Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / PrintConfig / PrtCap_Reader.cs / 1 / PrtCap_Reader.cs
/*++ Copyright (C) 2003 Microsoft Corporation All rights reserved. Module Name: PrtCap_Reader.cs Abstract: Definition and implementation of the private XmlPrintCapReader class. Author: [....] ([....]) 06/22/2003 --*/ using System; using System.Xml; using System.IO; using System.Diagnostics; using System.Globalization; using System.Printing; using MS.Internal.Printing.Configuration; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages namespace MS.Internal.Printing.Configuration { ////// Reader class of XML PrintCapabilities /// internal class XmlPrintCapReader { #region Constructors ////// Instantiates a reader object for the given XML PrintCapabilities /// ///Constructor verifies the root element is valid ///thrown if XML PrintCapabilities is not well-formed public XmlPrintCapReader(Stream xmlStream) { // Internally the XML PrintCapabilities reader uses XmlTextReader _xmlReader = new XmlTextReader(xmlStream); // We need namespace support from the reader. _xmlReader.Namespaces = true; // Don't resolve external resources. _xmlReader.XmlResolver = null; // Verify root element isin our standard namespace if ((_xmlReader.MoveToContent() != XmlNodeType.Element) || (_xmlReader.LocalName != PrintSchemaTags.Framework.PrintCapRoot) || (_xmlReader.NamespaceURI != PrintSchemaNamespaces.Framework)) { throw NewPrintCapFormatException(String.Format(CultureInfo.CurrentCulture, PTUtility.GetTextFromResource("FormatException.InvalidRootElement"), _xmlReader.NamespaceURI, _xmlReader.LocalName)); } // Verify the XML PrintCapabilities version is supported // For XML attribute without a prefix (e.g. <... name="prn:PageMediaSize">), // even though the XML document has default namespace defined as our standard // Print Schema framework namespace, the XML atribute still has NULL namespaceURI. // It will only have the correct namespaceURI when a prefix is used. This doesn't // apply to XML element, whose namespaceURI works fine with default namespace. // GetAttribute doesn't move the reader cursor away from the current element string version = _xmlReader.GetAttribute(PrintSchemaTags.Framework.RootVersionAttr, PrintSchemaNamespaces.FrameworkAttrForXmlReader); if (version == null) { throw NewPrintCapFormatException(String.Format(CultureInfo.CurrentCulture, PTUtility.GetTextFromResource("FormatException.RootMissingAttribute"), PrintSchemaTags.Framework.RootVersionAttr)); } // Convert string to number to verify decimal versionNum; try { versionNum = XmlConvertHelper.ConvertStringToDecimal(version); } catch (FormatException e) { throw NewPrintCapFormatException(String.Format(CultureInfo.CurrentCulture, PTUtility.GetTextFromResource("FormatException.RootInvalidAttribute"), PrintSchemaTags.Framework.RootVersionAttr, version), e); } if (versionNum != PrintSchemaTags.Framework.SchemaVersion) { throw NewPrintCapFormatException(String.Format(CultureInfo.CurrentCulture, PTUtility.GetTextFromResource("FormatException.VersionNotSupported"), versionNum)); } // Reset internal states to be ready for client's reading of the PrintCapabilities XML ResetCurrentElementState(); } #endregion Constructors #region Public Methods /// /// Moves the reader cursor to the next Print Schema Framework element at the given depth. /// (The element could be Feature, ParameterDefinition, Option, ScoredProperty or Property) /// /// client-requested traversing depth /// flags to indicate client interested node types ///True if next Framework element is ready to read. /// False if no more Framework element at the given depth. ///XML is not well-formed. public bool MoveToNextSchemaElement(int depth, PrintSchemaNodeTypes typeFilterFlags) { bool foundElement = false; while (!foundElement && _xmlReader.Read()) { // Read() throws XmlException if error occurred while parsing the XML. // If we hit an end-element tag at higher depth, we know there are no more // Framework elements at the client-requested depth. if ((_xmlReader.NodeType == XmlNodeType.EndElement) && (_xmlReader.Depth < depth)) { break; } // Stop at the next XML start element at the client-requested depth // and in the standard Framework element namespace. if ((_xmlReader.NodeType != XmlNodeType.Element) || (_xmlReader.Depth != depth) || (_xmlReader.NamespaceURI != PrintSchemaNamespaces.Framework)) { continue; } // Find a candidate, so reset internal states to be ready for its parsing. ResetCurrentElementState(); foundElement = true; _currentElementDepth = depth; _currentElementIsEmpty = _xmlReader.IsEmptyElement; // Map element name to Schema node type int enumValue = PrintSchemaMapper.SchemaNameToEnumValueWithMap( PrintSchemaTags.Framework.NodeTypeMapTable, _xmlReader.LocalName); if (enumValue > 0) { _currentElementNodeType = (PrintSchemaNodeTypes)enumValue; } else { #if _DEBUG Trace.WriteLine("-Warning- skip unknown element '" + _xmlReader.LocalName + "' at line " + _xmlReader.LineNumber + ", position " + _xmlReader.LinePosition); #endif foundElement = false; } if (foundElement) { // Check whether or not the found element type is what client is interested in. // If not, we will skip this element. if ((CurrentElementNodeType & typeFilterFlags) == 0) { #if _DEBUG Trace.WriteLine("-Warning- skip not-wanted element '" + _xmlReader.LocalName + "' at line " + _xmlReader.LineNumber + ", position " + _xmlReader.LinePosition); #endif foundElement = false; } } if (foundElement) { // The element is what the client wants. if (CurrentElementNodeType != PrintSchemaNodeTypes.Value) { // Element other thanshould have the "name" XML attribute. // Reader will verify the "name" XML attribute has a QName value that // is in our standard Keyword namespace. string QName = _xmlReader.GetAttribute(PrintSchemaTags.Framework.NameAttr, PrintSchemaNamespaces.FrameworkAttrForXmlReader); // Only
Link Menu
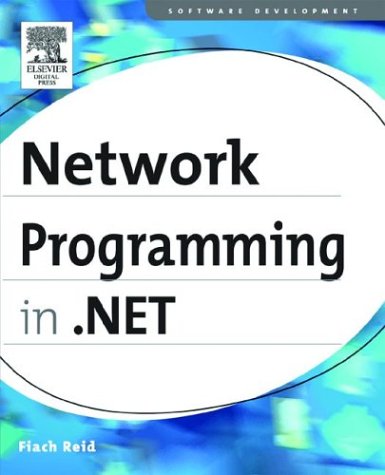
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MulticastDelegate.cs
- ColorInterpolationModeValidation.cs
- DataGridHelper.cs
- ThicknessAnimationBase.cs
- BitmapData.cs
- DecoderNLS.cs
- UniqueID.cs
- EventEntry.cs
- SecurityException.cs
- EntitySqlQueryBuilder.cs
- IISUnsafeMethods.cs
- SessionSwitchEventArgs.cs
- ViewgenGatekeeper.cs
- LogicalExpr.cs
- SendActivity.cs
- SharedPersonalizationStateInfo.cs
- Enum.cs
- URL.cs
- RemoteCryptoRsaServiceProvider.cs
- TextRangeProviderWrapper.cs
- MessageBox.cs
- Matrix3D.cs
- BufferBuilder.cs
- xml.cs
- MultiView.cs
- DiscoveryDocumentSerializer.cs
- Viewport2DVisual3D.cs
- AlternateViewCollection.cs
- SvcMapFileSerializer.cs
- EpmSourceTree.cs
- DataStorage.cs
- jithelpers.cs
- MailWebEventProvider.cs
- IdentityValidationException.cs
- UrlMappingsSection.cs
- SerializationAttributes.cs
- PasswordRecovery.cs
- TemplateBindingExpressionConverter.cs
- PropertyRef.cs
- TraceHandler.cs
- TextEditorContextMenu.cs
- MethodAccessException.cs
- RTLAwareMessageBox.cs
- WsdlWriter.cs
- RectAnimation.cs
- GestureRecognizer.cs
- CharacterHit.cs
- SafeArchiveContext.cs
- UriTemplateLiteralQueryValue.cs
- ListGeneralPage.cs
- HandlerMappingMemo.cs
- PeerToPeerException.cs
- ExpressionEditorAttribute.cs
- AutomationPatternInfo.cs
- SafeNativeMethods.cs
- Missing.cs
- HebrewCalendar.cs
- DataListItemCollection.cs
- TextPattern.cs
- KeyValueInternalCollection.cs
- XmlSchemas.cs
- ColumnMapVisitor.cs
- NativeMethods.cs
- SafeReversePInvokeHandle.cs
- DataGridColumnCollection.cs
- TableStyle.cs
- ParameterToken.cs
- TreeNodeEventArgs.cs
- ClientTarget.cs
- MaterializeFromAtom.cs
- TranslateTransform.cs
- HttpApplication.cs
- ClientTargetSection.cs
- LeaseManager.cs
- DocumentGridContextMenu.cs
- XmlObjectSerializerContext.cs
- CalendarDay.cs
- HostedAspNetEnvironment.cs
- CellCreator.cs
- BrowserDefinition.cs
- ValidatedControlConverter.cs
- MatrixAnimationUsingKeyFrames.cs
- OracleInternalConnection.cs
- WmiInstallComponent.cs
- FunctionMappingTranslator.cs
- RegistrySecurity.cs
- RadioButtonFlatAdapter.cs
- HtmlString.cs
- TransformedBitmap.cs
- InitializationEventAttribute.cs
- COM2IDispatchConverter.cs
- WindowsToolbar.cs
- _AutoWebProxyScriptEngine.cs
- ButtonBaseAdapter.cs
- JapaneseLunisolarCalendar.cs
- SamlSubjectStatement.cs
- ColorDialog.cs
- LicenseManager.cs
- HTMLTextWriter.cs
- NamedPipeAppDomainProtocolHandler.cs