Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Automation / Peers / ContentElementAutomationPeer.cs / 1 / ContentElementAutomationPeer.cs
//---------------------------------------------------------------------------- // // File: ContentElementAutomationPeer.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Automation element for ContentElements // //--------------------------------------------------------------------------- using System; // Object using System.Collections.Generic; // Listusing System.Windows.Input; // AccessKeyManager using MS.Internal.PresentationCore; // SR namespace System.Windows.Automation.Peers { /// public class ContentElementAutomationPeer : AutomationPeer { /// public ContentElementAutomationPeer(ContentElement owner) { if (owner == null) { throw new ArgumentNullException("owner"); } _owner = owner; } /// public ContentElement Owner { get { return _owner; } } /// /// This static helper creates an AutomationPeer for the specified element and /// caches it - that means the created peer is going to live long and shadow the /// element for its lifetime. The peer will be used by Automation to proxy the element, and /// to fire events to the Automation when something happens with the element. /// The created peer is returned from this method and also from subsequent calls to this method /// and public static AutomationPeer CreatePeerForElement(ContentElement element) { if (element == null) { throw new ArgumentNullException("element"); } return element.CreateAutomationPeer(); } /// public static AutomationPeer FromElement(ContentElement element) { if (element == null) { throw new ArgumentNullException("element"); } return element.GetAutomationPeer(); } ///. The type of the peer is determined by the /// virtual callback. If FrameworkContentElement does not /// implement the callback, there will be no peer and this method will return 'null' (in other /// words, there is no such thing as a 'default peer'). /// /// override protected List/// GetChildrenCore() { return null; } /// override public object GetPattern(PatternInterface patternInterface) { return null; } /// /// protected override AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Custom; } ////// /// protected override string GetAutomationIdCore() { return AutomationProperties.GetAutomationId(_owner); } ////// /// protected override string GetNameCore() { return AutomationProperties.GetName(_owner); } ////// /// protected override string GetHelpTextCore() { return AutomationProperties.GetHelpText(_owner); } ////// /// override protected Rect GetBoundingRectangleCore() { return Rect.Empty; } ////// /// override protected bool IsOffscreenCore() { return true; } ////// /// override protected AutomationOrientation GetOrientationCore() { return AutomationOrientation.None; } ////// /// override protected string GetItemTypeCore() { return string.Empty; } ////// /// override protected string GetClassNameCore() { return string.Empty; } ////// /// override protected string GetItemStatusCore() { return string.Empty; } ////// /// override protected bool IsRequiredForFormCore() { return false; } ////// /// override protected bool IsKeyboardFocusableCore() { return Keyboard.IsFocusable(_owner); } ////// /// override protected bool HasKeyboardFocusCore() { return _owner.IsKeyboardFocused; } ////// /// override protected bool IsEnabledCore() { return _owner.IsEnabled; } ////// /// override protected bool IsPasswordCore() { return false; } ////// /// override protected bool IsContentElementCore() { return true; } ////// /// override protected bool IsControlElementCore() { return false; } ////// /// override protected AutomationPeer GetLabeledByCore() { return null; } ////// /// override protected string GetAcceleratorKeyCore() { return string.Empty; } ////// /// override protected string GetAccessKeyCore() { return AccessKeyManager.InternalGetAccessKeyCharacter(_owner); } ////// /// override protected Point GetClickablePointCore() { return new Point(double.NaN, double.NaN); } ////// /// override protected void SetFocusCore() { if (!_owner.Focus()) throw new InvalidOperationException(SR.Get(SRID.SetFocusFailed)); } private ContentElement _owner; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: ContentElementAutomationPeer.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Automation element for ContentElements // //--------------------------------------------------------------------------- using System; // Object using System.Collections.Generic; // List/// using System.Windows.Input; // AccessKeyManager using MS.Internal.PresentationCore; // SR namespace System.Windows.Automation.Peers { /// public class ContentElementAutomationPeer : AutomationPeer { /// public ContentElementAutomationPeer(ContentElement owner) { if (owner == null) { throw new ArgumentNullException("owner"); } _owner = owner; } /// public ContentElement Owner { get { return _owner; } } /// /// This static helper creates an AutomationPeer for the specified element and /// caches it - that means the created peer is going to live long and shadow the /// element for its lifetime. The peer will be used by Automation to proxy the element, and /// to fire events to the Automation when something happens with the element. /// The created peer is returned from this method and also from subsequent calls to this method /// and public static AutomationPeer CreatePeerForElement(ContentElement element) { if (element == null) { throw new ArgumentNullException("element"); } return element.CreateAutomationPeer(); } /// public static AutomationPeer FromElement(ContentElement element) { if (element == null) { throw new ArgumentNullException("element"); } return element.GetAutomationPeer(); } ///. The type of the peer is determined by the /// virtual callback. If FrameworkContentElement does not /// implement the callback, there will be no peer and this method will return 'null' (in other /// words, there is no such thing as a 'default peer'). /// /// override protected List/// GetChildrenCore() { return null; } /// override public object GetPattern(PatternInterface patternInterface) { return null; } /// /// protected override AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Custom; } ////// /// protected override string GetAutomationIdCore() { return AutomationProperties.GetAutomationId(_owner); } ////// /// protected override string GetNameCore() { return AutomationProperties.GetName(_owner); } ////// /// protected override string GetHelpTextCore() { return AutomationProperties.GetHelpText(_owner); } ////// /// override protected Rect GetBoundingRectangleCore() { return Rect.Empty; } ////// /// override protected bool IsOffscreenCore() { return true; } ////// /// override protected AutomationOrientation GetOrientationCore() { return AutomationOrientation.None; } ////// /// override protected string GetItemTypeCore() { return string.Empty; } ////// /// override protected string GetClassNameCore() { return string.Empty; } ////// /// override protected string GetItemStatusCore() { return string.Empty; } ////// /// override protected bool IsRequiredForFormCore() { return false; } ////// /// override protected bool IsKeyboardFocusableCore() { return Keyboard.IsFocusable(_owner); } ////// /// override protected bool HasKeyboardFocusCore() { return _owner.IsKeyboardFocused; } ////// /// override protected bool IsEnabledCore() { return _owner.IsEnabled; } ////// /// override protected bool IsPasswordCore() { return false; } ////// /// override protected bool IsContentElementCore() { return true; } ////// /// override protected bool IsControlElementCore() { return false; } ////// /// override protected AutomationPeer GetLabeledByCore() { return null; } ////// /// override protected string GetAcceleratorKeyCore() { return string.Empty; } ////// /// override protected string GetAccessKeyCore() { return AccessKeyManager.InternalGetAccessKeyCharacter(_owner); } ////// /// override protected Point GetClickablePointCore() { return new Point(double.NaN, double.NaN); } ////// /// override protected void SetFocusCore() { if (!_owner.Focus()) throw new InvalidOperationException(SR.Get(SRID.SetFocusFailed)); } private ContentElement _owner; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
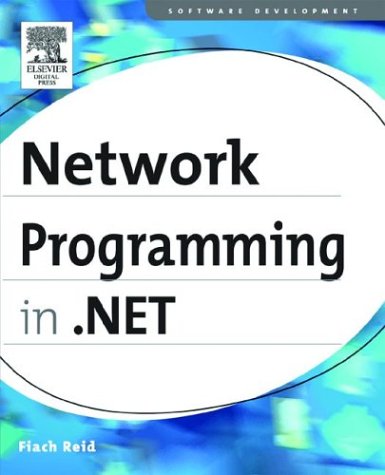
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UpdatePanelTriggerCollection.cs
- AssertUtility.cs
- DBPropSet.cs
- HtmlListAdapter.cs
- ValueUtilsSmi.cs
- BasicHttpSecurityMode.cs
- CollectionContainer.cs
- x509utils.cs
- Floater.cs
- HttpConfigurationSystem.cs
- ProtectedConfigurationSection.cs
- IEnumerable.cs
- ThousandthOfEmRealDoubles.cs
- TemplateControlCodeDomTreeGenerator.cs
- _NetRes.cs
- DataGridSortCommandEventArgs.cs
- XmlDocumentFragment.cs
- BypassElementCollection.cs
- NetCodeGroup.cs
- TemplatePartAttribute.cs
- NonParentingControl.cs
- GroupQuery.cs
- ParentUndoUnit.cs
- Evidence.cs
- MsmqMessageProperty.cs
- SimpleModelProvider.cs
- PolyBezierSegmentFigureLogic.cs
- ProfileModule.cs
- StrokeCollection.cs
- WhitespaceRule.cs
- TeredoHelper.cs
- ObjectNotFoundException.cs
- InternalDuplexChannelListener.cs
- CssClassPropertyAttribute.cs
- AddInBase.cs
- ILGen.cs
- FocusManager.cs
- SqlTopReducer.cs
- Border.cs
- LocalizationCodeDomSerializer.cs
- WebPartDescriptionCollection.cs
- BlockingCollection.cs
- CompilerGeneratedAttribute.cs
- CharAnimationUsingKeyFrames.cs
- rsa.cs
- SafeLocalMemHandle.cs
- SizeF.cs
- SqlXmlStorage.cs
- CompilationSection.cs
- _LocalDataStore.cs
- PageHandlerFactory.cs
- Thickness.cs
- CustomPopupPlacement.cs
- IgnoreFlushAndCloseStream.cs
- ActivityDesignerResources.cs
- DataObjectFieldAttribute.cs
- HttpModuleActionCollection.cs
- SystemNetHelpers.cs
- UpdateCompiler.cs
- UnauthorizedAccessException.cs
- PenThreadWorker.cs
- ThrowOnMultipleAssignment.cs
- WebExceptionStatus.cs
- RelationshipEndMember.cs
- SessionStateItemCollection.cs
- EditorPart.cs
- InfoCardRSAOAEPKeyExchangeFormatter.cs
- InteropBitmapSource.cs
- StateMachine.cs
- PropertyEmitter.cs
- AsyncCompletedEventArgs.cs
- DataControlButton.cs
- ListParagraph.cs
- VersionedStreamOwner.cs
- QueueProcessor.cs
- CfgArc.cs
- ClientSettingsSection.cs
- ComplexTypeEmitter.cs
- Pkcs7Recipient.cs
- Types.cs
- ICspAsymmetricAlgorithm.cs
- IndexExpression.cs
- X509ThumbprintKeyIdentifierClause.cs
- CodePrimitiveExpression.cs
- KeyValueSerializer.cs
- DataBinding.cs
- SqlError.cs
- TraceData.cs
- ValidationPropertyAttribute.cs
- DragStartedEventArgs.cs
- TreeViewTemplateSelector.cs
- XmlUtilWriter.cs
- InkCanvasAutomationPeer.cs
- WebBrowserProgressChangedEventHandler.cs
- COM2ColorConverter.cs
- OdbcErrorCollection.cs
- WebPartConnectionsCancelEventArgs.cs
- MimeObjectFactory.cs
- X509Utils.cs
- SafeUserTokenHandle.cs