Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / xsp / System / Web / Extensions / ui / ScriptRegistrationManager.cs / 1 / ScriptRegistrationManager.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Globalization; using System.Text; using System.Text.RegularExpressions; using System.Web.UI; using System.Web.Resources; using System.Web.Script.Serialization; internal sealed class ScriptRegistrationManager { private static Regex ScriptTagRegex = new Regex( @"", indexOfEndOfScriptBeginTag, StringComparison.OrdinalIgnoreCase); if (indexOfScriptEndTag == -1) { throw new InvalidOperationException(String.Format(CultureInfo.InvariantCulture, AtlasWeb.ScriptRegistrationManager_NoCloseTag, activeRegistration.Type.FullName, activeRegistration.Key)); } string scriptBlockContents = scriptContent.Substring(indexOfEndOfScriptBeginTag, (indexOfScriptEndTag - indexOfEndOfScriptBeginTag)); // Turn the text content into a text attribute attrs.Add("text", scriptBlockContents); lastIndex = indexOfScriptEndTag + 9; } // Process all the explicit attributes on the script tag CaptureCollection attrnames = match.Groups["attrname"].Captures; CaptureCollection attrvalues = match.Groups["attrval"].Captures; for (int i = 0; i < attrnames.Count; i++) { string attribName = attrnames[i].ToString(); string attribValue = attrvalues[i].ToString(); // DevDev Bugs 123213: script elements registered with RegisterStartupScript are normally rendered // into the html of the page. Any html encoded values in the attributes are interpreted by the // browser, so the actual data is not html encoded. We must HtmlDecode any attribute values we find // here to remain consistent during async posts, since the data will be dynamically injected into // the dom, bypassing the browser's natural html decoding. attribValue = HttpUtility.HtmlDecode(attribValue); attrs.Add(attribName, attribValue); } // Serialize the attributes to JSON and write them out JavaScriptSerializer serializer = new JavaScriptSerializer(); string attrText = serializer.Serialize(attrs); PageRequestManager.EncodeString(writer, token, "ScriptContentWithTags", attrText); } CheckScriptTagTweenSpace(activeRegistration, scriptContent, lastIndex, scriptContent.Length - lastIndex); if (lastIndex == 0) { throw new InvalidOperationException(String.Format(CultureInfo.InvariantCulture, AtlasWeb.ScriptRegistrationManager_NoTags, activeRegistration.Type.FullName, activeRegistration.Key)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Globalization; using System.Text; using System.Text.RegularExpressions; using System.Web.UI; using System.Web.Resources; using System.Web.Script.Serialization; internal sealed class ScriptRegistrationManager { private static Regex ScriptTagRegex = new Regex( @"", indexOfEndOfScriptBeginTag, StringComparison.OrdinalIgnoreCase); if (indexOfScriptEndTag == -1) { throw new InvalidOperationException(String.Format(CultureInfo.InvariantCulture, AtlasWeb.ScriptRegistrationManager_NoCloseTag, activeRegistration.Type.FullName, activeRegistration.Key)); } string scriptBlockContents = scriptContent.Substring(indexOfEndOfScriptBeginTag, (indexOfScriptEndTag - indexOfEndOfScriptBeginTag)); // Turn the text content into a text attribute attrs.Add("text", scriptBlockContents); lastIndex = indexOfScriptEndTag + 9; } // Process all the explicit attributes on the script tag CaptureCollection attrnames = match.Groups["attrname"].Captures; CaptureCollection attrvalues = match.Groups["attrval"].Captures; for (int i = 0; i < attrnames.Count; i++) { string attribName = attrnames[i].ToString(); string attribValue = attrvalues[i].ToString(); // DevDev Bugs 123213: script elements registered with RegisterStartupScript are normally rendered // into the html of the page. Any html encoded values in the attributes are interpreted by the // browser, so the actual data is not html encoded. We must HtmlDecode any attribute values we find // here to remain consistent during async posts, since the data will be dynamically injected into // the dom, bypassing the browser's natural html decoding. attribValue = HttpUtility.HtmlDecode(attribValue); attrs.Add(attribName, attribValue); } // Serialize the attributes to JSON and write them out JavaScriptSerializer serializer = new JavaScriptSerializer(); string attrText = serializer.Serialize(attrs); PageRequestManager.EncodeString(writer, token, "ScriptContentWithTags", attrText); } CheckScriptTagTweenSpace(activeRegistration, scriptContent, lastIndex, scriptContent.Length - lastIndex); if (lastIndex == 0) { throw new InvalidOperationException(String.Format(CultureInfo.InvariantCulture, AtlasWeb.ScriptRegistrationManager_NoTags, activeRegistration.Type.FullName, activeRegistration.Key)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
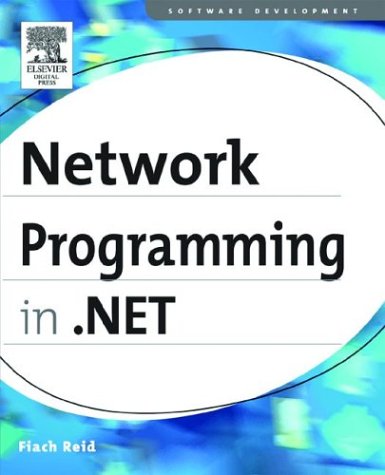
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RectAnimation.cs
- InputScopeConverter.cs
- Nodes.cs
- IPAddressCollection.cs
- TextWriterTraceListener.cs
- TextTreeTextElementNode.cs
- ConfigUtil.cs
- RegionInfo.cs
- Paragraph.cs
- OverflowException.cs
- Stackframe.cs
- FormatSelectingMessageInspector.cs
- PasswordDeriveBytes.cs
- AsymmetricCryptoHandle.cs
- VirtualizingPanel.cs
- Tool.cs
- HttpStreamMessageEncoderFactory.cs
- DataObjectPastingEventArgs.cs
- BindUriHelper.cs
- MailDefinition.cs
- MemoryMappedFileSecurity.cs
- ComplexTypeEmitter.cs
- CapiSafeHandles.cs
- WebPartVerbsEventArgs.cs
- Int64.cs
- MenuCommands.cs
- CompModSwitches.cs
- NonClientArea.cs
- ScheduleChanges.cs
- DrawToolTipEventArgs.cs
- XmlAttributeAttribute.cs
- BindingElementExtensionElement.cs
- StylusShape.cs
- RawStylusActions.cs
- CodeMemberProperty.cs
- RootDesignerSerializerAttribute.cs
- SchemaImporterExtensionElementCollection.cs
- UIPermission.cs
- MatrixTransform3D.cs
- DbParameterCollection.cs
- TreeChangeInfo.cs
- XmlQueryCardinality.cs
- SelfSignedCertificate.cs
- StatusStrip.cs
- CfgParser.cs
- DateTimeValueSerializer.cs
- DefaultValidator.cs
- CounterSampleCalculator.cs
- MissingFieldException.cs
- MemberAccessException.cs
- MLangCodePageEncoding.cs
- HashMembershipCondition.cs
- RuleSettings.cs
- FormsAuthenticationModule.cs
- UIHelper.cs
- _NegoState.cs
- PopOutPanel.cs
- XmlParserContext.cs
- EllipseGeometry.cs
- KeyEventArgs.cs
- PartitionResolver.cs
- SuppressMessageAttribute.cs
- StyleCollection.cs
- Font.cs
- TemplateControlCodeDomTreeGenerator.cs
- NamespaceTable.cs
- TemplateAction.cs
- _UriSyntax.cs
- Memoizer.cs
- BrowserTree.cs
- SoapFormatterSinks.cs
- RouteValueDictionary.cs
- InvokeHandlers.cs
- CollectionChangeEventArgs.cs
- Misc.cs
- EncoderNLS.cs
- ServerValidateEventArgs.cs
- StringCollection.cs
- PageAdapter.cs
- PropertyChangeTracker.cs
- UnsafeNativeMethods.cs
- SqlRemoveConstantOrderBy.cs
- ToolbarAUtomationPeer.cs
- ArgIterator.cs
- JournalNavigationScope.cs
- FixedSOMTable.cs
- ListControl.cs
- MsmqTransportSecurityElement.cs
- FunctionNode.cs
- TrackingExtract.cs
- SmiGettersStream.cs
- LeaseManager.cs
- CornerRadius.cs
- StructuredTypeEmitter.cs
- WebPartManagerInternals.cs
- BooleanExpr.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- Thread.cs
- HtmlInputPassword.cs
- ReflectionHelper.cs