Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / FontWeights.cs / 1 / FontWeights.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: Predefined FontWeight structures that correspond to common font weights. // // History: // 01/25/2005 mleonov - Created constants from FontWeight enum values. // //--------------------------------------------------------------------------- using System; using System.Globalization; namespace System.Windows { ////// FontWeights contains predefined font weight structures for common font weights. /// public static class FontWeights { ////// Predefined font weight : Thin. /// public static FontWeight Thin { get { return new FontWeight(100); } } ////// Predefined font weight : Extra-light. /// public static FontWeight ExtraLight { get { return new FontWeight(200); } } ////// Predefined font weight : Ultra-light. /// public static FontWeight UltraLight { get { return new FontWeight(200); } } ////// Predefined font weight : Light. /// public static FontWeight Light { get { return new FontWeight(300); } } ////// Predefined font weight : Normal. /// public static FontWeight Normal { get { return new FontWeight(400); } } ////// Predefined font weight : Regular. /// public static FontWeight Regular { get { return new FontWeight(400); } } ////// Predefined font weight : Medium. /// public static FontWeight Medium { get { return new FontWeight(500); } } ////// Predefined font weight : Demi-bold. /// public static FontWeight DemiBold { get { return new FontWeight(600); } } ////// Predefined font weight : Semi-bold. /// public static FontWeight SemiBold { get { return new FontWeight(600); } } ////// Predefined font weight : Bold. /// public static FontWeight Bold { get { return new FontWeight(700); } } ////// Predefined font weight : Extra-bold. /// public static FontWeight ExtraBold { get { return new FontWeight(800); } } ////// Predefined font weight : Ultra-bold. /// public static FontWeight UltraBold { get { return new FontWeight(800); } } ////// Predefined font weight : Black. /// public static FontWeight Black { get { return new FontWeight(900); } } ////// Predefined font weight : Heavy. /// public static FontWeight Heavy { get { return new FontWeight(900); } } ////// Predefined font weight : ExtraBlack. /// public static FontWeight ExtraBlack { get { return new FontWeight(950); } } ////// Predefined font weight : UltraBlack. /// public static FontWeight UltraBlack { get { return new FontWeight(950); } } internal static bool FontWeightStringToKnownWeight(string s, IFormatProvider provider, ref FontWeight fontWeight) { switch (s.Length) { case 4: if (s.Equals("Bold", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.Bold; return true; } if (s.Equals("Thin", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.Thin; return true; } break; case 5: if (s.Equals("Black", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.Black; return true; } if (s.Equals("Light", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.Light; return true; } if (s.Equals("Heavy", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.Heavy; return true; } break; case 6: if (s.Equals("Normal", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.Normal; return true; } if (s.Equals("Medium", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.Medium; return true; } break; case 7: if (s.Equals("Regular", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.Regular; return true; } break; case 8: if (s.Equals("SemiBold", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.SemiBold; return true; } if (s.Equals("DemiBold", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.DemiBold; return true; } break; case 9: if (s.Equals("ExtraBold", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.ExtraBold; return true; } if (s.Equals("UltraBold", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.UltraBold; return true; } break; case 10: if (s.Equals("ExtraLight", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.ExtraLight; return true; } if (s.Equals("UltraLight", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.UltraLight; return true; } if (s.Equals("ExtraBlack", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.ExtraBlack; return true; } if (s.Equals("UltraBlack", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.UltraBlack; return true; } break; } int weightValue; if (int.TryParse(s, NumberStyles.Integer, provider, out weightValue)) { fontWeight = FontWeight.FromOpenTypeWeight(weightValue); return true; } return false; } internal static bool FontWeightToString(int weight, out string convertedValue) { switch (weight) { case 100: convertedValue = "Thin"; return true; case 200: convertedValue = "ExtraLight"; return true; case 300: convertedValue = "Light"; return true; case 400: convertedValue = "Normal"; return true; case 500: convertedValue = "Medium"; return true; case 600: convertedValue = "SemiBold"; return true; case 700: convertedValue = "Bold"; return true; case 800: convertedValue = "ExtraBold"; return true; case 900: convertedValue = "Black"; return true; case 950: convertedValue = "ExtraBlack"; return true; } convertedValue = null; return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: Predefined FontWeight structures that correspond to common font weights. // // History: // 01/25/2005 mleonov - Created constants from FontWeight enum values. // //--------------------------------------------------------------------------- using System; using System.Globalization; namespace System.Windows { ////// FontWeights contains predefined font weight structures for common font weights. /// public static class FontWeights { ////// Predefined font weight : Thin. /// public static FontWeight Thin { get { return new FontWeight(100); } } ////// Predefined font weight : Extra-light. /// public static FontWeight ExtraLight { get { return new FontWeight(200); } } ////// Predefined font weight : Ultra-light. /// public static FontWeight UltraLight { get { return new FontWeight(200); } } ////// Predefined font weight : Light. /// public static FontWeight Light { get { return new FontWeight(300); } } ////// Predefined font weight : Normal. /// public static FontWeight Normal { get { return new FontWeight(400); } } ////// Predefined font weight : Regular. /// public static FontWeight Regular { get { return new FontWeight(400); } } ////// Predefined font weight : Medium. /// public static FontWeight Medium { get { return new FontWeight(500); } } ////// Predefined font weight : Demi-bold. /// public static FontWeight DemiBold { get { return new FontWeight(600); } } ////// Predefined font weight : Semi-bold. /// public static FontWeight SemiBold { get { return new FontWeight(600); } } ////// Predefined font weight : Bold. /// public static FontWeight Bold { get { return new FontWeight(700); } } ////// Predefined font weight : Extra-bold. /// public static FontWeight ExtraBold { get { return new FontWeight(800); } } ////// Predefined font weight : Ultra-bold. /// public static FontWeight UltraBold { get { return new FontWeight(800); } } ////// Predefined font weight : Black. /// public static FontWeight Black { get { return new FontWeight(900); } } ////// Predefined font weight : Heavy. /// public static FontWeight Heavy { get { return new FontWeight(900); } } ////// Predefined font weight : ExtraBlack. /// public static FontWeight ExtraBlack { get { return new FontWeight(950); } } ////// Predefined font weight : UltraBlack. /// public static FontWeight UltraBlack { get { return new FontWeight(950); } } internal static bool FontWeightStringToKnownWeight(string s, IFormatProvider provider, ref FontWeight fontWeight) { switch (s.Length) { case 4: if (s.Equals("Bold", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.Bold; return true; } if (s.Equals("Thin", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.Thin; return true; } break; case 5: if (s.Equals("Black", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.Black; return true; } if (s.Equals("Light", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.Light; return true; } if (s.Equals("Heavy", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.Heavy; return true; } break; case 6: if (s.Equals("Normal", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.Normal; return true; } if (s.Equals("Medium", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.Medium; return true; } break; case 7: if (s.Equals("Regular", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.Regular; return true; } break; case 8: if (s.Equals("SemiBold", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.SemiBold; return true; } if (s.Equals("DemiBold", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.DemiBold; return true; } break; case 9: if (s.Equals("ExtraBold", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.ExtraBold; return true; } if (s.Equals("UltraBold", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.UltraBold; return true; } break; case 10: if (s.Equals("ExtraLight", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.ExtraLight; return true; } if (s.Equals("UltraLight", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.UltraLight; return true; } if (s.Equals("ExtraBlack", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.ExtraBlack; return true; } if (s.Equals("UltraBlack", StringComparison.OrdinalIgnoreCase)) { fontWeight = FontWeights.UltraBlack; return true; } break; } int weightValue; if (int.TryParse(s, NumberStyles.Integer, provider, out weightValue)) { fontWeight = FontWeight.FromOpenTypeWeight(weightValue); return true; } return false; } internal static bool FontWeightToString(int weight, out string convertedValue) { switch (weight) { case 100: convertedValue = "Thin"; return true; case 200: convertedValue = "ExtraLight"; return true; case 300: convertedValue = "Light"; return true; case 400: convertedValue = "Normal"; return true; case 500: convertedValue = "Medium"; return true; case 600: convertedValue = "SemiBold"; return true; case 700: convertedValue = "Bold"; return true; case 800: convertedValue = "ExtraBold"; return true; case 900: convertedValue = "Black"; return true; case 950: convertedValue = "ExtraBlack"; return true; } convertedValue = null; return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
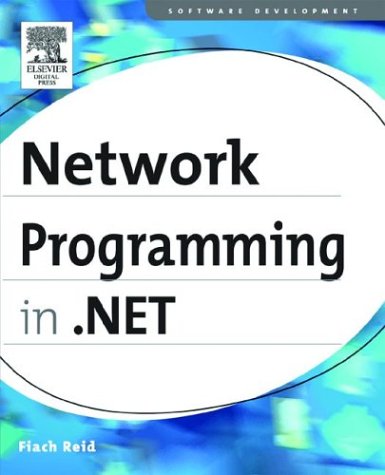
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridViewHeaderRowPresenterAutomationPeer.cs
- ResXResourceSet.cs
- ObjectSecurity.cs
- VectorCollection.cs
- _OSSOCK.cs
- SharedTcpTransportManager.cs
- DatePickerTextBox.cs
- BamlLocalizer.cs
- ApplicationDirectory.cs
- _ScatterGatherBuffers.cs
- StreamAsIStream.cs
- TableHeaderCell.cs
- WinEventQueueItem.cs
- MergeFailedEvent.cs
- CompilerLocalReference.cs
- InvokeHandlers.cs
- AsyncResult.cs
- ZoneLinkButton.cs
- InputScopeAttribute.cs
- LazyTextWriterCreator.cs
- QilInvokeEarlyBound.cs
- WebBrowserNavigatingEventHandler.cs
- DataGridViewTopRowAccessibleObject.cs
- Timeline.cs
- HighContrastHelper.cs
- XmlSchema.cs
- ControlIdConverter.cs
- JsonSerializer.cs
- VisualBrush.cs
- ActiveXContainer.cs
- ImageClickEventArgs.cs
- XamlTypeMapper.cs
- PenLineJoinValidation.cs
- SqlDataReader.cs
- CharacterMetricsDictionary.cs
- XPathDocumentIterator.cs
- XmlStreamStore.cs
- _TimerThread.cs
- UIElementParaClient.cs
- XmlSchemaExporter.cs
- NativeCompoundFileAPIs.cs
- IsolatedStorageFilePermission.cs
- RepeaterItem.cs
- CreateUserWizard.cs
- CombinedGeometry.cs
- NavigationService.cs
- PagerSettings.cs
- RowUpdatedEventArgs.cs
- XmlNamespaceDeclarationsAttribute.cs
- BitmapEffectDrawingContextWalker.cs
- PreProcessInputEventArgs.cs
- WindowsScroll.cs
- HwndSourceKeyboardInputSite.cs
- SubpageParaClient.cs
- SystemUdpStatistics.cs
- X509AsymmetricSecurityKey.cs
- NetCodeGroup.cs
- CollaborationHelperFunctions.cs
- HttpClientProtocol.cs
- CriticalFinalizerObject.cs
- Helpers.cs
- XmlArrayItemAttribute.cs
- ArrayListCollectionBase.cs
- SecurityTokenParametersEnumerable.cs
- ComponentManagerBroker.cs
- QilDataSource.cs
- PointConverter.cs
- TCPClient.cs
- Rect3D.cs
- MILUtilities.cs
- FilteredAttributeCollection.cs
- ImmutableCollection.cs
- XmlDataSourceNodeDescriptor.cs
- UnhandledExceptionEventArgs.cs
- HtmlInputSubmit.cs
- EmptyEnumerator.cs
- XPathSelfQuery.cs
- EntityDataSourceConfigureObjectContextPanel.cs
- ZoneButton.cs
- DispatcherObject.cs
- XslCompiledTransform.cs
- ExpandCollapseIsCheckedConverter.cs
- InvalidEnumArgumentException.cs
- TableColumnCollection.cs
- HtmlLink.cs
- XPathBuilder.cs
- Or.cs
- DbConnectionStringBuilder.cs
- _NetRes.cs
- InputEventArgs.cs
- FixedTextBuilder.cs
- Point4DValueSerializer.cs
- RelatedCurrencyManager.cs
- TimeoutException.cs
- RowsCopiedEventArgs.cs
- Camera.cs
- dbenumerator.cs
- ButtonFlatAdapter.cs
- ReachDocumentReferenceCollectionSerializer.cs
- FixedDocument.cs