Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Collections / Generic / Comparer.cs / 1305376 / Comparer.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics.Contracts; //using System.Globalization; using System.Runtime.CompilerServices; namespace System.Collections.Generic { [Serializable] [TypeDependencyAttribute("System.Collections.Generic.GenericComparer`1")] public abstract class Comparer: IComparer, IComparer { static Comparer defaultComparer; public static Comparer Default { [System.Security.SecuritySafeCritical] // auto-generated get { Contract.Ensures(Contract.Result >() != null); Comparer comparer = defaultComparer; if (comparer == null) { comparer = CreateComparer(); defaultComparer = comparer; } return comparer; } } [System.Security.SecuritySafeCritical] // auto-generated private static Comparer CreateComparer() { RuntimeType t = (RuntimeType)typeof(T); // If T implements IComparable return a GenericComparer if (typeof(IComparable ).IsAssignableFrom(t)) { //return (Comparer )Activator.CreateInstance(typeof(GenericComparer<>).MakeGenericType(t)); return (Comparer )RuntimeTypeHandle.CreateInstanceForAnotherGenericParameter((RuntimeType)typeof(GenericComparer ), t); } // If T is a Nullable where U implements IComparable return a NullableComparer if (t.IsGenericType && t.GetGenericTypeDefinition() == typeof(Nullable<>)) { RuntimeType u = (RuntimeType)t.GetGenericArguments()[0]; if (typeof(IComparable<>).MakeGenericType(u).IsAssignableFrom(u)) { //return (Comparer )Activator.CreateInstance(typeof(NullableComparer<>).MakeGenericType(u)); return (Comparer )RuntimeTypeHandle.CreateInstanceForAnotherGenericParameter((RuntimeType)typeof(NullableComparer ), u); } } // Otherwise return an ObjectComparer return new ObjectComparer (); } public abstract int Compare(T x, T y); int IComparer.Compare(object x, object y) { if (x == null) return y == null ? 0 : -1; if (y == null) return 1; if (x is T && y is T) return Compare((T)x, (T)y); ThrowHelper.ThrowArgumentException(ExceptionResource.Argument_InvalidArgumentForComparison); return 0; } } [Serializable] internal class GenericComparer : Comparer where T: IComparable { public override int Compare(T x, T y) { if (x != null) { if (y != null) return x.CompareTo(y); return 1; } if (y != null) return -1; return 0; } // Equals method for the comparer itself. public override bool Equals(Object obj){ GenericComparer comparer = obj as GenericComparer ; return comparer != null; } public override int GetHashCode() { return this.GetType().Name.GetHashCode(); } } [Serializable] internal class NullableComparer : Comparer > where T : struct, IComparable { public override int Compare(Nullable x, Nullable y) { if (x.HasValue) { if (y.HasValue) return x.value.CompareTo(y.value); return 1; } if (y.HasValue) return -1; return 0; } // Equals method for the comparer itself. public override bool Equals(Object obj){ NullableComparer comparer = obj as NullableComparer ; return comparer != null; } public override int GetHashCode() { return this.GetType().Name.GetHashCode(); } } [Serializable] internal class ObjectComparer : Comparer { public override int Compare(T x, T y) { return System.Collections.Comparer.Default.Compare(x, y); } // Equals method for the comparer itself. public override bool Equals(Object obj){ ObjectComparer comparer = obj as ObjectComparer ; return comparer != null; } public override int GetHashCode() { return this.GetType().Name.GetHashCode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // [....] // using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics.Contracts; //using System.Globalization; using System.Runtime.CompilerServices; namespace System.Collections.Generic { [Serializable] [TypeDependencyAttribute("System.Collections.Generic.GenericComparer`1")] public abstract class Comparer: IComparer, IComparer { static Comparer defaultComparer; public static Comparer Default { [System.Security.SecuritySafeCritical] // auto-generated get { Contract.Ensures(Contract.Result >() != null); Comparer comparer = defaultComparer; if (comparer == null) { comparer = CreateComparer(); defaultComparer = comparer; } return comparer; } } [System.Security.SecuritySafeCritical] // auto-generated private static Comparer CreateComparer() { RuntimeType t = (RuntimeType)typeof(T); // If T implements IComparable return a GenericComparer if (typeof(IComparable ).IsAssignableFrom(t)) { //return (Comparer )Activator.CreateInstance(typeof(GenericComparer<>).MakeGenericType(t)); return (Comparer )RuntimeTypeHandle.CreateInstanceForAnotherGenericParameter((RuntimeType)typeof(GenericComparer ), t); } // If T is a Nullable where U implements IComparable return a NullableComparer if (t.IsGenericType && t.GetGenericTypeDefinition() == typeof(Nullable<>)) { RuntimeType u = (RuntimeType)t.GetGenericArguments()[0]; if (typeof(IComparable<>).MakeGenericType(u).IsAssignableFrom(u)) { //return (Comparer )Activator.CreateInstance(typeof(NullableComparer<>).MakeGenericType(u)); return (Comparer )RuntimeTypeHandle.CreateInstanceForAnotherGenericParameter((RuntimeType)typeof(NullableComparer ), u); } } // Otherwise return an ObjectComparer return new ObjectComparer (); } public abstract int Compare(T x, T y); int IComparer.Compare(object x, object y) { if (x == null) return y == null ? 0 : -1; if (y == null) return 1; if (x is T && y is T) return Compare((T)x, (T)y); ThrowHelper.ThrowArgumentException(ExceptionResource.Argument_InvalidArgumentForComparison); return 0; } } [Serializable] internal class GenericComparer : Comparer where T: IComparable { public override int Compare(T x, T y) { if (x != null) { if (y != null) return x.CompareTo(y); return 1; } if (y != null) return -1; return 0; } // Equals method for the comparer itself. public override bool Equals(Object obj){ GenericComparer comparer = obj as GenericComparer ; return comparer != null; } public override int GetHashCode() { return this.GetType().Name.GetHashCode(); } } [Serializable] internal class NullableComparer : Comparer > where T : struct, IComparable { public override int Compare(Nullable x, Nullable y) { if (x.HasValue) { if (y.HasValue) return x.value.CompareTo(y.value); return 1; } if (y.HasValue) return -1; return 0; } // Equals method for the comparer itself. public override bool Equals(Object obj){ NullableComparer comparer = obj as NullableComparer ; return comparer != null; } public override int GetHashCode() { return this.GetType().Name.GetHashCode(); } } [Serializable] internal class ObjectComparer : Comparer { public override int Compare(T x, T y) { return System.Collections.Comparer.Default.Compare(x, y); } // Equals method for the comparer itself. public override bool Equals(Object obj){ ObjectComparer comparer = obj as ObjectComparer ; return comparer != null; } public override int GetHashCode() { return this.GetType().Name.GetHashCode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
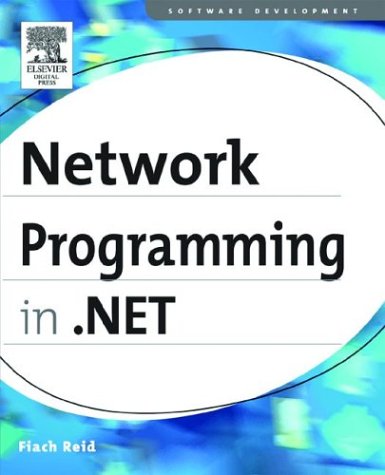
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RoutedEventConverter.cs
- QuaternionRotation3D.cs
- compensatingcollection.cs
- ToggleButtonAutomationPeer.cs
- ImageDrawing.cs
- PreviewControlDesigner.cs
- Baml2006KeyRecord.cs
- SrgsElement.cs
- InheritanceContextHelper.cs
- XslNumber.cs
- InkCanvasAutomationPeer.cs
- WindowsEditBox.cs
- ListViewItemEventArgs.cs
- DbModificationClause.cs
- KnownTypeDataContractResolver.cs
- PreservationFileReader.cs
- AtomMaterializerLog.cs
- XmlNode.cs
- SmtpNtlmAuthenticationModule.cs
- HtmlShim.cs
- ControllableStoryboardAction.cs
- DataGridItemCollection.cs
- RuntimeConfig.cs
- IndentedTextWriter.cs
- ProcessModule.cs
- BreakSafeBase.cs
- CompoundFileStorageReference.cs
- ValidatingPropertiesEventArgs.cs
- InfocardClientCredentials.cs
- ProviderIncompatibleException.cs
- TraceContextEventArgs.cs
- DecimalKeyFrameCollection.cs
- EntityCollection.cs
- QilPatternFactory.cs
- ObjectViewListener.cs
- HtmlHistory.cs
- ResourceExpressionBuilder.cs
- ExpressionTextBox.xaml.cs
- ConnectionsZone.cs
- ThemeDictionaryExtension.cs
- PermissionAttributes.cs
- LinearKeyFrames.cs
- TryCatch.cs
- FlowDocumentReaderAutomationPeer.cs
- SqlDataSourceCommandEventArgs.cs
- UnsafeNativeMethodsPenimc.cs
- FormatSettings.cs
- TdsParserHelperClasses.cs
- ShaderRenderModeValidation.cs
- MethodRental.cs
- MethodRental.cs
- DateTimeOffsetAdapter.cs
- RelationshipManager.cs
- ClientSideQueueItem.cs
- FixedSOMTextRun.cs
- PersonalizationProviderHelper.cs
- FrameworkPropertyMetadata.cs
- AnonymousIdentificationModule.cs
- PersonalizableTypeEntry.cs
- StringExpressionSet.cs
- DataKey.cs
- BamlRecordReader.cs
- ObjectDataSourceDisposingEventArgs.cs
- Nodes.cs
- CompilationLock.cs
- SqlInternalConnectionSmi.cs
- XhtmlTextWriter.cs
- UniqueEventHelper.cs
- JsonFormatWriterGenerator.cs
- SystemIcmpV6Statistics.cs
- PreloadedPackages.cs
- URLMembershipCondition.cs
- XmlSchemaExporter.cs
- ShapingEngine.cs
- ToolStripSystemRenderer.cs
- AppDomainGrammarProxy.cs
- RegionData.cs
- DockAndAnchorLayout.cs
- ParagraphResult.cs
- OutputCacheSection.cs
- ComplexTypeEmitter.cs
- FocusTracker.cs
- HttpWebRequestElement.cs
- HttpProfileBase.cs
- ClosableStream.cs
- InstanceCreationEditor.cs
- DataTable.cs
- StatusBarDrawItemEvent.cs
- SerializerProvider.cs
- Formatter.cs
- RelationshipSet.cs
- LoginView.cs
- UIElement3DAutomationPeer.cs
- Environment.cs
- CommonDialog.cs
- Message.cs
- TextFormatter.cs
- COAUTHIDENTITY.cs
- GlobalizationSection.cs
- RuntimeConfigLKG.cs