Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / Diagnostics / PresentationTraceSources.cs / 1305600 / PresentationTraceSources.cs
/****************************************************************************\ * * File: PresentationTraceSources.cs * * This class provides a public interface to access TraceSources for * enabling/disabling/filtering of trace messages by area. * * The other portion of this partial class is generated using * genTraceSource.pl and AvTraceMessages.txt * * Copyright (C) by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System.Collections.Generic; using System.Security; using System.Security.Permissions; using MS.Internal; using System.Windows; namespace System.Diagnostics { ////// PresentationTraceLevel - Enum which describes how much detail to trace about a particular object. /// public enum PresentationTraceLevel { ////// Trace no additional information. /// None, ////// Trace some additional information. /// Low, ////// Trace a medium amount of additional information. /// Medium, ////// Trace all available additional information. /// High, } ////// Helper class for retrieving TraceSources /// public static partial class PresentationTraceSources { //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// DependencyProperty for TraceLevel property. /// public static readonly DependencyProperty TraceLevelProperty = DependencyProperty.RegisterAttached( "TraceLevel", typeof(PresentationTraceLevel), typeof(PresentationTraceSources)); ////// Reads the attached property TraceLevel from the given element. /// public static PresentationTraceLevel GetTraceLevel(object element) { return TraceLevelStore.GetTraceLevel(element); } ////// Writes the attached property TraceLevel to the given element. /// public static void SetTraceLevel(object element, PresentationTraceLevel traceLevel) { TraceLevelStore.SetTraceLevel(element, traceLevel); } //----------------------------------------------------- // // Public Methods // //------------------------------------------------------ ////// Refresh TraceSources (re-read config file), creating if necessary. /// // Note: Better would be to separate enable from the Refresh method. public static void Refresh() { // Let AvTrace know that an explicit Refresh has been called. AvTrace.OnRefresh(); // Re-read the .config files System.Diagnostics.Trace.Refresh(); // Initialize any traces classes if needed if (TraceRefresh != null) { TraceRefresh(); } } internal static event TraceRefreshEventHandler TraceRefresh; ////// Critical: /// 1) Asserts for UMC to set trace level. /// /// TreatAsSafe: /// 1) The code path is only invoked when under a debugger /// if the caller can attach a debugger they already have /// unlimited access to the process. Also the only inputs /// to the assert are simply a label for the name. /// [SecurityCritical, SecurityTreatAsSafe] private static TraceSource CreateTraceSource(string sourceName) { // Create the trace source. Whether or not it will actually // trace anything is a decision of the trace source, e.g. it // depends on the app.config file settings. TraceSource source = new TraceSource(sourceName); // If we're attached to the debugger, ensure that at least // warnings/errors are getting traced. if (source.Switch.Level == SourceLevels.Off && AvTrace.IsDebuggerAttached()) { // we need to assert as PT callers under a debugger can invoke this code path // with out having the needed permission to peform this action new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Assert(); // BlessedAssert try { source.Switch.Level = SourceLevels.Warning; } finally { SecurityPermission.RevertAssert(); } } // returning source after reverting the assert to avoid // using exposed elements under the assert return source; } } internal delegate void TraceRefreshEventHandler(); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: PresentationTraceSources.cs * * This class provides a public interface to access TraceSources for * enabling/disabling/filtering of trace messages by area. * * The other portion of this partial class is generated using * genTraceSource.pl and AvTraceMessages.txt * * Copyright (C) by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System.Collections.Generic; using System.Security; using System.Security.Permissions; using MS.Internal; using System.Windows; namespace System.Diagnostics { ////// PresentationTraceLevel - Enum which describes how much detail to trace about a particular object. /// public enum PresentationTraceLevel { ////// Trace no additional information. /// None, ////// Trace some additional information. /// Low, ////// Trace a medium amount of additional information. /// Medium, ////// Trace all available additional information. /// High, } ////// Helper class for retrieving TraceSources /// public static partial class PresentationTraceSources { //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// DependencyProperty for TraceLevel property. /// public static readonly DependencyProperty TraceLevelProperty = DependencyProperty.RegisterAttached( "TraceLevel", typeof(PresentationTraceLevel), typeof(PresentationTraceSources)); ////// Reads the attached property TraceLevel from the given element. /// public static PresentationTraceLevel GetTraceLevel(object element) { return TraceLevelStore.GetTraceLevel(element); } ////// Writes the attached property TraceLevel to the given element. /// public static void SetTraceLevel(object element, PresentationTraceLevel traceLevel) { TraceLevelStore.SetTraceLevel(element, traceLevel); } //----------------------------------------------------- // // Public Methods // //------------------------------------------------------ ////// Refresh TraceSources (re-read config file), creating if necessary. /// // Note: Better would be to separate enable from the Refresh method. public static void Refresh() { // Let AvTrace know that an explicit Refresh has been called. AvTrace.OnRefresh(); // Re-read the .config files System.Diagnostics.Trace.Refresh(); // Initialize any traces classes if needed if (TraceRefresh != null) { TraceRefresh(); } } internal static event TraceRefreshEventHandler TraceRefresh; ////// Critical: /// 1) Asserts for UMC to set trace level. /// /// TreatAsSafe: /// 1) The code path is only invoked when under a debugger /// if the caller can attach a debugger they already have /// unlimited access to the process. Also the only inputs /// to the assert are simply a label for the name. /// [SecurityCritical, SecurityTreatAsSafe] private static TraceSource CreateTraceSource(string sourceName) { // Create the trace source. Whether or not it will actually // trace anything is a decision of the trace source, e.g. it // depends on the app.config file settings. TraceSource source = new TraceSource(sourceName); // If we're attached to the debugger, ensure that at least // warnings/errors are getting traced. if (source.Switch.Level == SourceLevels.Off && AvTrace.IsDebuggerAttached()) { // we need to assert as PT callers under a debugger can invoke this code path // with out having the needed permission to peform this action new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Assert(); // BlessedAssert try { source.Switch.Level = SourceLevels.Warning; } finally { SecurityPermission.RevertAssert(); } } // returning source after reverting the assert to avoid // using exposed elements under the assert return source; } } internal delegate void TraceRefreshEventHandler(); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
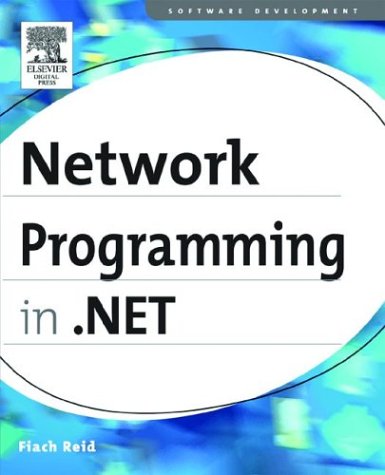
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PointCollection.cs
- Wow64ConfigurationLoader.cs
- StringHandle.cs
- EdmValidator.cs
- _HelperAsyncResults.cs
- MDIClient.cs
- DataObjectSettingDataEventArgs.cs
- IndentedWriter.cs
- StringComparer.cs
- ConfigurationPermission.cs
- GridView.cs
- SamlAudienceRestrictionCondition.cs
- ConcatQueryOperator.cs
- RegexMatch.cs
- ExecutionEngineException.cs
- PathSegmentCollection.cs
- EdmConstants.cs
- AsyncResult.cs
- IndexedEnumerable.cs
- ScriptResourceHandler.cs
- ParserHooks.cs
- PowerModeChangedEventArgs.cs
- PropertyValueChangedEvent.cs
- ListDataHelper.cs
- OdbcHandle.cs
- ManagementObjectSearcher.cs
- ConstraintConverter.cs
- TextTreeTextElementNode.cs
- Cursors.cs
- _SpnDictionary.cs
- Transform.cs
- CacheEntry.cs
- CellParagraph.cs
- KnownTypes.cs
- GridViewColumnHeader.cs
- FixedDocument.cs
- FormDocumentDesigner.cs
- XamlGridLengthSerializer.cs
- CharEntityEncoderFallback.cs
- MarshalByRefObject.cs
- ClassGenerator.cs
- CompensationParticipant.cs
- EntityDataSourceDesigner.cs
- XmlSchemaCompilationSettings.cs
- Light.cs
- TraceProvider.cs
- DivideByZeroException.cs
- XmlJsonWriter.cs
- XmlSchemaValidationException.cs
- Hashtable.cs
- BoolLiteral.cs
- Evidence.cs
- SystemInformation.cs
- ViewStateModeByIdAttribute.cs
- Attributes.cs
- SingleAnimationUsingKeyFrames.cs
- HandledMouseEvent.cs
- PerformanceCounter.cs
- SecurityContext.cs
- _TransmitFileOverlappedAsyncResult.cs
- NotifyIcon.cs
- SoapAttributes.cs
- XmlObjectSerializer.cs
- XmlCharacterData.cs
- EditorAttribute.cs
- FormViewPageEventArgs.cs
- ResXBuildProvider.cs
- PropertyCollection.cs
- Int32.cs
- XmlSchemaComplexContentRestriction.cs
- ReadOnlyDataSourceView.cs
- WhiteSpaceTrimStringConverter.cs
- iisPickupDirectory.cs
- _ProxyChain.cs
- XmlSerializerAssemblyAttribute.cs
- DataQuery.cs
- RawUIStateInputReport.cs
- SafeNativeMemoryHandle.cs
- WeakReferenceList.cs
- ReferencedType.cs
- RoutedEventConverter.cs
- SQLBytesStorage.cs
- FormatterConverter.cs
- PersonalizationStateQuery.cs
- QilDataSource.cs
- HiddenField.cs
- CompilationRelaxations.cs
- ControlPropertyNameConverter.cs
- DbgUtil.cs
- EntityDataSourceState.cs
- FileClassifier.cs
- AlternationConverter.cs
- CaseExpr.cs
- SecurityHelper.cs
- UrlAuthorizationModule.cs
- StylusCaptureWithinProperty.cs
- BitStream.cs
- ListControl.cs
- GiveFeedbackEventArgs.cs
- Line.cs