Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / WebExceptionStatus.cs / 1305376 / WebExceptionStatus.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { ////// public enum WebExceptionStatus { ////// Specifies the status of a network request. /// ////// Success = 0, ////// No error was encountered. /// ////// NameResolutionFailure = 1, ////// The name resolver service could not resolve the host name. /// ////// ConnectFailure = 2, ////// The remote service point could not be contacted at the transport level. /// ////// ReceiveFailure = 3, ////// A complete response was not received from the remote server. /// ////// SendFailure = 4, ////// A complete request could not be sent to the remote server. /// ////// /// PipelineFailure = 5, ////// RequestCanceled = 6, ////// The request was cancelled. /// ////// ProtocolError = 7, ////// The response received from the server was complete but indicated a /// protocol-level error. For example, an HTTP protocol error such as 401 Access /// Denied would use this status. /// ////// ConnectionClosed = 8, ////// The connection was prematurely closed. /// ////// TrustFailure = 9, ////// A server certificate could not be validated. /// ////// SecureChannelFailure = 10, ////// An error occurred in a secure channel link. /// ////// ServerProtocolViolation = 11, ///[To be supplied.] ////// KeepAliveFailure = 12, ///[To be supplied.] ////// Pending = 13, ///[To be supplied.] ////// Timeout = 14, ///[To be supplied.] ////// ProxyNameResolutionFailure = 15, ////// Similar to NameResolution Failure, but for proxy failures. /// ////// UnknownError = 16, ///[To be supplied.] ////// MessageLengthLimitExceeded = 17, // // A request could be served from Cache but was not found and effective CachePolicy=CacheOnly // CacheEntryNotFound = 18, // // A request is not suitable for caching and effective CachePolicy=CacheOnly // RequestProhibitedByCachePolicy = 19, // // The proxy script (or other proxy logic) declined to provide proxy info, effectively blocking the request. // RequestProhibitedByProxy = 20, // !! If new values are added, increase the size of the s_Mapping array below to the largest value + 1. }; // enum WebExceptionStatus // Mapping from enum value to error message. internal static class WebExceptionMapping { private static readonly string[] s_Mapping = new string[21]; internal static string GetWebStatusString(WebExceptionStatus status) { int statusInt = (int) status; if (statusInt >= s_Mapping.Length || statusInt < 0) { throw new InternalException(); } string message = s_Mapping[statusInt]; if (message == null) { message = "net_webstatus_" + status.ToString(); s_Mapping[statusInt] = message; } return message; } } } // namespace System.Net // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Sending the request to the server or receiving the response from it, /// required handling a message that exceeded the specified limit. /// ///
Link Menu
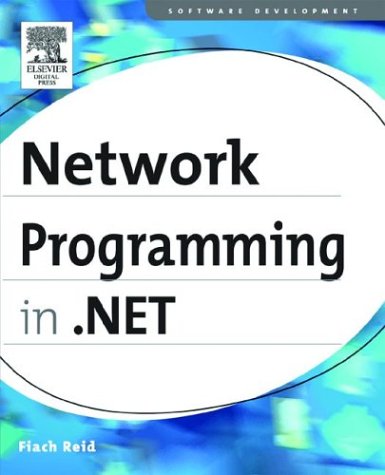
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RuleConditionDialog.Designer.cs
- ScriptReferenceBase.cs
- SqlDataSourceSelectingEventArgs.cs
- SkipStoryboardToFill.cs
- Rotation3DKeyFrameCollection.cs
- XmlILIndex.cs
- ImageListImage.cs
- ArcSegment.cs
- GlobalEventManager.cs
- SamlNameIdentifierClaimResource.cs
- Single.cs
- MultiByteCodec.cs
- RtfControlWordInfo.cs
- OleDbEnumerator.cs
- MenuAdapter.cs
- ContentDisposition.cs
- PagesSection.cs
- EntityStoreSchemaGenerator.cs
- BuilderPropertyEntry.cs
- TypeGeneratedEventArgs.cs
- ListChangedEventArgs.cs
- Util.cs
- CellConstant.cs
- SystemTcpStatistics.cs
- TagMapInfo.cs
- SplitterPanelDesigner.cs
- CompilationUtil.cs
- MobileErrorInfo.cs
- PathParser.cs
- DataComponentGenerator.cs
- OleDbInfoMessageEvent.cs
- KeyedCollection.cs
- PathSegment.cs
- DataSourceControlBuilder.cs
- XPathNodeIterator.cs
- QueryStatement.cs
- BamlCollectionHolder.cs
- ApplicationFileCodeDomTreeGenerator.cs
- MsmqActivation.cs
- Transform3DCollection.cs
- StreamSecurityUpgradeInitiator.cs
- ToolStripSplitStackLayout.cs
- NavigationProgressEventArgs.cs
- WebPartDisplayModeCancelEventArgs.cs
- CrossContextChannel.cs
- TableCell.cs
- DragSelectionMessageFilter.cs
- MetadataArtifactLoaderCompositeResource.cs
- AlternateView.cs
- CryptoApi.cs
- ButtonFlatAdapter.cs
- DataTemplate.cs
- ObjectDataSourceEventArgs.cs
- TcpHostedTransportConfiguration.cs
- ADMembershipProvider.cs
- ModelVisual3D.cs
- ContentOnlyMessage.cs
- InputScopeConverter.cs
- CreateUserErrorEventArgs.cs
- ObjectSet.cs
- EllipticalNodeOperations.cs
- XmlSchemaObjectCollection.cs
- CollectionBuilder.cs
- WebPartConnectionsCancelEventArgs.cs
- DataGridAddNewRow.cs
- EdmRelationshipRoleAttribute.cs
- BuildManager.cs
- ChtmlMobileTextWriter.cs
- SiteMapProvider.cs
- StagingAreaInputItem.cs
- Matrix3D.cs
- SqlRowUpdatingEvent.cs
- ReadOnlyDictionary.cs
- LOSFormatter.cs
- OleDbConnectionInternal.cs
- OdbcUtils.cs
- RIPEMD160.cs
- QuadraticBezierSegment.cs
- TrackingLocation.cs
- BasicViewGenerator.cs
- panel.cs
- isolationinterop.cs
- ImageFormatConverter.cs
- DictionaryCustomTypeDescriptor.cs
- DropShadowEffect.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- SaveFileDialog.cs
- StrokeIntersection.cs
- DataGridRow.cs
- FieldNameLookup.cs
- CallSiteOps.cs
- ModuleConfigurationInfo.cs
- DetailsViewRowCollection.cs
- TransformationRules.cs
- peernodeimplementation.cs
- CommandPlan.cs
- serverconfig.cs
- EqualityComparer.cs
- HttpCookiesSection.cs
- _ConnectOverlappedAsyncResult.cs