Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / ComponentModel / Design / HelpKeywordAttribute.cs / 1305376 / HelpKeywordAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel.Design { using System; using System.Security.Permissions; ////// Allows specification of the context keyword that will be specified for this class or member. By default, /// the help keyword for a class is the Type's full name, and for a member it's the full name of the type that declared the property, /// plus the property name itself. /// /// For example, consider System.Windows.Forms.Button and it's Text property: /// /// The class keyword is "System.Windows.Forms.Button", but the Text property keyword is "System.Windows.Forms.Control.Text", because the Text /// property is declared on the System.Windows.Forms.Control class rather than the Button class itself; the Button class inherits the property. /// By contrast, the DialogResult property is declared on the Button so its keyword would be "System.Windows.Forms.Button.DialogResult". /// /// When the help system gets the keywords, it will first look at this attribute. At the class level, it will return the string specified by the /// HelpContextAttribute. Note this will not be used for members of the Type in question. They will still reflect the declaring Type's actual /// full name, plus the member name. To override this, place the attribute on the member itself. /// /// Example: /// /// [HelpKeywordAttribute(typeof(Component))] /// public class MyComponent : Component { /// /// /// public string Property1 { get{return "";}; /// /// [HelpKeywordAttribute("SomeNamespace.SomeOtherClass.Property2")] /// public string Property2 { get{return "";}; /// /// } /// /// /// For the above class (default without attribution): /// /// Class keyword: "System.ComponentModel.Component" ("MyNamespace.MyComponent') /// Property1 keyword: "MyNamespace.MyComponent.Property1" (default) /// Property2 keyword: "SomeNamespace.SomeOtherClass.Property2" ("MyNamespace.MyComponent.Property2") /// /// [AttributeUsage(AttributeTargets.All, AllowMultiple = false, Inherited = false)] [Serializable] public sealed class HelpKeywordAttribute : Attribute { ////// Default value for HelpKeywordAttribute, which is null. /// public static readonly HelpKeywordAttribute Default = new HelpKeywordAttribute(); private string contextKeyword; ////// Default constructor, which creates an attribute with a null HelpKeyword. /// public HelpKeywordAttribute() { } ////// Creates a HelpKeywordAttribute with the value being the given keyword string. /// public HelpKeywordAttribute(string keyword) { if (keyword == null) { throw new ArgumentNullException("keyword"); } this.contextKeyword = keyword; } ////// Creates a HelpKeywordAttribute with the value being the full name of the given type. /// public HelpKeywordAttribute(Type t) { if (t == null) { throw new ArgumentNullException("t"); } this.contextKeyword = t.FullName; } ////// Retrieves the HelpKeyword this attribute supplies. /// public string HelpKeyword { get { return contextKeyword; } } ////// Two instances of a HelpKeywordAttribute are equal if they're HelpKeywords are equal. /// public override bool Equals(object obj) { if (obj == this) { return true; } if ((obj != null) && (obj is HelpKeywordAttribute)) { return ((HelpKeywordAttribute)obj).HelpKeyword == HelpKeyword; } return false; } ////// public override int GetHashCode() { return base.GetHashCode(); } ////// Returns true if this Attribute's HelpKeyword is null. /// public override bool IsDefaultAttribute() { return this.Equals(Default); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel.Design { using System; using System.Security.Permissions; ////// Allows specification of the context keyword that will be specified for this class or member. By default, /// the help keyword for a class is the Type's full name, and for a member it's the full name of the type that declared the property, /// plus the property name itself. /// /// For example, consider System.Windows.Forms.Button and it's Text property: /// /// The class keyword is "System.Windows.Forms.Button", but the Text property keyword is "System.Windows.Forms.Control.Text", because the Text /// property is declared on the System.Windows.Forms.Control class rather than the Button class itself; the Button class inherits the property. /// By contrast, the DialogResult property is declared on the Button so its keyword would be "System.Windows.Forms.Button.DialogResult". /// /// When the help system gets the keywords, it will first look at this attribute. At the class level, it will return the string specified by the /// HelpContextAttribute. Note this will not be used for members of the Type in question. They will still reflect the declaring Type's actual /// full name, plus the member name. To override this, place the attribute on the member itself. /// /// Example: /// /// [HelpKeywordAttribute(typeof(Component))] /// public class MyComponent : Component { /// /// /// public string Property1 { get{return "";}; /// /// [HelpKeywordAttribute("SomeNamespace.SomeOtherClass.Property2")] /// public string Property2 { get{return "";}; /// /// } /// /// /// For the above class (default without attribution): /// /// Class keyword: "System.ComponentModel.Component" ("MyNamespace.MyComponent') /// Property1 keyword: "MyNamespace.MyComponent.Property1" (default) /// Property2 keyword: "SomeNamespace.SomeOtherClass.Property2" ("MyNamespace.MyComponent.Property2") /// /// [AttributeUsage(AttributeTargets.All, AllowMultiple = false, Inherited = false)] [Serializable] public sealed class HelpKeywordAttribute : Attribute { ////// Default value for HelpKeywordAttribute, which is null. /// public static readonly HelpKeywordAttribute Default = new HelpKeywordAttribute(); private string contextKeyword; ////// Default constructor, which creates an attribute with a null HelpKeyword. /// public HelpKeywordAttribute() { } ////// Creates a HelpKeywordAttribute with the value being the given keyword string. /// public HelpKeywordAttribute(string keyword) { if (keyword == null) { throw new ArgumentNullException("keyword"); } this.contextKeyword = keyword; } ////// Creates a HelpKeywordAttribute with the value being the full name of the given type. /// public HelpKeywordAttribute(Type t) { if (t == null) { throw new ArgumentNullException("t"); } this.contextKeyword = t.FullName; } ////// Retrieves the HelpKeyword this attribute supplies. /// public string HelpKeyword { get { return contextKeyword; } } ////// Two instances of a HelpKeywordAttribute are equal if they're HelpKeywords are equal. /// public override bool Equals(object obj) { if (obj == this) { return true; } if ((obj != null) && (obj is HelpKeywordAttribute)) { return ((HelpKeywordAttribute)obj).HelpKeyword == HelpKeyword; } return false; } ////// public override int GetHashCode() { return base.GetHashCode(); } ////// Returns true if this Attribute's HelpKeyword is null. /// public override bool IsDefaultAttribute() { return this.Equals(Default); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
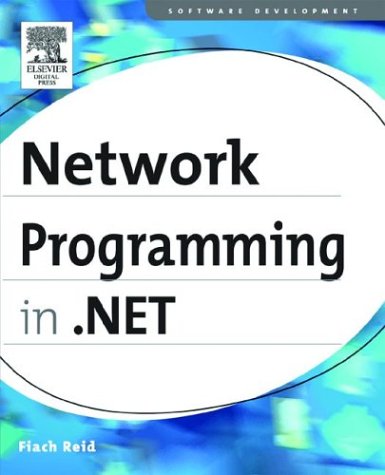
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AsymmetricSignatureFormatter.cs
- SmtpTransport.cs
- x509store.cs
- SQLSingle.cs
- DiscoveryClientRequestChannel.cs
- updateconfighost.cs
- BooleanSwitch.cs
- FontWeight.cs
- RowVisual.cs
- IdleTimeoutMonitor.cs
- UserValidatedEventArgs.cs
- PerformanceCounterPermissionAttribute.cs
- TemplateXamlTreeBuilder.cs
- IntegerFacetDescriptionElement.cs
- UidManager.cs
- ElapsedEventArgs.cs
- PhysicalFontFamily.cs
- RegexCharClass.cs
- ConfigXmlReader.cs
- TableHeaderCell.cs
- ListenerElementsCollection.cs
- ActivityTypeDesigner.xaml.cs
- ItemList.cs
- AttachedPropertyMethodSelector.cs
- OpCellTreeNode.cs
- BitStack.cs
- SR.cs
- WSHttpBindingCollectionElement.cs
- EncoderBestFitFallback.cs
- SerializationUtility.cs
- XPathEmptyIterator.cs
- NegotiateStream.cs
- GlyphTypeface.cs
- SynchronizationContext.cs
- Signature.cs
- PieceDirectory.cs
- ToolStripDropDownItem.cs
- SystemColors.cs
- InputEventArgs.cs
- DirectionalLight.cs
- TraceInternal.cs
- XmlElementList.cs
- SelectionItemProviderWrapper.cs
- HandleExceptionArgs.cs
- HwndSourceParameters.cs
- GridViewDeletedEventArgs.cs
- ForeignConstraint.cs
- Matrix3DConverter.cs
- FunctionQuery.cs
- BindingCompleteEventArgs.cs
- PreviewKeyDownEventArgs.cs
- ContainerControl.cs
- ScriptManager.cs
- IteratorFilter.cs
- PathFigureCollectionConverter.cs
- DataGridViewCellStateChangedEventArgs.cs
- SchemaImporterExtensionsSection.cs
- SimpleType.cs
- dbenumerator.cs
- OleDbCommand.cs
- ColumnHeader.cs
- DockPatternIdentifiers.cs
- StringResourceManager.cs
- RootBuilder.cs
- SessionIDManager.cs
- FileRecordSequenceCompletedAsyncResult.cs
- MonthChangedEventArgs.cs
- DragDeltaEventArgs.cs
- Point3D.cs
- IsolatedStoragePermission.cs
- SupportsEventValidationAttribute.cs
- TraceHwndHost.cs
- ObjectSet.cs
- StylusEditingBehavior.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- ObjectTag.cs
- ValidatedControlConverter.cs
- RoutedPropertyChangedEventArgs.cs
- XmlCodeExporter.cs
- TextServicesCompartmentEventSink.cs
- PriorityQueue.cs
- IApplicationTrustManager.cs
- HtmlObjectListAdapter.cs
- FixedDSBuilder.cs
- RootCodeDomSerializer.cs
- DataShape.cs
- StandardToolWindows.cs
- AnnotationAdorner.cs
- UInt16Storage.cs
- ToolStripPanelRenderEventArgs.cs
- Parser.cs
- WebPartMinimizeVerb.cs
- BindingValueChangedEventArgs.cs
- ObjRef.cs
- DocumentPageView.cs
- BitmapEffectOutputConnector.cs
- LayoutInformation.cs
- TextEditorSpelling.cs
- ApplicationActivator.cs
- ToolStripSeparatorRenderEventArgs.cs