Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Controls / ProgressBar.cs / 1 / ProgressBar.cs
//---------------------------------------------------------------------------- // File: ProgressBar.cs // // Description: // Implementation of ProgressBar control. // // History: // 06/28/2004 - t-sergin - Created // // Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.Specialized; using System.Threading; using System.Windows.Automation.Peers; using System.Windows.Automation.Provider; using System.Windows; using System.Windows.Controls.Primitives; using System.Windows.Media; using MS.Internal.KnownBoxes; namespace System.Windows.Controls { ////// The ProgressBar class /// ///[TemplatePart(Name = "PART_Track", Type = typeof(FrameworkElement))] [TemplatePart(Name = "PART_Indicator", Type = typeof(FrameworkElement))] public class ProgressBar : RangeBase { #region Constructors static ProgressBar() { FocusableProperty.OverrideMetadata(typeof(ProgressBar), new FrameworkPropertyMetadata(BooleanBoxes.FalseBox)); DefaultStyleKeyProperty.OverrideMetadata(typeof(ProgressBar), new FrameworkPropertyMetadata(typeof(ProgressBar))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(ProgressBar)); // Set default to 100.0 RangeBase.MaximumProperty.OverrideMetadata(typeof(ProgressBar), new FrameworkPropertyMetadata(100.0)); } /// /// Instantiates a new instance of Progressbar without Dispatcher. /// public ProgressBar() : base() { } #endregion Constructors #region Properties ////// The DependencyProperty for the IsIndeterminate property. /// Flags: none /// DefaultValue: false /// public static readonly DependencyProperty IsIndeterminateProperty = DependencyProperty.Register( "IsIndeterminate", typeof(bool), typeof(ProgressBar), new FrameworkPropertyMetadata( false, new PropertyChangedCallback(OnIsIndeterminateChanged))); ////// Determines if ProgressBar shows actual values (false) /// or generic, continuous progress feedback (true). /// ///public bool IsIndeterminate { get { return (bool) GetValue(IsIndeterminateProperty); } set { SetValue(IsIndeterminateProperty, value); } } /// /// Called when IsIndeterminateProperty is changed on "d". /// private static void OnIsIndeterminateChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ProgressBar progressBar = (ProgressBar)d; // Invalidate automation peer ProgressBarAutomationPeer peer = UIElementAutomationPeer.FromElement(progressBar) as ProgressBarAutomationPeer; if (peer != null) { peer.InvalidatePeer(); } progressBar.SetProgressBarIndicatorLength(); } ////// DependencyProperty for public static readonly DependencyProperty OrientationProperty = DependencyProperty.Register( "Orientation", typeof(Orientation), typeof(ProgressBar), new FrameworkPropertyMetadata( Orientation.Horizontal, FrameworkPropertyMetadataOptions.AffectsMeasure), new ValidateValueCallback(IsValidOrientation)); ///property. /// /// Specifies orientation of the ProgressBar. /// public Orientation Orientation { get { return (Orientation) GetValue(OrientationProperty); } set { SetValue(OrientationProperty, value); } } internal static bool IsValidOrientation(object o) { Orientation value = (Orientation)o; return value == Orientation.Horizontal || value == Orientation.Vertical; } #endregion Properties #region Event Handler // Set the width/height of the contract parts private void SetProgressBarIndicatorLength() { if (_track != null && _indicator != null) { double min = Minimum; double max = Maximum; double val = Value; // When indeterminate or maximum == minimum, have the indicator stretch the // whole length of track double percent = IsIndeterminate || max == min ? 1.0 : (val - min) / (max - min); if (Orientation == Orientation.Horizontal) { _indicator.Width = percent * _track.ActualWidth; } else // Oriention == Vertical { _indicator.Height = percent * _track.ActualHeight; } } } #endregion #region Method Overrides ////// Creates AutomationPeer ( protected override AutomationPeer OnCreateAutomationPeer() { return new ProgressBarAutomationPeer(this); } ///) /// /// This method is invoked when the Value property changes. /// ProgressBar updates its style parts when Value changes. /// /// The old value of the Value property. /// The new value of the Value property. protected override void OnValueChanged(double oldValue, double newValue) { base.OnValueChanged(oldValue, newValue); SetProgressBarIndicatorLength(); } ////// Called when the Template's tree has been generated /// public override void OnApplyTemplate() { base.OnApplyTemplate(); if (_track != null) { _track.SizeChanged -= OnTrackSizeChanged; } _track = GetTemplateChild(TrackTemplateName) as FrameworkElement; _indicator = GetTemplateChild(IndicatorTemplateName) as FrameworkElement; if (_track != null) { _track.SizeChanged += OnTrackSizeChanged; } } private void OnTrackSizeChanged(object sender, SizeChangedEventArgs e) { SetProgressBarIndicatorLength(); } #endregion #region Data private const string TrackTemplateName = "PART_Track"; private const string IndicatorTemplateName = "PART_Indicator"; private FrameworkElement _track; private FrameworkElement _indicator; #endregion Data #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // File: ProgressBar.cs // // Description: // Implementation of ProgressBar control. // // History: // 06/28/2004 - t-sergin - Created // // Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.Specialized; using System.Threading; using System.Windows.Automation.Peers; using System.Windows.Automation.Provider; using System.Windows; using System.Windows.Controls.Primitives; using System.Windows.Media; using MS.Internal.KnownBoxes; namespace System.Windows.Controls { ////// The ProgressBar class /// ///[TemplatePart(Name = "PART_Track", Type = typeof(FrameworkElement))] [TemplatePart(Name = "PART_Indicator", Type = typeof(FrameworkElement))] public class ProgressBar : RangeBase { #region Constructors static ProgressBar() { FocusableProperty.OverrideMetadata(typeof(ProgressBar), new FrameworkPropertyMetadata(BooleanBoxes.FalseBox)); DefaultStyleKeyProperty.OverrideMetadata(typeof(ProgressBar), new FrameworkPropertyMetadata(typeof(ProgressBar))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(ProgressBar)); // Set default to 100.0 RangeBase.MaximumProperty.OverrideMetadata(typeof(ProgressBar), new FrameworkPropertyMetadata(100.0)); } /// /// Instantiates a new instance of Progressbar without Dispatcher. /// public ProgressBar() : base() { } #endregion Constructors #region Properties ////// The DependencyProperty for the IsIndeterminate property. /// Flags: none /// DefaultValue: false /// public static readonly DependencyProperty IsIndeterminateProperty = DependencyProperty.Register( "IsIndeterminate", typeof(bool), typeof(ProgressBar), new FrameworkPropertyMetadata( false, new PropertyChangedCallback(OnIsIndeterminateChanged))); ////// Determines if ProgressBar shows actual values (false) /// or generic, continuous progress feedback (true). /// ///public bool IsIndeterminate { get { return (bool) GetValue(IsIndeterminateProperty); } set { SetValue(IsIndeterminateProperty, value); } } /// /// Called when IsIndeterminateProperty is changed on "d". /// private static void OnIsIndeterminateChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ProgressBar progressBar = (ProgressBar)d; // Invalidate automation peer ProgressBarAutomationPeer peer = UIElementAutomationPeer.FromElement(progressBar) as ProgressBarAutomationPeer; if (peer != null) { peer.InvalidatePeer(); } progressBar.SetProgressBarIndicatorLength(); } ////// DependencyProperty for public static readonly DependencyProperty OrientationProperty = DependencyProperty.Register( "Orientation", typeof(Orientation), typeof(ProgressBar), new FrameworkPropertyMetadata( Orientation.Horizontal, FrameworkPropertyMetadataOptions.AffectsMeasure), new ValidateValueCallback(IsValidOrientation)); ///property. /// /// Specifies orientation of the ProgressBar. /// public Orientation Orientation { get { return (Orientation) GetValue(OrientationProperty); } set { SetValue(OrientationProperty, value); } } internal static bool IsValidOrientation(object o) { Orientation value = (Orientation)o; return value == Orientation.Horizontal || value == Orientation.Vertical; } #endregion Properties #region Event Handler // Set the width/height of the contract parts private void SetProgressBarIndicatorLength() { if (_track != null && _indicator != null) { double min = Minimum; double max = Maximum; double val = Value; // When indeterminate or maximum == minimum, have the indicator stretch the // whole length of track double percent = IsIndeterminate || max == min ? 1.0 : (val - min) / (max - min); if (Orientation == Orientation.Horizontal) { _indicator.Width = percent * _track.ActualWidth; } else // Oriention == Vertical { _indicator.Height = percent * _track.ActualHeight; } } } #endregion #region Method Overrides ////// Creates AutomationPeer ( protected override AutomationPeer OnCreateAutomationPeer() { return new ProgressBarAutomationPeer(this); } ///) /// /// This method is invoked when the Value property changes. /// ProgressBar updates its style parts when Value changes. /// /// The old value of the Value property. /// The new value of the Value property. protected override void OnValueChanged(double oldValue, double newValue) { base.OnValueChanged(oldValue, newValue); SetProgressBarIndicatorLength(); } ////// Called when the Template's tree has been generated /// public override void OnApplyTemplate() { base.OnApplyTemplate(); if (_track != null) { _track.SizeChanged -= OnTrackSizeChanged; } _track = GetTemplateChild(TrackTemplateName) as FrameworkElement; _indicator = GetTemplateChild(IndicatorTemplateName) as FrameworkElement; if (_track != null) { _track.SizeChanged += OnTrackSizeChanged; } } private void OnTrackSizeChanged(object sender, SizeChangedEventArgs e) { SetProgressBarIndicatorLength(); } #endregion #region Data private const string TrackTemplateName = "PART_Track"; private const string IndicatorTemplateName = "PART_Indicator"; private FrameworkElement _track; private FrameworkElement _indicator; #endregion Data #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
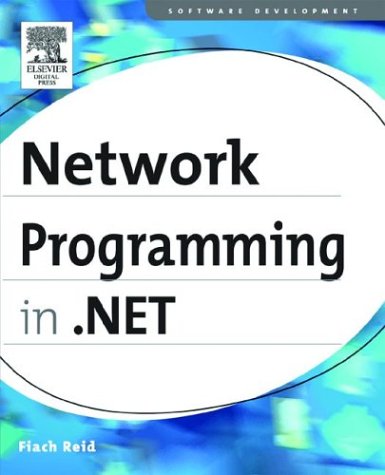
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlHelper.cs
- Visitor.cs
- CodeDomLoader.cs
- PasswordDeriveBytes.cs
- DefaultBinder.cs
- Int32RectConverter.cs
- CacheRequest.cs
- MsmqIntegrationSecurityElement.cs
- ElementUtil.cs
- NotifyIcon.cs
- BehaviorDragDropEventArgs.cs
- Rfc2898DeriveBytes.cs
- DbCommandDefinition.cs
- Substitution.cs
- PathFigureCollection.cs
- EtwProvider.cs
- PrivateFontCollection.cs
- HostingEnvironmentException.cs
- PriorityChain.cs
- SourceChangedEventArgs.cs
- TextEndOfLine.cs
- StylusButton.cs
- CommandLibraryHelper.cs
- SqlConnectionPoolProviderInfo.cs
- ModulesEntry.cs
- SamlSubject.cs
- CodeNamespace.cs
- ProcessManager.cs
- _NativeSSPI.cs
- TypeDescriptionProvider.cs
- CommonGetThemePartSize.cs
- ApplyImportsAction.cs
- Transform3DCollection.cs
- AppSettingsSection.cs
- SpeechAudioFormatInfo.cs
- AuthorizationRule.cs
- RootDesignerSerializerAttribute.cs
- CodeSnippetStatement.cs
- PropertyConverter.cs
- BufferedGraphicsManager.cs
- SqlBulkCopyColumnMappingCollection.cs
- CSharpCodeProvider.cs
- SafeProcessHandle.cs
- Graphics.cs
- GenericTypeParameterBuilder.cs
- HttpCachePolicyElement.cs
- DataGridViewCellCancelEventArgs.cs
- BypassElement.cs
- BamlRecordWriter.cs
- Stacktrace.cs
- WebServiceTypeData.cs
- DtrList.cs
- DependencyStoreSurrogate.cs
- NameScope.cs
- DefaultValueConverter.cs
- ValidationErrorCollection.cs
- webproxy.cs
- CqlErrorHelper.cs
- CacheDependency.cs
- login.cs
- StorageComplexTypeMapping.cs
- ScriptManager.cs
- ColorContextHelper.cs
- MutexSecurity.cs
- VersionedStreamOwner.cs
- LineGeometry.cs
- DataServiceConfiguration.cs
- ThrowHelper.cs
- SubMenuStyleCollection.cs
- ResourceReader.cs
- SqlXml.cs
- UnauthorizedWebPart.cs
- ColorEditor.cs
- EnvironmentPermission.cs
- CryptoConfig.cs
- Sorting.cs
- BuildProviderCollection.cs
- ElementAction.cs
- SqlTypesSchemaImporter.cs
- NonceCache.cs
- ContentType.cs
- safemediahandle.cs
- RequestQueryParser.cs
- GradientBrush.cs
- EntitySqlException.cs
- FloaterBaseParagraph.cs
- KnownTypes.cs
- TransportBindingElementImporter.cs
- MailHeaderInfo.cs
- EdmFunctions.cs
- SerialReceived.cs
- OptimizedTemplateContentHelper.cs
- TableCell.cs
- DefaultDiscoveryServiceExtension.cs
- ListViewDeleteEventArgs.cs
- SEHException.cs
- MultiTrigger.cs
- ThreadStateException.cs
- XmlNotation.cs
- ExpressionNormalizer.cs