Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / GlyphCache.cs / 2 / GlyphCache.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Security; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Composition; using System.Runtime.InteropServices; using MS.Internal; using MS.Internal.FontCache; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods = MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { ////// Master Glyph Cache class /// internal class GlyphCache { ////// Callback delegate. /// public delegate int CreateGlyphsCallbackDelegate( IntPtr /*CMilSlaveGlyphCache | CMilSlaveGlyphRun* */ nativeObject, IntPtr /*GLYPH_BITMAP_CREATE_REQUEST | GLYPH_GEOMETRY_CREATE_REQUEST* */ request, ushort isGeometryRequest); private DUCE.Resource _duceResource = new DUCE.Resource(); private SafeReversePInvokeWrapper _reversePInvokeWrapper; internal DUCE.ResourceHandle Handle { get { return _duceResource.Handle; } } // Service channel that serves for both // pre-commit and post-commit actions internal DUCE.Channel _channel; internal void RemoveFromChannel() { if (_channel != null) { _duceResource.ReleaseOnChannel(_channel); } } ////// /// /// ////// Critical - calls critical code /// [SecurityCritical] internal GlyphCache(DUCE.Channel channel) { _channel = channel; Debug.Assert(_channel != null); _duceResource.CreateOrAddRefOnChannel(_channel, DUCE.ResourceType.TYPE_GLYPHCACHE); SendCallbackEntryPoint(); } ////// Sends a callback pointer to this glyphcache for glyph generation requests. /// ////// Critical - This code sends a pointer to unmanaged code /// [SecurityCritical] private unsafe void SendCallbackEntryPoint() { _createGlyphBitmapsCallbackDelegate = new CreateGlyphsCallbackDelegate(FontCacheAccessor.CreateGlyphsCallback); IntPtr fcn = Marshal.GetFunctionPointerForDelegate(_createGlyphBitmapsCallbackDelegate); _reversePInvokeWrapper = new SafeReversePInvokeWrapper(fcn); DUCE.MILCMD_GLYPHCACHE_SETCALLBACK cmd; cmd.Type = MILCMD.MilCmdGlyphCacheSetCallback; cmd.Handle = Handle; // AddRef the reverse p-invoke wrapper while it is being transferred across the channel. There is a // small chance we would leak the wrapper. More specifically, if the app domain is shut down before // the wrapper is picked up by the composition engine. UnsafeNativeMethods.MILUnknown.AddRef(_reversePInvokeWrapper); cmd.CallbackPointer = (UInt64)_reversePInvokeWrapper.DangerousGetHandle(); _channel.SendSecurityCriticalCommand((byte*)&cmd, sizeof(DUCE.MILCMD_GLYPHCACHE_SETCALLBACK)); } private CreateGlyphsCallbackDelegate _createGlyphBitmapsCallbackDelegate; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Security; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Composition; using System.Runtime.InteropServices; using MS.Internal; using MS.Internal.FontCache; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods = MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { ////// Master Glyph Cache class /// internal class GlyphCache { ////// Callback delegate. /// public delegate int CreateGlyphsCallbackDelegate( IntPtr /*CMilSlaveGlyphCache | CMilSlaveGlyphRun* */ nativeObject, IntPtr /*GLYPH_BITMAP_CREATE_REQUEST | GLYPH_GEOMETRY_CREATE_REQUEST* */ request, ushort isGeometryRequest); private DUCE.Resource _duceResource = new DUCE.Resource(); private SafeReversePInvokeWrapper _reversePInvokeWrapper; internal DUCE.ResourceHandle Handle { get { return _duceResource.Handle; } } // Service channel that serves for both // pre-commit and post-commit actions internal DUCE.Channel _channel; internal void RemoveFromChannel() { if (_channel != null) { _duceResource.ReleaseOnChannel(_channel); } } ////// /// /// ////// Critical - calls critical code /// [SecurityCritical] internal GlyphCache(DUCE.Channel channel) { _channel = channel; Debug.Assert(_channel != null); _duceResource.CreateOrAddRefOnChannel(_channel, DUCE.ResourceType.TYPE_GLYPHCACHE); SendCallbackEntryPoint(); } ////// Sends a callback pointer to this glyphcache for glyph generation requests. /// ////// Critical - This code sends a pointer to unmanaged code /// [SecurityCritical] private unsafe void SendCallbackEntryPoint() { _createGlyphBitmapsCallbackDelegate = new CreateGlyphsCallbackDelegate(FontCacheAccessor.CreateGlyphsCallback); IntPtr fcn = Marshal.GetFunctionPointerForDelegate(_createGlyphBitmapsCallbackDelegate); _reversePInvokeWrapper = new SafeReversePInvokeWrapper(fcn); DUCE.MILCMD_GLYPHCACHE_SETCALLBACK cmd; cmd.Type = MILCMD.MilCmdGlyphCacheSetCallback; cmd.Handle = Handle; // AddRef the reverse p-invoke wrapper while it is being transferred across the channel. There is a // small chance we would leak the wrapper. More specifically, if the app domain is shut down before // the wrapper is picked up by the composition engine. UnsafeNativeMethods.MILUnknown.AddRef(_reversePInvokeWrapper); cmd.CallbackPointer = (UInt64)_reversePInvokeWrapper.DangerousGetHandle(); _channel.SendSecurityCriticalCommand((byte*)&cmd, sizeof(DUCE.MILCMD_GLYPHCACHE_SETCALLBACK)); } private CreateGlyphsCallbackDelegate _createGlyphBitmapsCallbackDelegate; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
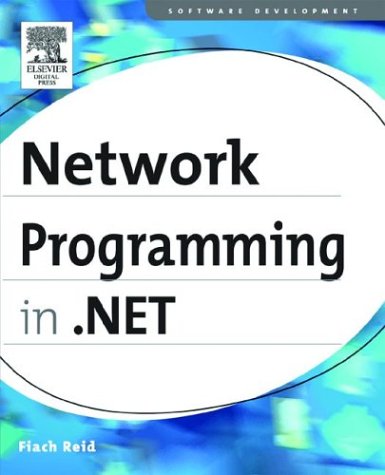
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WeakHashtable.cs
- CheckableControlBaseAdapter.cs
- TypedReference.cs
- MessagePropertyDescriptionCollection.cs
- HandleDictionary.cs
- SocketSettings.cs
- MessageDecoder.cs
- FormClosedEvent.cs
- HtmlInputReset.cs
- ToolStripCustomTypeDescriptor.cs
- SQLByte.cs
- RIPEMD160.cs
- PointCollectionValueSerializer.cs
- ProgressBarRenderer.cs
- RtfNavigator.cs
- ReaderWriterLock.cs
- ZipPackagePart.cs
- ServiceDocumentFormatter.cs
- CommandManager.cs
- DbDataSourceEnumerator.cs
- BasicCellRelation.cs
- ProcessInfo.cs
- TemplateBindingExtension.cs
- ConnectionOrientedTransportBindingElement.cs
- StorageMappingFragment.cs
- BaseCodePageEncoding.cs
- UserCancellationException.cs
- PackageDigitalSignatureManager.cs
- x509store.cs
- _Connection.cs
- XmlTextReaderImplHelpers.cs
- SHA512.cs
- IndividualDeviceConfig.cs
- SqlLiftWhereClauses.cs
- XamlValidatingReader.cs
- XmlSchemaImport.cs
- AttributeCollection.cs
- WebPartAddingEventArgs.cs
- DataGridColumn.cs
- SymbolEqualComparer.cs
- DataMemberListEditor.cs
- ContentElementAutomationPeer.cs
- TextParagraphCache.cs
- Point.cs
- HtmlTitle.cs
- FlowLayoutPanel.cs
- ServiceModelReg.cs
- DesignerDataSchemaClass.cs
- LinkConverter.cs
- KeyFrames.cs
- LineSegment.cs
- GridViewDeleteEventArgs.cs
- StaticSiteMapProvider.cs
- ButtonBaseAdapter.cs
- TableLayoutPanel.cs
- ClonableStack.cs
- SamlAuthenticationStatement.cs
- ArgumentNullException.cs
- HttpProcessUtility.cs
- NgenServicingAttributes.cs
- IdentityVerifier.cs
- TranslateTransform3D.cs
- PriorityBinding.cs
- IChannel.cs
- HttpModuleActionCollection.cs
- MulticastOption.cs
- CookielessHelper.cs
- CngKeyBlobFormat.cs
- ExpressionList.cs
- URI.cs
- UInt16Converter.cs
- SmiMetaDataProperty.cs
- SymbolTable.cs
- SymmetricSecurityProtocol.cs
- Quaternion.cs
- InvariantComparer.cs
- PageTheme.cs
- TextRangeEdit.cs
- X509ClientCertificateCredentialsElement.cs
- SafeThemeHandle.cs
- ContextQuery.cs
- XmlDictionaryReader.cs
- DbUpdateCommandTree.cs
- DeferredSelectedIndexReference.cs
- TempFiles.cs
- PixelShader.cs
- Quaternion.cs
- PropertyPathConverter.cs
- InfoCardSymmetricAlgorithm.cs
- DateTimePicker.cs
- ToolboxComponentsCreatingEventArgs.cs
- ReadOnlyCollection.cs
- SystemException.cs
- TextDecorationCollection.cs
- OSFeature.cs
- Converter.cs
- DetailsViewInsertedEventArgs.cs
- ParameterRetriever.cs
- XmlElement.cs
- StorageAssociationSetMapping.cs