Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / ComponentModel / ListChangedEventArgs.cs / 1305376 / ListChangedEventArgs.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- //can not fix - Everett breaking change [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Scope="member", Target="System.ComponentModel.ListChangedEventArgs..ctor(System.ComponentModel.ListChangedType,System.Int32,System.ComponentModel.PropertyDescriptor)")] [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Scope="member", Target="System.ComponentModel.ListChangedEventArgs..ctor(System.ComponentModel.ListChangedType,System.ComponentModel.PropertyDescriptor)")] namespace System.ComponentModel { using Microsoft.Win32; using System; using System.Diagnostics; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] public class ListChangedEventArgs : EventArgs { private ListChangedType listChangedType; private int newIndex; private int oldIndex; private PropertyDescriptor propDesc; ///[To be supplied.] ////// public ListChangedEventArgs(ListChangedType listChangedType, int newIndex) : this(listChangedType, newIndex, -1) { } ///[To be supplied.] ////// public ListChangedEventArgs(ListChangedType listChangedType, int newIndex, PropertyDescriptor propDesc) : this(listChangedType, newIndex) { this.propDesc = propDesc; this.oldIndex = newIndex; } ///[To be supplied.] ////// public ListChangedEventArgs(ListChangedType listChangedType, PropertyDescriptor propDesc) { Debug.Assert(listChangedType != ListChangedType.Reset, "this constructor is used only for changes in the list MetaData"); Debug.Assert(listChangedType != ListChangedType.ItemAdded, "this constructor is used only for changes in the list MetaData"); Debug.Assert(listChangedType != ListChangedType.ItemDeleted, "this constructor is used only for changes in the list MetaData"); Debug.Assert(listChangedType != ListChangedType.ItemChanged, "this constructor is used only for changes in the list MetaData"); this.listChangedType = listChangedType; this.propDesc = propDesc; } ///[To be supplied.] ////// public ListChangedEventArgs(ListChangedType listChangedType, int newIndex, int oldIndex) { Debug.Assert(listChangedType != ListChangedType.PropertyDescriptorAdded, "this constructor is used only for item changed in the list"); Debug.Assert(listChangedType != ListChangedType.PropertyDescriptorDeleted, "this constructor is used only for item changed in the list"); Debug.Assert(listChangedType != ListChangedType.PropertyDescriptorChanged, "this constructor is used only for item changed in the list"); this.listChangedType = listChangedType; this.newIndex = newIndex; this.oldIndex = oldIndex; } ///[To be supplied.] ////// public ListChangedType ListChangedType { get { return listChangedType; } } ///[To be supplied.] ////// public int NewIndex { get { return newIndex; } } ///[To be supplied.] ////// public int OldIndex { get { return oldIndex; } } ///[To be supplied.] ////// public PropertyDescriptor PropertyDescriptor { get { return propDesc; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- //can not fix - Everett breaking change [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Scope="member", Target="System.ComponentModel.ListChangedEventArgs..ctor(System.ComponentModel.ListChangedType,System.Int32,System.ComponentModel.PropertyDescriptor)")] [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Scope="member", Target="System.ComponentModel.ListChangedEventArgs..ctor(System.ComponentModel.ListChangedType,System.ComponentModel.PropertyDescriptor)")] namespace System.ComponentModel { using Microsoft.Win32; using System; using System.Diagnostics; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] public class ListChangedEventArgs : EventArgs { private ListChangedType listChangedType; private int newIndex; private int oldIndex; private PropertyDescriptor propDesc; ///[To be supplied.] ////// public ListChangedEventArgs(ListChangedType listChangedType, int newIndex) : this(listChangedType, newIndex, -1) { } ///[To be supplied.] ////// public ListChangedEventArgs(ListChangedType listChangedType, int newIndex, PropertyDescriptor propDesc) : this(listChangedType, newIndex) { this.propDesc = propDesc; this.oldIndex = newIndex; } ///[To be supplied.] ////// public ListChangedEventArgs(ListChangedType listChangedType, PropertyDescriptor propDesc) { Debug.Assert(listChangedType != ListChangedType.Reset, "this constructor is used only for changes in the list MetaData"); Debug.Assert(listChangedType != ListChangedType.ItemAdded, "this constructor is used only for changes in the list MetaData"); Debug.Assert(listChangedType != ListChangedType.ItemDeleted, "this constructor is used only for changes in the list MetaData"); Debug.Assert(listChangedType != ListChangedType.ItemChanged, "this constructor is used only for changes in the list MetaData"); this.listChangedType = listChangedType; this.propDesc = propDesc; } ///[To be supplied.] ////// public ListChangedEventArgs(ListChangedType listChangedType, int newIndex, int oldIndex) { Debug.Assert(listChangedType != ListChangedType.PropertyDescriptorAdded, "this constructor is used only for item changed in the list"); Debug.Assert(listChangedType != ListChangedType.PropertyDescriptorDeleted, "this constructor is used only for item changed in the list"); Debug.Assert(listChangedType != ListChangedType.PropertyDescriptorChanged, "this constructor is used only for item changed in the list"); this.listChangedType = listChangedType; this.newIndex = newIndex; this.oldIndex = oldIndex; } ///[To be supplied.] ////// public ListChangedType ListChangedType { get { return listChangedType; } } ///[To be supplied.] ////// public int NewIndex { get { return newIndex; } } ///[To be supplied.] ////// public int OldIndex { get { return oldIndex; } } ///[To be supplied.] ////// public PropertyDescriptor PropertyDescriptor { get { return propDesc; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
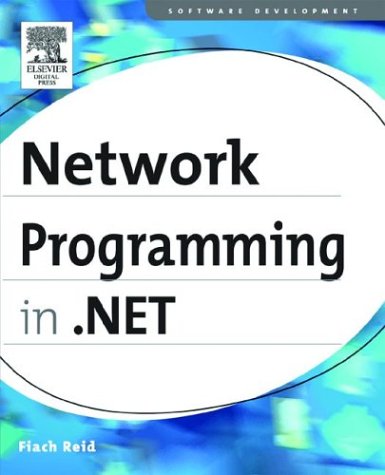
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CopyAction.cs
- SQLInt32Storage.cs
- XmlDataContract.cs
- EntityViewGenerationAttribute.cs
- ImageCodecInfo.cs
- QilFactory.cs
- WebServiceTypeData.cs
- XmlNamespaceMapping.cs
- SerializableAttribute.cs
- VectorValueSerializer.cs
- UriSectionData.cs
- RedBlackList.cs
- EntityConnection.cs
- DetailsViewDeletedEventArgs.cs
- ToolStripMenuItemCodeDomSerializer.cs
- Object.cs
- SQLInt32.cs
- DLinqColumnProvider.cs
- XmlSchemaAnnotated.cs
- SqlAggregateChecker.cs
- ISessionStateStore.cs
- AudioSignalProblemOccurredEventArgs.cs
- ErrorHandler.cs
- BulletDecorator.cs
- WebPartCancelEventArgs.cs
- SoapAttributes.cs
- NavigationEventArgs.cs
- RawTextInputReport.cs
- FrameworkContextData.cs
- FaultCallbackWrapper.cs
- CallContext.cs
- StyleBamlRecordReader.cs
- TextRunCacheImp.cs
- CheckoutException.cs
- DataObjectFieldAttribute.cs
- SamlConditions.cs
- ResourceExpressionBuilder.cs
- XmlKeywords.cs
- GeneralTransform3D.cs
- GenericTextProperties.cs
- TreeViewImageKeyConverter.cs
- CustomError.cs
- HierarchicalDataSourceIDConverter.cs
- Roles.cs
- GridViewColumnHeader.cs
- GraphicsPathIterator.cs
- PolicyException.cs
- Panel.cs
- ManualResetEvent.cs
- _NegoStream.cs
- GPRECT.cs
- TemplatedWizardStep.cs
- SelectionGlyph.cs
- Ipv6Element.cs
- HighContrastHelper.cs
- Context.cs
- Style.cs
- CalendarButton.cs
- UiaCoreApi.cs
- SoapFault.cs
- WebPartConnectionsEventArgs.cs
- TextProperties.cs
- RotateTransform.cs
- XmlSchemaSimpleTypeRestriction.cs
- JobDuplex.cs
- ProfileSection.cs
- GcHandle.cs
- AlignmentXValidation.cs
- MailAddressCollection.cs
- SHA512.cs
- FreezableOperations.cs
- MenuItemAutomationPeer.cs
- OracleConnection.cs
- FieldBuilder.cs
- HashSetDebugView.cs
- InvocationExpression.cs
- DataGridViewCellPaintingEventArgs.cs
- ArrayTypeMismatchException.cs
- EngineSiteSapi.cs
- RegexMatchCollection.cs
- ReferenceEqualityComparer.cs
- ToolBarTray.cs
- RotationValidation.cs
- BindableAttribute.cs
- ReversePositionQuery.cs
- SuppressIldasmAttribute.cs
- DbXmlEnabledProviderManifest.cs
- DecoderNLS.cs
- OleDbSchemaGuid.cs
- HostedHttpTransportManager.cs
- KnownColorTable.cs
- TraceHwndHost.cs
- TreeNodeCollection.cs
- WebPartDesigner.cs
- DbParameterHelper.cs
- SqlParameter.cs
- KeyValuePairs.cs
- StretchValidation.cs
- CreateUserWizardStep.cs
- FormClosedEvent.cs