Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / ChannelBuilder.cs / 1 / ChannelBuilder.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Collections.Generic; using System.ServiceModel.Dispatcher; class ChannelBuilder { CustomBinding binding; BindingContext context; BindingParameterCollection bindingParameters; Uri listenUri; public ChannelBuilder(BindingContext context, bool addChannelDemuxerIfRequired) { this.context = context; if (addChannelDemuxerIfRequired) { this.AddDemuxerBindingElement(context.RemainingBindingElements); } this.binding = new CustomBinding(context.Binding, context.RemainingBindingElements); this.bindingParameters = context.BindingParameters; } public ChannelBuilder(Binding binding, BindingParameterCollection bindingParameters, bool addChannelDemuxerIfRequired) { this.binding = new CustomBinding(binding); this.bindingParameters = bindingParameters; if (addChannelDemuxerIfRequired) { this.AddDemuxerBindingElement(this.binding.Elements); } } public ChannelBuilder(ChannelBuilder channelBuilder) { this.binding = new CustomBinding(channelBuilder.Binding); this.bindingParameters = channelBuilder.BindingParameters; if (this.binding.Elements.Find() == null) { DiagnosticUtility.DebugAssert("ChannelBuilder.ctor (this.binding.Elements.Find () != null)"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } } public CustomBinding Binding { get { return this.binding; } set { this.binding = value; } } public BindingParameterCollection BindingParameters { get { return this.bindingParameters; } set { this.bindingParameters = value; } } void AddDemuxerBindingElement(BindingElementCollection elements) { if (elements.Find () == null) { // add the channel demuxer binding element right above the transport TransportBindingElement transport = elements.Find (); if (transport == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.TransportBindingElementNotFound))); } // cache the context state in the demuxer so that the same context state can be provided to the transport // when building auxilliary channels and listeners (for ex, for security negotiation) elements.Insert(elements.IndexOf(transport), new ChannelDemuxerBindingElement(true)); } } public IChannelFactory BuildChannelFactory () { if (this.context != null) { IChannelFactory factory = this.context.BuildInnerChannelFactory (); this.context = null; return factory; } else { return this.binding.BuildChannelFactory (this.bindingParameters); } } public IChannelListener BuildChannelListener () where TChannel : class, IChannel { if (this.context != null) { IChannelListener listener = this.context.BuildInnerChannelListener (); this.listenUri = listener.Uri; this.context = null; return listener; } else { return this.binding.BuildChannelListener (this.listenUri, this.bindingParameters); } } public IChannelListener BuildChannelListener (MessageFilter filter, int priority) where TChannel : class, IChannel { this.bindingParameters.Add(new ChannelDemuxerFilter(filter, priority)); IChannelListener listener = this.BuildChannelListener (); this.bindingParameters.Remove (); return listener; } public bool CanBuildChannelFactory () { return this.binding.CanBuildChannelFactory (this.bindingParameters); } public bool CanBuildChannelListener () where TChannel : class, IChannel { return this.binding.CanBuildChannelListener (this.bindingParameters); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
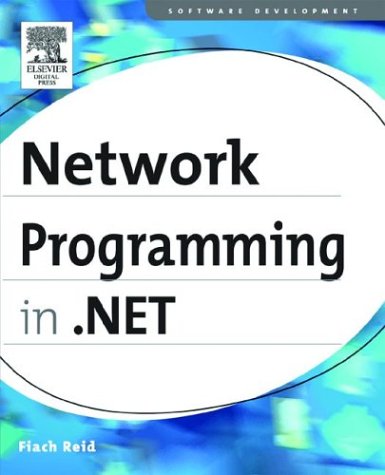
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Activator.cs
- DataSpaceManager.cs
- ControlPager.cs
- FrameworkTemplate.cs
- AttributeConverter.cs
- _FixedSizeReader.cs
- Mappings.cs
- AppendHelper.cs
- CounterSetInstance.cs
- BinaryObjectInfo.cs
- DataObject.cs
- ProbeMatchesCD1.cs
- StaticFileHandler.cs
- UInt32.cs
- HandleRef.cs
- RegexMatchCollection.cs
- XmlComment.cs
- MissingFieldException.cs
- HtmlForm.cs
- AutoCompleteStringCollection.cs
- MonikerProxyAttribute.cs
- ExpressionBuilder.cs
- CodeSubDirectoriesCollection.cs
- AssociationTypeEmitter.cs
- PortCache.cs
- DataSysAttribute.cs
- DynamicPropertyHolder.cs
- ReaderWriterLock.cs
- DataGridPagerStyle.cs
- XmlSerializerOperationBehavior.cs
- SharedPerformanceCounter.cs
- IChannel.cs
- Base64Decoder.cs
- DataStreams.cs
- Annotation.cs
- ScrollEventArgs.cs
- DataGridViewCellStyleConverter.cs
- entitydatasourceentitysetnameconverter.cs
- ServicesExceptionNotHandledEventArgs.cs
- CompositeActivityDesigner.cs
- SinglePhaseEnlistment.cs
- ViewManagerAttribute.cs
- ActivityWithResultConverter.cs
- SnapLine.cs
- DropDownList.cs
- ListViewGroup.cs
- SiteMapDataSourceView.cs
- ListViewItemMouseHoverEvent.cs
- XmlAttributes.cs
- FormatConvertedBitmap.cs
- SystemWebExtensionsSectionGroup.cs
- QuaternionKeyFrameCollection.cs
- CharAnimationUsingKeyFrames.cs
- Metadata.cs
- ErrorRuntimeConfig.cs
- OdbcPermission.cs
- WebHostedComPlusServiceHost.cs
- StrokeNode.cs
- TextServicesPropertyRanges.cs
- DefinitionUpdate.cs
- FileChangesMonitor.cs
- EditingScopeUndoUnit.cs
- _ServiceNameStore.cs
- SymbolType.cs
- SqlDataSourceFilteringEventArgs.cs
- SiteMapHierarchicalDataSourceView.cs
- Object.cs
- ControlValuePropertyAttribute.cs
- BinaryKeyIdentifierClause.cs
- BitmapImage.cs
- ResourceManagerWrapper.cs
- unsafenativemethodsother.cs
- ProviderCommandInfoUtils.cs
- CollectionViewSource.cs
- HttpStreamXmlDictionaryReader.cs
- HandledMouseEvent.cs
- ControlIdConverter.cs
- Parsers.cs
- StaticFileHandler.cs
- ISAPIWorkerRequest.cs
- CapabilitiesRule.cs
- DataGridColumn.cs
- DirectoryNotFoundException.cs
- RepeaterCommandEventArgs.cs
- Vertex.cs
- ScriptRegistrationManager.cs
- MetafileHeader.cs
- TypefaceMetricsCache.cs
- GenericPrincipal.cs
- ContentIterators.cs
- IndependentlyAnimatedPropertyMetadata.cs
- TrustSection.cs
- X509Certificate2.cs
- ResourceExpression.cs
- ObjectDataSourceMethodEditor.cs
- OdbcInfoMessageEvent.cs
- CornerRadiusConverter.cs
- InternalControlCollection.cs
- _CommandStream.cs
- BitVector32.cs