Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Diagnostics / Eventing / EventDescriptor.cs / 1305376 / EventDescriptor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; namespace System.Diagnostics.Eventing { [StructLayout(LayoutKind.Explicit, Size = 16)] [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] [System.Runtime.CompilerServices.FriendAccessAllowed] internal struct EventDescriptorInternal { # region private [FieldOffset(0)] private ushort m_id; [FieldOffset(2)] private byte m_version; [FieldOffset(3)] private byte m_channel; [FieldOffset(4)] private byte m_level; [FieldOffset(5)] private byte m_opcode; [FieldOffset(6)] private ushort m_task; [FieldOffset(8)] private long m_keywords; #endregion public EventDescriptorInternal( int id, byte version, byte channel, byte level, byte opcode, int task, long keywords ) { if (id < 0) { throw new ArgumentOutOfRangeException("id", SRETW.GetString(SRETW.ArgumentOutOfRange_NeedNonNegNum)); } if (id > ushort.MaxValue) { throw new ArgumentOutOfRangeException("id", SRETW.GetString(SRETW.ArgumentOutOfRange_NeedValidId, 1, ushort.MaxValue)); } m_id = (ushort)id; m_version = version; m_channel = channel; m_level = level; m_opcode = opcode; m_keywords = keywords; if (task < 0) { throw new ArgumentOutOfRangeException("task", SRETW.GetString(SRETW.ArgumentOutOfRange_NeedNonNegNum)); } if (task > ushort.MaxValue) { throw new ArgumentOutOfRangeException("task", SRETW.GetString(SRETW.ArgumentOutOfRange_NeedValidId, 1, ushort.MaxValue)); } m_task = (ushort)task; } public int EventId { get { return m_id; } } public byte Version { get { return m_version; } } public byte Channel { get { return m_channel; } } public byte Level { get { return m_level; } } public byte Opcode { get { return m_opcode; } } public int Task { get { return m_task; } } public long Keywords { get { return m_keywords; } } public override bool Equals(object obj) { if (!(obj is EventDescriptorInternal)) return false; return Equals((EventDescriptorInternal) obj); } public override int GetHashCode() { return m_id ^ m_version ^ m_channel ^ m_level ^ m_opcode ^ m_task ^ (int)m_keywords; } public bool Equals(EventDescriptorInternal other) { if ((m_id != other.m_id) || (m_version != other.m_version) || (m_channel != other.m_channel) || (m_level != other.m_level) || (m_opcode != other.m_opcode) || (m_task != other.m_task) || (m_keywords != other.m_keywords)) { return false; } return true; } public static bool operator ==(EventDescriptorInternal event1, EventDescriptorInternal event2) { return event1.Equals(event2); } public static bool operator !=(EventDescriptorInternal event1, EventDescriptorInternal event2) { return !event1.Equals(event2); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
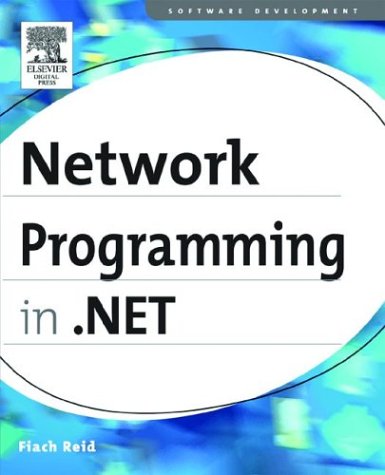
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DescendentsWalkerBase.cs
- ActivityExecutionWorkItem.cs
- DataGridViewSelectedRowCollection.cs
- BaseCollection.cs
- PassportAuthentication.cs
- FusionWrap.cs
- WebPageTraceListener.cs
- CompareValidator.cs
- AuthenticationServiceManager.cs
- InkPresenter.cs
- HttpListenerException.cs
- CharacterShapingProperties.cs
- SemanticAnalyzer.cs
- PerfService.cs
- FontStretch.cs
- FullTextState.cs
- FrameSecurityDescriptor.cs
- RSAPKCS1SignatureFormatter.cs
- Int32Rect.cs
- IIS7WorkerRequest.cs
- AsyncDataRequest.cs
- ErrorFormatter.cs
- MailDefinition.cs
- DateTimeStorage.cs
- DbCommandDefinition.cs
- DBProviderConfigurationHandler.cs
- NumberAction.cs
- ListBindingConverter.cs
- BaseCAMarshaler.cs
- Matrix3DConverter.cs
- XmlPreloadedResolver.cs
- PrimitiveXmlSerializers.cs
- ScrollProviderWrapper.cs
- StateManager.cs
- DLinqColumnProvider.cs
- InstanceKey.cs
- GenericsNotImplementedException.cs
- XmlObjectSerializerWriteContextComplex.cs
- StringDictionary.cs
- EdmTypeAttribute.cs
- DataTable.cs
- ModuleBuilder.cs
- PopupControlService.cs
- StickyNote.cs
- OrderedDictionaryStateHelper.cs
- XmlSchemaAttributeGroupRef.cs
- NameValueConfigurationElement.cs
- WebControlsSection.cs
- CodeDirectionExpression.cs
- StrongName.cs
- ServiceHandle.cs
- externdll.cs
- MLangCodePageEncoding.cs
- AssemblyNameEqualityComparer.cs
- TextSpan.cs
- NavigatingCancelEventArgs.cs
- SelectedDatesCollection.cs
- SystemMulticastIPAddressInformation.cs
- BamlLocalizer.cs
- DataGridToolTip.cs
- ActiveDocumentEvent.cs
- IPAddressCollection.cs
- FontSizeConverter.cs
- PointHitTestParameters.cs
- MD5HashHelper.cs
- ToolStripPanelRow.cs
- EncryptedData.cs
- ThreadPoolTaskScheduler.cs
- ExceptionHelpers.cs
- WebBrowserProgressChangedEventHandler.cs
- InitializerFacet.cs
- WorkflowEventArgs.cs
- Translator.cs
- MsmqChannelFactoryBase.cs
- DataGridViewTextBoxCell.cs
- ColumnResizeUndoUnit.cs
- Itemizer.cs
- RegexWriter.cs
- ElementFactory.cs
- CalendarButton.cs
- ReadOnlyTernaryTree.cs
- SettingsSection.cs
- ArrayElementGridEntry.cs
- LineBreakRecord.cs
- ViewLoader.cs
- Misc.cs
- APCustomTypeDescriptor.cs
- PassportAuthentication.cs
- contentDescriptor.cs
- Attributes.cs
- DataObjectPastingEventArgs.cs
- BitmapEffectDrawingContextState.cs
- SQLByteStorage.cs
- RectAnimationUsingKeyFrames.cs
- ArgIterator.cs
- Section.cs
- BaseInfoTable.cs
- AssemblyBuilder.cs
- IfAction.cs
- InnerItemCollectionView.cs