Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / DrawToolTipEventArgs.cs / 1305376 / DrawToolTipEventArgs.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Runtime.InteropServices; using System.ComponentModel; using System.Windows.Forms.VisualStyles; using System.Drawing; using System.Windows.Forms.Internal; using Microsoft.Win32; ////// /// This class contains the information a user needs to paint the ToolTip. /// public class DrawToolTipEventArgs : EventArgs { private readonly Graphics graphics; private readonly IWin32Window associatedWindow; private readonly Control associatedControl; private readonly Rectangle bounds; private readonly string toolTipText; private readonly Color backColor; private readonly Color foreColor; private readonly Font font; ////// /// Creates a new DrawToolTipEventArgs with the given parameters. /// public DrawToolTipEventArgs(Graphics graphics, IWin32Window associatedWindow, Control associatedControl, Rectangle bounds, string toolTipText, Color backColor, Color foreColor, Font font) { this.graphics = graphics; this.associatedWindow = associatedWindow; this.associatedControl = associatedControl; this.bounds = bounds; this.toolTipText = toolTipText; this.backColor = backColor; this.foreColor = foreColor; this.font = font; } ////// /// Graphics object with which painting should be done. /// public Graphics Graphics { get { return graphics; } } ////// /// The window for which the tooltip is being painted. /// public IWin32Window AssociatedWindow { get { return associatedWindow; } } ////// /// The control for which the tooltip is being painted. /// public Control AssociatedControl { get { return associatedControl; } } ////// /// The rectangle outlining the area in which the painting should be done. /// public Rectangle Bounds { get { return bounds; } } ////// /// The text that should be drawn. /// public string ToolTipText { get { return toolTipText; } } ////// /// The font used to draw tooltip text. /// public Font Font { get { return font; } } ////// /// Draws the background of the ToolTip. /// public void DrawBackground() { Brush backBrush = new SolidBrush(backColor); Graphics.FillRectangle(backBrush, bounds); backBrush.Dispose(); } ////// /// Draws the text (overloaded) /// public void DrawText() { //Pass in a set of flags to mimic default behavior DrawText(TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine | TextFormatFlags.HidePrefix); } ////// /// Draws the text (overloaded) - takes a TextFormatFlags argument. /// public void DrawText(TextFormatFlags flags) { TextRenderer.DrawText(graphics, toolTipText, font, bounds, foreColor, flags); } ////// /// Draws a border for the ToolTip similar to the default border. /// public void DrawBorder() { ControlPaint.DrawBorder(graphics, bounds, SystemColors.WindowFrame, ButtonBorderStyle.Solid); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Runtime.InteropServices; using System.ComponentModel; using System.Windows.Forms.VisualStyles; using System.Drawing; using System.Windows.Forms.Internal; using Microsoft.Win32; ////// /// This class contains the information a user needs to paint the ToolTip. /// public class DrawToolTipEventArgs : EventArgs { private readonly Graphics graphics; private readonly IWin32Window associatedWindow; private readonly Control associatedControl; private readonly Rectangle bounds; private readonly string toolTipText; private readonly Color backColor; private readonly Color foreColor; private readonly Font font; ////// /// Creates a new DrawToolTipEventArgs with the given parameters. /// public DrawToolTipEventArgs(Graphics graphics, IWin32Window associatedWindow, Control associatedControl, Rectangle bounds, string toolTipText, Color backColor, Color foreColor, Font font) { this.graphics = graphics; this.associatedWindow = associatedWindow; this.associatedControl = associatedControl; this.bounds = bounds; this.toolTipText = toolTipText; this.backColor = backColor; this.foreColor = foreColor; this.font = font; } ////// /// Graphics object with which painting should be done. /// public Graphics Graphics { get { return graphics; } } ////// /// The window for which the tooltip is being painted. /// public IWin32Window AssociatedWindow { get { return associatedWindow; } } ////// /// The control for which the tooltip is being painted. /// public Control AssociatedControl { get { return associatedControl; } } ////// /// The rectangle outlining the area in which the painting should be done. /// public Rectangle Bounds { get { return bounds; } } ////// /// The text that should be drawn. /// public string ToolTipText { get { return toolTipText; } } ////// /// The font used to draw tooltip text. /// public Font Font { get { return font; } } ////// /// Draws the background of the ToolTip. /// public void DrawBackground() { Brush backBrush = new SolidBrush(backColor); Graphics.FillRectangle(backBrush, bounds); backBrush.Dispose(); } ////// /// Draws the text (overloaded) /// public void DrawText() { //Pass in a set of flags to mimic default behavior DrawText(TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine | TextFormatFlags.HidePrefix); } ////// /// Draws the text (overloaded) - takes a TextFormatFlags argument. /// public void DrawText(TextFormatFlags flags) { TextRenderer.DrawText(graphics, toolTipText, font, bounds, foreColor, flags); } ////// /// Draws a border for the ToolTip similar to the default border. /// public void DrawBorder() { ControlPaint.DrawBorder(graphics, bounds, SystemColors.WindowFrame, ButtonBorderStyle.Solid); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
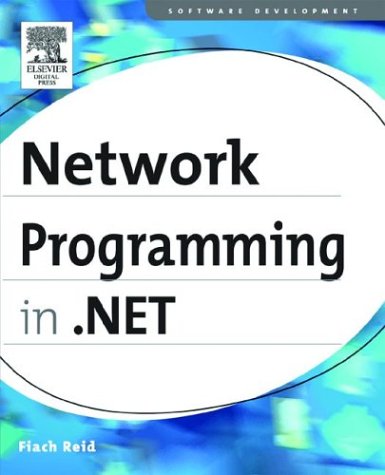
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CacheMode.cs
- VBIdentifierDesigner.xaml.cs
- VisemeEventArgs.cs
- Base64WriteStateInfo.cs
- PointCollection.cs
- CollectionViewGroup.cs
- StringStorage.cs
- ActiveXContainer.cs
- URI.cs
- OutputCacheModule.cs
- QuaternionConverter.cs
- SignedInfo.cs
- InputMethod.cs
- SqlDataAdapter.cs
- TextReturnReader.cs
- BinaryEditor.cs
- PeerInvitationResponse.cs
- XmlResolver.cs
- DBConcurrencyException.cs
- CodeAttachEventStatement.cs
- XmlDictionaryReaderQuotas.cs
- FragmentNavigationEventArgs.cs
- HtmlProps.cs
- ObjectTokenCategory.cs
- ListBase.cs
- GB18030Encoding.cs
- InfoCardPolicy.cs
- EventDescriptor.cs
- Enum.cs
- TextServicesLoader.cs
- InternalConfigConfigurationFactory.cs
- HwndKeyboardInputProvider.cs
- EventManager.cs
- ScriptingJsonSerializationSection.cs
- DefaultBinder.cs
- CookieParameter.cs
- BindingMemberInfo.cs
- BitmapEffectRenderDataResource.cs
- DependentList.cs
- RootBrowserWindowAutomationPeer.cs
- ConsoleKeyInfo.cs
- OleDbCommandBuilder.cs
- TagNameToTypeMapper.cs
- MatrixCamera.cs
- Group.cs
- DrawingAttributeSerializer.cs
- XmlMembersMapping.cs
- GridToolTip.cs
- WindowsEditBox.cs
- PEFileEvidenceFactory.cs
- WindowsSpinner.cs
- SerializationInfo.cs
- Quaternion.cs
- UpdateCompiler.cs
- ApplicationTrust.cs
- COM2ExtendedBrowsingHandler.cs
- activationcontext.cs
- ToolStripDropDownMenu.cs
- XmlAnyElementAttribute.cs
- AppliedDeviceFiltersDialog.cs
- PtsContext.cs
- QuaternionRotation3D.cs
- TreeNodeStyle.cs
- CssClassPropertyAttribute.cs
- AnnotationAdorner.cs
- ProgressBarBrushConverter.cs
- RadioButtonBaseAdapter.cs
- RequestQueryParser.cs
- ContainerVisual.cs
- GridItem.cs
- VectorKeyFrameCollection.cs
- CapabilitiesPattern.cs
- CompoundFileStreamReference.cs
- TreeIterator.cs
- DCSafeHandle.cs
- SqlBulkCopy.cs
- BitmapInitialize.cs
- ToolBar.cs
- LinqDataSourceHelper.cs
- WsdlBuildProvider.cs
- WebConvert.cs
- PathData.cs
- OleDbException.cs
- PageSetupDialog.cs
- Events.cs
- SchemaImporterExtensionElementCollection.cs
- ControlBuilder.cs
- TextBoxAutoCompleteSourceConverter.cs
- SetIndexBinder.cs
- DoubleLinkListEnumerator.cs
- RijndaelManagedTransform.cs
- AdCreatedEventArgs.cs
- WebPartDescriptionCollection.cs
- ExpressionBindings.cs
- GeneralTransform3DGroup.cs
- DirectoryObjectSecurity.cs
- ReverseQueryOperator.cs
- SqlCommandBuilder.cs
- OdbcTransaction.cs
- GridEntryCollection.cs