Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Security / Cryptography / RSAOAEPKeyExchangeFormatter.cs / 1 / RSAOAEPKeyExchangeFormatter.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System.Security.Cryptography { [System.Runtime.InteropServices.ComVisible(true)] public class RSAOAEPKeyExchangeFormatter : AsymmetricKeyExchangeFormatter { private byte[] ParameterValue; private RSA _rsaKey; private RandomNumberGenerator RngValue; // // public constructors // public RSAOAEPKeyExchangeFormatter() {} public RSAOAEPKeyExchangeFormatter(AsymmetricAlgorithm key) { if (key == null) throw new ArgumentNullException("key"); _rsaKey = (RSA) key; } // // public properties // ///public byte[] Parameter { get { if (ParameterValue != null) return (byte[]) ParameterValue.Clone(); return null; } set { if (value != null) ParameterValue = (byte[]) value.Clone(); else ParameterValue = null; } } /// public override String Parameters { get { return null; } } public RandomNumberGenerator Rng { get { return RngValue; } set { RngValue = value; } } // // public methods // public override void SetKey(AsymmetricAlgorithm key) { if (key == null) throw new ArgumentNullException("key"); _rsaKey = (RSA) key; } public override byte[] CreateKeyExchange(byte[] rgbData) { if (_rsaKey == null) throw new CryptographicUnexpectedOperationException(Environment.GetResourceString("Cryptography_MissingKey")); if (_rsaKey is RSACryptoServiceProvider) { return ((RSACryptoServiceProvider) _rsaKey).Encrypt(rgbData, true); } else { return Utils.RsaOaepEncrypt(_rsaKey, SHA1.Create(), new PKCS1MaskGenerationMethod(), RandomNumberGenerator.Create(), rgbData); } } public override byte[] CreateKeyExchange(byte[] rgbData, Type symAlgType) { return CreateKeyExchange(rgbData); } } }
Link Menu
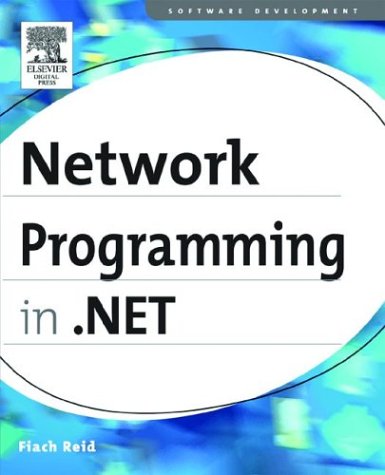
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OleDbPermission.cs
- InputLanguageCollection.cs
- NameValuePermission.cs
- Trace.cs
- DataGridCommandEventArgs.cs
- DriveInfo.cs
- ProcessThreadCollection.cs
- DigitShape.cs
- GroupQuery.cs
- BamlRecordReader.cs
- XmlCollation.cs
- ActionItem.cs
- SecurityHelper.cs
- Function.cs
- AbstractSvcMapFileLoader.cs
- HttpCookie.cs
- AppDomainShutdownMonitor.cs
- ConvertersCollection.cs
- ArraySet.cs
- _ChunkParse.cs
- Scripts.cs
- ServiceNameElementCollection.cs
- EntityKey.cs
- MemberInfoSerializationHolder.cs
- Certificate.cs
- PolicyAssertionCollection.cs
- ComponentCodeDomSerializer.cs
- DataTable.cs
- ComplusEndpointConfigContainer.cs
- InputEventArgs.cs
- hresults.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- HttpHostedTransportConfiguration.cs
- SqlServices.cs
- RuntimeUtils.cs
- Point4D.cs
- HostedHttpTransportManager.cs
- DateTimeFormat.cs
- ManageRequest.cs
- IntegerValidator.cs
- CodeMethodMap.cs
- Triangle.cs
- PackageFilter.cs
- ControllableStoryboardAction.cs
- NamespaceDisplay.xaml.cs
- DesignerOptionService.cs
- WebScriptClientGenerator.cs
- ValidationSummary.cs
- ProfileSettings.cs
- PolicyUnit.cs
- RuleConditionDialog.cs
- WebPartTransformer.cs
- BuildProviderAppliesToAttribute.cs
- Internal.cs
- MatrixCamera.cs
- DefaultTextStore.cs
- SimpleRecyclingCache.cs
- FileStream.cs
- OutputScopeManager.cs
- DataGridViewCellLinkedList.cs
- CopyOfAction.cs
- DiscoveryClientDuplexChannel.cs
- DetailsViewRowCollection.cs
- IntegerValidator.cs
- ModelPropertyImpl.cs
- HtmlContainerControl.cs
- RemoteWebConfigurationHostServer.cs
- DynamicActionMessageFilter.cs
- MexServiceChannelBuilder.cs
- EncoderFallback.cs
- RijndaelManagedTransform.cs
- Scene3D.cs
- Sql8ExpressionRewriter.cs
- SchemaElementDecl.cs
- CellParagraph.cs
- LinearGradientBrush.cs
- AutomationTextAttribute.cs
- SiteOfOriginPart.cs
- FieldNameLookup.cs
- Monitor.cs
- XmlDictionaryReaderQuotasElement.cs
- OdbcError.cs
- ToolStripPanelCell.cs
- NotificationContext.cs
- Expander.cs
- ChangesetResponse.cs
- XmlImplementation.cs
- DocumentPageHost.cs
- Control.cs
- XmlWrappingReader.cs
- DataPagerFieldCollection.cs
- HostingEnvironment.cs
- SqlTypesSchemaImporter.cs
- ActivityExecutor.cs
- EdmScalarPropertyAttribute.cs
- NativeObjectSecurity.cs
- XPathDocument.cs
- AxisAngleRotation3D.cs
- PageRequestManager.cs
- CodeIndexerExpression.cs