Code:
/ DotNET / DotNET / 8.0 / untmp / Orcas / RTM / ndp / fx / src / xsp / System / Web / Extensions / ui / ScriptManager.cs / 2 / ScriptManager.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Collections.Specialized; using System.ComponentModel; using System.Configuration; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Drawing; using System.Drawing.Design; using System.Globalization; using System.IO; using System.Reflection; using System.Security; using System.Security.Permissions; using System.Text; using System.Web; using System.Web.Configuration; using System.Web.UI; using System.Web.UI.HtmlControls; using System.Web.Globalization; using System.Web.Handlers; using System.Web.Resources; using System.Web.Script.Serialization; using System.Web.Script.Services; using System.Web.Util; [ AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal), DefaultProperty("Scripts"), Designer("System.Web.UI.Design.ScriptManagerDesigner, " + AssemblyRef.SystemWebExtensionsDesign), NonVisualControl(), ParseChildren(true), PersistChildren(false), ToolboxBitmap(typeof(EmbeddedResourceFinder), "System.Web.Resources.ScriptManager.bmp"), ] public class ScriptManager : Control, IPostBackDataHandler, IControl, IScriptManager, IScriptManagerInternal { private readonly new IPage _page; private readonly IControl _control; private readonly ICompilationSection _appLevelCompilationSection; private readonly IDeploymentSection _deploymentSection; private readonly ICustomErrorsSection _customErrorsSection; private const int AsyncPostBackTimeoutDefault = 90; private ScriptMode _scriptMode; private string _scriptPath; private ScriptReferenceCollection _scripts; private ServiceReferenceCollection _services; private List_proxies; private bool _enablePartialRendering = true; private bool _supportsPartialRendering = true; internal bool _supportsPartialRenderingSetByUser; private bool _enableScriptGlobalization; private bool _enableScriptLocalization = true; private bool _enablePageMethods; private bool _loadScriptsBeforeUI = true; private bool _initCompleted; private bool _isInAsyncPostBack; private int _asyncPostBackTimeout = AsyncPostBackTimeoutDefault; private bool _allowCustomErrorsRedirect = true; private string _asyncPostBackErrorMessage; private bool _zip; private bool _zipSet; private int _uniqueScriptCounter; private static readonly object AsyncPostBackErrorEvent = new object(); private static readonly object ResolveScriptReferenceEvent = new object(); private ScriptRegistrationManager _scriptRegistration; private PageRequestManager _pageRequestManager; private ScriptControlManager _scriptControlManager; private ProfileServiceManager _profileServiceManager; private AuthenticationServiceManager _authenticationServiceManager; private RoleServiceManager _roleServiceManager; public ScriptManager() { } internal ScriptManager(IControl control, IPage page, ICompilationSection appLevelCompilationSection, IDeploymentSection deploymentSection, ICustomErrorsSection customErrorsSection) { _control = control; _page = page; _appLevelCompilationSection = appLevelCompilationSection; _deploymentSection = deploymentSection; _customErrorsSection = customErrorsSection; } [ DefaultValue(true), ResourceDescription("ScriptManager_AllowCustomErrorsRedirect"), Category("Behavior"), ] public bool AllowCustomErrorsRedirect { get { return _allowCustomErrorsRedirect; } set { _allowCustomErrorsRedirect = value; } } private ICompilationSection AppLevelCompilationSection { get { if (_appLevelCompilationSection != null) { return _appLevelCompilationSection; } else { return AppLevelCompilationSectionCache.Instance; } } } [ DefaultValue(""), ResourceDescription("ScriptManager_AsyncPostBackErrorMessage"), Category("Behavior"), ] public string AsyncPostBackErrorMessage { get { if (_asyncPostBackErrorMessage == null) { return String.Empty; } return _asyncPostBackErrorMessage; } set { _asyncPostBackErrorMessage = value; } } // FxCop does not flag this as a violation, because it is an implicit implementation of // IScriptManagerInternal.AsyncPostBackSourceElementID. // [ Browsable(false), SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase") ] public string AsyncPostBackSourceElementID { get { return PageRequestManager.AsyncPostBackSourceElementID; } } [ ResourceDescription("ScriptManager_AsyncPostBackTimeout"), Category("Behavior"), DefaultValue(AsyncPostBackTimeoutDefault), ] public int AsyncPostBackTimeout { get { return _asyncPostBackTimeout; } set { if (value < 0) { throw new ArgumentOutOfRangeException("value"); } _asyncPostBackTimeout = value; } } [ ResourceDescription("ScriptManager_AuthenticationService"), Category("Behavior"), DefaultValue(null), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), PersistenceMode(PersistenceMode.InnerProperty), MergableProperty(false), ] public AuthenticationServiceManager AuthenticationService { get { if (_authenticationServiceManager == null) { _authenticationServiceManager = new AuthenticationServiceManager(); } return _authenticationServiceManager; } } internal IControl Control { get { if (_control != null) { return _control; } else { return this; } } } internal ICustomErrorsSection CustomErrorsSection { [SecurityCritical()] get { if (_customErrorsSection != null) { return _customErrorsSection; } else { return GetCustomErrorsSectionWithAssert(); } } } private IDeploymentSection DeploymentSection { get { if (_deploymentSection != null) { return _deploymentSection; } else { return DeploymentSectionCache.Instance; } } } internal bool DeploymentSectionRetail { get { return DeploymentSection.Retail; } } [ ResourceDescription("ScriptManager_EnablePageMethods"), Category("Behavior"), DefaultValue(false), ] public bool EnablePageMethods { get { return _enablePageMethods; } set { _enablePageMethods = value; } } [ ResourceDescription("ScriptManager_EnablePartialRendering"), Category("Behavior"), DefaultValue(true), ] public bool EnablePartialRendering { get { return _enablePartialRendering; } set { if (_initCompleted) { throw new InvalidOperationException(AtlasWeb.ScriptManager_CannotChangeEnablePartialRendering); } _enablePartialRendering = value; } } [ ResourceDescription("ScriptManager_EnableScriptGlobalization"), Category("Behavior"), DefaultValue(false), ] public bool EnableScriptGlobalization { get { return _enableScriptGlobalization; } set { if (_initCompleted) { throw new InvalidOperationException(AtlasWeb.ScriptManager_CannotChangeEnableScriptGlobalization); } _enableScriptGlobalization = value; } } [ ResourceDescription("ScriptManager_EnableScriptLocalization"), Category("Behavior"), DefaultValue(true), ] public bool EnableScriptLocalization { get { return _enableScriptLocalization; } set { _enableScriptLocalization = value; } } internal bool HasAuthenticationServiceManager { get { return this._authenticationServiceManager != null; } } internal bool HasProfileServiceManager { get { return this._profileServiceManager != null; } } internal bool HasRoleServiceManager { get { return this._roleServiceManager != null; } } [Browsable(false)] public bool IsDebuggingEnabled { get { // Returns false when: // - Deployment mode is set to retail (override all other settings) // - ScriptMode is set to Auto or Inherit, and debugging it not enabled in web.config // - ScriptMode is set to Release if (DeploymentSectionRetail) { return false; } if (ScriptMode == ScriptMode.Auto || ScriptMode == ScriptMode.Inherit) { return AppLevelCompilationSection.Debug; } return (ScriptMode == ScriptMode.Debug); } } [ Browsable(false), SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase") ] public bool IsInAsyncPostBack { get { return _isInAsyncPostBack; } } internal IPage IPage { get { if (_page != null) { return _page; } else { Page page = Page; if (page == null) { throw new InvalidOperationException(AtlasWeb.Common_PageCannotBeNull); } return new PageWrapper(page); } } } // DevDiv bugs #46710: Ability to specify whether scripts are loaded inline at the top of the form (before UI), or via ScriptLoader (after UI). [ ResourceDescription("ScriptManager_LoadScriptsBeforeUI"), Category("Behavior"), DefaultValue(true), ] public bool LoadScriptsBeforeUI { get { return _loadScriptsBeforeUI; } set { _loadScriptsBeforeUI = value; } } private PageRequestManager PageRequestManager { get { if (_pageRequestManager == null) { _pageRequestManager = new PageRequestManager(this); } return _pageRequestManager; } } [ ResourceDescription("ScriptManager_ProfileService"), Category("Behavior"), DefaultValue(null), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), PersistenceMode(PersistenceMode.InnerProperty), MergableProperty(false), ] public ProfileServiceManager ProfileService { get { if (_profileServiceManager == null) { _profileServiceManager = new ProfileServiceManager(); } return _profileServiceManager; } } internal List Proxies { get { if (_proxies == null) { _proxies = new List (); } return _proxies; } } [ ResourceDescription("ScriptManager_RoleService"), Category("Behavior"), DefaultValue(null), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), PersistenceMode(PersistenceMode.InnerProperty), MergableProperty(false), ] public RoleServiceManager RoleService { get { if(_roleServiceManager == null) { _roleServiceManager = new RoleServiceManager(); } return _roleServiceManager; } } internal ScriptControlManager ScriptControlManager { get { if (_scriptControlManager == null) { _scriptControlManager = new ScriptControlManager(this); } return _scriptControlManager; } } [ ResourceDescription("ScriptManager_ScriptMode"), Category("Behavior"), DefaultValue(ScriptMode.Auto), ] public ScriptMode ScriptMode { get { return _scriptMode; } set { if (value < ScriptMode.Auto || value > ScriptMode.Release) { throw new ArgumentOutOfRangeException("value"); } _scriptMode = value; } } internal ScriptRegistrationManager ScriptRegistration { get { if (_scriptRegistration == null) { _scriptRegistration = new ScriptRegistrationManager(this); } return _scriptRegistration; } } [ ResourceDescription("ScriptManager_Scripts"), Category("Behavior"), Editor("System.Web.UI.Design.CollectionEditorBase, " + AssemblyRef.SystemWebExtensionsDesign, typeof(UITypeEditor)), DefaultValue(null), PersistenceMode(PersistenceMode.InnerProperty), MergableProperty(false), ] public ScriptReferenceCollection Scripts { get { if (_scripts == null) { _scripts = new ScriptReferenceCollection(); } return _scripts; } } [ ResourceDescription("ScriptManager_ScriptPath"), Category("Behavior"), DefaultValue(""), ] public string ScriptPath { get { return (_scriptPath == null) ? String.Empty : _scriptPath; } set { _scriptPath = value; } } [ ResourceDescription("ScriptManager_Services"), Category("Behavior"), Editor("System.Web.UI.Design.CollectionEditorBase, " + AssemblyRef.SystemWebExtensionsDesign, typeof(UITypeEditor)), DefaultValue(null), PersistenceMode(PersistenceMode.InnerProperty), MergableProperty(false), ] public ServiceReferenceCollection Services { get { if (_services == null) { _services = new ServiceReferenceCollection(); } return _services; } } [ Browsable(false), DefaultValue(true), SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase") ] public bool SupportsPartialRendering { get { if (!EnablePartialRendering) { // If the user doesn't even want partial rendering then // we definitely don't support it. return false; } return _supportsPartialRendering; } set { if (!EnablePartialRendering) { throw new InvalidOperationException(AtlasWeb.ScriptManager_CannotSetSupportsPartialRenderingWhenDisabled); } if (_initCompleted) { throw new InvalidOperationException(AtlasWeb.ScriptManager_CannotChangeSupportsPartialRendering); } _supportsPartialRendering = value; // Mark that this was explicitly set. We'll set this back to false if we // explicitly set the value of this property. _supportsPartialRenderingSetByUser = true; } } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), EditorBrowsable(EditorBrowsableState.Never) ] public override bool Visible { get { return base.Visible; } set { throw new NotImplementedException(); } } internal bool Zip { get { if (!_zipSet) { _zip = HeaderUtility.IsEncodingInAcceptList(IPage.Request.Headers["Accept-encoding"], "gzip"); _zipSet = true; } return _zip; } } [ Category("Action"), ResourceDescription("ScriptManager_AsyncPostBackError"), ] public event EventHandler AsyncPostBackError { add { Events.AddHandler(AsyncPostBackErrorEvent, value); } remove { Events.RemoveHandler(AsyncPostBackErrorEvent, value); } } [ Category("Action"), ResourceDescription("ScriptManager_ResolveScriptReference"), ] public event EventHandler ResolveScriptReference { add { Events.AddHandler(ResolveScriptReferenceEvent, value); } remove { Events.RemoveHandler(ResolveScriptReferenceEvent, value); } } private void AddFrameworkLoadedCheck() { // Add check for Sys to give better error message when the framework failed to load. IPage.ClientScript.RegisterClientScriptBlock(typeof(ScriptManager), "FrameworkLoadedCheck", "if (typeof(Sys) === 'undefined') throw new Error('" + JavaScriptString.QuoteString(AtlasWeb.ScriptManager_FrameworkFailedToLoad) + "');\r\n", true); } private static void AddFrameworkScript(ScriptReference frameworkScript, List scripts, int scriptIndex) { // PERF: If scripts.Count <= scriptIndex, then there are no user-specified scripts that might match // the current framework script, so we don't even need to look. if (scripts.Count > scriptIndex) { // For each framework script we want to register, try to find it in the list of user-specified scripts. // If it's there, move it to the top of the list. If it's not there, add it with our default settings. // If multiple user-specified scripts match a framework script, the first one is moved to the top // of the list, and later ones will be removed via RemoveDuplicates(). foreach (ScriptReference script in scripts) { if (script.Name == frameworkScript.Name && script.GetAssembly() == frameworkScript.GetAssembly()) { frameworkScript = script; scripts.Remove(script); break; } } } frameworkScript.AlwaysLoadBeforeUI = true; scripts.Insert(scriptIndex, frameworkScript); } // Called by ScriptManagerDesigner.GetScriptReferences() internal void AddFrameworkScripts(List scripts) { // The 0 and 1 scriptIndex parameter to AddFrameworkScript() is how // we guarantee that the Atlas framework scripts get inserted // consecutively as the first script to be registered. If we add // more optional framework scripts we will have to increment the index // dynamically for each script so that there are no gaps between the // Atlas scripts. ScriptReference atlasCore = new ScriptReference("MicrosoftAjax.js", this, this); AddFrameworkScript(atlasCore, scripts, 0); if (SupportsPartialRendering) { ScriptReference atlasWebForms = new ScriptReference("MicrosoftAjaxWebForms.js", this, this); AddFrameworkScript(atlasWebForms, scripts, 1); } } // Add ScriptReferences from Scripts collections of ScriptManager and ScriptManagerProxies // Called by ScriptManagerDesigner.GetScriptReferences(). internal void AddScriptCollections(List scripts, IEnumerable proxies) { // Register user-specified scripts from the ScriptManager // PERF: Use field directly to avoid creating List if not already created if (_scripts != null) { foreach (ScriptReference scriptReference in _scripts) { scriptReference.ClientUrlResolver = Control; scriptReference.ContainingControl = this; scriptReference.IsStaticReference = true; scripts.Add(scriptReference); } } // Register user-specified scripts from ScriptManagerProxy controls, if any if (proxies != null) { foreach (ScriptManagerProxy proxy in proxies) { proxy.CollectScripts(scripts); } } } private void ConfigureApplicationServices() { StringBuilder sb = null; // Script that configures the application service proxies. For example setting the path properties. ProfileServiceManager.ConfigureProfileService(ref sb, Context, this, _proxies); AuthenticationServiceManager.ConfigureAuthenticationService(ref sb, Context, this, _proxies); RoleServiceManager.ConfigureRoleService(ref sb, Context, this, _proxies); if (sb != null && sb.Length > 0) { this.IPage.ClientScript.RegisterClientScriptBlock(typeof(ScriptManager), /* key */ "AppServicesConfig", sb.ToString(), true); } } internal string CreateUniqueScriptKey() { _uniqueScriptCounter++; return "UniqueScript_" + _uniqueScriptCounter.ToString(CultureInfo.InvariantCulture); } public static ScriptManager GetCurrent(Page page) { if (page == null) { throw new ArgumentNullException("page"); } return page.Items[typeof(ScriptManager)] as ScriptManager; } // AspNetHostingPermission attributes must be copied to this method, to satisfy FxCop rule // CA2114:MethodSecurityShouldBeASupersetOfType. [ AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal), ConfigurationPermission(SecurityAction.Assert, Unrestricted = true), SecurityCritical() ] private static ICustomErrorsSection GetCustomErrorsSectionWithAssert() { return new CustomErrorsSectionWrapper( (CustomErrorsSection)WebConfigurationManager.GetSection("system.web/customErrors")); } [SuppressMessage("Microsoft.Design", "CA1024:UsePropertiesWhereAppropriate", Justification="Depends on registered resources so order of execution is important.")] public ReadOnlyCollection GetRegisteredArrayDeclarations() { return new ReadOnlyCollection (ScriptRegistration.ScriptArrays); } [SuppressMessage("Microsoft.Design", "CA1024:UsePropertiesWhereAppropriate", Justification="Depends on registered resources so order of execution is important.")] public ReadOnlyCollection GetRegisteredClientScriptBlocks() { // includes RegisterClientScriptBlock, RegisterClientScriptInclude, RegisterClientScriptResource return new ReadOnlyCollection (ScriptRegistration.ScriptBlocks); } [SuppressMessage("Microsoft.Design", "CA1024:UsePropertiesWhereAppropriate", Justification="Depends on registered resources so order of execution is important.")] public ReadOnlyCollection GetRegisteredDisposeScripts() { return new ReadOnlyCollection (ScriptRegistration.ScriptDisposes); } [SuppressMessage("Microsoft.Design", "CA1024:UsePropertiesWhereAppropriate", Justification="Depends on registered resources so order of execution is important.")] public ReadOnlyCollection GetRegisteredExpandoAttributes() { return new ReadOnlyCollection (ScriptRegistration.ScriptExpandos); } [SuppressMessage("Microsoft.Design", "CA1024:UsePropertiesWhereAppropriate", Justification="Depends on registered resources so order of execution is important.")] public ReadOnlyCollection GetRegisteredHiddenFields() { return new ReadOnlyCollection (ScriptRegistration.ScriptHiddenFields); } [SuppressMessage("Microsoft.Design", "CA1024:UsePropertiesWhereAppropriate", Justification="Depends on registered resources so order of execution is important.")] public ReadOnlyCollection GetRegisteredOnSubmitStatements() { return new ReadOnlyCollection (ScriptRegistration.ScriptSubmitStatements); } [SuppressMessage("Microsoft.Design", "CA1024:UsePropertiesWhereAppropriate", Justification="Depends on registered resources so order of execution is important.")] public ReadOnlyCollection GetRegisteredStartupScripts() { return new ReadOnlyCollection (ScriptRegistration.ScriptStartupBlocks); } internal string GetScriptResourceUrl(string resourceName, Assembly assembly) { return ScriptResourceHandler.GetScriptResourceUrl( assembly, resourceName, (EnableScriptLocalization ? CultureInfo.CurrentUICulture : CultureInfo.InvariantCulture), Zip, true); } protected virtual bool LoadPostData(string postDataKey, NameValueCollection postCollection) { PageRequestManager.LoadPostData(postDataKey, postCollection); return false; } [SuppressMessage("Microsoft.Security", "CA2109:ReviewVisibleEventHandlers")] protected internal virtual void OnAsyncPostBackError(AsyncPostBackErrorEventArgs e) { EventHandler handler = (EventHandler )Events[AsyncPostBackErrorEvent]; if (handler != null) { handler(this, e); } } [SuppressMessage("Microsoft.Security", "CA2109:ReviewVisibleEventHandlers")] protected internal override void OnInit(EventArgs e) { base.OnInit(e); if (!DesignMode) { IPage page = IPage; ScriptManager existingInstance = ScriptManager.GetCurrent(Page); if (existingInstance != null) { throw new InvalidOperationException(AtlasWeb.ScriptManager_OnlyOneScriptManager); } page.Items[typeof(IScriptManager)] = this; page.Items[typeof(ScriptManager)] = this; page.InitComplete += OnPageInitComplete; page.PreRenderComplete += OnPagePreRenderComplete; if (page.IsPostBack) { _isInAsyncPostBack = PageRequestManager.IsAsyncPostBackRequest(page.Request.Headers); } // Delegate to PageRequestManager to hook up error handling for async posts PageRequestManager.OnInit(); page.PreRender += ScriptControlManager.OnPagePreRender; } } private void OnPagePreRenderComplete(object sender, EventArgs e) { if (!IsInAsyncPostBack) { if (SupportsPartialRendering) { // Force ASP.NET to include the __doPostBack function. If we don't do // this then on the client we might not be able to override the function // (since it won't be defined). // We also need to force it to include the ASP.NET WebForms.js since it // has other required functionality. IPage.ClientScript.GetPostBackEventReference(new PostBackOptions(this, null, null, false, false, false, false, true, null)); } // on GET request we register the glob block... RegisterGlobalizationScriptBlock(); // all script references, declared and from script controls... RegisterScripts(); // and all service references. RegisterServices(); } else { // on async postbacks we only need to register script control references and inline references. RegisterScripts(); } } private void OnPageInitComplete(object sender, EventArgs e) { if (IPage.IsPostBack) { if (IsInAsyncPostBack && !SupportsPartialRendering) { throw new InvalidOperationException(AtlasWeb.ScriptManager_AsyncPostBackNotInPartialRenderingMode); } } _initCompleted = true; } [SuppressMessage("Microsoft.Security", "CA2109:ReviewVisibleEventHandlers")] protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); if (IsInAsyncPostBack) { PageRequestManager.OnPreRender(); } } [SuppressMessage("Microsoft.Security", "CA2109:ReviewVisibleEventHandlers")] protected virtual void OnResolveScriptReference(ScriptReferenceEventArgs e) { EventHandler handler = (EventHandler )Events[ResolveScriptReferenceEvent]; if (handler != null) { handler(this, e); } } [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate", Justification = "Matches IPostBackDataHandler interface.")] protected virtual void RaisePostDataChangedEvent() { } // [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "The overload specifically exists to strengthen the support for passing in a Page parameter.")] public static void RegisterArrayDeclaration(Page page, string arrayName, string arrayValue) { ScriptRegistrationManager.RegisterArrayDeclaration(page, arrayName, arrayValue); } public static void RegisterArrayDeclaration(Control control, string arrayName, string arrayValue) { ScriptRegistrationManager.RegisterArrayDeclaration(control, arrayName, arrayValue); } // Registers a control as causing an async postback instead of a regular postback [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public void RegisterAsyncPostBackControl(Control control) { PageRequestManager.RegisterAsyncPostBackControl(control); } // Internal virtual for testing. Cannot mock static RegisterClientScriptBlock(). internal virtual void RegisterClientScriptBlockInternal(Control control, Type type, string key, string script, bool addScriptTags) { RegisterClientScriptBlock(control, type, key, script, addScriptTags); } [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "The overload specifically exists to strengthen the support for passing in a Page parameter.")] public static void RegisterClientScriptBlock(Page page, Type type, string key, string script, bool addScriptTags) { ScriptRegistrationManager.RegisterClientScriptBlock(page, type, key, script, addScriptTags); } public static void RegisterClientScriptBlock(Control control, Type type, string key, string script, bool addScriptTags) { ScriptRegistrationManager.RegisterClientScriptBlock(control, type, key, script, addScriptTags); } // Internal virtual for testing. Cannot mock static RegisterClientScriptInclude(). internal virtual void RegisterClientScriptIncludeInternal(Control control, Type type, string key, string url) { RegisterClientScriptInclude(control, type, key, url); } [SuppressMessage("Microsoft.Design", "CA1054:UriParametersShouldNotBeStrings", Justification = "Needs to take same parameters as ClientScriptManager.RegisterClientScriptInclude()." + "We could provide an overload that takes a System.Uri parameter, but then FxCop rule " + "StringUriOverloadsCallSystemUriOverloads would require that the string overload call the Uri overload. " + "But we cannot do this, because the ClientScriptManager API allows any string, even invalid Uris. " + "We cannot start throwing exceptions on input we previously passed to the browser. So it does not make " + "sense to add an overload that takes System.Uri.")] [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "The overload specifically exists to strengthen the support for passing in a Page parameter.")] public static void RegisterClientScriptInclude(Page page, Type type, string key, string url) { ScriptRegistrationManager.RegisterClientScriptInclude(page, type, key, url); } [SuppressMessage("Microsoft.Design", "CA1054:UriParametersShouldNotBeStrings", Justification = "Needs to take same parameters as ClientScriptManager.RegisterClientScriptInclude()." + "We could provide an overload that takes a System.Uri parameter, but then FxCop rule " + "StringUriOverloadsCallSystemUriOverloads would require that the string overload call the Uri overload. " + "But we cannot do this, because the ClientScriptManager API allows any string, even invalid Uris. " + "We cannot start throwing exceptions on input we previously passed to the browser. So it does not make " + "sense to add an overload that takes System.Uri.")] public static void RegisterClientScriptInclude(Control control, Type type, string key, string url) { ScriptRegistrationManager.RegisterClientScriptInclude(control, type, key, url); } [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "The overload specifically exists to strengthen the support for passing in a Page parameter.")] public static void RegisterClientScriptResource(Page page, Type type, string resourceName) { ScriptRegistrationManager.RegisterClientScriptResource(page, type, resourceName); } public static void RegisterClientScriptResource(Control control, Type type, string resourceName) { ScriptRegistrationManager.RegisterClientScriptResource(control, type, resourceName); } public void RegisterDataItem(Control control, string dataItem) { RegisterDataItem(control, dataItem, false); } public void RegisterDataItem(Control control, string dataItem, bool isJsonSerialized) { PageRequestManager.RegisterDataItem(control, dataItem, isJsonSerialized); } public void RegisterDispose(Control control, string disposeScript) { if (SupportsPartialRendering) { // DevDiv Bugs 124041: Do not register if SupportsPartialRendering=false // It would cause a script error since PageRequestManager will not exist on the client. ScriptRegistration.RegisterDispose(control, disposeScript); } } public static void RegisterExpandoAttribute(Control control, string controlId, string attributeName, string attributeValue, bool encode) { ScriptRegistrationManager.RegisterExpandoAttribute(control, controlId, attributeName, attributeValue, encode); } [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public void RegisterExtenderControl (TExtenderControl extenderControl, Control targetControl) where TExtenderControl : Control, IExtenderControl { ScriptControlManager.RegisterExtenderControl(extenderControl, targetControl); } private void RegisterGlobalizationScriptBlock() { if (EnableScriptGlobalization) { string script = ClientCultureInfo.GetClientCultureScriptBlock(CultureInfo.CurrentCulture); if (script != null) { ScriptRegistrationManager.RegisterClientScriptBlock(this, typeof(ScriptManager), "CultureInfo", script, true); } } } [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "The overload specifically exists to strengthen the support for passing in a Page parameter.")] public static void RegisterHiddenField(Page page, string hiddenFieldName, string hiddenFieldInitialValue) { ScriptRegistrationManager.RegisterHiddenField(page, hiddenFieldName, hiddenFieldInitialValue); } public static void RegisterHiddenField(Control control, string hiddenFieldName, string hiddenFieldInitialValue) { ScriptRegistrationManager.RegisterHiddenField(control, hiddenFieldName, hiddenFieldInitialValue); } [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "The overload specifically exists to strengthen the support for passing in a Page parameter.")] public static void RegisterOnSubmitStatement(Page page, Type type, string key, string script) { ScriptRegistrationManager.RegisterOnSubmitStatement(page, type, key, script); } public static void RegisterOnSubmitStatement(Control control, Type type, string key, string script) { ScriptRegistrationManager.RegisterOnSubmitStatement(control, type, key, script); } [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public void RegisterScriptControl (TScriptControl scriptControl) where TScriptControl : Control, IScriptControl { ScriptControlManager.RegisterScriptControl(scriptControl); } [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public void RegisterScriptDescriptors(IExtenderControl extenderControl) { ScriptControlManager.RegisterScriptDescriptors(extenderControl); } [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public void RegisterScriptDescriptors(IScriptControl scriptControl) { ScriptControlManager.RegisterScriptDescriptors(scriptControl); } // Registers a control as causing a regular postback instead of an async postback [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public void RegisterPostBackControl(Control control) { PageRequestManager.RegisterPostBackControl(control); } private void RegisterScripts() { List scripts = new List (); // Add ScriptReferences from Scripts collections of ScriptManager and ScriptManagerProxies // PERF: Use _proxies field directly to avoid creating List if not already created AddScriptCollections(scripts, _proxies); // Add ScriptReferences registered by ScriptControls and ExtenderControls ScriptControlManager.AddScriptReferences(scripts); // Inject Atlas Framework scripts AddFrameworkScripts(scripts); // Allow custom resolve work to happen foreach (ScriptReference script in scripts) { OnResolveScriptReference(new ScriptReferenceEventArgs(script)); } // Remove duplicate Name+Assembly references List uniqueScripts = RemoveDuplicates(scripts); // Register the final list of unique scripts RegisterUniqueScripts(uniqueScripts); } private void RegisterUniqueScripts(List uniqueScripts) { bool inlineScriptRegistered = false; bool loadScriptsBeforeUI = this.LoadScriptsBeforeUI; bool debug = IsDebuggingEnabled; foreach (ScriptReference script in uniqueScripts) { string url = script.GetUrl(this, Control, Zip); if (loadScriptsBeforeUI || script.AlwaysLoadBeforeUI) { RegisterClientScriptIncludeInternal(script.ContainingControl, typeof(ScriptManager), url, url); } else { // this method of building the script tag matches exactly ClientScriptManager.RegisterClientScriptInclude string scriptTag = "\r\n"; RegisterStartupScriptInternal(script.ContainingControl, typeof(ScriptManager), url, scriptTag, false); } // configure app services and check framework right after framework script is included & before other scripts if (!inlineScriptRegistered && script.IsFrameworkAssembly()) { // In debug mode, detect if the framework loaded properly before we reference Sys in any way. if (debug && !IsInAsyncPostBack) { AddFrameworkLoadedCheck(); } ConfigureApplicationServices(); inlineScriptRegistered = true; } } } private void RegisterServices() { if (_services != null) { foreach (ServiceReference serviceReference in _services) { serviceReference.Register(this, Context, this, IsDebuggingEnabled); } } if (_proxies != null) { foreach (ScriptManagerProxy proxy in _proxies) { proxy.RegisterServices(this); } } if (EnablePageMethods) { string pageMethods = PageClientProxyGenerator.GetClientProxyScript(Context, IPage, IsDebuggingEnabled); if (!String.IsNullOrEmpty(pageMethods)) { RegisterClientScriptBlockInternal(this, typeof(ScriptManager), pageMethods, pageMethods, true); } } } // Called by ScriptManagerDesigner.GetScriptReferences(). internal static List RemoveDuplicates(List scripts) { // ClientScriptManager.RegisterClientScriptInclude() does not register multiple scripts // with the same type and key. We use the url as the key, so multiple scripts with // the same final url are handled by ClientScriptManager. In ScriptManager, we only // need to remove ScriptReferences that we consider "the same" but that will generate // different urls. For example, Name+Assembly+Path and Name+Assembly will generate // different urls, but we consider them to represent the same script. For this purpose, // two scripts are considered "the same" iff Name is non-empty, and they have the same // Name and Assembly. // Scenario: // Two references from two new instances of the same component that are each in different // UpdatePanels, during an async post, where one update panel is updating and the other is not. // If we remove one of the references because we consider one a duplicate of the other, the // reference remaining may be for the control in the update panel that is not updating, and // it wouldn't be included (incorrectly). // So, references from components can only be considered duplicate against static // script references. The returned list may contain duplicates, but they will be duplicates // across script controls, which may potentially live in different update panels. // When rendering the partial update content, duplicate paths are handled and only one is output. // PERF: Optimize for the following common cases. // - One ScriptReference (i.e. MicrosoftAjax.js). // - Two unique ScriptReferences (i.e. MicrosoftAjax.js and MicrosoftAjaxWebForms.js). It is // unlikely there will be two non-unique ScriptReferences, since the first ScriptReference is always // MicrosoftAjax.js. // - Reduced cost from 0.43% to 0.04% in ScriptManager\HelloWorld\Scenario.aspx. int numScripts = scripts.Count; if (numScripts == 1) { return scripts; } else if (numScripts == 2) { ScriptReference script1 = scripts[0]; ScriptReference script2 = scripts[1]; bool scriptsUnique = (script1.Name != script2.Name) || (script1.Assembly != script2.Assembly); if (scriptsUnique) { return scripts; } } // PERF: HybridDictionary is significantly more performant than Dictionary , since the number // of scripts will frequently be small. Reduced cost from 1.49% to 0.43% in // ScriptManager\HelloWorld\Scenario.aspx. HybridDictionary uniqueScriptDict = new HybridDictionary(numScripts); List filteredScriptList = new List (numScripts); foreach (ScriptReference script in scripts) { if (String.IsNullOrEmpty(script.Name)) { filteredScriptList.Add(script); } else { Pair key = new Pair (script.Name, script.GetAssembly()); if (!uniqueScriptDict.Contains(key)) { if (script.IsStaticReference) { // only static script references are compared against for duplicates. uniqueScriptDict.Add(key, script); } filteredScriptList.Add(script); } } } return filteredScriptList; } // Internal virtual for testing. Cannot mock static RegisterStartupScript(). internal virtual void RegisterStartupScriptInternal(Control control, Type type, string key, string script, bool addScriptTags) { RegisterStartupScript(control, type, key, script, addScriptTags); } [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "The overload specifically exists to strengthen the support for passing in a Page parameter.")] public static void RegisterStartupScript(Page page, Type type, string key, string script, bool addScriptTags) { ScriptRegistrationManager.RegisterStartupScript(page, type, key, script, addScriptTags); } public static void RegisterStartupScript(Control control, Type type, string key, string script, bool addScriptTags) { ScriptRegistrationManager.RegisterStartupScript(control, type, key, script, addScriptTags); } protected internal override void Render(HtmlTextWriter writer) { PageRequestManager.Render(writer); if (!IsInAsyncPostBack) { // call app.initialize for perf -- so it occurs before binary content is downloaded IPage.ClientScript.RegisterStartupScript(typeof(ScriptManager), "AppInitialize", "Sys.Application.initialize();\r\n", true); } base.Render(writer); } public void SetFocus(Control control) { PageRequestManager.SetFocus(control); } [SuppressMessage("Microsoft.Naming", "CA1706:ShortAcronymsShouldBeUppercase", MessageId = "0#")] public void SetFocus(string clientID) { PageRequestManager.SetFocus(clientID); } #region IControl Members IHttpContext IControl.Context { get { return new HttpContextWrapper(Context); } } bool IControl.DesignMode { get { return DesignMode; } } #endregion #region IScriptManagerInternal Members void IScriptManagerInternal.RegisterProxy(ScriptManagerProxy proxy) { // Under normal circumstances a ScriptManagerProxy will only register once per page lifecycle. // However, a malicious page developer could trick a proxy into registering more than once, // and this is guarding against that. if (!Proxies.Contains(proxy)) { Proxies.Add(proxy); } } void IScriptManagerInternal.RegisterUpdatePanel(UpdatePanel updatePanel) { PageRequestManager.RegisterUpdatePanel(updatePanel); } void IScriptManagerInternal.UnregisterUpdatePanel(UpdatePanel updatePanel) { PageRequestManager.UnregisterUpdatePanel(updatePanel); } #endregion #region IPostBackDataHandler Members bool IPostBackDataHandler.LoadPostData(string postDataKey, NameValueCollection postCollection) { return LoadPostData(postDataKey, postCollection); } void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } #endregion #region IScriptManager Members void IScriptManager.RegisterArrayDeclaration(Control control, string arrayName, string arrayValue) { RegisterArrayDeclaration(control, arrayName, arrayValue); } void IScriptManager.RegisterClientScriptBlock(Control control, Type type, string key, string script, bool addScriptTags) { RegisterClientScriptBlock(control, type, key, script, addScriptTags); } void IScriptManager.RegisterClientScriptInclude(Control control, Type type, string key, string url) { RegisterClientScriptInclude(control, type, key, url); } void IScriptManager.RegisterClientScriptResource(Control control, Type type, string resourceName) { RegisterClientScriptResource(control, type, resourceName); } void IScriptManager.RegisterDispose(Control control, string disposeScript) { RegisterDispose(control, disposeScript); } void IScriptManager.RegisterExpandoAttribute(Control control, string controlId, string attributeName, string attributeValue, bool encode) { RegisterExpandoAttribute(control, controlId, attributeName, attributeValue, encode); } void IScriptManager.RegisterHiddenField(Control control, string hiddenFieldName, string hiddenFieldValue) { RegisterHiddenField(control, hiddenFieldName, hiddenFieldValue); } void IScriptManager.RegisterOnSubmitStatement(Control control, Type type, string key, string script) { RegisterOnSubmitStatement(control, type, key, script); } void IScriptManager.RegisterPostBackControl(Control control) { RegisterPostBackControl(control); } void IScriptManager.RegisterStartupScript(Control control, Type type, string key, string script, bool addScriptTags) { RegisterStartupScript(control, type, key, script, addScriptTags); } void IScriptManager.SetFocusInternal(string clientID) { PageRequestManager.SetFocusInternal(clientID); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
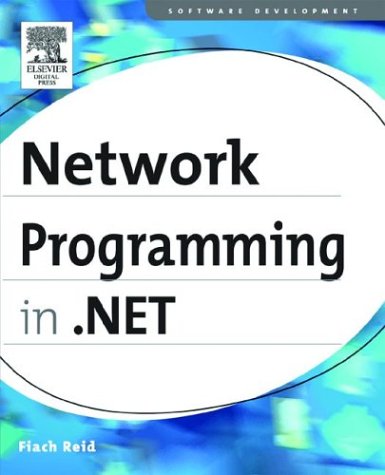
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpVersion.cs
- MetadataItem.cs
- IdlingCommunicationPool.cs
- FileDialog.cs
- TextShapeableCharacters.cs
- ComboBox.cs
- BufferedWebEventProvider.cs
- UniqueConstraint.cs
- GlyphCollection.cs
- EventProviderWriter.cs
- UserCancellationException.cs
- GuidelineSet.cs
- AuthenticationException.cs
- ObjectSelectorEditor.cs
- Function.cs
- Attributes.cs
- TextReader.cs
- GestureRecognitionResult.cs
- EntityClassGenerator.cs
- IndexedString.cs
- ArglessEventHandlerProxy.cs
- DecodeHelper.cs
- HttpException.cs
- DiscoveryVersion.cs
- SqlReorderer.cs
- _Connection.cs
- DataTemplateKey.cs
- FixedFindEngine.cs
- MetadataHelper.cs
- TreeBuilderXamlTranslator.cs
- OuterGlowBitmapEffect.cs
- MediaCommands.cs
- IPipelineRuntime.cs
- TypefaceMetricsCache.cs
- InlinedAggregationOperator.cs
- CustomErrorCollection.cs
- StrokeCollection.cs
- ListParaClient.cs
- Transform3D.cs
- AddInPipelineAttributes.cs
- Transform.cs
- SqlException.cs
- GenericIdentity.cs
- BackoffTimeoutHelper.cs
- HighlightOverlayGlyph.cs
- ContentElement.cs
- XAMLParseException.cs
- FixedSOMPage.cs
- File.cs
- SemaphoreFullException.cs
- InkPresenter.cs
- FastPropertyAccessor.cs
- CodeGenerator.cs
- SamlSecurityTokenAuthenticator.cs
- DataGridViewButtonColumn.cs
- OutputCacheEntry.cs
- ListViewSortEventArgs.cs
- DataGridViewSortCompareEventArgs.cs
- WinCategoryAttribute.cs
- PathTooLongException.cs
- DataServiceHost.cs
- GlyphElement.cs
- JsonSerializer.cs
- DesignerCapabilities.cs
- CatalogZoneBase.cs
- GenericPrincipal.cs
- DynamicQueryableWrapper.cs
- MarkupExtensionReturnTypeAttribute.cs
- DynamicValidatorEventArgs.cs
- ImageAutomationPeer.cs
- ProcessHostMapPath.cs
- XmlKeywords.cs
- XhtmlBasicLiteralTextAdapter.cs
- Encoding.cs
- OleDbReferenceCollection.cs
- SamlDelegatingWriter.cs
- PointAnimation.cs
- CompilerParameters.cs
- FileLogRecordEnumerator.cs
- UnmanagedMarshal.cs
- ZipIOCentralDirectoryBlock.cs
- altserialization.cs
- CallSiteBinder.cs
- WindowsIPAddress.cs
- ResourceReader.cs
- FixedSOMSemanticBox.cs
- BuildProviderCollection.cs
- AuthorizationRuleCollection.cs
- CollaborationHelperFunctions.cs
- MdImport.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- SendingRequestEventArgs.cs
- UnknownBitmapDecoder.cs
- X509Certificate2.cs
- TextOnlyOutput.cs
- XPathDocument.cs
- KnownColorTable.cs
- DataRelationPropertyDescriptor.cs
- AsymmetricSignatureDeformatter.cs
- KeyPullup.cs