Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / _ServiceNameStore.cs / 1305376 / _ServiceNameStore.cs
using System; using System.Collections.Generic; using System.Security.Authentication.ExtendedProtection; using System.Diagnostics; namespace System.Net { internal class ServiceNameStore { private ListserviceNames; private ServiceNameCollection serviceNameCollection; public ServiceNameCollection ServiceNames { get { if (serviceNameCollection == null) { serviceNameCollection = new ServiceNameCollection(serviceNames); } return serviceNameCollection; } } public ServiceNameStore() { serviceNames = new List (); serviceNameCollection = null; // set only when needed (due to expensive item-by-item copy) } private bool AddSingleServiceName(string spn) { if (Contains(spn)) { return false; } else { serviceNames.Add(spn); return true; } } public bool Add(string uriPrefix) { Debug.Assert(!String.IsNullOrEmpty(uriPrefix)); string[] newServiceNames = BuildServiceNames(uriPrefix); bool addedAny = false; foreach (string spn in newServiceNames) { if (AddSingleServiceName(spn)) { addedAny = true; if (Logging.On) { Logging.PrintInfo(Logging.HttpListener, "ServiceNameStore#" + ValidationHelper.HashString(this) + "::Add() adding default SPNs '" + spn + "' from prefix '" + uriPrefix + "'"); } } } if (addedAny) { serviceNameCollection = null; } else if (Logging.On) { Logging.PrintInfo(Logging.HttpListener, "ServiceNameStore#" + ValidationHelper.HashString(this) + "::Add() no default SPN added for prefix '" + uriPrefix + "'"); } return addedAny; } public bool Remove(string uriPrefix) { Debug.Assert(!String.IsNullOrEmpty(uriPrefix)); string newServiceName = BuildSimpleServiceName(uriPrefix); bool needToRemove = Contains(newServiceName); if (needToRemove) { serviceNames.Remove(newServiceName); serviceNameCollection = null; //invalidate (readonly) ServiceNameCollection } if (Logging.On) { if (needToRemove) { Logging.PrintInfo(Logging.HttpListener, "ServiceNameStore#" + ValidationHelper.HashString(this) + "::Remove() removing default SPN '" + newServiceName + "' from prefix '" + uriPrefix + "'"); } else { Logging.PrintInfo(Logging.HttpListener, "ServiceNameStore#" + ValidationHelper.HashString(this) + "::Remove() no default SPN removed for prefix '" + uriPrefix + "'"); } } return needToRemove; } private bool Contains(string newServiceName) { if (newServiceName == null) { return false; } bool found = false; foreach (string serviceName in serviceNames) { if (String.Compare(serviceName, newServiceName, StringComparison.InvariantCultureIgnoreCase) == 0) { found = true; break; } } return found; } public void Clear() { serviceNames.Clear(); serviceNameCollection = null; //invalidate (readonly) ServiceNameCollection } private string ExtractHostname(string uriPrefix, bool allowInvalidUriStrings) { if (Uri.IsWellFormedUriString(uriPrefix, UriKind.Absolute)) { Uri hostUri = new Uri(uriPrefix); return hostUri.Host; } else if (allowInvalidUriStrings) { int i = uriPrefix.IndexOf("://") + 3; int j = i; bool inSquareBrackets = false; while(j < uriPrefix.Length && uriPrefix[j] != '/' && (uriPrefix[j] != ':' || inSquareBrackets)) { if (uriPrefix[j] == '[') { if (inSquareBrackets) { j = i; break; } inSquareBrackets = true; } if (inSquareBrackets && uriPrefix[j] == ']') { inSquareBrackets = false; } j++; } return uriPrefix.Substring(i, j - i); } return null; } public string BuildSimpleServiceName(string uriPrefix) { string hostname = ExtractHostname(uriPrefix, false); if (hostname != null) { return "HTTP/" + hostname; } else { return null; } } public string[] BuildServiceNames(string uriPrefix) { string hostname = ExtractHostname(uriPrefix, true); IPAddress ipAddress = null; if (String.Compare(hostname, "*", StringComparison.InvariantCultureIgnoreCase) == 0 || String.Compare(hostname, "+", StringComparison.InvariantCultureIgnoreCase) == 0 || IPAddress.TryParse(hostname, out ipAddress)) { // for a wildcard, register the machine name. If the caller doesn't have DNS permission // or the query fails for some reason, don't add an SPN. try { string machineName = Dns.GetHostEntry(String.Empty).HostName; return new string[] { "HTTP/" + machineName }; } catch (System.Net.Sockets.SocketException) { return new string[0]; } catch (System.Security.SecurityException) { return new string[0]; } } else if (!hostname.Contains(".")) { // for a dotless name, try to resolve the FQDN. If the caller doesn't have DNS permission // or the query fails for some reason, add only the dotless name. try { string fqdn = Dns.GetHostEntry(hostname).HostName; return new string[] { "HTTP/" + hostname, "HTTP/" + fqdn }; } catch (System.Net.Sockets.SocketException) { return new string[] { "HTTP/" + hostname }; } catch (System.Security.SecurityException) { return new string[] { "HTTP/" + hostname }; } } else { return new string[] { "HTTP/" + hostname }; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
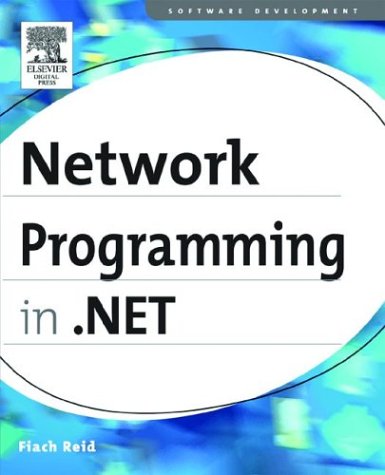
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QuadraticBezierSegment.cs
- LocalizedNameDescriptionPair.cs
- ConnectorEditor.cs
- MeshGeometry3D.cs
- Aes.cs
- Set.cs
- EventSinkHelperWriter.cs
- _Connection.cs
- DataQuery.cs
- MessageQueueConverter.cs
- XmlQualifiedName.cs
- HandlerMappingMemo.cs
- ZipIOExtraFieldPaddingElement.cs
- InheritablePropertyChangeInfo.cs
- PersonalizableTypeEntry.cs
- ActionItem.cs
- EntityDataSourceChangedEventArgs.cs
- FilterableAttribute.cs
- TargetInvocationException.cs
- XDRSchema.cs
- CodeSubDirectory.cs
- GeneralTransform2DTo3D.cs
- WindowsToolbar.cs
- DesignerAdapterAttribute.cs
- InputLanguage.cs
- ReflectPropertyDescriptor.cs
- KeyedHashAlgorithm.cs
- GeometryHitTestResult.cs
- XmlILStorageConverter.cs
- ContactManager.cs
- InvalidComObjectException.cs
- DoubleUtil.cs
- BaseParaClient.cs
- MsmqTransportElement.cs
- BinaryMessageEncoder.cs
- SetterBase.cs
- StorageAssociationSetMapping.cs
- LinkButton.cs
- DistributedTransactionPermission.cs
- TypeDescriptionProviderAttribute.cs
- DataQuery.cs
- EventArgs.cs
- TextElementCollection.cs
- GroupStyle.cs
- EntitySetDataBindingList.cs
- OneOfElement.cs
- ActivationService.cs
- BaseCodePageEncoding.cs
- Select.cs
- PropertySourceInfo.cs
- SqlInternalConnectionSmi.cs
- TextBox.cs
- TextTreeUndoUnit.cs
- PropertyItemInternal.cs
- DatePickerDateValidationErrorEventArgs.cs
- WebPartExportVerb.cs
- ContextDataSource.cs
- WmlControlAdapter.cs
- DictionaryBase.cs
- VectorAnimationBase.cs
- EncryptedData.cs
- Exceptions.cs
- NumberFormatter.cs
- codemethodreferenceexpression.cs
- IFormattable.cs
- GlyphRunDrawing.cs
- VersionPair.cs
- DbModificationClause.cs
- TableColumnCollectionInternal.cs
- AuthenticationSection.cs
- GroupBox.cs
- XmlSchemaIdentityConstraint.cs
- SortQuery.cs
- StreamGeometry.cs
- ProxySimple.cs
- SchemaImporterExtension.cs
- MachineKeySection.cs
- MembershipValidatePasswordEventArgs.cs
- HGlobalSafeHandle.cs
- AlphabetConverter.cs
- CaretElement.cs
- DataGrid.cs
- ToolboxItemCollection.cs
- TypeUsage.cs
- BasicAsyncResult.cs
- ParallelActivityDesigner.cs
- Table.cs
- ServiceNameElement.cs
- TrimSurroundingWhitespaceAttribute.cs
- InputQueueChannelAcceptor.cs
- ReferenceService.cs
- TagMapInfo.cs
- ProgressBar.cs
- ScriptResourceInfo.cs
- TextRangeSerialization.cs
- WrapPanel.cs
- StrokeCollection.cs
- ImageSource.cs
- XmlSchemaRedefine.cs
- Normalization.cs