Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Documents / TextServicesDisplayAttribute.cs / 1 / TextServicesDisplayAttribute.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: TextServicesDisplayAttribute // // History: // 08/01/2003 : yutakas - Ported from dotnet tree. // //--------------------------------------------------------------------------- using System.Runtime.InteropServices; using System.Windows.Threading; using System.Collections; using System.Diagnostics; using System.Windows.Media; using System.Windows.Input; using System.Windows.Documents; using MS.Win32; using System; namespace System.Windows.Documents { //----------------------------------------------------- // // TextServicesDisplayAttribute class // //----------------------------------------------------- ////// The helper class to wrap TF_DISPLAYATTRIBUTE. /// internal class TextServicesDisplayAttribute { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- internal TextServicesDisplayAttribute(UnsafeNativeMethods.TF_DISPLAYATTRIBUTE attr) { _attr = attr; } //------------------------------------------------------ // // Internal Method // //------------------------------------------------------ ////// Check if this is empty. /// internal bool IsEmptyAttribute() { if (_attr.crText.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.crBk.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.crLine.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.lsStyle != UnsafeNativeMethods.TF_DA_LINESTYLE.TF_LS_NONE) return false; return true; } ////// Apply the display attribute to to the given range. /// internal void Apply(ITextPointer start, ITextPointer end) { // // #if NOT_YET if (_attr.crText.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE) { } if (_attr.crBk.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE) { } if (_attr.lsStyle != UnsafeNativeMethods.TF_DA_LINESTYLE.TF_LS_NONE) { } #endif } ////// Convert TF_DA_COLOR to Color. /// internal static Color GetColor(UnsafeNativeMethods.TF_DA_COLOR dacolor) { if (dacolor.type == UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_SYSCOLOR) { return GetSystemColor(dacolor.indexOrColorRef); } else if (dacolor.type == UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_COLORREF) { int color = dacolor.indexOrColorRef; uint argb = (uint)FromWin32Value(color); return Color.FromArgb((byte)((argb&0xff000000)>>24), (byte)((argb&0x00ff0000)>>16),(byte)((argb&0x0000ff00)>>8), (byte)(argb&0x000000ff)); } return Color.FromArgb(0xff,0,0,0); } ////// Line color of the composition line draw /// internal Color LineColor { get { return GetColor(_attr.crLine); } } ////// Line style of the composition line draw /// internal UnsafeNativeMethods.TF_DA_LINESTYLE LineStyle { get { return _attr.lsStyle; } } ////// Line bold of the composition line draw /// internal bool IsBoldLine { get { return _attr.fBoldLine; } } /** * Shift count and bit mask for A, R, G, B components */ private const int AlphaShift = 24; private const int RedShift = 16; private const int GreenShift = 8; private const int BlueShift = 0; private const int Win32RedShift = 0; private const int Win32GreenShift = 8; private const int Win32BlueShift = 16; private static int Encode(int alpha, int red, int green, int blue) { return red << RedShift | green << GreenShift | blue << BlueShift | alpha << AlphaShift; } private static int FromWin32Value(int value) { return Encode(255, (value >> Win32RedShift) & 0xFF, (value >> Win32GreenShift) & 0xFF, (value >> Win32BlueShift) & 0xFF); } ////// Query for system colors. /// /// Same parameter as Win32's GetSysColor ///The system color. private static Color GetSystemColor(int index) { uint argb; int color = SafeNativeMethods.GetSysColor(index); argb = (uint)FromWin32Value(color); return Color.FromArgb((byte)((argb&0xff000000)>>24), (byte)((argb&0x00ff0000)>>16),(byte)((argb&0x0000ff00)>>8), (byte)(argb&0x000000ff)); } //----------------------------------------------------- // // Private Field // //------------------------------------------------------ private UnsafeNativeMethods.TF_DISPLAYATTRIBUTE _attr; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: TextServicesDisplayAttribute // // History: // 08/01/2003 : yutakas - Ported from dotnet tree. // //--------------------------------------------------------------------------- using System.Runtime.InteropServices; using System.Windows.Threading; using System.Collections; using System.Diagnostics; using System.Windows.Media; using System.Windows.Input; using System.Windows.Documents; using MS.Win32; using System; namespace System.Windows.Documents { //----------------------------------------------------- // // TextServicesDisplayAttribute class // //----------------------------------------------------- ////// The helper class to wrap TF_DISPLAYATTRIBUTE. /// internal class TextServicesDisplayAttribute { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- internal TextServicesDisplayAttribute(UnsafeNativeMethods.TF_DISPLAYATTRIBUTE attr) { _attr = attr; } //------------------------------------------------------ // // Internal Method // //------------------------------------------------------ ////// Check if this is empty. /// internal bool IsEmptyAttribute() { if (_attr.crText.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.crBk.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.crLine.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.lsStyle != UnsafeNativeMethods.TF_DA_LINESTYLE.TF_LS_NONE) return false; return true; } ////// Apply the display attribute to to the given range. /// internal void Apply(ITextPointer start, ITextPointer end) { // // #if NOT_YET if (_attr.crText.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE) { } if (_attr.crBk.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE) { } if (_attr.lsStyle != UnsafeNativeMethods.TF_DA_LINESTYLE.TF_LS_NONE) { } #endif } ////// Convert TF_DA_COLOR to Color. /// internal static Color GetColor(UnsafeNativeMethods.TF_DA_COLOR dacolor) { if (dacolor.type == UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_SYSCOLOR) { return GetSystemColor(dacolor.indexOrColorRef); } else if (dacolor.type == UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_COLORREF) { int color = dacolor.indexOrColorRef; uint argb = (uint)FromWin32Value(color); return Color.FromArgb((byte)((argb&0xff000000)>>24), (byte)((argb&0x00ff0000)>>16),(byte)((argb&0x0000ff00)>>8), (byte)(argb&0x000000ff)); } return Color.FromArgb(0xff,0,0,0); } ////// Line color of the composition line draw /// internal Color LineColor { get { return GetColor(_attr.crLine); } } ////// Line style of the composition line draw /// internal UnsafeNativeMethods.TF_DA_LINESTYLE LineStyle { get { return _attr.lsStyle; } } ////// Line bold of the composition line draw /// internal bool IsBoldLine { get { return _attr.fBoldLine; } } /** * Shift count and bit mask for A, R, G, B components */ private const int AlphaShift = 24; private const int RedShift = 16; private const int GreenShift = 8; private const int BlueShift = 0; private const int Win32RedShift = 0; private const int Win32GreenShift = 8; private const int Win32BlueShift = 16; private static int Encode(int alpha, int red, int green, int blue) { return red << RedShift | green << GreenShift | blue << BlueShift | alpha << AlphaShift; } private static int FromWin32Value(int value) { return Encode(255, (value >> Win32RedShift) & 0xFF, (value >> Win32GreenShift) & 0xFF, (value >> Win32BlueShift) & 0xFF); } ////// Query for system colors. /// /// Same parameter as Win32's GetSysColor ///The system color. private static Color GetSystemColor(int index) { uint argb; int color = SafeNativeMethods.GetSysColor(index); argb = (uint)FromWin32Value(color); return Color.FromArgb((byte)((argb&0xff000000)>>24), (byte)((argb&0x00ff0000)>>16),(byte)((argb&0x0000ff00)>>8), (byte)(argb&0x000000ff)); } //----------------------------------------------------- // // Private Field // //------------------------------------------------------ private UnsafeNativeMethods.TF_DISPLAYATTRIBUTE _attr; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
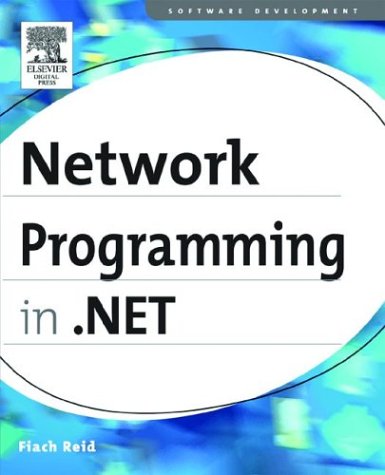
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Transform3D.cs
- CounterCreationDataCollection.cs
- Closure.cs
- SubMenuStyle.cs
- ButtonStandardAdapter.cs
- GetImportedCardRequest.cs
- oledbmetadatacolumnnames.cs
- ClockGroup.cs
- BuilderPropertyEntry.cs
- SecureEnvironment.cs
- Int64Animation.cs
- StringBuilder.cs
- ScriptResourceInfo.cs
- ByteRangeDownloader.cs
- TouchesCapturedWithinProperty.cs
- PageCodeDomTreeGenerator.cs
- HashStream.cs
- HtmlWindowCollection.cs
- Assert.cs
- MappingModelBuildProvider.cs
- SynchronizedPool.cs
- User.cs
- dsa.cs
- UpdatableWrapper.cs
- TypedReference.cs
- PrinterResolution.cs
- AlignmentXValidation.cs
- SafeEventLogWriteHandle.cs
- TraceLevelStore.cs
- HttpBindingExtension.cs
- SineEase.cs
- XmlSchemaExporter.cs
- ADRoleFactoryConfiguration.cs
- StatusBarAutomationPeer.cs
- OperationAbortedException.cs
- SqlFunctions.cs
- TdsParserHelperClasses.cs
- IgnoreSectionHandler.cs
- DataGridComboBoxColumn.cs
- LocalizabilityAttribute.cs
- TimelineCollection.cs
- FixedSchema.cs
- FontConverter.cs
- EntityContainer.cs
- DataRowExtensions.cs
- ToolBarTray.cs
- ISAPIRuntime.cs
- OletxResourceManager.cs
- RegexCode.cs
- HttpCapabilitiesSectionHandler.cs
- SubpageParaClient.cs
- RepeatButton.cs
- Menu.cs
- EmptyEnumerator.cs
- SortExpressionBuilder.cs
- VisualStateGroup.cs
- WebHostScriptMappingsInstallComponent.cs
- _UriSyntax.cs
- WebPartActionVerb.cs
- ProviderCollection.cs
- DeleteIndexBinder.cs
- FileLevelControlBuilderAttribute.cs
- RestHandlerFactory.cs
- SpellerHighlightLayer.cs
- DrawingContextWalker.cs
- HotSpotCollection.cs
- StringUtil.cs
- MemberCollection.cs
- Reference.cs
- TemplatePagerField.cs
- Activation.cs
- MulticastOption.cs
- PermissionSetTriple.cs
- TaskResultSetter.cs
- DesignerCalendarAdapter.cs
- AssemblyAttributesGoHere.cs
- NetworkStream.cs
- PlainXmlSerializer.cs
- TypeConverter.cs
- MethodAccessException.cs
- Calendar.cs
- AlphabetConverter.cs
- WebPartZoneBase.cs
- BooleanExpr.cs
- DbException.cs
- IsolationInterop.cs
- InvalidDocumentContentsException.cs
- safesecurityhelperavalon.cs
- ImportContext.cs
- ListViewItemEventArgs.cs
- ThreadAttributes.cs
- TemplateLookupAction.cs
- MatrixKeyFrameCollection.cs
- BitArray.cs
- QualifiedCellIdBoolean.cs
- ToolZone.cs
- LocalizationComments.cs
- MarkupCompilePass1.cs
- sqlpipe.cs
- Hex.cs