Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / FrameworkPropertyMetadata.cs / 1 / FrameworkPropertyMetadata.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using MS.Utility; using System; using System.ComponentModel; // InvalidEnumArgumentException using System.Windows.Data; // UpdateSourceTrigger namespace System.Windows { ////// [Flags] public enum FrameworkPropertyMetadataOptions: int { ///No flags None = 0x000, ///This property affects measurement AffectsMeasure = 0x001, ///This property affects arragement AffectsArrange = 0x002, ///This property affects parent's measurement AffectsParentMeasure = 0x004, ///This property affects parent's arrangement AffectsParentArrange = 0x008, ///This property affects rendering AffectsRender = 0x010, ///This property inherits to children Inherits = 0x020, ////// This property causes inheritance and resource lookup to override values /// of InheritanceBehavior that may be set on any FE in the path of lookup /// OverridesInheritanceBehavior = 0x040, ///This property does not support data binding NotDataBindable = 0x080, ///Data bindings on this property default to two-way BindsTwoWayByDefault = 0x100, ///This property should be saved/restored when journaling/navigating by URI Journal = 0x400, ////// This property's subproperties do not affect rendering. /// For instance, a property X may have a subproperty Y. /// Changing X.Y does not require rendering to be updated. /// SubPropertiesDoNotAffectRender = 0x800, } ////// Metadata for supported Framework features /// public class FrameworkPropertyMetadata : UIPropertyMetadata { ////// Framework type metadata construction. Marked as no inline to reduce code size. /// [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata() : base() { Initialize(); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Default value of property [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(object defaultValue) : base(defaultValue) { Initialize(); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Called when the property has been changed [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(PropertyChangedCallback propertyChangedCallback) : base(propertyChangedCallback) { Initialize(); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Called when the property has been changed /// Called on update of value [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(PropertyChangedCallback propertyChangedCallback, CoerceValueCallback coerceValueCallback) : base(propertyChangedCallback) { Initialize(); CoerceValueCallback = coerceValueCallback; } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Default value of property /// Called when the property has been changed [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(object defaultValue, PropertyChangedCallback propertyChangedCallback) : base(defaultValue, propertyChangedCallback) { Initialize(); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Default value of property /// Called when the property has been changed /// Called on update of value [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(object defaultValue, PropertyChangedCallback propertyChangedCallback, CoerceValueCallback coerceValueCallback) : base(defaultValue, propertyChangedCallback, coerceValueCallback) { Initialize(); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Default value of property /// Metadata option flags [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(object defaultValue, FrameworkPropertyMetadataOptions flags) : base(defaultValue) { TranslateFlags(flags); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Default value of property /// Metadata option flags /// Called when the property has been changed [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(object defaultValue, FrameworkPropertyMetadataOptions flags, PropertyChangedCallback propertyChangedCallback) : base(defaultValue, propertyChangedCallback) { TranslateFlags(flags); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Default value of property /// Metadata option flags /// Called when the property has been changed /// Called on update of value [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(object defaultValue, FrameworkPropertyMetadataOptions flags, PropertyChangedCallback propertyChangedCallback, CoerceValueCallback coerceValueCallback) : base(defaultValue, propertyChangedCallback, coerceValueCallback) { TranslateFlags(flags); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Default value of property /// Metadata option flags /// Called when the property has been changed /// Called on update of value /// Should animation of this property be prohibited? [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(object defaultValue, FrameworkPropertyMetadataOptions flags, PropertyChangedCallback propertyChangedCallback, CoerceValueCallback coerceValueCallback, bool isAnimationProhibited) : base(defaultValue, propertyChangedCallback, coerceValueCallback, isAnimationProhibited) { TranslateFlags(flags); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Default value of property /// Metadata option flags /// Called when the property has been changed /// Called on update of value /// Should animation of this property be prohibited? /// The UpdateSourceTrigger to use for bindings that have UpdateSourceTriger=Default. [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(object defaultValue, FrameworkPropertyMetadataOptions flags, PropertyChangedCallback propertyChangedCallback, CoerceValueCallback coerceValueCallback, bool isAnimationProhibited, UpdateSourceTrigger defaultUpdateSourceTrigger) : base(defaultValue, propertyChangedCallback, coerceValueCallback, isAnimationProhibited) { if (!BindingOperations.IsValidUpdateSourceTrigger(defaultUpdateSourceTrigger)) throw new InvalidEnumArgumentException("defaultUpdateSourceTrigger", (int) defaultUpdateSourceTrigger, typeof(UpdateSourceTrigger)); if (defaultUpdateSourceTrigger == UpdateSourceTrigger.Default) throw new ArgumentException(SR.Get(SRID.NoDefaultUpdateSourceTrigger), "defaultUpdateSourceTrigger"); TranslateFlags(flags); DefaultUpdateSourceTrigger = defaultUpdateSourceTrigger; } private void Initialize() { // FW_DefaultUpdateSourceTriggerEnumBit1 = 0x40000000, // FW_DefaultUpdateSourceTriggerEnumBit2 = 0x80000000, _flags = (MetadataFlags)(((uint)_flags & 0x3FFFFFFF) | ((uint) UpdateSourceTrigger.PropertyChanged) << 30); } private static bool IsFlagSet(FrameworkPropertyMetadataOptions flag, FrameworkPropertyMetadataOptions flags) { return (flags & flag) != 0; } private void TranslateFlags(FrameworkPropertyMetadataOptions flags) { Initialize(); // Convert flags to state sets. If a flag is set, then, // the value is set on the respective property. Otherwise, // the state remains unset // This means that state is cumulative across base classes // on a merge where appropriate if (IsFlagSet(FrameworkPropertyMetadataOptions.AffectsMeasure, flags)) { AffectsMeasure = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.AffectsArrange, flags)) { AffectsArrange = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.AffectsParentMeasure, flags)) { AffectsParentMeasure = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.AffectsParentArrange, flags)) { AffectsParentArrange = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.AffectsRender, flags)) { AffectsRender = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.Inherits, flags)) { IsInherited = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.OverridesInheritanceBehavior, flags)) { OverridesInheritanceBehavior = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.NotDataBindable, flags)) { IsNotDataBindable = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.BindsTwoWayByDefault, flags)) { BindsTwoWayByDefault = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.Journal, flags)) { Journal = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.SubPropertiesDoNotAffectRender, flags)) { SubPropertiesDoNotAffectRender = true; } } ////// Property affects measurement /// public bool AffectsMeasure { get { return ReadFlag(MetadataFlags.FW_AffectsMeasureID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_AffectsMeasureID, value); } } ////// Property affects arragement /// public bool AffectsArrange { get { return ReadFlag(MetadataFlags.FW_AffectsArrangeID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_AffectsArrangeID, value); } } ////// Property affects parent's measurement /// public bool AffectsParentMeasure { get { return ReadFlag(MetadataFlags.FW_AffectsParentMeasureID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_AffectsParentMeasureID, value); } } ////// Property affects parent's arrangement /// public bool AffectsParentArrange { get { return ReadFlag(MetadataFlags.FW_AffectsParentArrangeID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_AffectsParentArrangeID, value); } } ////// Property affects rendering /// public bool AffectsRender { get { return ReadFlag(MetadataFlags.FW_AffectsRenderID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_AffectsRenderID, value); } } ////// Property is inheritable /// public bool Inherits { get { return IsInherited; } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } IsInherited = value; SetModified(MetadataFlags.FW_InheritsModifiedID); } } ////// Property evaluation must span separated trees /// public bool OverridesInheritanceBehavior { get { return ReadFlag(MetadataFlags.FW_OverridesInheritanceBehaviorID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_OverridesInheritanceBehaviorID, value); SetModified(MetadataFlags.FW_OverridesInheritanceBehaviorModifiedID); } } ////// Property cannot be data-bound /// public bool IsNotDataBindable { get { return ReadFlag(MetadataFlags.FW_IsNotDataBindableID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_IsNotDataBindableID, value); } } ////// Data bindings on this property default to two-way /// public bool BindsTwoWayByDefault { get { return ReadFlag(MetadataFlags.FW_BindsTwoWayByDefaultID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_BindsTwoWayByDefaultID, value); } } ////// The default UpdateSourceTrigger for two-way data bindings on this property. /// public UpdateSourceTrigger DefaultUpdateSourceTrigger { // FW_DefaultUpdateSourceTriggerEnumBit1 = 0x40000000, // FW_DefaultUpdateSourceTriggerEnumBit2 = 0x80000000, get { return (UpdateSourceTrigger) (((uint) _flags >> 30) & 0x3); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } if (!BindingOperations.IsValidUpdateSourceTrigger(value)) throw new InvalidEnumArgumentException("value", (int) value, typeof(UpdateSourceTrigger)); if (value == UpdateSourceTrigger.Default) throw new ArgumentException(SR.Get(SRID.NoDefaultUpdateSourceTrigger), "value"); // FW_DefaultUpdateSourceTriggerEnumBit1 = 0x40000000, // FW_DefaultUpdateSourceTriggerEnumBit2 = 0x80000000, _flags = (MetadataFlags)(((uint) _flags & 0x3FFFFFFF) | ((uint) value) << 30); SetModified(MetadataFlags.FW_DefaultUpdateSourceTriggerModifiedID); } } ////// The value of this property should be saved/restored when journaling by URI /// public bool Journal { get { return ReadFlag(MetadataFlags.FW_ShouldBeJournaledID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_ShouldBeJournaledID, value); SetModified(MetadataFlags.FW_ShouldBeJournaledModifiedID); } } ////// This property's subproperties do not affect rendering. /// For instance, a property X may have a subproperty Y. /// Changing X.Y does not require rendering to be updated. /// public bool SubPropertiesDoNotAffectRender { get { return ReadFlag(MetadataFlags.FW_SubPropertiesDoNotAffectRenderID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_SubPropertiesDoNotAffectRenderID, value); SetModified(MetadataFlags.FW_SubPropertiesDoNotAffectRenderModifiedID); } } ////// Does the represent the metadata for a ReadOnly property /// private bool ReadOnly { get { return ReadFlag(MetadataFlags.FW_ReadOnlyID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_ReadOnlyID, value); } } ////// Creates a new instance of this property metadata. This method is used /// when metadata needs to be cloned. After CreateInstance is called the /// framework will call Merge to merge metadata into the new instance. /// Deriving classes must override this and return a new instance of /// themselves. /// internal override PropertyMetadata CreateInstance() { return new FrameworkPropertyMetadata(); } ////// Merge set source state into this /// ////// Used when overriding metadata /// /// Base metadata to merge /// DependencyProperty that this metadata is being applied to protected override void Merge(PropertyMetadata baseMetadata, DependencyProperty dp) { // Does parameter validation base.Merge(baseMetadata, dp); // Source type is guaranteed to be the same type or base type FrameworkPropertyMetadata fbaseMetadata = baseMetadata as FrameworkPropertyMetadata; if (fbaseMetadata != null) { // Merge source metadata into this // Modify metadata merge state fields directly (not through accessors // so that "modified" bits remain intact // Merge state // Defaults to false, derived classes can only enable WriteFlag(MetadataFlags.FW_AffectsMeasureID, ReadFlag(MetadataFlags.FW_AffectsMeasureID) | fbaseMetadata.AffectsMeasure); WriteFlag(MetadataFlags.FW_AffectsArrangeID, ReadFlag(MetadataFlags.FW_AffectsArrangeID) | fbaseMetadata.AffectsArrange); WriteFlag(MetadataFlags.FW_AffectsParentMeasureID, ReadFlag(MetadataFlags.FW_AffectsParentMeasureID) | fbaseMetadata.AffectsParentMeasure); WriteFlag(MetadataFlags.FW_AffectsParentArrangeID, ReadFlag(MetadataFlags.FW_AffectsParentArrangeID) | fbaseMetadata.AffectsParentArrange); WriteFlag(MetadataFlags.FW_AffectsRenderID, ReadFlag(MetadataFlags.FW_AffectsRenderID) | fbaseMetadata.AffectsRender); WriteFlag(MetadataFlags.FW_BindsTwoWayByDefaultID, ReadFlag(MetadataFlags.FW_BindsTwoWayByDefaultID) | fbaseMetadata.BindsTwoWayByDefault); WriteFlag(MetadataFlags.FW_IsNotDataBindableID, ReadFlag(MetadataFlags.FW_IsNotDataBindableID) | fbaseMetadata.IsNotDataBindable); // Override state if (!IsModified(MetadataFlags.FW_SubPropertiesDoNotAffectRenderModifiedID)) { WriteFlag(MetadataFlags.FW_SubPropertiesDoNotAffectRenderID, fbaseMetadata.SubPropertiesDoNotAffectRender); } if (!IsModified(MetadataFlags.FW_InheritsModifiedID)) { IsInherited = fbaseMetadata.Inherits; } if (!IsModified(MetadataFlags.FW_OverridesInheritanceBehaviorModifiedID)) { WriteFlag(MetadataFlags.FW_OverridesInheritanceBehaviorID, fbaseMetadata.OverridesInheritanceBehavior); } if (!IsModified(MetadataFlags.FW_ShouldBeJournaledModifiedID)) { WriteFlag(MetadataFlags.FW_ShouldBeJournaledID, fbaseMetadata.Journal); } if (!IsModified(MetadataFlags.FW_DefaultUpdateSourceTriggerModifiedID)) { // FW_DefaultUpdateSourceTriggerEnumBit1 = 0x40000000, // FW_DefaultUpdateSourceTriggerEnumBit2 = 0x80000000, _flags = (MetadataFlags)(((uint)_flags & 0x3FFFFFFF) | ((uint) fbaseMetadata.DefaultUpdateSourceTrigger) << 30); } } } ////// Notification that this metadata has been applied to a property /// and the metadata is being sealed /// ////// Normally, any mutability of the data structure should be marked /// as immutable at this point /// /// DependencyProperty /// Type associating metadata (null if default metadata) protected override void OnApply(DependencyProperty dp, Type targetType) { // Remember if this is the metadata for a ReadOnly property ReadOnly = dp.ReadOnly; base.OnApply(dp, targetType); } ////// Determines if data binding is supported /// ////// Data binding is not allowed if a property read-only, regardless /// of the value of the IsNotDataBindable flag /// public bool IsDataBindingAllowed { get { return !ReadFlag(MetadataFlags.FW_IsNotDataBindableID) && !ReadOnly; } } internal void SetModified(MetadataFlags id) { WriteFlag(id, true); } internal bool IsModified(MetadataFlags id) { return ReadFlag(id); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using MS.Utility; using System; using System.ComponentModel; // InvalidEnumArgumentException using System.Windows.Data; // UpdateSourceTrigger namespace System.Windows { ////// [Flags] public enum FrameworkPropertyMetadataOptions: int { ///No flags None = 0x000, ///This property affects measurement AffectsMeasure = 0x001, ///This property affects arragement AffectsArrange = 0x002, ///This property affects parent's measurement AffectsParentMeasure = 0x004, ///This property affects parent's arrangement AffectsParentArrange = 0x008, ///This property affects rendering AffectsRender = 0x010, ///This property inherits to children Inherits = 0x020, ////// This property causes inheritance and resource lookup to override values /// of InheritanceBehavior that may be set on any FE in the path of lookup /// OverridesInheritanceBehavior = 0x040, ///This property does not support data binding NotDataBindable = 0x080, ///Data bindings on this property default to two-way BindsTwoWayByDefault = 0x100, ///This property should be saved/restored when journaling/navigating by URI Journal = 0x400, ////// This property's subproperties do not affect rendering. /// For instance, a property X may have a subproperty Y. /// Changing X.Y does not require rendering to be updated. /// SubPropertiesDoNotAffectRender = 0x800, } ////// Metadata for supported Framework features /// public class FrameworkPropertyMetadata : UIPropertyMetadata { ////// Framework type metadata construction. Marked as no inline to reduce code size. /// [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata() : base() { Initialize(); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Default value of property [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(object defaultValue) : base(defaultValue) { Initialize(); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Called when the property has been changed [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(PropertyChangedCallback propertyChangedCallback) : base(propertyChangedCallback) { Initialize(); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Called when the property has been changed /// Called on update of value [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(PropertyChangedCallback propertyChangedCallback, CoerceValueCallback coerceValueCallback) : base(propertyChangedCallback) { Initialize(); CoerceValueCallback = coerceValueCallback; } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Default value of property /// Called when the property has been changed [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(object defaultValue, PropertyChangedCallback propertyChangedCallback) : base(defaultValue, propertyChangedCallback) { Initialize(); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Default value of property /// Called when the property has been changed /// Called on update of value [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(object defaultValue, PropertyChangedCallback propertyChangedCallback, CoerceValueCallback coerceValueCallback) : base(defaultValue, propertyChangedCallback, coerceValueCallback) { Initialize(); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Default value of property /// Metadata option flags [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(object defaultValue, FrameworkPropertyMetadataOptions flags) : base(defaultValue) { TranslateFlags(flags); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Default value of property /// Metadata option flags /// Called when the property has been changed [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(object defaultValue, FrameworkPropertyMetadataOptions flags, PropertyChangedCallback propertyChangedCallback) : base(defaultValue, propertyChangedCallback) { TranslateFlags(flags); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Default value of property /// Metadata option flags /// Called when the property has been changed /// Called on update of value [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(object defaultValue, FrameworkPropertyMetadataOptions flags, PropertyChangedCallback propertyChangedCallback, CoerceValueCallback coerceValueCallback) : base(defaultValue, propertyChangedCallback, coerceValueCallback) { TranslateFlags(flags); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Default value of property /// Metadata option flags /// Called when the property has been changed /// Called on update of value /// Should animation of this property be prohibited? [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(object defaultValue, FrameworkPropertyMetadataOptions flags, PropertyChangedCallback propertyChangedCallback, CoerceValueCallback coerceValueCallback, bool isAnimationProhibited) : base(defaultValue, propertyChangedCallback, coerceValueCallback, isAnimationProhibited) { TranslateFlags(flags); } ////// Framework type metadata construction. Marked as no inline to reduce code size. /// /// Default value of property /// Metadata option flags /// Called when the property has been changed /// Called on update of value /// Should animation of this property be prohibited? /// The UpdateSourceTrigger to use for bindings that have UpdateSourceTriger=Default. [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] public FrameworkPropertyMetadata(object defaultValue, FrameworkPropertyMetadataOptions flags, PropertyChangedCallback propertyChangedCallback, CoerceValueCallback coerceValueCallback, bool isAnimationProhibited, UpdateSourceTrigger defaultUpdateSourceTrigger) : base(defaultValue, propertyChangedCallback, coerceValueCallback, isAnimationProhibited) { if (!BindingOperations.IsValidUpdateSourceTrigger(defaultUpdateSourceTrigger)) throw new InvalidEnumArgumentException("defaultUpdateSourceTrigger", (int) defaultUpdateSourceTrigger, typeof(UpdateSourceTrigger)); if (defaultUpdateSourceTrigger == UpdateSourceTrigger.Default) throw new ArgumentException(SR.Get(SRID.NoDefaultUpdateSourceTrigger), "defaultUpdateSourceTrigger"); TranslateFlags(flags); DefaultUpdateSourceTrigger = defaultUpdateSourceTrigger; } private void Initialize() { // FW_DefaultUpdateSourceTriggerEnumBit1 = 0x40000000, // FW_DefaultUpdateSourceTriggerEnumBit2 = 0x80000000, _flags = (MetadataFlags)(((uint)_flags & 0x3FFFFFFF) | ((uint) UpdateSourceTrigger.PropertyChanged) << 30); } private static bool IsFlagSet(FrameworkPropertyMetadataOptions flag, FrameworkPropertyMetadataOptions flags) { return (flags & flag) != 0; } private void TranslateFlags(FrameworkPropertyMetadataOptions flags) { Initialize(); // Convert flags to state sets. If a flag is set, then, // the value is set on the respective property. Otherwise, // the state remains unset // This means that state is cumulative across base classes // on a merge where appropriate if (IsFlagSet(FrameworkPropertyMetadataOptions.AffectsMeasure, flags)) { AffectsMeasure = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.AffectsArrange, flags)) { AffectsArrange = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.AffectsParentMeasure, flags)) { AffectsParentMeasure = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.AffectsParentArrange, flags)) { AffectsParentArrange = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.AffectsRender, flags)) { AffectsRender = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.Inherits, flags)) { IsInherited = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.OverridesInheritanceBehavior, flags)) { OverridesInheritanceBehavior = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.NotDataBindable, flags)) { IsNotDataBindable = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.BindsTwoWayByDefault, flags)) { BindsTwoWayByDefault = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.Journal, flags)) { Journal = true; } if (IsFlagSet(FrameworkPropertyMetadataOptions.SubPropertiesDoNotAffectRender, flags)) { SubPropertiesDoNotAffectRender = true; } } ////// Property affects measurement /// public bool AffectsMeasure { get { return ReadFlag(MetadataFlags.FW_AffectsMeasureID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_AffectsMeasureID, value); } } ////// Property affects arragement /// public bool AffectsArrange { get { return ReadFlag(MetadataFlags.FW_AffectsArrangeID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_AffectsArrangeID, value); } } ////// Property affects parent's measurement /// public bool AffectsParentMeasure { get { return ReadFlag(MetadataFlags.FW_AffectsParentMeasureID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_AffectsParentMeasureID, value); } } ////// Property affects parent's arrangement /// public bool AffectsParentArrange { get { return ReadFlag(MetadataFlags.FW_AffectsParentArrangeID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_AffectsParentArrangeID, value); } } ////// Property affects rendering /// public bool AffectsRender { get { return ReadFlag(MetadataFlags.FW_AffectsRenderID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_AffectsRenderID, value); } } ////// Property is inheritable /// public bool Inherits { get { return IsInherited; } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } IsInherited = value; SetModified(MetadataFlags.FW_InheritsModifiedID); } } ////// Property evaluation must span separated trees /// public bool OverridesInheritanceBehavior { get { return ReadFlag(MetadataFlags.FW_OverridesInheritanceBehaviorID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_OverridesInheritanceBehaviorID, value); SetModified(MetadataFlags.FW_OverridesInheritanceBehaviorModifiedID); } } ////// Property cannot be data-bound /// public bool IsNotDataBindable { get { return ReadFlag(MetadataFlags.FW_IsNotDataBindableID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_IsNotDataBindableID, value); } } ////// Data bindings on this property default to two-way /// public bool BindsTwoWayByDefault { get { return ReadFlag(MetadataFlags.FW_BindsTwoWayByDefaultID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_BindsTwoWayByDefaultID, value); } } ////// The default UpdateSourceTrigger for two-way data bindings on this property. /// public UpdateSourceTrigger DefaultUpdateSourceTrigger { // FW_DefaultUpdateSourceTriggerEnumBit1 = 0x40000000, // FW_DefaultUpdateSourceTriggerEnumBit2 = 0x80000000, get { return (UpdateSourceTrigger) (((uint) _flags >> 30) & 0x3); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } if (!BindingOperations.IsValidUpdateSourceTrigger(value)) throw new InvalidEnumArgumentException("value", (int) value, typeof(UpdateSourceTrigger)); if (value == UpdateSourceTrigger.Default) throw new ArgumentException(SR.Get(SRID.NoDefaultUpdateSourceTrigger), "value"); // FW_DefaultUpdateSourceTriggerEnumBit1 = 0x40000000, // FW_DefaultUpdateSourceTriggerEnumBit2 = 0x80000000, _flags = (MetadataFlags)(((uint) _flags & 0x3FFFFFFF) | ((uint) value) << 30); SetModified(MetadataFlags.FW_DefaultUpdateSourceTriggerModifiedID); } } ////// The value of this property should be saved/restored when journaling by URI /// public bool Journal { get { return ReadFlag(MetadataFlags.FW_ShouldBeJournaledID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_ShouldBeJournaledID, value); SetModified(MetadataFlags.FW_ShouldBeJournaledModifiedID); } } ////// This property's subproperties do not affect rendering. /// For instance, a property X may have a subproperty Y. /// Changing X.Y does not require rendering to be updated. /// public bool SubPropertiesDoNotAffectRender { get { return ReadFlag(MetadataFlags.FW_SubPropertiesDoNotAffectRenderID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_SubPropertiesDoNotAffectRenderID, value); SetModified(MetadataFlags.FW_SubPropertiesDoNotAffectRenderModifiedID); } } ////// Does the represent the metadata for a ReadOnly property /// private bool ReadOnly { get { return ReadFlag(MetadataFlags.FW_ReadOnlyID); } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.TypeMetadataCannotChangeAfterUse)); } WriteFlag(MetadataFlags.FW_ReadOnlyID, value); } } ////// Creates a new instance of this property metadata. This method is used /// when metadata needs to be cloned. After CreateInstance is called the /// framework will call Merge to merge metadata into the new instance. /// Deriving classes must override this and return a new instance of /// themselves. /// internal override PropertyMetadata CreateInstance() { return new FrameworkPropertyMetadata(); } ////// Merge set source state into this /// ////// Used when overriding metadata /// /// Base metadata to merge /// DependencyProperty that this metadata is being applied to protected override void Merge(PropertyMetadata baseMetadata, DependencyProperty dp) { // Does parameter validation base.Merge(baseMetadata, dp); // Source type is guaranteed to be the same type or base type FrameworkPropertyMetadata fbaseMetadata = baseMetadata as FrameworkPropertyMetadata; if (fbaseMetadata != null) { // Merge source metadata into this // Modify metadata merge state fields directly (not through accessors // so that "modified" bits remain intact // Merge state // Defaults to false, derived classes can only enable WriteFlag(MetadataFlags.FW_AffectsMeasureID, ReadFlag(MetadataFlags.FW_AffectsMeasureID) | fbaseMetadata.AffectsMeasure); WriteFlag(MetadataFlags.FW_AffectsArrangeID, ReadFlag(MetadataFlags.FW_AffectsArrangeID) | fbaseMetadata.AffectsArrange); WriteFlag(MetadataFlags.FW_AffectsParentMeasureID, ReadFlag(MetadataFlags.FW_AffectsParentMeasureID) | fbaseMetadata.AffectsParentMeasure); WriteFlag(MetadataFlags.FW_AffectsParentArrangeID, ReadFlag(MetadataFlags.FW_AffectsParentArrangeID) | fbaseMetadata.AffectsParentArrange); WriteFlag(MetadataFlags.FW_AffectsRenderID, ReadFlag(MetadataFlags.FW_AffectsRenderID) | fbaseMetadata.AffectsRender); WriteFlag(MetadataFlags.FW_BindsTwoWayByDefaultID, ReadFlag(MetadataFlags.FW_BindsTwoWayByDefaultID) | fbaseMetadata.BindsTwoWayByDefault); WriteFlag(MetadataFlags.FW_IsNotDataBindableID, ReadFlag(MetadataFlags.FW_IsNotDataBindableID) | fbaseMetadata.IsNotDataBindable); // Override state if (!IsModified(MetadataFlags.FW_SubPropertiesDoNotAffectRenderModifiedID)) { WriteFlag(MetadataFlags.FW_SubPropertiesDoNotAffectRenderID, fbaseMetadata.SubPropertiesDoNotAffectRender); } if (!IsModified(MetadataFlags.FW_InheritsModifiedID)) { IsInherited = fbaseMetadata.Inherits; } if (!IsModified(MetadataFlags.FW_OverridesInheritanceBehaviorModifiedID)) { WriteFlag(MetadataFlags.FW_OverridesInheritanceBehaviorID, fbaseMetadata.OverridesInheritanceBehavior); } if (!IsModified(MetadataFlags.FW_ShouldBeJournaledModifiedID)) { WriteFlag(MetadataFlags.FW_ShouldBeJournaledID, fbaseMetadata.Journal); } if (!IsModified(MetadataFlags.FW_DefaultUpdateSourceTriggerModifiedID)) { // FW_DefaultUpdateSourceTriggerEnumBit1 = 0x40000000, // FW_DefaultUpdateSourceTriggerEnumBit2 = 0x80000000, _flags = (MetadataFlags)(((uint)_flags & 0x3FFFFFFF) | ((uint) fbaseMetadata.DefaultUpdateSourceTrigger) << 30); } } } ////// Notification that this metadata has been applied to a property /// and the metadata is being sealed /// ////// Normally, any mutability of the data structure should be marked /// as immutable at this point /// /// DependencyProperty /// Type associating metadata (null if default metadata) protected override void OnApply(DependencyProperty dp, Type targetType) { // Remember if this is the metadata for a ReadOnly property ReadOnly = dp.ReadOnly; base.OnApply(dp, targetType); } ////// Determines if data binding is supported /// ////// Data binding is not allowed if a property read-only, regardless /// of the value of the IsNotDataBindable flag /// public bool IsDataBindingAllowed { get { return !ReadFlag(MetadataFlags.FW_IsNotDataBindableID) && !ReadOnly; } } internal void SetModified(MetadataFlags id) { WriteFlag(id, true); } internal bool IsModified(MetadataFlags id) { return ReadFlag(id); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
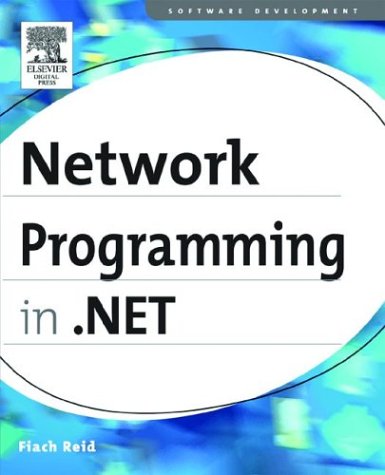
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VisualStyleElement.cs
- PeerNearMe.cs
- SerializationStore.cs
- SourceItem.cs
- StatusStrip.cs
- BlobPersonalizationState.cs
- XmlArrayAttribute.cs
- FontWeights.cs
- ErrorFormatterPage.cs
- UpDownEvent.cs
- FileUtil.cs
- UInt32Converter.cs
- StringCollection.cs
- FlowDocumentReaderAutomationPeer.cs
- MouseButton.cs
- SiteMapDataSource.cs
- SplitterPanel.cs
- SqlLiftWhereClauses.cs
- HeaderCollection.cs
- TreeIterator.cs
- httpapplicationstate.cs
- StackOverflowException.cs
- RuleProcessor.cs
- WizardSideBarListControlItem.cs
- StringInfo.cs
- DataKey.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- HtmlForm.cs
- ObjectItemCollection.cs
- BitmapCodecInfoInternal.cs
- DiffuseMaterial.cs
- WebServiceParameterData.cs
- AssemblyResourceLoader.cs
- Point3DCollection.cs
- WebAdminConfigurationHelper.cs
- MobileContainerDesigner.cs
- DbMetaDataColumnNames.cs
- TextEditorTyping.cs
- FactoryGenerator.cs
- XmlBinaryReaderSession.cs
- DeclaredTypeValidatorAttribute.cs
- XmlReflectionMember.cs
- WebPartMinimizeVerb.cs
- ConfigXmlText.cs
- CaseStatement.cs
- StaticExtension.cs
- SqlUtils.cs
- HttpFormatExtensions.cs
- MdbDataFileEditor.cs
- BitmapCodecInfoInternal.cs
- EtwTrace.cs
- BulletDecorator.cs
- Rect.cs
- SerializationAttributes.cs
- TransformCollection.cs
- BindingOperations.cs
- RelationshipSet.cs
- DataGridViewAddColumnDialog.cs
- SHA512.cs
- XmlSerializerVersionAttribute.cs
- ProjectionNode.cs
- PathFigure.cs
- NetNamedPipeSecurityElement.cs
- IntegrationExceptionEventArgs.cs
- Debug.cs
- PreviousTrackingServiceAttribute.cs
- SecurityPermission.cs
- PreservationFileWriter.cs
- AssemblyHash.cs
- DeriveBytes.cs
- DiagnosticTraceSource.cs
- MediaTimeline.cs
- CookieProtection.cs
- DataServiceBehavior.cs
- AnnotationComponentChooser.cs
- PackageFilter.cs
- ThreadPoolTaskScheduler.cs
- WebPartAuthorizationEventArgs.cs
- NominalTypeEliminator.cs
- XmlHierarchicalDataSourceView.cs
- AbstractDataSvcMapFileLoader.cs
- SafeLibraryHandle.cs
- DragDropHelper.cs
- TableLayoutRowStyleCollection.cs
- IsolatedStorage.cs
- TCEAdapterGenerator.cs
- Listbox.cs
- x509store.cs
- SecurityKeyIdentifierClause.cs
- _AuthenticationState.cs
- RecordManager.cs
- BitmapSourceSafeMILHandle.cs
- ListView.cs
- ComponentManagerBroker.cs
- GlyphCache.cs
- ControlType.cs
- OracleBFile.cs
- TcpClientSocketManager.cs
- Scene3D.cs
- UpdatePanelTriggerCollection.cs