Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / Configuration / UrlMapping.cs / 1 / UrlMapping.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.ComponentModel; using System.Web.Util; using System.Diagnostics; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class UrlMapping : ConfigurationElement { private static ConfigurationPropertyCollection _properties; #region Property Declarations private static readonly ConfigurationProperty _propUrl = new ConfigurationProperty("url", typeof(string), null, StdValidatorsAndConverters.WhiteSpaceTrimStringConverter, new CallbackValidator(typeof(string), ValidateUrl), ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propMappedUrl = new ConfigurationProperty("mappedUrl", typeof(string), null, StdValidatorsAndConverters.WhiteSpaceTrimStringConverter, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired); #endregion static UrlMapping() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propUrl); _properties.Add(_propMappedUrl); } internal UrlMapping() { } public UrlMapping(string url, string mappedUrl) { base[_propUrl] = url; base[_propMappedUrl] = mappedUrl; } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("url", IsRequired = true, IsKey = true)] public string Url { get { return (string)base[_propUrl]; } } [ConfigurationProperty("mappedUrl", IsRequired = true)] public string MappedUrl { get { return (string)base[_propMappedUrl]; } } static private void ValidateUrl(object value) { // The Url cannot be an empty string. Use the std validator for that StdValidatorsAndConverters.NonEmptyStringValidator.Validate(value); string url = (string)value; if (!UrlPath.IsAppRelativePath(url)) { throw new ConfigurationErrorsException(SR.GetString(SR.UrlMappings_only_app_relative_url_allowed, url)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.ComponentModel; using System.Web.Util; using System.Diagnostics; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class UrlMapping : ConfigurationElement { private static ConfigurationPropertyCollection _properties; #region Property Declarations private static readonly ConfigurationProperty _propUrl = new ConfigurationProperty("url", typeof(string), null, StdValidatorsAndConverters.WhiteSpaceTrimStringConverter, new CallbackValidator(typeof(string), ValidateUrl), ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propMappedUrl = new ConfigurationProperty("mappedUrl", typeof(string), null, StdValidatorsAndConverters.WhiteSpaceTrimStringConverter, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired); #endregion static UrlMapping() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propUrl); _properties.Add(_propMappedUrl); } internal UrlMapping() { } public UrlMapping(string url, string mappedUrl) { base[_propUrl] = url; base[_propMappedUrl] = mappedUrl; } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("url", IsRequired = true, IsKey = true)] public string Url { get { return (string)base[_propUrl]; } } [ConfigurationProperty("mappedUrl", IsRequired = true)] public string MappedUrl { get { return (string)base[_propMappedUrl]; } } static private void ValidateUrl(object value) { // The Url cannot be an empty string. Use the std validator for that StdValidatorsAndConverters.NonEmptyStringValidator.Validate(value); string url = (string)value; if (!UrlPath.IsAppRelativePath(url)) { throw new ConfigurationErrorsException(SR.GetString(SR.UrlMappings_only_app_relative_url_allowed, url)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
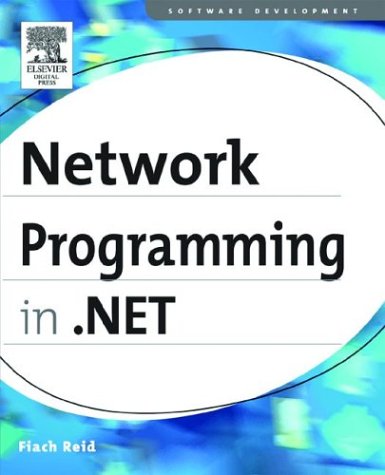
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GlyphTypeface.cs
- ReplyAdapterChannelListener.cs
- TextServicesDisplayAttribute.cs
- SynchronizedInputAdaptor.cs
- HttpChannelListener.cs
- ModelItem.cs
- BamlBinaryReader.cs
- GridProviderWrapper.cs
- EpmSyndicationContentDeSerializer.cs
- DataSourceIDConverter.cs
- MULTI_QI.cs
- OleDbEnumerator.cs
- XmlCodeExporter.cs
- ReflectionPermission.cs
- SqlFlattener.cs
- ClientBuildManager.cs
- AddInToken.cs
- HttpDictionary.cs
- MSAAEventDispatcher.cs
- AtomPub10CategoriesDocumentFormatter.cs
- TreeIterators.cs
- CheckoutException.cs
- CheckBoxAutomationPeer.cs
- ValidatingReaderNodeData.cs
- LocalValueEnumerator.cs
- PrivateUnsafeNativeCompoundFileMethods.cs
- CapabilitiesUse.cs
- CustomCategoryAttribute.cs
- MenuAutomationPeer.cs
- HttpException.cs
- XmlFormatExtensionPrefixAttribute.cs
- PenThreadPool.cs
- PropertyGroupDescription.cs
- HttpChannelHelpers.cs
- FieldNameLookup.cs
- IndentTextWriter.cs
- SqlDependency.cs
- PointCollection.cs
- DataMemberAttribute.cs
- HttpProfileBase.cs
- SuspendDesigner.cs
- ComplexObject.cs
- ExpressionConverter.cs
- InternalControlCollection.cs
- CommentEmitter.cs
- SiteIdentityPermission.cs
- DependencyPropertyDescriptor.cs
- FileInfo.cs
- ValueUtilsSmi.cs
- NativeMethods.cs
- NativeMethods.cs
- WinFormsSecurity.cs
- XmlSerializerVersionAttribute.cs
- NavigatorInput.cs
- CodeBinaryOperatorExpression.cs
- PassportPrincipal.cs
- RawKeyboardInputReport.cs
- RTLAwareMessageBox.cs
- FeedUtils.cs
- AnnotationService.cs
- BindValidationContext.cs
- InputEventArgs.cs
- InstanceHandleConflictException.cs
- ImageIndexEditor.cs
- DiscoveryDocumentLinksPattern.cs
- ForwardPositionQuery.cs
- XPathNodeIterator.cs
- ConfigXmlDocument.cs
- HttpRequest.cs
- NopReturnReader.cs
- SslStream.cs
- SQLSingle.cs
- DocumentXmlWriter.cs
- DataGridTable.cs
- EditorBrowsableAttribute.cs
- SrgsGrammarCompiler.cs
- HostProtectionPermission.cs
- CheckBoxField.cs
- SynchronizedCollection.cs
- DataGridColumn.cs
- ApplicationFileParser.cs
- DataGridViewRowHeaderCell.cs
- XPathNavigator.cs
- SplitContainer.cs
- PropertyTabAttribute.cs
- SetterBase.cs
- DataListCommandEventArgs.cs
- CountdownEvent.cs
- TokenBasedSet.cs
- EasingQuaternionKeyFrame.cs
- DispatchWrapper.cs
- FormatterConverter.cs
- ImageSourceValueSerializer.cs
- WizardSideBarListControlItem.cs
- HttpCookie.cs
- EventWaitHandle.cs
- AnimationClockResource.cs
- FlowDecisionDesigner.xaml.cs
- XPathPatternParser.cs
- RandomNumberGenerator.cs