Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / Security / CookieProtection.cs / 1 / CookieProtection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Security { using System.Security.Cryptography; using System.Web.Configuration; using System.Web.Management; public enum CookieProtection { None, Validation, Encryption, All } internal class CookieProtectionHelper { internal static string Encode (CookieProtection cookieProtection, byte [] buf, int count) { if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Validation) { byte[] bMac = MachineKeySection.HashData (buf, null, 0, count); if (bMac == null || bMac.Length != 20) return null; if (buf.Length >= count + 20) { Buffer.BlockCopy (bMac, 0, buf, count, 20); } else { byte[] bTemp = buf; buf = new byte[count + 20]; Buffer.BlockCopy (bTemp, 0, buf, 0, count); Buffer.BlockCopy (bMac, 0, buf, count, 20); } count += 20; } if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Encryption) { buf = MachineKeySection.EncryptOrDecryptData (true, buf, null, 0, count); count = buf.Length; } if (count < buf.Length) { byte[] bTemp = buf; buf = new byte[count]; Buffer.BlockCopy (bTemp, 0, buf, 0, count); } return HttpServerUtility.UrlTokenEncode(buf); } internal static byte[] Decode (CookieProtection cookieProtection, string data) { byte [] buf = HttpServerUtility.UrlTokenDecode(data); if (buf == null || cookieProtection == CookieProtection.None) return buf; if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Encryption) { buf = MachineKeySection.EncryptOrDecryptData (false, buf, null, 0, buf.Length); if (buf == null) return null; } if (cookieProtection == CookieProtection.All || cookieProtection == CookieProtection.Validation) { ////////////////////////////////////////////////////////////////////// // Step 2: Get the MAC: Last 20 bytes if (buf.Length <= 20) return null; byte[] buf2 = new byte[buf.Length - 20]; Buffer.BlockCopy (buf, 0, buf2, 0, buf2.Length); byte[] bMac = MachineKeySection.HashData (buf2, null, 0, buf2.Length); ////////////////////////////////////////////////////////////////////// // Step 3: Make sure the MAC is correct if (bMac == null || bMac.Length != 20) return null; for (int iter = 0; iter < 20; iter++) if (bMac[iter] != buf[buf2.Length + iter]) return null; buf = buf2; } return buf; } } }
Link Menu
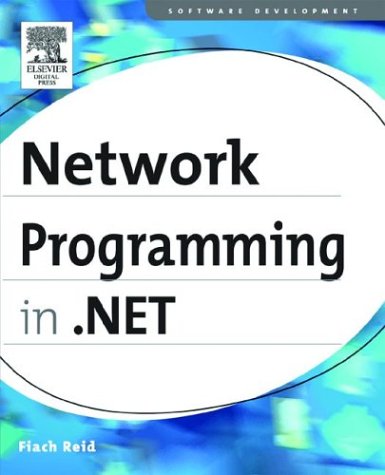
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IImplicitResourceProvider.cs
- TypedReference.cs
- UInt64.cs
- SqlMethods.cs
- BoolExpression.cs
- NotifyParentPropertyAttribute.cs
- XmlProcessingInstruction.cs
- ColumnClickEvent.cs
- FrameworkElement.cs
- DescriptionCreator.cs
- FunctionParameter.cs
- If.cs
- XslAst.cs
- UpdateTracker.cs
- CodeTypeReference.cs
- DbConnectionOptions.cs
- TableLayoutRowStyleCollection.cs
- ErrorTolerantObjectWriter.cs
- LinkedResourceCollection.cs
- BindingMAnagerBase.cs
- ObjectConverter.cs
- MeasureItemEvent.cs
- PointIndependentAnimationStorage.cs
- ColumnMap.cs
- OleDbParameterCollection.cs
- ExtendedPropertyInfo.cs
- XmlTextWriter.cs
- ExpressionTextBox.xaml.cs
- DataServiceExpressionVisitor.cs
- DrawingDrawingContext.cs
- CodeGeneratorOptions.cs
- ParallelTimeline.cs
- querybuilder.cs
- UIElementParaClient.cs
- DeferredBinaryDeserializerExtension.cs
- XamlParser.cs
- Int64KeyFrameCollection.cs
- PkcsUtils.cs
- WebPartCancelEventArgs.cs
- Relationship.cs
- MarkupCompilePass1.cs
- ListManagerBindingsCollection.cs
- LocalizationParserHooks.cs
- InheritanceRules.cs
- TextRange.cs
- ContentElement.cs
- NavigationHelper.cs
- Menu.cs
- WebPartExportVerb.cs
- Inflater.cs
- complextypematerializer.cs
- ListChangedEventArgs.cs
- DataObjectMethodAttribute.cs
- CodeSnippetCompileUnit.cs
- Merger.cs
- ValueTypeFixupInfo.cs
- PriorityChain.cs
- DESCryptoServiceProvider.cs
- ModuleBuilderData.cs
- PowerModeChangedEventArgs.cs
- AssemblyCacheEntry.cs
- HMACSHA1.cs
- DoubleLinkListEnumerator.cs
- OracleException.cs
- TypeListConverter.cs
- Sql8ConformanceChecker.cs
- PropertyValueUIItem.cs
- DtdParser.cs
- HitTestDrawingContextWalker.cs
- ListSortDescription.cs
- KeyboardNavigation.cs
- ProfileProvider.cs
- DefaultDiscoveryService.cs
- TransformPattern.cs
- SystemColorTracker.cs
- safemediahandle.cs
- TextEffect.cs
- DBSqlParserColumnCollection.cs
- LiteralSubsegment.cs
- PanelDesigner.cs
- Fault.cs
- SimpleTypesSurrogate.cs
- FunctionUpdateCommand.cs
- XmlSchemas.cs
- HeaderFilter.cs
- ResXDataNode.cs
- DataServiceRequestOfT.cs
- TextPattern.cs
- DeviceContext2.cs
- NativeMethods.cs
- ToolStripGrip.cs
- FusionWrap.cs
- EntityClassGenerator.cs
- TCPListener.cs
- RectangleHotSpot.cs
- WebErrorHandler.cs
- XmlUtf8RawTextWriter.cs
- Random.cs
- StrongNameKeyPair.cs
- util.cs