Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / DataOracleClient / System / Data / OracleClient / OracleException.cs / 4 / OracleException.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.Data; using System.Data.Common; using System.Diagnostics; using System.IO; using System.Globalization; using System.Runtime.CompilerServices; using System.Runtime.InteropServices; using System.Runtime.Serialization; using System.Runtime.ConstrainedExecution; //--------------------------------------------------------------------- // OracleException // // You end up with one of these when an OCI call fails unexpectedly. // [Serializable] sealed public class OracleException : System.Data.Common.DbException { private int _code; // runtime will call even if private... private OracleException(SerializationInfo si, StreamingContext sc) : base(si, sc) { _code = (int) si.GetValue("code", typeof(int)); HResult = HResults.OracleException; } private OracleException(string message, int code) : base(message) { _code = code; HResult = HResults.OracleException; } public int Code { get { return _code; } } static private bool ConnectionIsBroken(int code) { bool result; if (12500 <= code && code <= 12699) { // TNS errors result = true; } else { switch (code) { case 18: // max sessions exceeded case 19: // max session licenses exceeded case 24: // server is in single process mode case 28: // session has been killed case 436: // oracle isn't licensed case 1012: // not logged on. case 1033: // startup/shutdown in progress case 1034: // oracle not available case 1075: // currently logged on case 2392: // exceeded session limit on CPU usage,you are being logged off. case 2399: // exceeded maximum connect time, you are being logged off. case 3113: // end-of-file on communication channel case 3114: // not connected to ORACLE result = true; break; default: result = false; break; } } return result; } static internal OracleException CreateException(OciErrorHandle errorHandle, int rc) { int code; string message; using (NativeBuffer buf = new NativeBuffer_Exception(1000)) { if (null != errorHandle) { int record = 1; int rcTemp = TracedNativeMethods.OCIErrorGet( errorHandle, record, out code, buf ); if (0 == rcTemp) { message = errorHandle.PtrToString(buf); // For warning messages, revert back to the OCI7 routine to get // the text of the message. if (code != 0 && message.StartsWith("ORA-00000", StringComparison.Ordinal)) { rcTemp = TracedNativeMethods.oermsg((short)code, buf); if (0 == rcTemp) { message = errorHandle.PtrToString(buf); } } } else { Debug.Assert(false, "Failed to get oracle message text"); // If we couldn't successfully read the message text, we pick // something more descriptive, like "we couldn't read the message" // instead of just handing back an empty string... message = Res.GetString(Res.ADP_NoMessageAvailable, rc, rcTemp); code = 0; } // Make sure we doom the connection if its broken. if (ConnectionIsBroken(code)) { errorHandle.ConnectionIsBroken = true; } } else { Debug.Assert(false, "Failed to get oracle message text"); // If we couldn't successfully read the message text, we pick // something more descriptive, like "we couldn't read the message" // instead of just handing back an empty string... message = Res.GetString(Res.ADP_NoMessageAvailable, rc, -1); code = 0; } return new OracleException(message, code); } } static internal OracleException CreateException(int rc, OracleInternalConnection internalConnection) { int code; string message; using (NativeBuffer buf = new NativeBuffer_Exception(1000)) { int length = buf.Length; code = 0; int rcTemp = TracedNativeMethods.OraMTSOCIErrGet(ref code, buf, ref length); if (1 == rcTemp) { message = buf.PtrToStringAnsi(0, length); } else { Debug.Assert(false, "Failed to get oracle message text"); // If we couldn't successfully read the message text, we pick // something more descriptive, like "we couldn't read the message" // instead of just handing back an empty string... message = Res.GetString(Res.ADP_NoMessageAvailable, rc, rcTemp); code = 0; } // Make sure we doom the connection if its broken. if (ConnectionIsBroken(code)) { internalConnection.DoomThisConnection(); } return new OracleException(message, code); } } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] static internal void Check(OciErrorHandle errorHandle, int rc) { switch ((OCI.RETURNCODE)rc) { case OCI.RETURNCODE.OCI_ERROR: // -1: Bad programming by the customer. case OCI.RETURNCODE.OCI_NO_DATA: //100: Bad programming by the customer/expected error. throw ADP.OracleError(errorHandle, rc); case OCI.RETURNCODE.OCI_INVALID_HANDLE: // -2: Bad programming on my part. throw ADP.InvalidOperation(Res.GetString(Res.ADP_InternalError, rc)); default: if (rc < 0 || rc == (int)OCI.RETURNCODE.OCI_NEED_DATA || rc == (int)OCI.RETURNCODE.OCI_SUCCESS_WITH_INFO) throw ADP.Simple(Res.GetString(Res.ADP_UnexpectedReturnCode, rc.ToString(CultureInfo.CurrentCulture))); Debug.Assert(false, "Unexpected return code: " + rc); // shouldn't be here for any reason. break; } } static internal void Check(int rc, OracleInternalConnection internalConnection) { if (0 != rc) throw ADP.OracleError(rc, internalConnection); } [System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.SerializationFormatter)] override public void GetObjectData(SerializationInfo si, StreamingContext context) { if (null == si) { throw new ArgumentNullException("si"); } si.AddValue("code", _code, typeof(int)); base.GetObjectData(si, context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.Data; using System.Data.Common; using System.Diagnostics; using System.IO; using System.Globalization; using System.Runtime.CompilerServices; using System.Runtime.InteropServices; using System.Runtime.Serialization; using System.Runtime.ConstrainedExecution; //--------------------------------------------------------------------- // OracleException // // You end up with one of these when an OCI call fails unexpectedly. // [Serializable] sealed public class OracleException : System.Data.Common.DbException { private int _code; // runtime will call even if private... private OracleException(SerializationInfo si, StreamingContext sc) : base(si, sc) { _code = (int) si.GetValue("code", typeof(int)); HResult = HResults.OracleException; } private OracleException(string message, int code) : base(message) { _code = code; HResult = HResults.OracleException; } public int Code { get { return _code; } } static private bool ConnectionIsBroken(int code) { bool result; if (12500 <= code && code <= 12699) { // TNS errors result = true; } else { switch (code) { case 18: // max sessions exceeded case 19: // max session licenses exceeded case 24: // server is in single process mode case 28: // session has been killed case 436: // oracle isn't licensed case 1012: // not logged on. case 1033: // startup/shutdown in progress case 1034: // oracle not available case 1075: // currently logged on case 2392: // exceeded session limit on CPU usage,you are being logged off. case 2399: // exceeded maximum connect time, you are being logged off. case 3113: // end-of-file on communication channel case 3114: // not connected to ORACLE result = true; break; default: result = false; break; } } return result; } static internal OracleException CreateException(OciErrorHandle errorHandle, int rc) { int code; string message; using (NativeBuffer buf = new NativeBuffer_Exception(1000)) { if (null != errorHandle) { int record = 1; int rcTemp = TracedNativeMethods.OCIErrorGet( errorHandle, record, out code, buf ); if (0 == rcTemp) { message = errorHandle.PtrToString(buf); // For warning messages, revert back to the OCI7 routine to get // the text of the message. if (code != 0 && message.StartsWith("ORA-00000", StringComparison.Ordinal)) { rcTemp = TracedNativeMethods.oermsg((short)code, buf); if (0 == rcTemp) { message = errorHandle.PtrToString(buf); } } } else { Debug.Assert(false, "Failed to get oracle message text"); // If we couldn't successfully read the message text, we pick // something more descriptive, like "we couldn't read the message" // instead of just handing back an empty string... message = Res.GetString(Res.ADP_NoMessageAvailable, rc, rcTemp); code = 0; } // Make sure we doom the connection if its broken. if (ConnectionIsBroken(code)) { errorHandle.ConnectionIsBroken = true; } } else { Debug.Assert(false, "Failed to get oracle message text"); // If we couldn't successfully read the message text, we pick // something more descriptive, like "we couldn't read the message" // instead of just handing back an empty string... message = Res.GetString(Res.ADP_NoMessageAvailable, rc, -1); code = 0; } return new OracleException(message, code); } } static internal OracleException CreateException(int rc, OracleInternalConnection internalConnection) { int code; string message; using (NativeBuffer buf = new NativeBuffer_Exception(1000)) { int length = buf.Length; code = 0; int rcTemp = TracedNativeMethods.OraMTSOCIErrGet(ref code, buf, ref length); if (1 == rcTemp) { message = buf.PtrToStringAnsi(0, length); } else { Debug.Assert(false, "Failed to get oracle message text"); // If we couldn't successfully read the message text, we pick // something more descriptive, like "we couldn't read the message" // instead of just handing back an empty string... message = Res.GetString(Res.ADP_NoMessageAvailable, rc, rcTemp); code = 0; } // Make sure we doom the connection if its broken. if (ConnectionIsBroken(code)) { internalConnection.DoomThisConnection(); } return new OracleException(message, code); } } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] static internal void Check(OciErrorHandle errorHandle, int rc) { switch ((OCI.RETURNCODE)rc) { case OCI.RETURNCODE.OCI_ERROR: // -1: Bad programming by the customer. case OCI.RETURNCODE.OCI_NO_DATA: //100: Bad programming by the customer/expected error. throw ADP.OracleError(errorHandle, rc); case OCI.RETURNCODE.OCI_INVALID_HANDLE: // -2: Bad programming on my part. throw ADP.InvalidOperation(Res.GetString(Res.ADP_InternalError, rc)); default: if (rc < 0 || rc == (int)OCI.RETURNCODE.OCI_NEED_DATA || rc == (int)OCI.RETURNCODE.OCI_SUCCESS_WITH_INFO) throw ADP.Simple(Res.GetString(Res.ADP_UnexpectedReturnCode, rc.ToString(CultureInfo.CurrentCulture))); Debug.Assert(false, "Unexpected return code: " + rc); // shouldn't be here for any reason. break; } } static internal void Check(int rc, OracleInternalConnection internalConnection) { if (0 != rc) throw ADP.OracleError(rc, internalConnection); } [System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.SerializationFormatter)] override public void GetObjectData(SerializationInfo si, StreamingContext context) { if (null == si) { throw new ArgumentNullException("si"); } si.AddValue("code", _code, typeof(int)); base.GetObjectData(si, context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
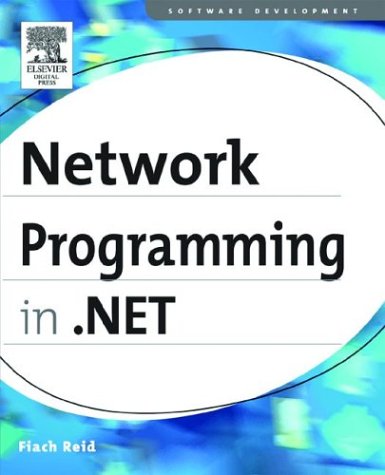
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridItemEventArgs.cs
- TransactionManager.cs
- _NestedMultipleAsyncResult.cs
- WindowsGrip.cs
- IdentitySection.cs
- XmlParserContext.cs
- OdbcException.cs
- InkCanvas.cs
- ColorConvertedBitmap.cs
- DocumentPageViewAutomationPeer.cs
- PropagatorResult.cs
- ButtonDesigner.cs
- FixedSOMGroup.cs
- SystemIPInterfaceStatistics.cs
- Compiler.cs
- RowToFieldTransformer.cs
- RuntimeIdentifierPropertyAttribute.cs
- ProcessHostMapPath.cs
- CookieParameter.cs
- ToolStripRenderEventArgs.cs
- CodePageEncoding.cs
- SoapFault.cs
- HttpHandlerAction.cs
- ValidateNames.cs
- DataColumn.cs
- OleDbDataReader.cs
- DataViewListener.cs
- DataKeyArray.cs
- ProxyWebPartManager.cs
- ToolStripContentPanel.cs
- Relationship.cs
- glyphs.cs
- __FastResourceComparer.cs
- PropertyDescriptors.cs
- XslTransformFileEditor.cs
- SeparatorAutomationPeer.cs
- Rect3D.cs
- RetrieveVirtualItemEventArgs.cs
- SqlUDTStorage.cs
- MetaForeignKeyColumn.cs
- MsmqPoisonMessageException.cs
- FunctionCommandText.cs
- WizardStepBase.cs
- PolyLineSegmentFigureLogic.cs
- WindowsGraphics2.cs
- ClockController.cs
- StrongNameMembershipCondition.cs
- BoundField.cs
- CharKeyFrameCollection.cs
- UIElementIsland.cs
- TextTreePropertyUndoUnit.cs
- Exceptions.cs
- CallbackValidator.cs
- StrokeCollection2.cs
- RenderData.cs
- TemplateNameScope.cs
- ObjectDataSourceMethodEventArgs.cs
- PermissionAttributes.cs
- HttpProtocolReflector.cs
- Int64AnimationBase.cs
- AncestorChangedEventArgs.cs
- SslStream.cs
- DataKey.cs
- MinimizableAttributeTypeConverter.cs
- RowCache.cs
- GetPageNumberCompletedEventArgs.cs
- ServiceThrottle.cs
- ScriptResourceAttribute.cs
- Schema.cs
- SkewTransform.cs
- XmlEncodedRawTextWriter.cs
- GridViewPageEventArgs.cs
- MethodImplAttribute.cs
- HtmlInputPassword.cs
- EntityDataSourceDesignerHelper.cs
- XmlSchemas.cs
- followingquery.cs
- ToolStripDesignerAvailabilityAttribute.cs
- HttpSessionStateWrapper.cs
- returneventsaver.cs
- DbXmlEnabledProviderManifest.cs
- XmlStringTable.cs
- _ConnectionGroup.cs
- OleDbConnection.cs
- PathFigureCollectionConverter.cs
- Vector3D.cs
- OperationCanceledException.cs
- SequentialWorkflowHeaderFooter.cs
- SEHException.cs
- SecurityUtils.cs
- AsymmetricKeyExchangeDeformatter.cs
- Rect3D.cs
- CompositionAdorner.cs
- WebRequestModuleElement.cs
- SqlProfileProvider.cs
- RC2CryptoServiceProvider.cs
- HttpModule.cs
- Subtree.cs
- CustomAssemblyResolver.cs
- TrustLevel.cs