Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / XmlUtils / System / Xml / Xsl / Runtime / XmlILStorageConverter.cs / 1 / XmlILStorageConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.IO; using System.Xml; using System.Xml.XPath; using System.Xml.Schema; using System.Xml.Xsl; using System.ComponentModel; namespace System.Xml.Xsl.Runtime { ////// This is a simple convenience wrapper internal class that contains static helper methods that get a value /// converter from XmlQueryRuntime and use it convert among several physical Clr representations for /// the same logical Xml type. For example, an external function might have an argument typed as /// xs:integer, with Clr type Decimal. Since ILGen stores xs:integer as Clr type Int64 instead of /// Decimal, a conversion to the desired storage type must take place. /// [EditorBrowsable(EditorBrowsableState.Never)] public static class XmlILStorageConverter { //----------------------------------------------- // ToAtomicValue //----------------------------------------------- public static XmlAtomicValue StringToAtomicValue(string value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue DecimalToAtomicValue(decimal value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue Int64ToAtomicValue(long value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue Int32ToAtomicValue(int value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue BooleanToAtomicValue(bool value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue DoubleToAtomicValue(double value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue SingleToAtomicValue(float value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue DateTimeToAtomicValue(DateTime value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue XmlQualifiedNameToAtomicValue(XmlQualifiedName value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue TimeSpanToAtomicValue(TimeSpan value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue BytesToAtomicValue(byte[] value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static IListNavigatorsToItems(IList listNavigators) { // Check to see if the navigator cache implements IList IList listItems = listNavigators as IList ; if (listItems != null) return listItems; // Create XmlQueryNodeSequence, which does implement IList return new XmlQueryNodeSequence(listNavigators); } public static IList ItemsToNavigators(IList listItems) { // Check to see if the navigator cache implements IList IList listNavs = listItems as IList ; if (listNavs != null) return listNavs; // Create XmlQueryNodeSequence, which does implement IList XmlQueryNodeSequence seq = new XmlQueryNodeSequence(listItems.Count); for (int i = 0; i < listItems.Count; i++) seq.Add((XPathNavigator) listItems[i]); return seq; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.IO; using System.Xml; using System.Xml.XPath; using System.Xml.Schema; using System.Xml.Xsl; using System.ComponentModel; namespace System.Xml.Xsl.Runtime { ////// This is a simple convenience wrapper internal class that contains static helper methods that get a value /// converter from XmlQueryRuntime and use it convert among several physical Clr representations for /// the same logical Xml type. For example, an external function might have an argument typed as /// xs:integer, with Clr type Decimal. Since ILGen stores xs:integer as Clr type Int64 instead of /// Decimal, a conversion to the desired storage type must take place. /// [EditorBrowsable(EditorBrowsableState.Never)] public static class XmlILStorageConverter { //----------------------------------------------- // ToAtomicValue //----------------------------------------------- public static XmlAtomicValue StringToAtomicValue(string value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue DecimalToAtomicValue(decimal value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue Int64ToAtomicValue(long value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue Int32ToAtomicValue(int value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue BooleanToAtomicValue(bool value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue DoubleToAtomicValue(double value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue SingleToAtomicValue(float value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue DateTimeToAtomicValue(DateTime value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue XmlQualifiedNameToAtomicValue(XmlQualifiedName value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue TimeSpanToAtomicValue(TimeSpan value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue BytesToAtomicValue(byte[] value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static IListNavigatorsToItems(IList listNavigators) { // Check to see if the navigator cache implements IList IList listItems = listNavigators as IList ; if (listItems != null) return listItems; // Create XmlQueryNodeSequence, which does implement IList return new XmlQueryNodeSequence(listNavigators); } public static IList ItemsToNavigators(IList listItems) { // Check to see if the navigator cache implements IList IList listNavs = listItems as IList ; if (listNavs != null) return listNavs; // Create XmlQueryNodeSequence, which does implement IList XmlQueryNodeSequence seq = new XmlQueryNodeSequence(listItems.Count); for (int i = 0; i < listItems.Count; i++) seq.Add((XPathNavigator) listItems[i]); return seq; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
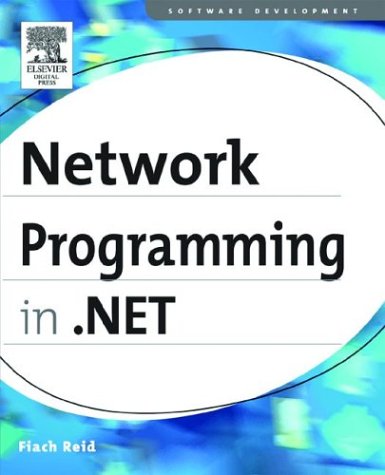
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GraphicsPathIterator.cs
- CellIdBoolean.cs
- ResourceDescriptionAttribute.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- TextContainerHelper.cs
- TextTreePropertyUndoUnit.cs
- XmlSchemaAll.cs
- DescriptionAttribute.cs
- ListChangedEventArgs.cs
- XpsFilter.cs
- DesignerVerbCollection.cs
- Thread.cs
- ModifierKeysValueSerializer.cs
- IDispatchConstantAttribute.cs
- X509CertificateValidator.cs
- JavaScriptString.cs
- BrowserCapabilitiesFactoryBase.cs
- XmlNamedNodeMap.cs
- MouseActionConverter.cs
- SettingsSection.cs
- ClientData.cs
- WebPartsPersonalizationAuthorization.cs
- LiteralTextContainerControlBuilder.cs
- CodeCommentStatementCollection.cs
- XamlDesignerSerializationManager.cs
- CacheRequest.cs
- XdrBuilder.cs
- NotifyParentPropertyAttribute.cs
- ExternalException.cs
- XmlEventCache.cs
- NonBatchDirectoryCompiler.cs
- LoadRetryHandler.cs
- CorrelationKeyCalculator.cs
- SqlBooleanizer.cs
- XmlValidatingReader.cs
- FieldBuilder.cs
- DependencyObjectValidator.cs
- ExecutionContext.cs
- TextWriter.cs
- XDeferredAxisSource.cs
- RadioButtonBaseAdapter.cs
- Events.cs
- TCPClient.cs
- SqlPersonalizationProvider.cs
- WebPartManager.cs
- StyleSheet.cs
- TrimSurroundingWhitespaceAttribute.cs
- FlowLayoutSettings.cs
- LightweightEntityWrapper.cs
- SafeArchiveContext.cs
- MailMessage.cs
- QueryOperationResponseOfT.cs
- SafeRightsManagementHandle.cs
- StdValidatorsAndConverters.cs
- ViewBase.cs
- ADMembershipProvider.cs
- Stylesheet.cs
- OperationResponse.cs
- PointCollection.cs
- XmlSchemaIdentityConstraint.cs
- ListViewUpdatedEventArgs.cs
- SecurityKeyEntropyMode.cs
- ServiceAppDomainAssociationProvider.cs
- DoubleAnimation.cs
- QuaternionAnimationBase.cs
- SqlConnectionHelper.cs
- LineProperties.cs
- UInt16Converter.cs
- SortedSet.cs
- HttpRuntime.cs
- CreateUserErrorEventArgs.cs
- WinFormsSpinner.cs
- LassoSelectionBehavior.cs
- BooleanAnimationUsingKeyFrames.cs
- SweepDirectionValidation.cs
- BaseProcessProtocolHandler.cs
- HtmlTable.cs
- ContentFilePart.cs
- UnSafeCharBuffer.cs
- CachingParameterInspector.cs
- FormatConvertedBitmap.cs
- MemberInfoSerializationHolder.cs
- TranslateTransform3D.cs
- ModifierKeysValueSerializer.cs
- SyndicationSerializer.cs
- FlowDocumentReaderAutomationPeer.cs
- StateItem.cs
- FontStyle.cs
- CellQuery.cs
- securestring.cs
- SQLSingleStorage.cs
- ResourcesGenerator.cs
- ContentElement.cs
- DataGridViewColumnCollection.cs
- VideoDrawing.cs
- DebugView.cs
- TableItemStyle.cs
- TextTreePropertyUndoUnit.cs
- SettingsPropertyValue.cs
- SemaphoreFullException.cs