Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / EventManager.cs / 1 / EventManager.cs
using System; using System.Diagnostics; using MS.Internal.PresentationCore; using MS.Utility; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// Allows management of RoutedEvents /// ///public static class EventManager { #region External API /// /// Registers a ////// with the given parameters /// /// /// ///must be /// unique within the /// (super class types not considered when talking about /// uniqueness) and cannot be null /// must be a /// type of delegate and cannot be null /// must be any /// object type and cannot be null /// /// /// NOTE: Caller must be the static constructor of the /// - /// enforced by stack walk /// /// /// /// /// /// /// /// /// /// /// /// /// The new registered ////// public static RoutedEvent RegisterRoutedEvent( string name, RoutingStrategy routingStrategy, Type handlerType, Type ownerType) { if (name == null) { throw new ArgumentNullException("name"); } if (routingStrategy != RoutingStrategy.Tunnel && routingStrategy != RoutingStrategy.Bubble && routingStrategy != RoutingStrategy.Direct) { throw new System.ComponentModel.InvalidEnumArgumentException("routingStrategy", (int)routingStrategy, typeof(RoutingStrategy)); } if (handlerType == null) { throw new ArgumentNullException("handlerType"); } if (ownerType == null) { throw new ArgumentNullException("ownerType"); } if (GlobalEventManager.GetRoutedEventFromName(name, ownerType, false) != null) { throw new ArgumentException(SR.Get(SRID.DuplicateEventName, name, ownerType)); } return GlobalEventManager.RegisterRoutedEvent(name, routingStrategy, handlerType, ownerType); } /// /// See overloaded method for details /// ////// handledEventsToo defaults to false /// See overloaded method for details /// /// /// /// ///public static void RegisterClassHandler( Type classType, RoutedEvent routedEvent, Delegate handler) { // HandledEventToo defaults to false // Call forwarded RegisterClassHandler(classType, routedEvent, handler, false); } /// /// Add a routed event handler to all instances of a /// particular type inclusive of its sub-class types /// ////// The handlers added thus are also known as /// an class handlers /// /// Target object type on which the handler will be invoked /// when the RoutedEvent is raised /// /// ////// /// /// Class handlers are invoked before the /// instance handlers. Also see /// /// Sub-class class handlers are invoked before /// the super-class class handlers /// /// /// Input parameters classType, /// and handler cannot be null /// handledEventsToo input parameter when false means /// that listener does not care about already handled events. /// Hence the handler will not be invoked on the target if /// the RoutedEvent has already been /// /// handledEventsToo input parameter when true means /// that the listener wants to hear about all events even if /// they have already been handled. Hence the handler will /// be invoked irrespective of the event being /// /// for which the handler /// is attached /// /// /// The handler that will be invoked on the target object /// when the RoutedEvent is raised /// /// /// Flag indicating whether or not the listener wants to /// hear about events that have already been handled /// /// public static void RegisterClassHandler( Type classType, RoutedEvent routedEvent, Delegate handler, bool handledEventsToo) { if (classType == null) { throw new ArgumentNullException("classType"); } if (routedEvent == null) { throw new ArgumentNullException("routedEvent"); } if (handler == null) { throw new ArgumentNullException("handler"); } if (!typeof(UIElement).IsAssignableFrom(classType) && !typeof(ContentElement).IsAssignableFrom(classType) && !typeof(UIElement3D).IsAssignableFrom(classType)) { throw new ArgumentException(SR.Get(SRID.ClassTypeIllegal)); } if (!routedEvent.IsLegalHandler(handler)) { throw new ArgumentException(SR.Get(SRID.HandlerTypeIllegal)); } GlobalEventManager.RegisterClassHandler(classType, routedEvent, handler, handledEventsToo); } /// /// Returns ///s /// that have been registered so far /// /// Also see /// ////// /// /// /// NOTE: There may be more /// s registered later /// /// The ///s /// that have been registered so far /// public static RoutedEvent[] GetRoutedEvents() { return GlobalEventManager.GetRoutedEvents(); } /// /// Finds ///s for the /// given /// /// More specifically finds /// /// ///s starting /// on the /// and looking at its super class types /// /// /// If no matches are found, this method returns null /// to start /// search with and follow through to super class types /// /// /// Matching ///s /// public static RoutedEvent[] GetRoutedEventsForOwner(Type ownerType) { if (ownerType == null) { throw new ArgumentNullException("ownerType"); } return GlobalEventManager.GetRoutedEventsForOwner(ownerType); } /// /// Finds a ///with a /// matching /// and /// /// More specifically finds a /// /// ///with a matching /// starting /// on the /// and looking at its super class types /// /// /// If no matches are found, this method returns null /// to be matched /// /// /// to start /// search with and follow through to super class types /// /// /// Matching [FriendAccessAllowed] internal static RoutedEvent GetRoutedEventFromName(string name, Type ownerType) { if (name == null) { throw new ArgumentNullException("name"); } if (ownerType == null) { throw new ArgumentNullException("ownerType"); } return GlobalEventManager.GetRoutedEventFromName(name, ownerType, true); } #endregion ExternalAPI } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Diagnostics; using MS.Internal.PresentationCore; using MS.Utility; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// /// Allows management of RoutedEvents /// ///public static class EventManager { #region External API /// /// Registers a ////// with the given parameters /// /// /// ///must be /// unique within the /// (super class types not considered when talking about /// uniqueness) and cannot be null /// must be a /// type of delegate and cannot be null /// must be any /// object type and cannot be null /// /// /// NOTE: Caller must be the static constructor of the /// - /// enforced by stack walk /// /// /// /// /// /// /// /// /// /// /// /// /// The new registered ////// public static RoutedEvent RegisterRoutedEvent( string name, RoutingStrategy routingStrategy, Type handlerType, Type ownerType) { if (name == null) { throw new ArgumentNullException("name"); } if (routingStrategy != RoutingStrategy.Tunnel && routingStrategy != RoutingStrategy.Bubble && routingStrategy != RoutingStrategy.Direct) { throw new System.ComponentModel.InvalidEnumArgumentException("routingStrategy", (int)routingStrategy, typeof(RoutingStrategy)); } if (handlerType == null) { throw new ArgumentNullException("handlerType"); } if (ownerType == null) { throw new ArgumentNullException("ownerType"); } if (GlobalEventManager.GetRoutedEventFromName(name, ownerType, false) != null) { throw new ArgumentException(SR.Get(SRID.DuplicateEventName, name, ownerType)); } return GlobalEventManager.RegisterRoutedEvent(name, routingStrategy, handlerType, ownerType); } /// /// See overloaded method for details /// ////// handledEventsToo defaults to false /// See overloaded method for details /// /// /// /// ///public static void RegisterClassHandler( Type classType, RoutedEvent routedEvent, Delegate handler) { // HandledEventToo defaults to false // Call forwarded RegisterClassHandler(classType, routedEvent, handler, false); } /// /// Add a routed event handler to all instances of a /// particular type inclusive of its sub-class types /// ////// The handlers added thus are also known as /// an class handlers /// /// Target object type on which the handler will be invoked /// when the RoutedEvent is raised /// /// ////// /// /// Class handlers are invoked before the /// instance handlers. Also see /// /// Sub-class class handlers are invoked before /// the super-class class handlers /// /// /// Input parameters classType, /// and handler cannot be null /// handledEventsToo input parameter when false means /// that listener does not care about already handled events. /// Hence the handler will not be invoked on the target if /// the RoutedEvent has already been /// /// handledEventsToo input parameter when true means /// that the listener wants to hear about all events even if /// they have already been handled. Hence the handler will /// be invoked irrespective of the event being /// /// for which the handler /// is attached /// /// /// The handler that will be invoked on the target object /// when the RoutedEvent is raised /// /// /// Flag indicating whether or not the listener wants to /// hear about events that have already been handled /// /// public static void RegisterClassHandler( Type classType, RoutedEvent routedEvent, Delegate handler, bool handledEventsToo) { if (classType == null) { throw new ArgumentNullException("classType"); } if (routedEvent == null) { throw new ArgumentNullException("routedEvent"); } if (handler == null) { throw new ArgumentNullException("handler"); } if (!typeof(UIElement).IsAssignableFrom(classType) && !typeof(ContentElement).IsAssignableFrom(classType) && !typeof(UIElement3D).IsAssignableFrom(classType)) { throw new ArgumentException(SR.Get(SRID.ClassTypeIllegal)); } if (!routedEvent.IsLegalHandler(handler)) { throw new ArgumentException(SR.Get(SRID.HandlerTypeIllegal)); } GlobalEventManager.RegisterClassHandler(classType, routedEvent, handler, handledEventsToo); } /// /// Returns ///s /// that have been registered so far /// /// Also see /// ////// /// /// /// NOTE: There may be more /// s registered later /// /// The ///s /// that have been registered so far /// public static RoutedEvent[] GetRoutedEvents() { return GlobalEventManager.GetRoutedEvents(); } /// /// Finds ///s for the /// given /// /// More specifically finds /// /// ///s starting /// on the /// and looking at its super class types /// /// /// If no matches are found, this method returns null /// to start /// search with and follow through to super class types /// /// /// Matching ///s /// public static RoutedEvent[] GetRoutedEventsForOwner(Type ownerType) { if (ownerType == null) { throw new ArgumentNullException("ownerType"); } return GlobalEventManager.GetRoutedEventsForOwner(ownerType); } /// /// Finds a ///with a /// matching /// and /// /// More specifically finds a /// /// ///with a matching /// starting /// on the /// and looking at its super class types /// /// /// If no matches are found, this method returns null /// to be matched /// /// /// to start /// search with and follow through to super class types /// /// /// Matching [FriendAccessAllowed] internal static RoutedEvent GetRoutedEventFromName(string name, Type ownerType) { if (name == null) { throw new ArgumentNullException("name"); } if (ownerType == null) { throw new ArgumentNullException("ownerType"); } return GlobalEventManager.GetRoutedEventFromName(name, ownerType, true); } #endregion ExternalAPI } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
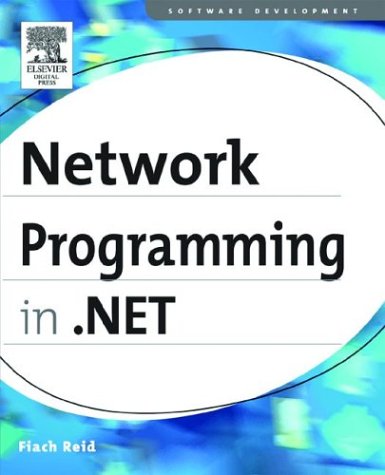
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FormsAuthenticationCredentials.cs
- IconHelper.cs
- SspiSafeHandles.cs
- PagePropertiesChangingEventArgs.cs
- PointHitTestParameters.cs
- METAHEADER.cs
- SelectionChangedEventArgs.cs
- COMException.cs
- HandlerBase.cs
- TextReturnReader.cs
- DllNotFoundException.cs
- AccessorTable.cs
- IfAction.cs
- APCustomTypeDescriptor.cs
- GenericWebPart.cs
- HttpModuleActionCollection.cs
- ToolboxItemWrapper.cs
- TouchPoint.cs
- C14NUtil.cs
- EnlistmentTraceIdentifier.cs
- TableCellCollection.cs
- CheckBoxBaseAdapter.cs
- GroupItem.cs
- ActionMismatchAddressingException.cs
- PolyQuadraticBezierSegment.cs
- DesignerToolboxInfo.cs
- UserInitiatedRoutedEventPermissionAttribute.cs
- dsa.cs
- CatalogPartChrome.cs
- DataGridViewEditingControlShowingEventArgs.cs
- MatchingStyle.cs
- JapaneseCalendar.cs
- WebScriptMetadataFormatter.cs
- PropertyItemInternal.cs
- SqlRecordBuffer.cs
- MethodBuilder.cs
- Cursors.cs
- SrgsSemanticInterpretationTag.cs
- BaseTemplatedMobileComponentEditor.cs
- Evidence.cs
- Journaling.cs
- PieceDirectory.cs
- RuleCache.cs
- CodeMemberProperty.cs
- Vector3D.cs
- ProbeDuplexCD1AsyncResult.cs
- CustomValidator.cs
- HashCodeCombiner.cs
- EncoderParameter.cs
- MailWebEventProvider.cs
- Preprocessor.cs
- HScrollProperties.cs
- Int64Converter.cs
- LineSegment.cs
- _SslStream.cs
- HMAC.cs
- AllMembershipCondition.cs
- ViewStateException.cs
- HtmlElementCollection.cs
- SoapObjectInfo.cs
- ColorContext.cs
- BitSet.cs
- DocumentGridContextMenu.cs
- ToolTip.cs
- MemberDescriptor.cs
- SourceInterpreter.cs
- MarkupExtensionReturnTypeAttribute.cs
- NamespaceTable.cs
- RemotingSurrogateSelector.cs
- CommandEventArgs.cs
- ObjectSerializerFactory.cs
- TransformGroup.cs
- Hash.cs
- EdmRelationshipRoleAttribute.cs
- Soap.cs
- CursorConverter.cs
- DataKey.cs
- InternalBufferOverflowException.cs
- ISAPIApplicationHost.cs
- InProcStateClientManager.cs
- LogEntryHeaderSerializer.cs
- InputLanguageEventArgs.cs
- MultiTargetingUtil.cs
- WebPartCatalogCloseVerb.cs
- SingleAnimationUsingKeyFrames.cs
- SamlAuthenticationClaimResource.cs
- QilTargetType.cs
- SearchForVirtualItemEventArgs.cs
- XmlDownloadManager.cs
- PrimitiveXmlSerializers.cs
- HtmlContainerControl.cs
- BmpBitmapEncoder.cs
- SqlProcedureAttribute.cs
- _PooledStream.cs
- SqlUDTStorage.cs
- UpdatePanelControlTrigger.cs
- AuthenticationSection.cs
- TreeNode.cs
- ValidatedControlConverter.cs
- PropertyGroupDescription.cs