Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / DataServiceQueryContinuation.cs / 1305376 / DataServiceQueryContinuation.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a class to represent the continuation of a query. // //--------------------------------------------------------------------- namespace System.Data.Services.Client { #region Namespaces. using System.Collections.Generic; using System.Diagnostics; using System.Linq.Expressions; using System.Text; using System.Reflection; #endregion Namespaces. ///Use this class to represent the continuation of a query. [DebuggerDisplay("{NextLinkUri}")] public abstract class DataServiceQueryContinuation { #region Private fields. ///URI to next page of data. private readonly Uri nextLinkUri; ///Projection plan for results of next page. private readonly ProjectionPlan plan; #endregion Private fields. #region Constructors. ///Initializes a new /// URI to next page of data. /// Projection plan for results of next page. internal DataServiceQueryContinuation(Uri nextLinkUri, ProjectionPlan plan) { Debug.Assert(nextLinkUri != null, "nextLinkUri != null"); Debug.Assert(plan != null, "plan != null"); this.nextLinkUri = nextLinkUri; this.plan = plan; } #endregion Contructors. #region Properties. ///instance. The URI to the next page of data. public Uri NextLinkUri { get { return this.nextLinkUri; } } ///Type of element to be paged over. internal abstract Type ElementType { get; } ///Projection plan for the next page of data; null if not available. internal ProjectionPlan Plan { get { return this.plan; } } #endregion Properties. #region Methods. ///Provides a string representation of this continuation. ///String representation. public override string ToString() { return this.NextLinkUri.ToString(); } ///Creates a new /// Link to next page of data (possibly null). /// Plan to materialize the data (only null if nextLinkUri is null). ///instance. A new continuation object; null if nextLinkUri is null. internal static DataServiceQueryContinuation Create(Uri nextLinkUri, ProjectionPlan plan) { Debug.Assert(plan != null || nextLinkUri == null, "plan != null || nextLinkUri == null"); if (nextLinkUri == null) { return null; } var constructors = typeof(DataServiceQueryContinuation<>).MakeGenericType(plan.ProjectedType).GetConstructors(BindingFlags.NonPublic | BindingFlags.Instance); Debug.Assert(constructors.Length == 1, "constructors.Length == 1"); object result = Util.ConstructorInvoke(constructors[0], new object[] { nextLinkUri, plan }); return (DataServiceQueryContinuation)result; } ////// Initializes a new ///instance that can /// be used for this continuation. /// A new initializes internal QueryComponents CreateQueryComponents() { QueryComponents result = new QueryComponents(this.NextLinkUri, Util.DataServiceVersionEmpty, this.Plan.LastSegmentType, null, null); return result; } #endregion Methods. } ///. Use this class to represent the continuation of a query. ///Element type. public sealed class DataServiceQueryContinuation: DataServiceQueryContinuation { #region Contructors. /// Initializes a new typed instance. /// URI to next page of data. /// Projection plan for results of next page. internal DataServiceQueryContinuation(Uri nextLinkUri, ProjectionPlan plan) : base(nextLinkUri, plan) { } #endregion Contructors. #region Properties. ///Type of element to be paged over. internal override Type ElementType { get { return typeof(T); } } #endregion Properties. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
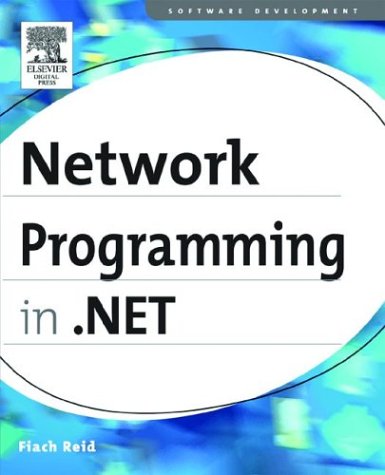
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RouteParameter.cs
- FormViewPageEventArgs.cs
- EditableTreeList.cs
- InfoCardSchemas.cs
- ObjectStateEntry.cs
- _SSPIWrapper.cs
- ClientType.cs
- HttpUnhandledOperationInvoker.cs
- UpdateExpressionVisitor.cs
- PixelShader.cs
- TextContainerChangedEventArgs.cs
- OutputCacheSettingsSection.cs
- RecipientInfo.cs
- _DomainName.cs
- ConfigurationManagerInternalFactory.cs
- Attributes.cs
- RuntimeWrappedException.cs
- CodeValidator.cs
- Int64Converter.cs
- ResourcesChangeInfo.cs
- ImageBrush.cs
- OlePropertyStructs.cs
- Misc.cs
- Renderer.cs
- isolationinterop.cs
- ChildTable.cs
- RegexMatch.cs
- X509InitiatorCertificateClientElement.cs
- XmlNamespaceDeclarationsAttribute.cs
- ErrorsHelper.cs
- GetLedgerEntryForRecipientRequest.cs
- XPathCompileException.cs
- InputLanguageSource.cs
- lengthconverter.cs
- ConfigurationCollectionAttribute.cs
- MetadataSource.cs
- AspNetSynchronizationContext.cs
- ProjectionPath.cs
- GlobalEventManager.cs
- _LoggingObject.cs
- SQLConvert.cs
- SourceItem.cs
- VideoDrawing.cs
- RuntimeVariablesExpression.cs
- Html32TextWriter.cs
- EnumBuilder.cs
- SRef.cs
- StorageFunctionMapping.cs
- InternalConfigEventArgs.cs
- CharStorage.cs
- _SslSessionsCache.cs
- LinearGradientBrush.cs
- XPathNavigatorKeyComparer.cs
- SpellerInterop.cs
- DbProviderFactoriesConfigurationHandler.cs
- RelationshipEndCollection.cs
- QueryOutputWriter.cs
- AutomationElementIdentifiers.cs
- AttributeProviderAttribute.cs
- PreservationFileWriter.cs
- ThreadStateException.cs
- ProviderMetadata.cs
- TaskHelper.cs
- XamlPoint3DCollectionSerializer.cs
- UIElement.cs
- SHA1Managed.cs
- WebPartDisplayModeEventArgs.cs
- ResourceDescriptionAttribute.cs
- StrongBox.cs
- Variant.cs
- SmiSettersStream.cs
- HwndStylusInputProvider.cs
- EventQueueState.cs
- DelegateTypeInfo.cs
- EndPoint.cs
- ContextStaticAttribute.cs
- XmlAtomErrorReader.cs
- XmlSchemaSet.cs
- ImageAutomationPeer.cs
- XmlHierarchicalDataSourceView.cs
- Configuration.cs
- UserPersonalizationStateInfo.cs
- CanonicalXml.cs
- AnimationTimeline.cs
- _NetworkingPerfCounters.cs
- WeakEventManager.cs
- ContractHandle.cs
- StateManagedCollection.cs
- SafeRightsManagementPubHandle.cs
- CapabilitiesState.cs
- BinHexEncoding.cs
- XmlImplementation.cs
- Utils.cs
- NavigateEvent.cs
- TextServicesCompartmentEventSink.cs
- AuthenticationSection.cs
- CacheOutputQuery.cs
- CodeTypeReferenceCollection.cs
- Span.cs
- PropertyMetadata.cs