Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataWebControls / System / Data / WebControls / EntityDataSourceSelectedEventArgs.cs / 1 / EntityDataSourceSelectedEventArgs.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner objsdev //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Data.Objects; using System.Collections; namespace System.Web.UI.WebControls { public class EntityDataSourceSelectedEventArgs : EventArgs { private readonly ObjectContext _context; private readonly Exception _exception = null; private bool _exceptionHandled = false; private readonly IEnumerable _results = null; private readonly int _totalRowCount = 0; private readonly DataSourceSelectArguments _selectArguments; internal EntityDataSourceSelectedEventArgs(ObjectContext context, IEnumerable results, int totalRowCount, DataSourceSelectArguments selectArgs) { _context = context; _results = results; _totalRowCount = totalRowCount; _selectArguments = selectArgs; } internal EntityDataSourceSelectedEventArgs(Exception exception) { _exception = exception; } public Exception Exception { get { return _exception; } } public bool ExceptionHandled { get { return _exceptionHandled; } set { _exceptionHandled = value; } } public IEnumerable Results { get { return _results; } } public ObjectContext Context { get { return _context; } } public int TotalRowCount { get { return _totalRowCount; } } public DataSourceSelectArguments SelectArguments { get { return _selectArguments; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner objsdev //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Data.Objects; using System.Collections; namespace System.Web.UI.WebControls { public class EntityDataSourceSelectedEventArgs : EventArgs { private readonly ObjectContext _context; private readonly Exception _exception = null; private bool _exceptionHandled = false; private readonly IEnumerable _results = null; private readonly int _totalRowCount = 0; private readonly DataSourceSelectArguments _selectArguments; internal EntityDataSourceSelectedEventArgs(ObjectContext context, IEnumerable results, int totalRowCount, DataSourceSelectArguments selectArgs) { _context = context; _results = results; _totalRowCount = totalRowCount; _selectArguments = selectArgs; } internal EntityDataSourceSelectedEventArgs(Exception exception) { _exception = exception; } public Exception Exception { get { return _exception; } } public bool ExceptionHandled { get { return _exceptionHandled; } set { _exceptionHandled = value; } } public IEnumerable Results { get { return _results; } } public ObjectContext Context { get { return _context; } } public int TotalRowCount { get { return _totalRowCount; } } public DataSourceSelectArguments SelectArguments { get { return _selectArguments; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
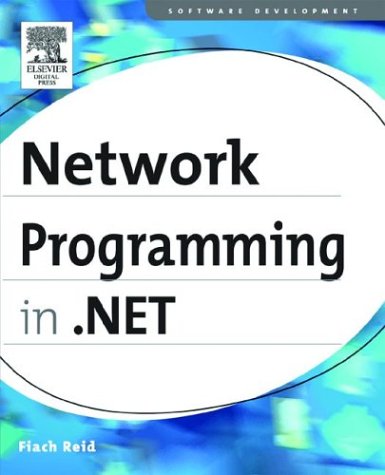
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReflectionPermission.cs
- NumericExpr.cs
- MetafileHeader.cs
- GroupDescription.cs
- Material.cs
- RuntimeConfig.cs
- Span.cs
- SchemaNotation.cs
- RSAOAEPKeyExchangeFormatter.cs
- BufferedStream2.cs
- ButtonDesigner.cs
- TextEndOfParagraph.cs
- AtlasWeb.Designer.cs
- ScriptingAuthenticationServiceSection.cs
- BorderGapMaskConverter.cs
- CodeLinePragma.cs
- DataGrid.cs
- AttachedAnnotationChangedEventArgs.cs
- SynchronizingStream.cs
- QilValidationVisitor.cs
- XsdDateTime.cs
- XmlNamedNodeMap.cs
- ExpressionBuilder.cs
- LingerOption.cs
- CompiledQuery.cs
- ToolBarTray.cs
- DescendantBaseQuery.cs
- Convert.cs
- App.cs
- COM2ExtendedTypeConverter.cs
- BaseTemplateBuildProvider.cs
- QilFunction.cs
- HyperLinkStyle.cs
- EntityDataSourceWizardForm.cs
- LocatorManager.cs
- LayoutTable.cs
- IPEndPointCollection.cs
- GeneralTransformCollection.cs
- ManualResetEvent.cs
- XmlnsCompatibleWithAttribute.cs
- ImageUrlEditor.cs
- ControllableStoryboardAction.cs
- DesignerEventService.cs
- Help.cs
- StrongNameUtility.cs
- ExpressionVisitor.cs
- EntityClientCacheKey.cs
- IndentedWriter.cs
- SrgsRuleRef.cs
- SmiXetterAccessMap.cs
- TextEditorCharacters.cs
- BaseHashHelper.cs
- UriScheme.cs
- TypeDescriptionProviderAttribute.cs
- ConfigurationProperty.cs
- DbCommandDefinition.cs
- ConnectionsZone.cs
- CommandDesigner.cs
- ObjectDataSourceDisposingEventArgs.cs
- RequiredAttributeAttribute.cs
- PointAnimationBase.cs
- SqlDataSourceStatusEventArgs.cs
- MarginsConverter.cs
- ImageClickEventArgs.cs
- BackgroundWorker.cs
- OSFeature.cs
- FilePrompt.cs
- Double.cs
- DataColumn.cs
- HtmlContainerControl.cs
- SelectedDatesCollection.cs
- ListBoxItemWrapperAutomationPeer.cs
- FileLoadException.cs
- NativeMethods.cs
- CfgRule.cs
- DataBindingsDialog.cs
- UpdateInfo.cs
- NullableIntSumAggregationOperator.cs
- RealProxy.cs
- Avt.cs
- ModelUtilities.cs
- oledbconnectionstring.cs
- BinHexDecoder.cs
- InfiniteTimeSpanConverter.cs
- SapiRecoContext.cs
- HtmlElementEventArgs.cs
- Border.cs
- WindowsProgressbar.cs
- PageSetupDialog.cs
- lengthconverter.cs
- HuffModule.cs
- FramingEncoders.cs
- DataRelationPropertyDescriptor.cs
- SynchronizingStream.cs
- WmpBitmapDecoder.cs
- DebugView.cs
- PersonalizationProviderHelper.cs
- DebugInfoGenerator.cs
- StylusPoint.cs
- StrongNameMembershipCondition.cs