Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / LineGeometry.cs / 1305600 / LineGeometry.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: LineGeometry.cs //----------------------------------------------------------------------------- using System; using MS.Internal; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Text; using System.Globalization; using System.Windows.Media; using System.Windows; using System.Text.RegularExpressions; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// This is the Geometry class for Lines. /// public sealed partial class LineGeometry : Geometry { #region Constructors ////// /// public LineGeometry() { } ////// /// public LineGeometry(Point startPoint, Point endPoint) { StartPoint = startPoint; EndPoint = endPoint; } ////// /// public LineGeometry( Point startPoint, Point endPoint, Transform transform) : this(startPoint, endPoint) { Transform = transform; } #endregion ////// Gets the bounds of this Geometry as an axis-aligned bounding box /// public override Rect Bounds { get { ReadPreamble(); Rect rect = new Rect(StartPoint, EndPoint); Transform transform = Transform; if (transform != null && !transform.IsIdentity) { transform.TransformRect(ref rect); } return rect; } } ////// Returns the axis-aligned bounding rectangle when stroked with a pen, after applying /// the supplied transform (if non-null). /// internal override Rect GetBoundsInternal(Pen pen, Matrix worldMatrix, double tolerance, ToleranceType type) { Matrix geometryMatrix; Transform.GetTransformValue(Transform, out geometryMatrix); return LineGeometry.GetBoundsHelper( pen, worldMatrix, StartPoint, EndPoint, geometryMatrix, tolerance, type); } ////// Critical - it calls a critical method, Geometry.GetBoundsHelper and has an unsafe block /// TreatAsSafe - returning a LineGeometry's bounds is considered safe /// [SecurityCritical, SecurityTreatAsSafe] internal static Rect GetBoundsHelper(Pen pen, Matrix worldMatrix, Point pt1, Point pt2, Matrix geometryMatrix, double tolerance, ToleranceType type) { Debug.Assert(worldMatrix != null); Debug.Assert(geometryMatrix != null); if (pen == null && worldMatrix.IsIdentity && geometryMatrix.IsIdentity) { return new Rect(pt1, pt2); } else { unsafe { Point* pPoints = stackalloc Point[2]; pPoints[0] = pt1; pPoints[1] = pt2; fixed (byte *pTypes = LineGeometry.s_lineTypes) { return Geometry.GetBoundsHelper( pen, &worldMatrix, pPoints, pTypes, c_pointCount, c_segmentCount, &geometryMatrix, tolerance, type, false); // skip hollows - meaningless here, this is never a hollow } } } } ////// Critical - contains unsafe block and calls critical method Geometry.ContainsInternal. /// TreatAsSafe - as this doesn't expose anything sensitive. /// [SecurityCritical, SecurityTreatAsSafe] internal override bool ContainsInternal(Pen pen, Point hitPoint, double tolerance, ToleranceType type) { unsafe { Point *pPoints = stackalloc Point[2]; pPoints[0] = StartPoint; pPoints[1] = EndPoint; fixed (byte* pTypes = GetTypeList()) { return ContainsInternal( pen, hitPoint, tolerance, type, pPoints, GetPointCount(), pTypes, GetSegmentCount()); } } } ////// Returns true if this geometry is empty /// public override bool IsEmpty() { return false; } ////// Returns true if this geometry may have curved segments /// public override bool MayHaveCurves() { return false; } ////// Gets the area of this geometry /// /// The computational error tolerance /// The way the error tolerance will be interpreted - relative or absolute public override double GetArea(double tolerance, ToleranceType type) { return 0.0; } private byte[] GetTypeList() { return s_lineTypes; } private static byte[] s_lineTypes = new byte[] { (byte)MILCoreSegFlags.SegTypeLine }; private uint GetPointCount() { return c_pointCount; } private uint GetSegmentCount() { return c_segmentCount; } ////// GetAsPathGeometry - return a PathGeometry version of this Geometry /// internal override PathGeometry GetAsPathGeometry() { PathStreamGeometryContext ctx = new PathStreamGeometryContext(FillRule.EvenOdd, Transform); PathGeometry.ParsePathGeometryData(GetPathGeometryData(), ctx); return ctx.GetPathGeometry(); } internal override PathFigureCollection GetTransformedFigureCollection(Transform transform) { // This is lossy for consistency with other GetPathFigureCollection() implementations // however this limitation doesn't otherwise need to exist for LineGeometry. Point startPoint = StartPoint; Point endPoint = EndPoint; // Apply internal transform Transform internalTransform = Transform; if (internalTransform != null && !internalTransform.IsIdentity) { Matrix matrix = internalTransform.Value; startPoint *= matrix; endPoint *= matrix; } // Apply external transform if (transform != null && !transform.IsIdentity) { Matrix matrix = transform.Value; startPoint *= matrix; endPoint *= matrix; } PathFigureCollection collection = new PathFigureCollection(); collection.Add( new PathFigure( startPoint, new PathSegment[]{new LineSegment(endPoint, true)}, false // ==> not closed ) ); return collection; } ////// GetPathGeometryData - returns a byte[] which contains this Geometry represented /// as a path geometry's serialized format. /// internal override PathGeometryData GetPathGeometryData() { if (IsObviouslyEmpty()) { return Geometry.GetEmptyPathGeometryData(); } PathGeometryData data = new PathGeometryData(); data.FillRule = FillRule.EvenOdd; data.Matrix = CompositionResourceManager.TransformToMilMatrix3x2D(Transform); ByteStreamGeometryContext ctx = new ByteStreamGeometryContext(); ctx.BeginFigure(StartPoint, true /* is filled */, false /* is closed */); ctx.LineTo(EndPoint, true /* is stroked */, false /* is smooth join */); ctx.Close(); data.SerializedData = ctx.GetData(); return data; } #region Static Data private const UInt32 c_segmentCount = 1; private const UInt32 c_pointCount = 2; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: LineGeometry.cs //----------------------------------------------------------------------------- using System; using MS.Internal; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Text; using System.Globalization; using System.Windows.Media; using System.Windows; using System.Text.RegularExpressions; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// This is the Geometry class for Lines. /// public sealed partial class LineGeometry : Geometry { #region Constructors ////// /// public LineGeometry() { } ////// /// public LineGeometry(Point startPoint, Point endPoint) { StartPoint = startPoint; EndPoint = endPoint; } ////// /// public LineGeometry( Point startPoint, Point endPoint, Transform transform) : this(startPoint, endPoint) { Transform = transform; } #endregion ////// Gets the bounds of this Geometry as an axis-aligned bounding box /// public override Rect Bounds { get { ReadPreamble(); Rect rect = new Rect(StartPoint, EndPoint); Transform transform = Transform; if (transform != null && !transform.IsIdentity) { transform.TransformRect(ref rect); } return rect; } } ////// Returns the axis-aligned bounding rectangle when stroked with a pen, after applying /// the supplied transform (if non-null). /// internal override Rect GetBoundsInternal(Pen pen, Matrix worldMatrix, double tolerance, ToleranceType type) { Matrix geometryMatrix; Transform.GetTransformValue(Transform, out geometryMatrix); return LineGeometry.GetBoundsHelper( pen, worldMatrix, StartPoint, EndPoint, geometryMatrix, tolerance, type); } ////// Critical - it calls a critical method, Geometry.GetBoundsHelper and has an unsafe block /// TreatAsSafe - returning a LineGeometry's bounds is considered safe /// [SecurityCritical, SecurityTreatAsSafe] internal static Rect GetBoundsHelper(Pen pen, Matrix worldMatrix, Point pt1, Point pt2, Matrix geometryMatrix, double tolerance, ToleranceType type) { Debug.Assert(worldMatrix != null); Debug.Assert(geometryMatrix != null); if (pen == null && worldMatrix.IsIdentity && geometryMatrix.IsIdentity) { return new Rect(pt1, pt2); } else { unsafe { Point* pPoints = stackalloc Point[2]; pPoints[0] = pt1; pPoints[1] = pt2; fixed (byte *pTypes = LineGeometry.s_lineTypes) { return Geometry.GetBoundsHelper( pen, &worldMatrix, pPoints, pTypes, c_pointCount, c_segmentCount, &geometryMatrix, tolerance, type, false); // skip hollows - meaningless here, this is never a hollow } } } } ////// Critical - contains unsafe block and calls critical method Geometry.ContainsInternal. /// TreatAsSafe - as this doesn't expose anything sensitive. /// [SecurityCritical, SecurityTreatAsSafe] internal override bool ContainsInternal(Pen pen, Point hitPoint, double tolerance, ToleranceType type) { unsafe { Point *pPoints = stackalloc Point[2]; pPoints[0] = StartPoint; pPoints[1] = EndPoint; fixed (byte* pTypes = GetTypeList()) { return ContainsInternal( pen, hitPoint, tolerance, type, pPoints, GetPointCount(), pTypes, GetSegmentCount()); } } } ////// Returns true if this geometry is empty /// public override bool IsEmpty() { return false; } ////// Returns true if this geometry may have curved segments /// public override bool MayHaveCurves() { return false; } ////// Gets the area of this geometry /// /// The computational error tolerance /// The way the error tolerance will be interpreted - relative or absolute public override double GetArea(double tolerance, ToleranceType type) { return 0.0; } private byte[] GetTypeList() { return s_lineTypes; } private static byte[] s_lineTypes = new byte[] { (byte)MILCoreSegFlags.SegTypeLine }; private uint GetPointCount() { return c_pointCount; } private uint GetSegmentCount() { return c_segmentCount; } ////// GetAsPathGeometry - return a PathGeometry version of this Geometry /// internal override PathGeometry GetAsPathGeometry() { PathStreamGeometryContext ctx = new PathStreamGeometryContext(FillRule.EvenOdd, Transform); PathGeometry.ParsePathGeometryData(GetPathGeometryData(), ctx); return ctx.GetPathGeometry(); } internal override PathFigureCollection GetTransformedFigureCollection(Transform transform) { // This is lossy for consistency with other GetPathFigureCollection() implementations // however this limitation doesn't otherwise need to exist for LineGeometry. Point startPoint = StartPoint; Point endPoint = EndPoint; // Apply internal transform Transform internalTransform = Transform; if (internalTransform != null && !internalTransform.IsIdentity) { Matrix matrix = internalTransform.Value; startPoint *= matrix; endPoint *= matrix; } // Apply external transform if (transform != null && !transform.IsIdentity) { Matrix matrix = transform.Value; startPoint *= matrix; endPoint *= matrix; } PathFigureCollection collection = new PathFigureCollection(); collection.Add( new PathFigure( startPoint, new PathSegment[]{new LineSegment(endPoint, true)}, false // ==> not closed ) ); return collection; } ////// GetPathGeometryData - returns a byte[] which contains this Geometry represented /// as a path geometry's serialized format. /// internal override PathGeometryData GetPathGeometryData() { if (IsObviouslyEmpty()) { return Geometry.GetEmptyPathGeometryData(); } PathGeometryData data = new PathGeometryData(); data.FillRule = FillRule.EvenOdd; data.Matrix = CompositionResourceManager.TransformToMilMatrix3x2D(Transform); ByteStreamGeometryContext ctx = new ByteStreamGeometryContext(); ctx.BeginFigure(StartPoint, true /* is filled */, false /* is closed */); ctx.LineTo(EndPoint, true /* is stroked */, false /* is smooth join */); ctx.Close(); data.SerializedData = ctx.GetData(); return data; } #region Static Data private const UInt32 c_segmentCount = 1; private const UInt32 c_pointCount = 2; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
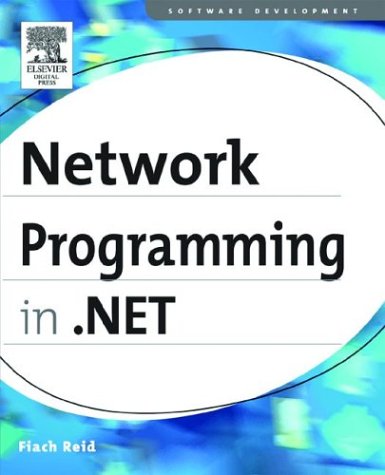
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemas.cs
- EventProxy.cs
- SelectionItemProviderWrapper.cs
- HTTPNotFoundHandler.cs
- ProgressBarRenderer.cs
- PageAsyncTask.cs
- DetailsViewRow.cs
- EllipticalNodeOperations.cs
- StrongNameIdentityPermission.cs
- RadioButton.cs
- UniqueConstraint.cs
- OverloadGroupAttribute.cs
- Figure.cs
- CacheForPrimitiveTypes.cs
- CacheForPrimitiveTypes.cs
- CacheSection.cs
- MetadataArtifactLoaderComposite.cs
- ImageKeyConverter.cs
- ListViewInsertedEventArgs.cs
- StubHelpers.cs
- Publisher.cs
- ValidationException.cs
- FixedSOMContainer.cs
- ValueUnavailableException.cs
- ProcessProtocolHandler.cs
- IntranetCredentialPolicy.cs
- EndEvent.cs
- Html32TextWriter.cs
- RelationshipManager.cs
- FixedTextPointer.cs
- TimeSpanFormat.cs
- Token.cs
- RelatedView.cs
- CommandBinding.cs
- WeakReferenceList.cs
- ListBoxChrome.cs
- MessageDecoder.cs
- RtType.cs
- DefaultAsyncDataDispatcher.cs
- RestClientProxyHandler.cs
- GlyphRun.cs
- SqlDataSourceCustomCommandPanel.cs
- PtsHost.cs
- SevenBitStream.cs
- PersonalizationStateInfo.cs
- ProviderUtil.cs
- ToolboxItem.cs
- UIElement.cs
- elementinformation.cs
- PersonalizableAttribute.cs
- Encoder.cs
- Page.cs
- MsmqReceiveHelper.cs
- XpsFilter.cs
- DataRowComparer.cs
- ComponentSerializationService.cs
- NumericUpDownAcceleration.cs
- AutomationPropertyInfo.cs
- LinkedResourceCollection.cs
- DataGridSortCommandEventArgs.cs
- peernodestatemanager.cs
- ParserHooks.cs
- PrintDocument.cs
- CopyEncoder.cs
- TextLineResult.cs
- TransformGroup.cs
- ParameterRefs.cs
- ReflectionHelper.cs
- FrugalList.cs
- LookupBindingPropertiesAttribute.cs
- QueryInterceptorAttribute.cs
- SoapObjectWriter.cs
- DataGridViewBindingCompleteEventArgs.cs
- ArglessEventHandlerProxy.cs
- ModuleElement.cs
- MethodCallTranslator.cs
- SubtreeProcessor.cs
- BinHexDecoder.cs
- LineMetrics.cs
- SmiSettersStream.cs
- RoleBoolean.cs
- OdbcConnection.cs
- ValidationRuleCollection.cs
- Configuration.cs
- SoapCodeExporter.cs
- WindowsFormsHostPropertyMap.cs
- InvokeMethodDesigner.xaml.cs
- RegistryConfigurationProvider.cs
- UnaryQueryOperator.cs
- RecordManager.cs
- ThicknessConverter.cs
- PropertyCondition.cs
- CultureMapper.cs
- RolePrincipal.cs
- Literal.cs
- EntityCodeGenerator.cs
- StrokeNodeEnumerator.cs
- ComponentResourceKey.cs
- RadioButtonBaseAdapter.cs
- EntityDesignerBuildProvider.cs