Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Documents / DocumentPaginator.cs / 1305600 / DocumentPaginator.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: DocumentPaginator.cs // // Description: This is the abstract base class for all paginating layouts. // It provides default implementations for the asynchronous // versions of GetPage and ComputePageCount. // // History: // 08/29/2005 : [....] - created. // //--------------------------------------------------------------------------- using System.ComponentModel; // AsyncCompletedEventArgs using System.Windows.Media; // Visual using MS.Internal.PresentationCore; // SR, SRID namespace System.Windows.Documents { ////// This is the abstract base class for all paginating layouts. It /// provides default implementations for the asynchronous versions of /// GetPage and ComputePageCount. /// public abstract class DocumentPaginator { //------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// Retrieves the DocumentPage for the given page number. PageNumber /// is zero-based. /// /// Page number. ////// Returns DocumentPage.Missing if the given page does not exist. /// ////// Multiple requests for the same page number may return the same /// object (this is implementation specific). /// ////// Throws ArgumentOutOfRangeException if PageNumber is negative. /// public abstract DocumentPage GetPage(int pageNumber); ////// Async version of /// Page number. ////// /// Throws ArgumentOutOfRangeException if PageNumber is negative. /// public virtual void GetPageAsync(int pageNumber) { GetPageAsync(pageNumber, null); } ////// Async version of /// Page number. /// Unique identifier for the asynchronous task. ////// /// Throws ArgumentOutOfRangeException if PageNumber is negative. /// public virtual void GetPageAsync(int pageNumber, object userState) { DocumentPage page; // Page number cannot be negative. if (pageNumber < 0) { throw new ArgumentOutOfRangeException("pageNumber", SR.Get(SRID.PaginatorNegativePageNumber)); } page = GetPage(pageNumber); OnGetPageCompleted(new GetPageCompletedEventArgs(page, pageNumber, null, false, userState)); } ////// Computes the number of pages of content. IsPageCountValid will be /// True immediately after this is called. /// ////// If content is modified or PageSize is changed (or any other change /// that causes a repagination) after this method is called, /// IsPageCountValid will likely revert to False. /// public virtual void ComputePageCount() { // Force pagination of entire content. GetPage(int.MaxValue); } ////// Async version of public virtual void ComputePageCountAsync() { ComputePageCountAsync(null); } ////// /// Async version of /// Unique identifier for the asynchronous task. public virtual void ComputePageCountAsync(object userState) { ComputePageCount(); OnComputePageCountCompleted(new AsyncCompletedEventArgs(null, false, userState)); } ////// /// Cancels all asynchronous calls made with the given userState. /// If userState is NULL, all asynchronous calls are cancelled. /// /// Unique identifier for the asynchronous task. public virtual void CancelAsync(object userState) { } #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// Whether PageCount is currently valid. If False, then the value of /// PageCount is the number of pages that have currently been formatted. /// ////// This value may revert to False after being True, in cases where /// PageSize or content changes, forcing a repagination. /// public abstract bool IsPageCountValid { get; } ////// If IsPageCountValid is True, this value is the number of pages /// of content. If False, this is the number of pages that have /// currently been formatted. /// ////// Value may change depending upon changes in PageSize or content changes. /// public abstract int PageCount { get; } ////// The suggested size for formatting pages. /// ////// Note that the paginator may override the specified page size. Users /// should check DocumentPage.Size. /// public abstract Size PageSize { get; set; } ////// A pointer back to the element being paginated. /// public abstract IDocumentPaginatorSource Source { get; } #endregion Public Properties //-------------------------------------------------------------------- // // Public Events // //-------------------------------------------------------------------- #region Public Events ////// Fired when a GetPageAsync call has completed. /// public event GetPageCompletedEventHandler GetPageCompleted; ////// Fired when a ComputePageCountAsync call has completed. /// public event AsyncCompletedEventHandler ComputePageCountCompleted; ////// Fired when one of the properties of a DocumentPage changes. /// Affected pages must be re-fetched if currently used. /// ////// Existing DocumentPage objects may be destroyed when this /// event is fired. /// public event PagesChangedEventHandler PagesChanged; #endregion Public Events //------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods ////// Override for subclasses that wish to add logic when this event is fired. /// /// Event arguments for the GetPageCompleted event. protected virtual void OnGetPageCompleted(GetPageCompletedEventArgs e) { if (this.GetPageCompleted != null) { this.GetPageCompleted(this, e); } } ////// Override for subclasses that wish to add logic when this event is fired. /// /// Event arguments for the ComputePageCountCompleted event. protected virtual void OnComputePageCountCompleted(AsyncCompletedEventArgs e) { if (this.ComputePageCountCompleted != null) { this.ComputePageCountCompleted(this, e); } } ////// Override for subclasses that wish to add logic when this event is fired. /// /// Event arguments for the PagesChanged event. protected virtual void OnPagesChanged(PagesChangedEventArgs e) { if (this.PagesChanged != null) { this.PagesChanged(this, e); } } #endregion Protected Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
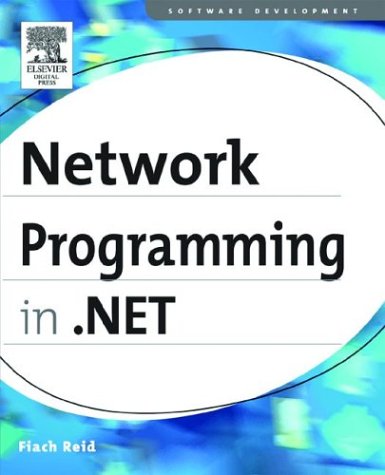
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Viewport3DAutomationPeer.cs
- InheritanceContextHelper.cs
- ColorKeyFrameCollection.cs
- MainMenu.cs
- BinaryMethodMessage.cs
- HtmlControl.cs
- StickyNote.cs
- DataServiceResponse.cs
- DataControlButton.cs
- StateMachineHelpers.cs
- HttpResponseHeader.cs
- DataGridViewHeaderCell.cs
- DataGridViewAutoSizeModeEventArgs.cs
- IListConverters.cs
- OperationAbortedException.cs
- LicFileLicenseProvider.cs
- TabControlAutomationPeer.cs
- Function.cs
- ProcessProtocolHandler.cs
- _SSPIWrapper.cs
- XamlPathDataSerializer.cs
- TextureBrush.cs
- DependencyPropertyKind.cs
- ByteStreamGeometryContext.cs
- ChtmlImageAdapter.cs
- OdbcHandle.cs
- AssemblyCache.cs
- EncoderReplacementFallback.cs
- OutputCacheSettingsSection.cs
- NotSupportedException.cs
- ClientBuildManager.cs
- BinaryFormatter.cs
- TaskSchedulerException.cs
- SchemaManager.cs
- WebBaseEventKeyComparer.cs
- CodeVariableReferenceExpression.cs
- OleDbRowUpdatingEvent.cs
- DebuggerAttributes.cs
- TemplateXamlTreeBuilder.cs
- TextLineBreak.cs
- InheritanceRules.cs
- SectionUpdates.cs
- XmlSchemaValidator.cs
- BamlWriter.cs
- IQueryable.cs
- ObjectDataSourceView.cs
- DataObjectSettingDataEventArgs.cs
- XmlUtil.cs
- PerformanceCounterLib.cs
- ColorContext.cs
- CodeBinaryOperatorExpression.cs
- TableItemPatternIdentifiers.cs
- ReferencedType.cs
- OperationPickerDialog.designer.cs
- DataExpression.cs
- SafeSerializationManager.cs
- JsonFormatGeneratorStatics.cs
- ProtocolElementCollection.cs
- PageAsyncTaskManager.cs
- MSHTMLHost.cs
- HttpCachePolicy.cs
- XmlAtomicValue.cs
- HuffCodec.cs
- AssemblyNameProxy.cs
- CodeSpit.cs
- Pointer.cs
- AssemblyAttributesGoHere.cs
- TargetConverter.cs
- SingleTagSectionHandler.cs
- JsonSerializer.cs
- InlineCollection.cs
- KnownTypesHelper.cs
- Comparer.cs
- Ops.cs
- DrawingImage.cs
- GcHandle.cs
- QilGeneratorEnv.cs
- _UriSyntax.cs
- NegatedConstant.cs
- RecordsAffectedEventArgs.cs
- BinaryReader.cs
- InkCanvas.cs
- XmlUtf8RawTextWriter.cs
- MimeTypeAttribute.cs
- ProfileInfo.cs
- ActivationArguments.cs
- ImageListUtils.cs
- LogStore.cs
- HostedElements.cs
- DurableServiceAttribute.cs
- CodeTypeDeclarationCollection.cs
- DataGridViewCellStyleConverter.cs
- ContravarianceAdapter.cs
- ThreadPool.cs
- TranslateTransform.cs
- RotateTransform.cs
- GeometryModel3D.cs
- TransformerConfigurationWizardBase.cs
- _LocalDataStoreMgr.cs
- PolygonHotSpot.cs