Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Syndication / AtomPub10CategoriesDocumentFormatter.cs / 2 / AtomPub10CategoriesDocumentFormatter.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Syndication { using System.Collections.ObjectModel; using System.Runtime.Serialization; using System.Xml.Serialization; using System.Collections.Generic; using System.Xml; using System.Xml.Schema; using System.ServiceModel.Channels; using System.ServiceModel.Diagnostics; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; [XmlRoot(ElementName = App10Constants.Categories, Namespace = App10Constants.Namespace)] public class AtomPub10CategoriesDocumentFormatter : CategoriesDocumentFormatter, IXmlSerializable { Type inlineDocumentType; int maxExtensionSize; bool preserveAttributeExtensions; bool preserveElementExtensions; Type referencedDocumentType; public AtomPub10CategoriesDocumentFormatter() : this(typeof(InlineCategoriesDocument), typeof(ReferencedCategoriesDocument)) { } public AtomPub10CategoriesDocumentFormatter(Type inlineDocumentType, Type referencedDocumentType) : base() { if (inlineDocumentType == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("inlineDocumentType"); } if (!typeof(InlineCategoriesDocument).IsAssignableFrom(inlineDocumentType)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("inlineDocumentType", SR2.GetString(SR2.InvalidObjectTypePassed, "inlineDocumentType", "InlineCategoriesDocument")); } if (referencedDocumentType == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("referencedDocumentType"); } if (!typeof(ReferencedCategoriesDocument).IsAssignableFrom(referencedDocumentType)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("referencedDocumentType", SR2.GetString(SR2.InvalidObjectTypePassed, "referencedDocumentType", "ReferencedCategoriesDocument")); } this.maxExtensionSize = int.MaxValue; this.preserveAttributeExtensions = true; this.preserveElementExtensions = true; this.inlineDocumentType = inlineDocumentType; this.referencedDocumentType = referencedDocumentType; } public AtomPub10CategoriesDocumentFormatter(CategoriesDocument documentToWrite) : base(documentToWrite) { // No need to check that the parameter passed is valid - it is checked by the c'tor of the base class this.maxExtensionSize = int.MaxValue; preserveAttributeExtensions = true; preserveElementExtensions = true; if (documentToWrite.IsInline) { this.inlineDocumentType = documentToWrite.GetType(); this.referencedDocumentType = typeof(ReferencedCategoriesDocument); } else { this.referencedDocumentType = documentToWrite.GetType(); this.inlineDocumentType = typeof(InlineCategoriesDocument); } } public override string Version { get { return App10Constants.Namespace; } } public override bool CanRead(XmlReader reader) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } return reader.IsStartElement(App10Constants.Categories, App10Constants.Namespace); } [SuppressMessage("Microsoft.Design", "CA1033:InterfaceMethodsShouldBeCallableByChildTypes", Justification = "The IXmlSerializable implementation is only for exposing under WCF DataContractSerializer. The funcionality is exposed to derived class through the ReadFrom\\WriteTo methods")] XmlSchema IXmlSerializable.GetSchema() { return null; } [SuppressMessage("Microsoft.Design", "CA1033:InterfaceMethodsShouldBeCallableByChildTypes", Justification = "The IXmlSerializable implementation is only for exposing under WCF DataContractSerializer. The funcionality is exposed to derived class through the ReadFrom\\WriteTo methods")] void IXmlSerializable.ReadXml(XmlReader reader) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } TraceCategoriesDocumentReadBegin(); ReadDocument(reader); TraceCategoriesDocumentReadEnd(); } [SuppressMessage("Microsoft.Design", "CA1033:InterfaceMethodsShouldBeCallableByChildTypes", Justification = "The IXmlSerializable implementation is only for exposing under WCF DataContractSerializer. The funcionality is exposed to derived class through the ReadFrom\\WriteTo methods")] void IXmlSerializable.WriteXml(XmlWriter writer) { if (writer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("writer"); } if (this.Document == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR2.GetString(SR2.DocumentFormatterDoesNotHaveDocument))); } TraceCategoriesDocumentWriteBegin(); WriteDocument(writer); TraceCategoriesDocumentWriteEnd(); } public override void ReadFrom(XmlReader reader) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } if (!CanRead(reader)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(SR2.GetString(SR2.UnknownDocumentXml, reader.LocalName, reader.NamespaceURI))); } TraceCategoriesDocumentReadBegin(); ReadDocument(reader); TraceCategoriesDocumentReadEnd(); } public override void WriteTo(XmlWriter writer) { if (writer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("writer"); } if (this.Document == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR2.GetString(SR2.DocumentFormatterDoesNotHaveDocument))); } TraceCategoriesDocumentWriteBegin(); writer.WriteStartElement(App10Constants.Prefix, App10Constants.Categories, App10Constants.Namespace); WriteDocument(writer); writer.WriteEndElement(); TraceCategoriesDocumentWriteEnd(); } internal static void TraceCategoriesDocumentReadBegin() { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.DiagnosticTrace.TraceEvent(TraceEventType.Information, TraceCode.SyndicationReadCategoriesDocumentBegin, SR2.GetString(SR2.TraceCodeSyndicationReadCategoriesDocumentBegin)); } } internal static void TraceCategoriesDocumentReadEnd() { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.DiagnosticTrace.TraceEvent(TraceEventType.Information, TraceCode.SyndicationReadCategoriesDocumentEnd, SR2.GetString(SR2.TraceCodeSyndicationReadCategoriesDocumentEnd)); } } internal static void TraceCategoriesDocumentWriteBegin() { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.DiagnosticTrace.TraceEvent(TraceEventType.Information, TraceCode.SyndicationWriteCategoriesDocumentBegin, SR2.GetString(SR2.TraceCodeSyndicationWriteCategoriesDocumentBegin)); } } internal static void TraceCategoriesDocumentWriteEnd() { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.DiagnosticTrace.TraceEvent(TraceEventType.Information, TraceCode.SyndicationWriteCategoriesDocumentEnd, SR2.GetString(SR2.TraceCodeSyndicationWriteCategoriesDocumentEnd)); } } protected override InlineCategoriesDocument CreateInlineCategoriesDocument() { if (inlineDocumentType == typeof(InlineCategoriesDocument)) { return new InlineCategoriesDocument(); } else { return (InlineCategoriesDocument) Activator.CreateInstance(this.inlineDocumentType); } } protected override ReferencedCategoriesDocument CreateReferencedCategoriesDocument() { if (referencedDocumentType == typeof(ReferencedCategoriesDocument)) { return new ReferencedCategoriesDocument(); } else { return (ReferencedCategoriesDocument) Activator.CreateInstance(this.referencedDocumentType); } } void ReadDocument(XmlReader reader) { try { SyndicationFeedFormatter.MoveToStartElement(reader); SetDocument(AtomPub10ServiceDocumentFormatter.ReadCategories(reader, null, delegate() { return this.CreateInlineCategoriesDocument(); } , delegate() { return this.CreateReferencedCategoriesDocument(); }, this.Version, this.preserveElementExtensions, this.preserveAttributeExtensions, this.maxExtensionSize)); } catch (FormatException e) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(FeedUtils.AddLineInfo(reader, SR2.ErrorParsingDocument), e)); } catch (ArgumentException e) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(FeedUtils.AddLineInfo(reader, SR2.ErrorParsingDocument), e)); } } void WriteDocument(XmlWriter writer) { // declare the atom10 namespace upfront for compactness writer.WriteAttributeString(Atom10Constants.Atom10Prefix, Atom10FeedFormatter.XmlNsNs, Atom10Constants.Atom10Namespace); AtomPub10ServiceDocumentFormatter.WriteCategoriesInnerXml(writer, this.Document, null, this.Version); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
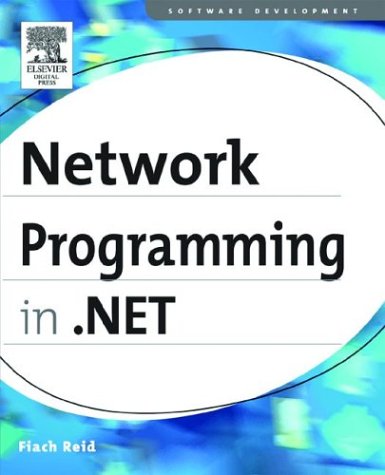
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventMappingSettingsCollection.cs
- RedirectionProxy.cs
- Animatable.cs
- DecoderNLS.cs
- Lasso.cs
- WebHttpSecurityModeHelper.cs
- InputScopeManager.cs
- ICspAsymmetricAlgorithm.cs
- Stackframe.cs
- SpeakCompletedEventArgs.cs
- CallContext.cs
- DependencyObject.cs
- ResourcesGenerator.cs
- RecordConverter.cs
- ServiceProviders.cs
- WebServiceErrorEvent.cs
- FileDialog_Vista.cs
- clipboard.cs
- FormViewInsertedEventArgs.cs
- DaylightTime.cs
- BaseDataBoundControl.cs
- SslSecurityTokenParameters.cs
- NavigationExpr.cs
- CFStream.cs
- DataServiceQueryException.cs
- Dump.cs
- ScriptReference.cs
- RuntimeComponentFilter.cs
- XmlDictionaryReaderQuotas.cs
- ByteRangeDownloader.cs
- WebPartConnectionsCancelVerb.cs
- NamespaceQuery.cs
- __Filters.cs
- EventProviderWriter.cs
- Quaternion.cs
- ContractListAdapter.cs
- DataMemberConverter.cs
- XPathDocumentBuilder.cs
- FontCollection.cs
- AdjustableArrowCap.cs
- WebPartChrome.cs
- CompositeDataBoundControl.cs
- BitmapSourceSafeMILHandle.cs
- DataControlCommands.cs
- OdbcException.cs
- PolicyManager.cs
- WindowsListViewItem.cs
- CodeAccessSecurityEngine.cs
- ServiceModelReg.cs
- SystemException.cs
- IsolatedStoragePermission.cs
- BindingCompleteEventArgs.cs
- _ConnectionGroup.cs
- ParserExtension.cs
- ImageDesigner.cs
- PassportAuthenticationEventArgs.cs
- PopupEventArgs.cs
- ToolStripPanel.cs
- DataColumnSelectionConverter.cs
- HttpResponseInternalWrapper.cs
- HttpListenerRequestUriBuilder.cs
- SourceChangedEventArgs.cs
- BasePattern.cs
- PersonalizationEntry.cs
- Int64KeyFrameCollection.cs
- AssemblyInfo.cs
- EmbeddedObject.cs
- SqlDelegatedTransaction.cs
- ClientSettingsStore.cs
- FlowDocumentView.cs
- DecoderNLS.cs
- TriggerActionCollection.cs
- RunWorkerCompletedEventArgs.cs
- JavascriptCallbackBehaviorAttribute.cs
- Typeface.cs
- SafeBitVector32.cs
- BamlCollectionHolder.cs
- TreeNodeCollection.cs
- ViewKeyConstraint.cs
- TypeToArgumentTypeConverter.cs
- ResourcePermissionBaseEntry.cs
- Size.cs
- ImageMap.cs
- XmlSchemas.cs
- PrtCap_Reader.cs
- EventProxy.cs
- DateTimeValueSerializerContext.cs
- PreProcessInputEventArgs.cs
- DisableDpiAwarenessAttribute.cs
- EmptyEnumerable.cs
- EdmProviderManifest.cs
- SingleObjectCollection.cs
- DataGridClipboardCellContent.cs
- ConfigXmlWhitespace.cs
- Processor.cs
- MbpInfo.cs
- DataGridViewTopLeftHeaderCell.cs
- XamlDesignerSerializationManager.cs
- CodeMemberField.cs
- Main.cs