Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / FontStyle.cs / 1305600 / FontStyle.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: FontStyle structure. // // History: // 01/25/2005 mleonov - Converted FontStyle from enum to a value type and moved it to a separate file. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; namespace System.Windows { ////// FontStyle structure describes the style of a font face, such as Normal, Italic or Oblique. /// [TypeConverter(typeof(FontStyleConverter))] [Localizability(LocalizationCategory.None)] public struct FontStyle : IFormattable { internal FontStyle(int style) { Debug.Assert(0 <= style && style <= 2); _style = style; } ////// Checks whether two font weight objects are equal. /// /// First object to compare. /// Second object to compare. ///Returns true when the font weight values are equal for both objects, /// and false otherwise. public static bool operator ==(FontStyle left, FontStyle right) { return left._style == right._style; } ////// Checks whether two font weight objects are not equal. /// /// First object to compare. /// Second object to compare. ///Returns false when the font weight values are equal for both objects, /// and true otherwise. public static bool operator !=(FontStyle left, FontStyle right) { return !(left == right); } ////// Checks whether the object is equal to another FontStyle object. /// /// FontStyle object to compare with. ///Returns true when the object is equal to the input object, /// and false otherwise. public bool Equals(FontStyle obj) { return this == obj; } ////// Checks whether an object is equal to another character hit object. /// /// FontStyle object to compare with. ///Returns true when the object is equal to the input object, /// and false otherwise. public override bool Equals(object obj) { if (!(obj is FontStyle)) return false; return this == (FontStyle)obj; } ////// Compute hash code for this object. /// ///A 32-bit signed integer hash code. public override int GetHashCode() { return _style; } ////// Creates a string representation of this object based on the current culture. /// ////// A string representation of this object. /// public override string ToString() { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null, null); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// This method is used only in type converter for construction via reflection. /// ///THe value of _style member. internal int GetStyleForInternalConstruction() { Debug.Assert(0 <= _style && _style <= 2); return _style; } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// private string ConvertToString(string format, IFormatProvider provider) { if (_style == 0) return "Normal"; if (_style == 1) return "Oblique"; Debug.Assert(_style == 2); return "Italic"; } private int _style; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
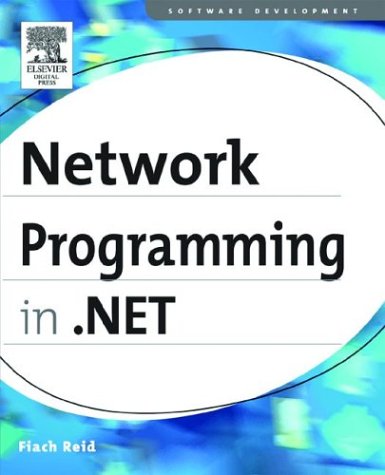
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartsPersonalizationAuthorization.cs
- ActivityExecutionContext.cs
- HttpListener.cs
- ELinqQueryState.cs
- Int32Collection.cs
- SoapReflectionImporter.cs
- Line.cs
- CompensationDesigner.cs
- Annotation.cs
- lengthconverter.cs
- Point3DAnimationUsingKeyFrames.cs
- EntityDataSourceUtil.cs
- NumberAction.cs
- StructuralCache.cs
- CacheMemory.cs
- GridViewColumnCollectionChangedEventArgs.cs
- DataGridViewRowCollection.cs
- MembershipUser.cs
- Thumb.cs
- IisTraceWebEventProvider.cs
- HttpFileCollection.cs
- RichTextBox.cs
- DbInsertCommandTree.cs
- OleDbPropertySetGuid.cs
- TypeConverterValueSerializer.cs
- WindowsListBox.cs
- SimpleHandlerBuildProvider.cs
- Win32.cs
- MimeImporter.cs
- DetailsViewDeleteEventArgs.cs
- EventHandlersStore.cs
- ProxyHwnd.cs
- SqlDataSourceCommandEventArgs.cs
- PersonalizablePropertyEntry.cs
- MissingSatelliteAssemblyException.cs
- NotifyCollectionChangedEventArgs.cs
- TriggerAction.cs
- SoapParser.cs
- MimeMultiPart.cs
- BoundField.cs
- SubMenuStyle.cs
- RecipientInfo.cs
- HtmlPanelAdapter.cs
- ProcessManager.cs
- MessageDecoder.cs
- compensatingcollection.cs
- InputLanguageManager.cs
- XmlLanguage.cs
- ColorContext.cs
- TemplateEditingService.cs
- NodeLabelEditEvent.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- BinaryNode.cs
- DeviceSpecificChoiceCollection.cs
- ResourceAttributes.cs
- RSAPKCS1KeyExchangeFormatter.cs
- Timer.cs
- CellPartitioner.cs
- ModuleConfigurationInfo.cs
- TreeNodeClickEventArgs.cs
- NavigationWindowAutomationPeer.cs
- XamlTreeBuilder.cs
- WindowsIPAddress.cs
- DebugTrace.cs
- XmlFormatMapping.cs
- DesigntimeLicenseContextSerializer.cs
- XhtmlBasicLinkAdapter.cs
- ClientBuildManagerCallback.cs
- PropertyPathConverter.cs
- UriParserTemplates.cs
- EventToken.cs
- IteratorDescriptor.cs
- MemberProjectionIndex.cs
- XmlDataLoader.cs
- StateItem.cs
- GroupItemAutomationPeer.cs
- DataConnectionHelper.cs
- DBCommandBuilder.cs
- MarkupCompilePass2.cs
- TableLayoutStyle.cs
- MD5.cs
- XmlCodeExporter.cs
- DiscoveryInnerClientAdhocCD1.cs
- StructuredTypeEmitter.cs
- CachedFontFamily.cs
- PresentationTraceSources.cs
- Tokenizer.cs
- SimpleExpression.cs
- BinaryExpression.cs
- SubMenuStyleCollection.cs
- TextShapeableCharacters.cs
- ValidationManager.cs
- WebControlsSection.cs
- UrlMapping.cs
- PlacementWorkspace.cs
- CodeAttributeDeclaration.cs
- EventWaitHandle.cs
- HelpKeywordAttribute.cs
- FileSystemEventArgs.cs
- XmlElementAttributes.cs