function invocation. ////// ///is null, or is null or contains null. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbFunctionExpression CreateFunctionExpression(EdmFunction function, IListis not associated with this command tree's metadata workspace, /// the count of does not equal the number of parameters declared by , /// or contains an expression that is associated with a different command tree or has /// a result type that is not equal or promotable to the corresponding function parameter type. /// args) { return new DbFunctionExpression(this, function, null, args); } /// /// Creates a /// The names and types of the formal arguments to the Lambda function /// An expression that defines the logic of the Lambda function /// A list of expressions that provides the values on which the function is invoked. ///that represents the invocation of the inline (Lambda) function described by the given formal parameters and body expression. /// A new DbFunctionExpression that invokes the specified Lambda function with the given arguments ////// , oris null or contains an element with a null name or type, /// is null is null or contains null. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbFunctionExpression CreateLambdaFunctionExpression(IEnumerablecontains an element with a type associated with a different metadata workspace, /// is associated with a different command tree, or constains /// an expression that is associated with a different command tree. /// > formals, DbExpression body, IList args) { EntityUtil.CheckArgumentNull(formals, "formals"); EntityUtil.CheckArgumentNull(body, "body"); List funcParams = new List (); List > formalArgs = new List >(formals); int idx = 0; CommandTreeUtils.CheckNamedList ( "formals", formalArgs, true, delegate(KeyValuePair formal, int index) { this.TypeHelper.CheckType(formal.Value, CommandTreeUtils.FormatIndex("formals", idx)); idx++; funcParams.Add(new FunctionParameter(formal.Key, formal.Value, ParameterMode.In)); } ); FunctionParameter retParam = new FunctionParameter("Return", body.ResultType, ParameterMode.ReturnValue); EdmFunction lambdaFunction = new EdmFunction(DbFunctionExpression.LambdaFunctionName, DbFunctionExpression.LambdaFunctionNameSpace, retParam.TypeUsage.EdmType.DataSpace, new EdmFunctionPayload { ReturnParameter = retParam, Parameters = funcParams.ToArray(), }); lambdaFunction.SetReadOnly(); return new DbFunctionExpression(this, lambdaFunction, body, args); } #endregion #region Arithmetic Operators /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that divides the left argument by the right argument. /// A new DbArithmeticExpression representing the division operation. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbArithmeticExpression CreateDivideExpression(DbExpression left, DbExpression right) { return new DbArithmeticExpression(this, DbExpressionKind.Divide, CommandTreeUtils.CreateListor is associated with a different command tree, /// or no common numeric result type exists between them. /// (left, right)); } /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that subtracts the right argument from the left argument. /// A new DbArithmeticExpression representing the subtraction operation. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbArithmeticExpression CreateMinusExpression(DbExpression left, DbExpression right) { return new DbArithmeticExpression(this, DbExpressionKind.Minus, CommandTreeUtils.CreateListor is associated with a different command tree, /// or no common numeric result type exists between them. /// (left, right)); } /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that computes the remainder of the left argument divided by the right argument. /// A new DbArithmeticExpression representing the modulo operation. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbArithmeticExpression CreateModuloExpression(DbExpression left, DbExpression right) { return new DbArithmeticExpression(this, DbExpressionKind.Modulo, CommandTreeUtils.CreateListor is associated with a different command tree, /// or no common numeric result type exists between them. /// (left, right)); } /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that multiplies the left argument by the right argument. /// A new DbArithmeticExpression representing the multiplication operation. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbArithmeticExpression CreateMultiplyExpression(DbExpression left, DbExpression right) { return new DbArithmeticExpression(this, DbExpressionKind.Multiply, CommandTreeUtils.CreateListor is associated with a different command tree, /// or no common numeric result type exists between them. /// (left, right)); } /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that adds the left argument to the right argument. /// A new DbArithmeticExpression representing the addition operation. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbArithmeticExpression CreatePlusExpression(DbExpression left, DbExpression right) { return new DbArithmeticExpression(this, DbExpressionKind.Plus, CommandTreeUtils.CreateListor is associated with a different command tree, /// or no common numeric result type exists between them. /// (left, right)); } /// /// Creates a new /// An expression that specifies the argument. ///that negates the value of the argument. /// A new DbArithmeticExpression representing the negation operation. ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbArithmeticExpression CreateUnaryMinusExpression(DbExpression argument) { return new DbArithmeticExpression(this, DbExpressionKind.UnaryMinus, CommandTreeUtils.CreateListis associated with a different command tree, /// or has a non-numeric result type. /// (argument)); } #endregion #region Comparison Operators /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that compares the left and right arguments for equality. /// A new DbComparisonExpression representing the equality comparison. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbComparisonExpression CreateEqualsExpression(DbExpression left, DbExpression right) { return new DbComparisonExpression(this, DbExpressionKind.Equals, left, right); } ///or is associated with a different command tree, /// or no common equality-comparable result type exists between them. /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that determines whether the left argument is greater than the right argument. /// A new DbComparisonExpression representing the greater-than comparison. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbComparisonExpression CreateGreaterThanExpression(DbExpression left, DbExpression right) { return new DbComparisonExpression(this, DbExpressionKind.GreaterThan, left, right); } ///or is associated with a different command tree, /// or no common order-comparable result type exists between them. /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that determines whether the left argument is greater than or equal to the right argument. /// A new DbComparisonExpression representing the greater-than-or-equal-to comparison. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbComparisonExpression CreateGreaterThanOrEqualsExpression(DbExpression left, DbExpression right) { return new DbComparisonExpression(this, DbExpressionKind.GreaterThanOrEquals, left, right); } ///or is associated with a different command tree, /// or no common result type that is both equality- and order-comparable exists between them. /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that determines whether the left argument is less than the right argument. /// A new DbComparisonExpression representing the less-than comparison. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbComparisonExpression CreateLessThanExpression(DbExpression left, DbExpression right) { return new DbComparisonExpression(this, DbExpressionKind.LessThan, left, right); } ///or is associated with a different command tree, /// or no common order-comparable result type exists between them. /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that determines whether the left argument is less than or equal to the right argument. /// A new DbComparisonExpression representing the less-than-or-equal-to comparison. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbComparisonExpression CreateLessThanOrEqualsExpression(DbExpression left, DbExpression right) { return new DbComparisonExpression(this, DbExpressionKind.LessThanOrEquals, left, right); } ///or is associated with a different command tree, /// or no common result type that is both equality- and order-comparable exists between them. /// /// Creates a new /// An expression that specifies the left argument. /// An expression that specifies the right argument. ///that compares the left and right arguments for inequality. /// A new DbComparisonExpression representing the inequality comparison. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbComparisonExpression CreateNotEqualsExpression(DbExpression left, DbExpression right) { return new DbComparisonExpression(this, DbExpressionKind.NotEquals, left, right); } #endregion #region Boolean Connectors ///or is associated with a different command tree, /// or no common equality-comparable result type exists between them. /// /// Creates an /// A Boolean expression that specifies the left argument. /// A Boolean expression that specifies the right argument. ///that performs the logical And of the left and right arguments. /// A new DbAndExpression with the specified arguments. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbAndExpression CreateAndExpression(DbExpression left, DbExpression right) { return new DbAndExpression(this, left, right); } ///or is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates an /// A Boolean expression that specifies the left argument. /// A Boolean expression that specifies the right argument. ///that performs the logical Or of the left and right arguments. /// A new DbOrExpression with the specified arguments. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbOrExpression CreateOrExpression(DbExpression left, DbExpression right) { return new DbOrExpression(this, left, right); } ///or is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates a /// A Boolean expression that specifies the argument. ///that performs the logical negation of the given argument. /// A new DbNotExpression with the specified argument. ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbNotExpression CreateNotExpression(DbExpression argument) { return new DbNotExpression(this, argument); } #endregion #region Relational Operators ///is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates a new /// An expression binding that specifies the input set. /// An expression representing a predicate to evaluate for each member of the input set. ///that determines whether the given predicate holds for any element of the input set. /// A new DbQuantifierExpression that represents the Any operation. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbQuantifierExpression CreateAnyExpression(DbExpressionBinding input, DbExpression predicate) { return new DbQuantifierExpression(this, DbExpressionKind.Any, input, predicate); } ///or is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates a new /// An expression binding that specifies the input set. /// An expression representing a predicate to evaluate for each member of the input set. ///that determines whether the given predicate holds for all elements of the input set. /// A new DbQuantifierExpression that represents the All operation. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbQuantifierExpression CreateAllExpression(DbExpressionBinding input, DbExpression predicate) { return new DbQuantifierExpression(this, DbExpressionKind.All, input, predicate); } ///or is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates a new /// Anthat evaluates the given expression once for each element of a given input set, /// producing a collection of rows with corresponding input and apply columns. Rows for which evaluates to an empty set are not included. /// that specifies the input set. /// An that specifies logic to evaluate once for each member of the input set. /// An new DbApplyExpression with the specified input and apply bindings and an ///of CrossApply. /// or is null /*CQT_PUBLIC_API(*/internal/*)*/ DbApplyExpression CreateCrossApplyExpression(DbExpressionBinding input, DbExpressionBinding apply) { return new DbApplyExpression(this, input, apply, DbExpressionKind.CrossApply); } /// or is associated with a different command tree /// Creates a new /// Anthat evaluates the given expression once for each element of a given input set, /// producing a collection of rows with corresponding input and apply columns. Rows for which evaluates to an empty set have an apply column value of null
. ///that specifies the input set. /// An that specifies logic to evaluate once for each member of the input set. /// An new DbApplyExpression with the specified input and apply bindings and an ///of OuterApply. /// or is null /*CQT_PUBLIC_API(*/internal/*)*/ DbApplyExpression CreateOuterApplyExpression(DbExpressionBinding input, DbExpressionBinding apply) { return new DbApplyExpression(this, input, apply, DbExpressionKind.OuterApply); } /// or is associated with a different command tree /// Creates a new /// An expression that defines the set over which to perfom the distinct operation. ///that removes duplicates from the given set argument. /// A new DbDistinctExpression that represents the distinct operation applied to the specified set argument. ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbDistinctExpression CreateDistinctExpression(DbExpression argument) { return new DbDistinctExpression(this, argument); } ///is associated with a different command tree, /// or does not have a collection result type. /// /// Creates a new /// An expression that specifies the input set. ///that converts a single-member set into a singleton. /// A DbElementExpression that represents the conversion of the single-member set argument to a singleton. ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbElementExpression CreateElementExpression(DbExpression argument) { return new DbElementExpression(this, argument, false); } ///is associated with a different command tree, /// or does not have a collection result type. /// /// Creates a new /// An expression that specifies the input set. ///that converts a single-member set with a single property /// into a singleton. The result type of the created equals the result type /// of the single property of the element of the argument. /// /// This method should only be used when the argument is of a collection type with /// element of structured type with only one property. /// A DbElementExpression that represents the conversion of the single-member set argument to a singleton. ////// is null /// internal DbElementExpression CreateElementExpressionUnwrapSingleProperty(DbExpression argument) { return new DbElementExpression(this, argument, true); } ///is associated with a different command tree, /// or does not have a collection result type, or its element type is not a structured type /// with only one property /// /// Creates a new /// An expression that defines the left set argument. /// An expression that defines the right set argument. ///that computes the subtraction of the right set argument from the left set argument. /// A new DbExceptExpression that represents the difference of the left argument from the right argument. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbExceptExpression CreateExceptExpression(DbExpression left, DbExpression right) { return new DbExceptExpression(this, left, right); } ///or is associated with a different command tree, /// or no common collection result type exists between them. /// /// Creates a new /// An expression binding that specifies the input set. /// An expression representing a predicate to evaluate for each member of the input set. ///that filters the elements in the given input set using the specified predicate. /// A new DbFilterExpression that produces the filtered set. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbFilterExpression CreateFilterExpression(DbExpressionBinding input, DbExpression predicate) { return new DbFilterExpression(this, input, predicate); } ///or is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates a new /// Athat groups the elements of the input set according to the specified group keys and applies the given aggregates. /// that specifies the input set. /// A list of string-expression pairs that define the grouping columns. /// A list of expressions that specify aggregates to apply. /// A new DbGroupByExpression with the specified input set, grouping keys and aggregates. ////// ///, or is null, /// contains a null key column name or expression, or /// contains a null aggregate column name or aggregate. /// /// ///is associated with a different command tree, /// both and are empty, /// contains an expression that is associated with a different command tree, /// or contains an aggregate that is not associated with the command tree's metadata workspace, /// or an invalid or duplicate column name was specified. /// /// DbGroupByExpression allows either the list of keys or the list of aggregates to be empty, but not both. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbGroupByExpression CreateGroupByExpression(DbGroupExpressionBinding input, IList> keys, IList > aggregates) { return new DbGroupByExpression(this, input, keys, aggregates); } /// /// Creates a new /// An expression that defines the left set argument. /// An expression that defines the right set argument. ///that computes the intersection of the left and right set arguments. /// A new DbIntersectExpression that represents the intersection of the left and right arguments. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbIntersectExpression CreateIntersectExpression(DbExpression left, DbExpression right) { return new DbIntersectExpression(this, left, right); } ///or is associated with a different command tree, /// or no common collection result type exists between them. /// /// Creates a new /// A list of expression bindings that specifies the input sets. ///that unconditionally joins the sets specified by the list of input expression bindings. /// A new DbCrossJoinExpression, with an ///of CrossJoin, that represents the unconditional join of the input sets. /// is null or contains null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbCrossJoinExpression CreateCrossJoinExpression(IListcontains fewer than 2 expression bindings, /// or contains an expression binding that is associated with a different command tree. /// inputs) { return new DbCrossJoinExpression(this, inputs); } /// /// Creates a new /// Anthat joins the sets specified by the left and right /// expression bindings, on the specified join condition, using InnerJoin as the . /// that specifies the left set argument. /// An that specifies the right set argument. /// An expression that specifies the condition on which to join. /// /// A new DbJoinExpression, with an ///of InnerJoin, that represents the inner join operation applied to the left and right /// input sets under the given join condition. /// /// ///, or is null. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbJoinExpression CreateInnerJoinExpression(DbExpressionBinding left, DbExpressionBinding right, DbExpression joinCondition) { return new DbJoinExpression(this, DbExpressionKind.InnerJoin, left, right, joinCondition); } ///, or is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates a new /// Anthat joins the sets specified by the left and right /// expression bindings, on the specified join condition, using LeftOuterJoin as the . /// that specifies the left set argument. /// An that specifies the right set argument. /// An expression that specifies the condition on which to join. /// /// A new DbJoinExpression, with an ///of LeftOuterJoin, that represents the left outer join operation applied to the left and right /// input sets under the given join condition. /// /// ///, or is null. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbJoinExpression CreateLeftOuterJoinExpression(DbExpressionBinding left, DbExpressionBinding right, DbExpression joinCondition) { return new DbJoinExpression(this, DbExpressionKind.LeftOuterJoin, left, right, joinCondition); } ///, or is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates a new /// Anthat joins the sets specified by the left and right /// expression bindings, on the specified join condition, using FullOuterJoin as the . /// that specifies the left set argument. /// An that specifies the right set argument. /// An expression that specifies the condition on which to join. /// /// A new DbJoinExpression, with an ///of FullOuterJoin, that represents the full outer join operation applied to the left and right /// input sets under the given join condition. /// /// ///, or is null. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbJoinExpression CreateFullOuterJoinExpression(DbExpressionBinding left, DbExpressionBinding right, DbExpression joinCondition) { return new DbJoinExpression(this, DbExpressionKind.FullOuterJoin, left, right, joinCondition); } ///, or is associated with a different command tree, /// or does not have a Boolean result type. /// /// Creates a new /// An expression binding that specifies the input set. /// An expression to project over the set. ///that projects the specified expression over the given input set. /// A new DbProjectExpression that represents the projection operation. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbProjectExpression CreateProjectExpression(DbExpressionBinding input, DbExpression projection) { return new DbProjectExpression(this, input, projection); } ///or is associated with a different command tree. /// /// Creates a new /// An expression binding that specifies the input set. /// A list of sort specifications that determine how the elements of the input set should be sorted. /// An expression the specifies how many elements of the ordered set to skip. ///that sorts the given input set by the given sort specifications before skipping the specified number of elements. /// A new DbSkipExpression that represents the skip operation. ////// ///, or is null, /// or contains null. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbSkipExpression CreateSkipExpression(DbExpressionBinding input, IListor is associated with a different command tree, /// is empty or contains a that is associated with a different command tree, /// or is not or or has a /// result type that is not equal or promotable to a 64-bit integer type. /// sortOrder, DbExpression count) { return new DbSkipExpression(this, input, sortOrder, count); } /// /// Creates a new /// An expression binding that specifies the input set. /// A list of sort specifications that determine how the elements of the input set should be sorted. ///that sorts the given input set by the specified sort specifications. /// A new DbSortExpression that represents the sort operation. ////// ///or is null, /// or contains null. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbSortExpression CreateSortExpression(DbExpressionBinding input, IListis associated with a different command tree, /// or is empty or contains a that is associated with a different command tree. /// sortOrder) { return new DbSortExpression(this, input, sortOrder); } /// /// Creates a new /// An expression that defines the left set argument. /// An expression that defines the right set argument. ///that computes the union of the left and right set arguments and does not remove duplicates. /// A new DbUnionAllExpression that union, including duplicates, of the the left and right arguments. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbUnionAllExpression CreateUnionAllExpression(DbExpression left, DbExpression right) { return new DbUnionAllExpression(this, left, right); } #endregion #region General Operators ///or is associated with a different command tree, /// or no common collection result type exists between them. /// /// Creates a new /// A list of expressions that provide the conditional for of each case. /// A list of expressions that provide the result of each case. /// An expression that defines the result when no case is matched. ///. /// A new DbCaseExpression with the specified cases and default result. ////// ///or is null or contains null, /// or is null. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbCaseExpression CreateCaseExpression(IListor is empty or contains an expression associated with a different command tree, /// is associated with a different command tree, or no common result type exists for all expressions in /// and . /// whenExpressions, IList thenExpressions, DbExpression elseExpression) { return new DbCaseExpression(this, whenExpressions, thenExpressions, elseExpression); } /// /// Creates a new /// The argument to which the cast should be applied. /// Type metadata that specifies the type to cast to. ///that applies a cast operation to a polymorphic argument. /// A new DbCastExpression with the specified argument and target type. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbCastExpression CreateCastExpression(DbExpression argument, TypeUsage toType) { return new DbCastExpression(this, toType, argument); } ///is associated with a different command tree, /// is not associated with this command tree's metadata workspace, /// or the specified cast is not valid. /// /// Creates a new /// Metadata for the entity or relationship set to reference. ///that references the specified entity or relationship set. /// A new DbScanExpression based on the specified entity or relationship set. ////// is null /*CQT_PUBLIC_API(*/internal/*)*/ DbScanExpression CreateScanExpression(EntitySetBase target) { return new DbScanExpression(this, target); } /// is not associated with the metadata workspace of this command tree /// Creates a new /// An expression that specifies the input set ///that determines whether the specified set argument is an empty set. /// A new DbIsEmptyExpression with the specified argument. ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbIsEmptyExpression CreateIsEmptyExpression(DbExpression argument) { return new DbIsEmptyExpression(this, argument); } ///is associated with a different command tree, /// or does not have a collection result type. /// /// Creates a new /// An expression that specifies the argument. ///that determines whether the specified argument is null. /// A new DbIsNullExpression with the specified argument. ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbIsNullExpression CreateIsNullExpression(DbExpression argument) { return new DbIsNullExpression(this, argument, false); } ///is associated with a different command tree, or /// has a collection result type. /// /// Creates a new /// An expression that specifies the argument. ///that determines whether the specified argument is null. /// Used only by span rewriter, when a row could is specified as an argument /// A new DbIsNullExpression with the specified argument. ////// is null /// internal DbIsNullExpression CreateIsNullExpressionAllowingRowTypeArgument(DbExpression argument) { return new DbIsNullExpression(this, argument, true); } ///is associated with a different command tree, or /// has a collection result type. /// /// Creates a new /// An expression that specifies the instance. /// Type metadata that specifies the type that the instance's result type should be compared to. ///that determines whether the given argument is of the specified type or a subtype. /// A new DbIsOfExpression with the specified instance and type and DbExpressionKind IsOf. ////// or is null /// ///is associated with a different command tree, /// is not associated with this command tree's metadata workspace, or /// is not in the same type hierarchy as the result type of . /// /// DbIsOfExpression requires that /*CQT_PUBLIC_API(*/internal/*)*/ DbIsOfExpression CreateIsOfExpression(DbExpression argument, TypeUsage type) { return new DbIsOfExpression(this, DbExpressionKind.IsOf, type, argument); } ///has a polymorphic result type, /// and that is a type from the same type hierarchy as that result type. /// /// Creates a new /// An expression that specifies the instance. /// Type metadata that specifies the type that the instance's result type should be compared to. ///expression that determines whether the given argument is of the specified type, and only that type (not a subtype). /// A new DbIsOfExpression with the specified instance and type and DbExpressionKind IsOfOnly. ////// or is null /// ///is associated with a different command tree, /// is not associated with this command tree's metadata workspace, or /// is not in the same type hierarchy as the result type of . /// /// DbIsOfExpression requires that /*CQT_PUBLIC_API(*/internal/*)*/ DbIsOfExpression CreateIsOfOnlyExpression(DbExpression argument, TypeUsage type) { return new DbIsOfExpression(this, DbExpressionKind.IsOfOnly, type, argument); } ///has a polymorphic result type, /// and that is a type from the same type hierarchy as that result type. /// /// Creates a new /// An expression that specifies the input string. /// An expression that specifies the pattern string. ///that compares the specified input string to the given pattern. /// A new DbLikeExpression with the specified input, pattern and a null escape. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbLikeExpression CreateLikeExpression(DbExpression input, DbExpression pattern) { return new DbLikeExpression(this, input, pattern, null); } ///or is associated with a different command tree, /// or does not have a string result type. /// /// Creates a new /// An expression that specifies the input string. /// An expression that specifies the pattern string. /// An optional expression that specifies the escape string. ///that compares the specified input string to the given pattern using the optional escape. /// A new DbLikeExpression with the specified input, pattern and escape. ////// , or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbLikeExpression CreateLikeExpression(DbExpression input, DbExpression pattern, DbExpression escape) { EntityUtil.CheckArgumentNull(escape, "escape"); return new DbLikeExpression(this, input, pattern, escape); } ///, or is associated with a different command tree, /// or does not have a string result type. /// /// Creates a new /// An expression that specifies the input collection. /// An expression that specifies the limit value. ///that restricts the number of elements in the Argument collection to the specified Limit value. /// Tied results are not included in the output. /// A new DbLimitExpression with the specified argument and limit values that does not include tied results. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbLimitExpression CreateLimitExpression(DbExpression argument, DbExpression limit) { return new DbLimitExpression(this, argument, limit, false); } ///is associated with a different command tree or does not have a collection result type, /// or is associated with a different command tree or does not have a result type that is equal or promotable to a 64-bit integer type. /// /// Creates a new /// An expression that specifies the input collection. /// An expression that specifies the limit value. ///that restricts the number of elements in the Argument collection to the specified Limit value, /// including tied results in the output. /// A new DbLimitExpression with the specified argument and limit values that includes tied results. ////// or is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbLimitExpression CreateLimitWithTiesExpression(DbExpression argument, DbExpression limit) { return new DbLimitExpression(this, argument, limit, true); } ///is associated with a different command tree or does not have a collection result type, /// or is associated with a different command tree or does not have a result type that is equal or promotable to a 64-bit integer type. /// /// Creates a new /// The type of the new instance. /// Expressions that specify values of the new instances, interpreted according to the instance's type. ///. If the type argument is a collection type, the arguments specify the elements of the collection. Otherwise the arguments are used as property or column values in the new instance. /// A new DbNewInstanceExpression with the specified type and arguments. ////// or is null, or contains null /// ///is not associated with this command tree's metadata workspace, /// is empty or contains an expression associated with a different command tree, /// or the result types of the contained expressions do not match the requirements of (as explained in the remarks section). /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbNewInstanceExpression CreateNewInstanceExpression(TypeUsage type, IList/// if ///is a a collection type then every expression in must have a result type that is promotable to the element type of the . /// /// if ///is a row type, must contain as many expressions as there are columns in the record /// type, and the result type of each expression must be equal or promotable to the type of the corresponding column. A row type that does not declare any columns is invalid. /// /// if ///is an entity type, must contain as many expressions as there are properties defined by the type, /// and the result type of each expression must be equal or promotable to the type of the corresponding property. /// args) { return new DbNewInstanceExpression(this, type, args); } /// /// Creates a new /// A list of string-DbExpression key-value pairs that defines the structure and values of the row. ///that produces a row with the specified named columns and the given values, specified as expressions. /// A new DbNewInstanceExpression that represents the construction of the row. ////// is null or contains an element with a null column name or expression /// /*CQT_PUBLIC_API(*/internal/*)*/ DbNewInstanceExpression CreateNewRowExpression(IListis empty, contains a duplicate or invalid column name, /// or contains an expression that is associated with a different command tree. /// > recordColumns) { List args = new List (); List > colTypes = new List >(); CommandTreeUtils.CheckNamedList ( "recordColumns", recordColumns, false, // Don't allow empty list delegate(KeyValuePair exprInfo, int index) { // Use ExpressionLink for standard expression argument validation ExpressionLink testLink = new ExpressionLink(CommandTreeUtils.FormatIndex("recordColumns", index), this, exprInfo.Value); args.Add(exprInfo.Value); colTypes.Add(new KeyValuePair (exprInfo.Key, exprInfo.Value.ResultType)); } ); TypeUsage resultType = CommandTreeTypeHelper.CreateResultType(TypeHelpers.CreateRowType(colTypes)); return new DbNewInstanceExpression(this, resultType, args); } /// /// Creates a new /// A list of expressions that provide the elements of the collection ///that constructs a collection containing the specified elements. The type of the collection is based on the common type of the elements. If no common element type exists an exception is thrown. /// A new DbNewInstanceExpression with the specified collection type and arguments. ////// is null, or contains null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbNewInstanceExpression CreateNewCollectionExpression(IListis empty, contains an expression that is not associated with this command tree, /// or contains expressions for which no common result type exists. /// collectionElements) { if (null == collectionElements) { throw EntityUtil.ArgumentNull("collectionElements"); } if (collectionElements.Count < 1) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Factory_NewCollectionElementsRequired, "collectionElements"); } // // Use an ExpressionList to determine the common element type of the collection // (Note that this ExpressionList is not used by the DbNewInstanceExpression itself) // ExpressionList elements = new ExpressionList("collectionElements", this, collectionElements.Count); elements.SetElements(collectionElements); TypeUsage commonElementType = elements.GetCommonElementType(); if (TypeSemantics.IsNullOrNullType(commonElementType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Factory_NewCollectionInvalidCommonType, "collectionElements"); } TypeUsage resultType = CommandTreeTypeHelper.CreateCollectionResultType(commonElementType); return new DbNewInstanceExpression(this, resultType, elements); } /// /// Creates a new /// The type metadata for the collection to create ///that constructs an empty collection of the specified collection type. /// A new DbNewInstanceExpression with the specified collection type and an empty ///Arguments
list./// is null /*CQT_PUBLIC_API(*/internal/*)*/ DbNewInstanceExpression CreateNewEmptyCollectionExpression(TypeUsage collectionType) { EntityUtil.CheckArgumentNull(collectionType, "collectionType"); if (!TypeSemantics.IsCollectionType(collectionType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_NewInstance_CollectionTypeRequired, "collectionType"); } return new DbNewInstanceExpression(this, collectionType, null); } /// is not associated with this command tree's metadata workspace /// Creates a new /// The type of the Entity instance that is being constructed /// Values for each (non-relationship) property of the Entity /// A (possibly empty) list ofthat constructs an instance of an Entity type /// together with the specified information about Entities related to the newly constructed Entity by' /// relationship navigations where the target end has multiplicity of at most one. /// Note that this factory method is not intended to be part of the public Command Tree API since it /// its intent is to support Entity constructors in view definitions that express information about /// related Entities using the 'WITH RELATIONSHIPS' clause in eSQL. /// s that describe Entities that are related to the constructed Entity by various relationship types. /// A new DbNewInstanceExpression that represents the construction of the Entity, and includes the specified related Entity information in the see internal DbNewInstanceExpression CreateNewEntityWithRelationshipsExpression(EntityType instanceType, IListcollection. attributeValues, IList relationships) { return new DbNewInstanceExpression(this, instanceType, attributeValues, relationships); } /// /// Creates a new /// Anthat produces a set consisting of the elements of the given input set that are of the specified type. /// that specifies the input set. /// Type metadata for the type that elements of the input set must have to be included in the resulting set. /// A new DbOfTypeExpression with the specified set argument and type, and an ExpressionKind of ///. /// or is null /// ///is associated with a different command tree or does not have a collection result type, /// is not associated with this command tree's metadata workspace, or /// is not a type in the same type hierarchy as the element type of the /// collection result type of . /// /// DbOfTypeExpression requires that /*CQT_PUBLIC_API(*/internal/*)*/ DbOfTypeExpression CreateOfTypeExpression(DbExpression argument, TypeUsage ofType) { return new DbOfTypeExpression(this, DbExpressionKind.OfType, ofType, argument); } ///has a collection result type with /// a polymorphic element type, and that is a type from the same type hierarchy as that element type. /// /// Creates a new /// Anthat produces a set consisting of the elements of the given input set that are of exactly the specified type. /// that specifies the input set. /// Type metadata for the type that elements of the input set must match exactly to be included in the resulting set. /// A new DbOfTypeExpression with the specified set argument and type, and an ExpressionKind of ///. /// or is null /// ///is associated with a different command tree or does not have a collection result type, /// is not associated with this command tree's metadata workspace, or /// is not a type in the same type hierarchy as the element type of the /// collection result type of . /// /// DbOfTypeExpression requires that /*CQT_PUBLIC_API(*/internal/*)*/ DbOfTypeExpression CreateOfTypeOnlyExpression(DbExpression argument, TypeUsage ofType) { return new DbOfTypeExpression(this, DbExpressionKind.OfTypeOnly, ofType, argument); } ///has a collection result type with /// a polymorphic element type, and that is a type from the same type hierarchy as that element type. /// /// Creates a new /// The Entity set in which the referenced element resides. /// Anthat encodes a reference to a specific Entity based on key values. /// that constructs a record type with columns that match (in number and type) the Key properties of the referenced Entity type. /// A new DbRefExpression that references the element with the specified key values in the given Entity set. ////// or is null /// ///is not associated with this command tree's metadata workspace, /// or is associated with a different command tree or does not have a /// record result type that matches the key properties of the referenced entity set's entity type. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbRefExpression CreateRefExpression(EntitySet entitySet, DbExpression refKeys) { EntityUtil.CheckArgumentNull(entitySet, "entitySet"); return new DbRefExpression(this, entitySet, refKeys, entitySet.ElementType); } ///should be an expression that specifies the key values that identify the referenced entity within the given entity set. /// The result type of should contain a corresponding column for each key property defined by 's entity type. /// /// Creates a new /// The Entity set in which the referenced element resides. /// Anthat encodes a reference to a specific Entity based on key values. /// that constructs a record type with columns that match (in number and type) the Key properties of the referenced Entity type. /// The type of the Entity that the reference should refer to. /// A new DbRefExpression that references the element with the specified key values in the given Entity set. ////// , or is null /// ///or is not associated with this command tree's metadata workspace, /// or is associated with a different command tree; /// is not in the same type hierarchy as the entity set's entity type, or does not have a /// record result type that matches the key properties of the referenced entity set's entity type. /// /// /*CQT_PUBLIC_API(*/internal/*)*/ DbRefExpression CreateRefExpression(EntitySet entitySet, DbExpression refKeys, EntityType entityType) { return new DbRefExpression(this, entitySet, refKeys, entityType); } ///should be an expression that specifies the key values that identify the referenced entity within the given entity set. /// The result type of should contain a corresponding column for each key property defined by 's entity type. /// /// Creates a new /// Anthat retrieves a specific Entity given a reference expression /// that provides the reference. This expression must have a reference Type /// A new DbDerefExpression that retrieves the specified Entity ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbDerefExpression CreateDerefExpression(DbExpression reference) { return new DbDerefExpression(this, reference); } ///is associated with a different command tree, /// or does not have a reference result type. /// /// Creates a new /// The expression that provides the reference. This expression must have a reference Type with an Entity element type. ///that retrieves the key values of the specifed reference in structural form. /// A new DbRefKeyExpression that retrieves the key values of the specified reference. ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbRefKeyExpression CreateRefKeyExpression(DbExpression reference) { return new DbRefKeyExpression(this, reference); } ///is associated with a different command tree, /// or does not have a reference result type. /// /// Creates a new /// The expression that provides the entity. This expression must have an entity result type. ///that retrieves the ref of the specifed entity in structural form. /// A new DbEntityRefExpression that retrieves a reference to the specified entity. ////// is null /// /*CQT_PUBLIC_API(*/internal/*)*/ DbEntityRefExpression CreateEntityRefExpression(DbExpression entity) { return new DbEntityRefExpression(this, entity); } ///is associated with a different command tree, /// or does not have an entity result type. /// /// Creates a new /// An expression that specifies the instance. /// Type metadata for the treat-as type. ///. /// A new DbTreatExpression with the specified argument and type. ////// or is null /// ///is associated with a different command tree, /// is not associated with this command tree's metadata workspace, or /// is not in the same type hierarchy as the result type of . /// /// DbTreatExpression requires that /*CQT_PUBLIC_API(*/internal/*)*/ DbTreatExpression CreateTreatExpression(DbExpression argument, TypeUsage treatType) { return new DbTreatExpression(this, treatType, argument); } #endregion // // Additional expression builders // ... #endregion #endregion #region Internal Implementation ///has a polymorphic result type, /// and that is a type from the same type hierarchy as that result type. /// /// Gets the kind of this command tree. /// internal abstract DbCommandTreeKind CommandTreeKind { get; } ////// Gets the metadata workspace used by this command tree. /// internal MetadataWorkspace MetadataWorkspace { get { return _metadata; } } ////// Gets the data space in which metadata used by this command tree must reside. /// internal DataSpace DataSpace { get { return _dataSpace; } } internal CommandTreeTypeHelper TypeHelper { get { return _typeHelp; } } internal virtual void Validate(System.Data.Common.CommandTrees.Internal.Validator v) { } internal bool ValidationRequired { get { bool valReq = _validatedVersion < _currentVersion; EntityBid.Trace("%d#, %d{bool}\n", this.ObjectId, valReq); return valReq; } } internal void SetValid() { EntityBid.Trace(" %d#\n", this.ObjectId); _validatedVersion = _currentVersion; } internal void SetModified() { int prev = _currentVersion; if (int.MaxValue == _currentVersion) { _currentVersion = 1; _validatedVersion = 0; } else { _currentVersion++; } EntityBid.Trace(" %d# previous version=%d, new version=%d\n", this.ObjectId, prev, _currentVersion); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] // we intend for this to be public someday; called from test code for now. internal void ClearParameters() { EntityBid.Trace(" %d#\n", this.ObjectId); _paramMappings.Clear(); } internal void CopyParametersTo(DbCommandTree tree) { foreach (KeyValuePair paramInfo in _paramMappings.Values) { tree.AddParameter(paramInfo.Key, paramInfo.Value); } } internal bool HasParameter(string parameterName, TypeUsage parameterType) { Debug.Assert(!string.IsNullOrEmpty(parameterName), "Parameter name should not be null or empty"); Debug.Assert(parameterType != null, "Parameter type should not be null"); KeyValuePair foundParam; if(this._paramMappings.TryGetValue(parameterName, out foundParam)) { return (foundParam.Key.Equals(parameterName, StringComparison.Ordinal) && // SQLBUDT#545720: Equivalence is not a sufficient check (consider row types for TVPs) - equality is required. TypeSemantics.IsStructurallyEqualTo(foundParam.Value, parameterType)); } return false; } #region Dump/Print Support internal void Dump(ExpressionDumper dumper) { // // Dump information about this command tree to the specified ExpressionDumper // // First dump standard information - the DataSpace of the command tree and its parameters // Dictionary attrs = new Dictionary (); attrs.Add("DataSpace", this.DataSpace); dumper.Begin(this.GetType().Name, attrs); // // The name and type of each Parameter in turn is added to the output // dumper.Begin("Parameters", null); foreach (KeyValuePair param in this.Parameters) { Dictionary paramAttrs = new Dictionary (); paramAttrs.Add("Name", param.Key); dumper.Begin("Parameter", paramAttrs); dumper.Dump(param.Value, "ParameterType"); dumper.End("Parameter"); } dumper.End("Parameters"); // // Delegate to the derived type's implementation that dumps the structure of the command tree // this.DumpStructure(dumper); // // Matching call to End to correspond with the call to Begin above // dumper.End(this.GetType().Name); } internal abstract void DumpStructure(ExpressionDumper dumper); internal string DumpXml() { // // This is a convenience method that dumps the command tree in an XML format. // This is intended primarily as a debugging aid to allow inspection of the tree structure. // // Create a new MemoryStream that the XML dumper should write to. // MemoryStream stream = new MemoryStream(); // // Create the dumper // XmlExpressionDumper dumper = new XmlExpressionDumper(stream); // // Dump this tree and then close the XML dumper so that the end document tag is written // and the output is flushed to the stream. // this.Dump(dumper); dumper.Close(); // // Construct a string from the resulting memory stream and return it to the caller // return XmlExpressionDumper.DefaultEncoding.GetString(stream.ToArray()); } internal string Print() { return this.PrintTree(new ExpressionPrinter()); } internal abstract string PrintTree(ExpressionPrinter printer); #endregion #region DbExpression Replacement internal void Replace(ReplacerCallback callback) { using (new EntityBid.ScopeAuto(" %d#", this.ObjectId)) { ExpressionReplacer replacer = new ExpressionReplacer(callback); this.Replace(replacer); } } internal abstract void Replace(ExpressionReplacer replacer); #endregion #region Tracing internal void Trace() { if (EntityBid.AdvancedOn) { EntityBid.PutStrChunked(this.DumpXml()); EntityBid.Trace("\n"); } else if (EntityBid.TraceOn) { EntityBid.PutStrChunked(this.Print()); EntityBid.Trace("\n"); } } #endregion #region Container Tracking internal void TrackContainer(EntityContainer container) { using (new EntityBid.ScopeAuto(" %d#", this.ObjectId)) { if (!_containers.Contains(container)) { EntityBid.Trace(" %d#, Adding container to tracked containers list: '%ls'\n", this.ObjectId, container.Name); _containers.Add(container); } } } #endregion #region Internal Helper API internal DbApplyExpression CreateApplyExpressionByKind(DbExpressionKind applyKind, DbExpressionBinding input, DbExpressionBinding apply) { Debug.Assert(DbExpressionKind.CrossApply == applyKind || DbExpressionKind.OuterApply == applyKind, "Invalid ApplyType"); switch(applyKind) { case DbExpressionKind.CrossApply: return this.CreateCrossApplyExpression(input, apply); case DbExpressionKind.OuterApply: return this.CreateOuterApplyExpression(input, apply); default: throw EntityUtil.InvalidEnumerationValue(typeof(DbExpressionKind), (int)applyKind); } } internal DbExpression CreateJoinExpressionByKind(DbExpressionKind joinKind, DbExpression joinCondition, DbExpressionBinding input1, DbExpressionBinding input2) { Debug.Assert(DbExpressionKind.CrossJoin == joinKind || DbExpressionKind.FullOuterJoin == joinKind || DbExpressionKind.InnerJoin == joinKind || DbExpressionKind.LeftOuterJoin == joinKind, "Invalid DbExpressionKind for CreateJoinExpressionByKind"); if (DbExpressionKind.CrossJoin == joinKind) { Debug.Assert(null == joinCondition, "Condition should not be specified for CrossJoin"); return this.CreateCrossJoinExpression(new DbExpressionBinding[2] { input1, input2 }); } else { Debug.Assert(joinCondition != null, "Condition must be specified for non-CrossJoin"); switch (joinKind) { case DbExpressionKind.InnerJoin: return this.CreateInnerJoinExpression(input1, input2, joinCondition); case DbExpressionKind.LeftOuterJoin: return this.CreateLeftOuterJoinExpression(input1, input2, joinCondition); case DbExpressionKind.FullOuterJoin: return this.CreateFullOuterJoinExpression(input1, input2, joinCondition); default: throw EntityUtil.InvalidEnumerationValue(typeof(DbExpressionKind), (int)joinKind); } } } internal static bool IsValidParameterName(string name) { return (!StringUtil.IsNullOrEmptyOrWhiteSpace(name) && _paramNameRegex.IsMatch(name)); } private static readonly Regex _paramNameRegex = new Regex("^([A-Za-z])([A-Za-z0-9_])*$"); #endregion #endregion } /// /// Describes the different "kinds" (classes) of command trees. /// internal enum DbCommandTreeKind { Query, Update, Insert, Delete, Function, } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
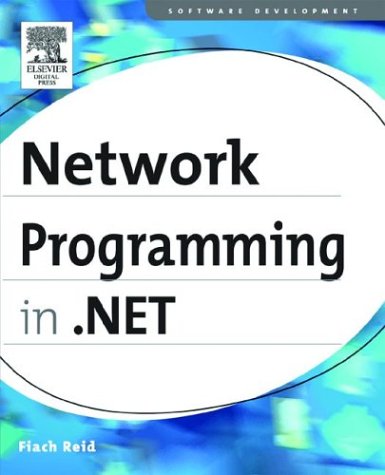
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ArgumentException.cs
- DatePickerTextBox.cs
- SelectionProcessor.cs
- DefaultTypeArgumentAttribute.cs
- OleDbInfoMessageEvent.cs
- WebBaseEventKeyComparer.cs
- PreviewPageInfo.cs
- Walker.cs
- XmlHierarchicalDataSourceView.cs
- CacheMode.cs
- DataServiceEntityAttribute.cs
- GlobalizationSection.cs
- TableLayoutStyle.cs
- AutomationPropertyInfo.cs
- _StreamFramer.cs
- ProxyGenerator.cs
- SelectionUIHandler.cs
- EntityDataSourceStatementEditor.cs
- SQLStringStorage.cs
- ConstrainedDataObject.cs
- GlyphsSerializer.cs
- ReceiveActivity.cs
- PnrpPeerResolverElement.cs
- WebPermission.cs
- IntSecurity.cs
- TableLayoutColumnStyleCollection.cs
- Helper.cs
- SnapshotChangeTrackingStrategy.cs
- _NestedMultipleAsyncResult.cs
- RtfToXamlLexer.cs
- RNGCryptoServiceProvider.cs
- NameValueCollection.cs
- FrugalMap.cs
- RegexBoyerMoore.cs
- SafeWaitHandle.cs
- WpfKnownTypeInvoker.cs
- XmlReaderSettings.cs
- NamespaceInfo.cs
- Stack.cs
- DrawingVisualDrawingContext.cs
- FormattedText.cs
- EllipticalNodeOperations.cs
- TextTreeObjectNode.cs
- WeakReferenceKey.cs
- DispatcherHookEventArgs.cs
- NameValueCollection.cs
- ModulesEntry.cs
- XmlExceptionHelper.cs
- DataGridViewComponentPropertyGridSite.cs
- DefaultTextStore.cs
- CopyOfAction.cs
- DrawingBrush.cs
- ClassicBorderDecorator.cs
- XhtmlBasicListAdapter.cs
- StickyNoteHelper.cs
- securitymgrsite.cs
- TextServicesContext.cs
- EncoderParameter.cs
- UserControl.cs
- VersionUtil.cs
- EntityStoreSchemaGenerator.cs
- BitmapCodecInfo.cs
- DBCommand.cs
- StreamUpdate.cs
- SafeFindHandle.cs
- SymmetricSecurityProtocol.cs
- SID.cs
- AttributedMetaModel.cs
- JavaScriptObjectDeserializer.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- DataGridLinkButton.cs
- PictureBox.cs
- ComponentChangingEvent.cs
- SerTrace.cs
- Enum.cs
- PrimaryKeyTypeConverter.cs
- TypeGeneratedEventArgs.cs
- HtmlElementEventArgs.cs
- DecimalConverter.cs
- UserInitiatedNavigationPermission.cs
- Error.cs
- DataGridCellAutomationPeer.cs
- EventSetter.cs
- SimpleWorkerRequest.cs
- KeyFrames.cs
- ExpressionNormalizer.cs
- PersonalizableAttribute.cs
- Rect3D.cs
- HybridDictionary.cs
- TrackingAnnotationCollection.cs
- FieldAccessException.cs
- PageCodeDomTreeGenerator.cs
- ResourceDisplayNameAttribute.cs
- TextEndOfLine.cs
- MergePropertyDescriptor.cs
- TextBoxView.cs
- VirtualizedItemPattern.cs
- DataIdProcessor.cs
- FormattedText.cs
- XmlILOptimizerVisitor.cs