Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / InputOutput / Activation.cs / 1 / Activation.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // This file implements activation-related messaging using System; using System.ServiceModel.Channels; using System.Diagnostics; using System.ServiceModel; using System.Xml; using Microsoft.Transactions.Wsat.Messaging; using Microsoft.Transactions.Wsat.Protocol; using Microsoft.Transactions.Wsat.StateMachines; namespace Microsoft.Transactions.Wsat.InputOutput { class ActivationCoordinator : IActivationCoordinator { ProtocolState state; public ActivationCoordinator (ProtocolState state) { this.state = state; } // // IActivationCoordinator // public void CreateCoordinationContext(Message message, RequestAsyncResult result) { CreateCoordinationContext create = new CreateCoordinationContext (message, this.state.ProtocolVersion); CoordinationContext context = create.CurrentContext; if (context == null) { CompletionEnlistment completion = new CompletionEnlistment(state); completion.StateMachine.Enqueue(new MsgCreateTransactionEvent(completion, ref create, result)); } else { // Find or create a new transaction context manager TransactionContextManager contextManager; contextManager = state.Lookup.FindTransactionContextManager(context.Identifier); if (contextManager == null) { contextManager = new TransactionContextManager(state, context.Identifier); bool found; contextManager = state.Lookup.FindOrAddTransactionContextManager(contextManager, out found); } // We can't just return contextManager.TransactionContext, since we may not be in a state // for which that property is valid. The state machine is wise and will take things from here. contextManager.StateMachine.Enqueue(new TransactionContextEnlistTransactionEvent(contextManager, ref create, result)); } } // // Sending messages // public void SendCreateCoordinationContextResponse(TransactionContext txContext, RequestAsyncResult result) { CreateCoordinationContextResponse response = new CreateCoordinationContextResponse(this.state.ProtocolVersion); response.CoordinationContext = txContext.CoordinationContext; response.IssuedToken = txContext.IssuedToken; if (DebugTrace.Info) { DebugTrace.Trace(TraceLevel.Info, "Sending CreateCoordinationContextResponse"); } ActivationProxy.SendCreateCoordinationContextResponse(result, ref response); } public void SendFault(RequestAsyncResult result, Microsoft.Transactions.Wsat.Messaging.Fault fault) { state.Perf.FaultsSentCountPerInterval.Increment(); if (DebugTrace.Warning) { DebugTrace.Trace(TraceLevel.Warning, "Sending {0} fault to activation participant", fault.Code.Name); } ActivationProxy.SendFaultResponse (result, fault); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
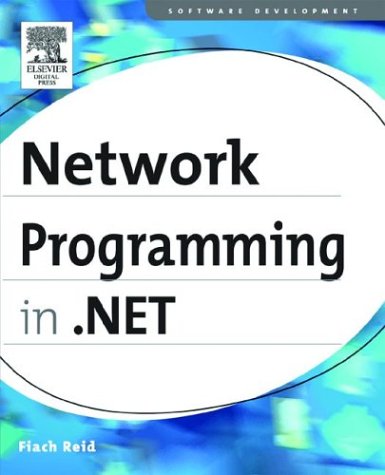
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AssemblyLoader.cs
- ServerValidateEventArgs.cs
- TraceHandlerErrorFormatter.cs
- SoapAttributeAttribute.cs
- BuilderInfo.cs
- WpfGeneratedKnownTypes.cs
- SystemBrushes.cs
- BaseCAMarshaler.cs
- ImageListUtils.cs
- SharedStream.cs
- CodeAttributeArgumentCollection.cs
- CommandField.cs
- ToolStripDropTargetManager.cs
- CacheChildrenQuery.cs
- SocketPermission.cs
- TransformerInfoCollection.cs
- ResourceDictionaryCollection.cs
- ImageSourceConverter.cs
- LateBoundChannelParameterCollection.cs
- EntityViewGenerator.cs
- JpegBitmapEncoder.cs
- Event.cs
- EncoderParameter.cs
- WebPartManager.cs
- DynamicILGenerator.cs
- CheckBoxPopupAdapter.cs
- ClientRoleProvider.cs
- DataGridLinkButton.cs
- HtmlTextArea.cs
- HandleRef.cs
- EntityDataSourceDataSelectionPanel.cs
- DataServiceQueryContinuation.cs
- TextTreeRootNode.cs
- PageThemeParser.cs
- ListDataBindEventArgs.cs
- ClonableStack.cs
- AggregatePushdown.cs
- RequestContext.cs
- _DomainName.cs
- LogEntry.cs
- Utils.cs
- MultiView.cs
- GenerateHelper.cs
- entityreference_tresulttype.cs
- SqlBulkCopyColumnMapping.cs
- AppSettingsReader.cs
- HttpSysSettings.cs
- DataGridViewCellStyle.cs
- PrivateUnsafeNativeCompoundFileMethods.cs
- ParseElementCollection.cs
- QueryRewriter.cs
- CFStream.cs
- ServiceDescription.cs
- ActiveDocumentEvent.cs
- EntryPointNotFoundException.cs
- TaskScheduler.cs
- SqlAliasesReferenced.cs
- Activity.cs
- ProgressBar.cs
- ellipse.cs
- FileDialog_Vista.cs
- Application.cs
- EdmTypeAttribute.cs
- XmlSerializableWriter.cs
- BlockUIContainer.cs
- HttpSysSettings.cs
- Atom10FormatterFactory.cs
- StrokeNodeEnumerator.cs
- CodePrimitiveExpression.cs
- DirectoryInfo.cs
- VisualBrush.cs
- Selection.cs
- HealthMonitoringSectionHelper.cs
- SchemaImporter.cs
- srgsitem.cs
- DesignerVerbToolStripMenuItem.cs
- ActivationServices.cs
- ConfigurationSection.cs
- SafeCryptHandles.cs
- MenuItem.cs
- ServiceHttpHandlerFactory.cs
- TextRange.cs
- SessionViewState.cs
- EventEntry.cs
- BitArray.cs
- DetailsViewPageEventArgs.cs
- XPathPatternParser.cs
- IntSecurity.cs
- ColumnMapCopier.cs
- XmlSerializableServices.cs
- graph.cs
- InitializationEventAttribute.cs
- QueryOutputWriter.cs
- BridgeDataRecord.cs
- TreeViewImageKeyConverter.cs
- DSASignatureDeformatter.cs
- OpenTypeLayoutCache.cs
- DirectionalLight.cs
- ServiceModelEnhancedConfigurationElementCollection.cs
- MimeWriter.cs