Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / Structures / CellConstant.cs / 1 / CellConstant.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Collections.Generic; using System.Text; using System.Data.Mapping.ViewGeneration.CqlGeneration; using System.Diagnostics; using System.Data.Metadata.Edm; using System.Data.Mapping.ViewGeneration.Utils; namespace System.Data.Mapping.ViewGeneration.Structures { // This class denotes a constant that can be stored in multiconstants or // projected in fields internal class CellConstant : InternalBase { protected CellConstant() { // Private default constuctor so that no can construct this // object externally other than via subclasses } #region Fields // Two constants to denote nulls and undefined values // Order is important -- it is guaranteed by C# (see Section 10.4.5.1) internal static readonly IEqualityComparerEqualityComparer = new CellConstantComparer(); internal static readonly CellConstant Null = new CellConstant(); internal static readonly CellConstant Undefined = new CellConstant(); internal static readonly CellConstant NotNull = NegatedCellConstant.CreateNotNull(); //Represents scalar constants within a finite set that are not specified explicitly in the domain // Currently only used as a Sentinel node to prevent expression optimization internal static readonly CellConstant AllOtherConstants = new CellConstant(); #endregion #region Methods internal bool IsNull() { return EqualityComparer.Equals(this, CellConstant.Null); } internal bool IsUndefined() { return EqualityComparer.Equals(this, CellConstant.Undefined); } internal virtual bool IsNotNull() { // Provide a default implementation and let the subclasses // override it return false; // NegatedCellConstant handles it } // effects: Returns true if this contains not null internal virtual bool HasNotNull() { // Provide a default implementation and let the subclasses // override it return false; // NegatedCellConstant handles it } // effects: Modifies builder to contain the appropriate expression // corresponding to this. outputMember is the member to which this constant is directed. // blockAlias is the alias for the SELECT FROM WHERE block where this constant is being projected // if not null. Returns the modified stringbuilder internal virtual StringBuilder AsCql(StringBuilder builder, MemberPath outputMember, string blockAlias) { // Constants will never be simply entity sets -- so we can use Property on the path // constType is the type of this we need to cast to EdmType constType = Helper.GetModelTypeUsage(outputMember.LastMember).EdmType; Debug.Assert(this != Undefined && this != NotNull, "Generating Cql for undefined slots or not-nulls?"); Debug.Assert(this == Null, "Probably forgot to override this method in a subclass"); // need to cast builder.Append("CAST(NULL AS "); CqlWriter.AppendEscapedTypeName(builder, constType); builder.Append(')'); return builder; } // effects: Returns true if right has the same contents as this protected virtual int GetHash() { Debug.Assert(this == Null || this == Undefined || this == AllOtherConstants, "CellConstants can only be Null, Undefined, or AllOtherConstants"); return 0; } // effects: Returns true if right has the same contents as this protected virtual bool IsEqualTo(CellConstant right) { Debug.Assert(this == Null || this == Undefined || this == AllOtherConstants, "CellConstants can only be Null, Undefined, or AllOtherConstants"); return this == right; } public override bool Equals(object obj) { CellConstant cellConst = obj as CellConstant; if (cellConst == null) { return false; } else { return IsEqualTo(cellConst); } } public override int GetHashCode() { return base.GetHashCode(); } internal virtual string ToUserString() { StringBuilder builder = new StringBuilder(); InternalToString(builder, false); return builder.ToString(); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal static void ConstantsToUserString(StringBuilder builder, Set constants) { bool isFirst = true; foreach (CellConstant constant in constants) { if (isFirst == false) { builder.Append(System.Data.Entity.Strings.ViewGen_CommaBlank); } isFirst = false; string constrStr = constant.ToUserString(); builder.Append(constrStr); } } private void InternalToString(StringBuilder builder, bool isInvariant) { string result; if (this == Null) { result = isInvariant ? "NULL" : System.Data.Entity.Strings.ViewGen_Null; } else if (this == Undefined) { Debug.Assert(isInvariant, "We should not emit a message about Undefined constants to the user"); result = "?"; } else if (this == NotNull) { result = isInvariant? "NOT_NULL": System.Data.Entity.Strings.ViewGen_NotNull; } else if (this == AllOtherConstants){ result = "AllOtherConstants"; } else { Debug.Fail("No other constants handled by this class"); result = isInvariant? "FAILURE": System.Data.Entity.Strings.ViewGen_Failure; } builder.Append(result); } internal override void ToCompactString(StringBuilder builder) { InternalToString(builder, true); } #endregion #region Comparer class private class CellConstantComparer : IEqualityComparer { public bool Equals(CellConstant left, CellConstant right) { // Quick check with references if (object.ReferenceEquals(left, right)) { // Gets the Null and Undefined case as well return true; } // One of them is non-null at least. So if the other one is // null, we cannot be equal if (left == null || right == null) { return false; } // Both are non-null at this point return left.IsEqualTo(right); } public int GetHashCode(CellConstant key) { EntityUtil.CheckArgumentNull(key, "key"); return key.GetHash(); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Collections.Generic; using System.Text; using System.Data.Mapping.ViewGeneration.CqlGeneration; using System.Diagnostics; using System.Data.Metadata.Edm; using System.Data.Mapping.ViewGeneration.Utils; namespace System.Data.Mapping.ViewGeneration.Structures { // This class denotes a constant that can be stored in multiconstants or // projected in fields internal class CellConstant : InternalBase { protected CellConstant() { // Private default constuctor so that no can construct this // object externally other than via subclasses } #region Fields // Two constants to denote nulls and undefined values // Order is important -- it is guaranteed by C# (see Section 10.4.5.1) internal static readonly IEqualityComparerEqualityComparer = new CellConstantComparer(); internal static readonly CellConstant Null = new CellConstant(); internal static readonly CellConstant Undefined = new CellConstant(); internal static readonly CellConstant NotNull = NegatedCellConstant.CreateNotNull(); //Represents scalar constants within a finite set that are not specified explicitly in the domain // Currently only used as a Sentinel node to prevent expression optimization internal static readonly CellConstant AllOtherConstants = new CellConstant(); #endregion #region Methods internal bool IsNull() { return EqualityComparer.Equals(this, CellConstant.Null); } internal bool IsUndefined() { return EqualityComparer.Equals(this, CellConstant.Undefined); } internal virtual bool IsNotNull() { // Provide a default implementation and let the subclasses // override it return false; // NegatedCellConstant handles it } // effects: Returns true if this contains not null internal virtual bool HasNotNull() { // Provide a default implementation and let the subclasses // override it return false; // NegatedCellConstant handles it } // effects: Modifies builder to contain the appropriate expression // corresponding to this. outputMember is the member to which this constant is directed. // blockAlias is the alias for the SELECT FROM WHERE block where this constant is being projected // if not null. Returns the modified stringbuilder internal virtual StringBuilder AsCql(StringBuilder builder, MemberPath outputMember, string blockAlias) { // Constants will never be simply entity sets -- so we can use Property on the path // constType is the type of this we need to cast to EdmType constType = Helper.GetModelTypeUsage(outputMember.LastMember).EdmType; Debug.Assert(this != Undefined && this != NotNull, "Generating Cql for undefined slots or not-nulls?"); Debug.Assert(this == Null, "Probably forgot to override this method in a subclass"); // need to cast builder.Append("CAST(NULL AS "); CqlWriter.AppendEscapedTypeName(builder, constType); builder.Append(')'); return builder; } // effects: Returns true if right has the same contents as this protected virtual int GetHash() { Debug.Assert(this == Null || this == Undefined || this == AllOtherConstants, "CellConstants can only be Null, Undefined, or AllOtherConstants"); return 0; } // effects: Returns true if right has the same contents as this protected virtual bool IsEqualTo(CellConstant right) { Debug.Assert(this == Null || this == Undefined || this == AllOtherConstants, "CellConstants can only be Null, Undefined, or AllOtherConstants"); return this == right; } public override bool Equals(object obj) { CellConstant cellConst = obj as CellConstant; if (cellConst == null) { return false; } else { return IsEqualTo(cellConst); } } public override int GetHashCode() { return base.GetHashCode(); } internal virtual string ToUserString() { StringBuilder builder = new StringBuilder(); InternalToString(builder, false); return builder.ToString(); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal static void ConstantsToUserString(StringBuilder builder, Set constants) { bool isFirst = true; foreach (CellConstant constant in constants) { if (isFirst == false) { builder.Append(System.Data.Entity.Strings.ViewGen_CommaBlank); } isFirst = false; string constrStr = constant.ToUserString(); builder.Append(constrStr); } } private void InternalToString(StringBuilder builder, bool isInvariant) { string result; if (this == Null) { result = isInvariant ? "NULL" : System.Data.Entity.Strings.ViewGen_Null; } else if (this == Undefined) { Debug.Assert(isInvariant, "We should not emit a message about Undefined constants to the user"); result = "?"; } else if (this == NotNull) { result = isInvariant? "NOT_NULL": System.Data.Entity.Strings.ViewGen_NotNull; } else if (this == AllOtherConstants){ result = "AllOtherConstants"; } else { Debug.Fail("No other constants handled by this class"); result = isInvariant? "FAILURE": System.Data.Entity.Strings.ViewGen_Failure; } builder.Append(result); } internal override void ToCompactString(StringBuilder builder) { InternalToString(builder, true); } #endregion #region Comparer class private class CellConstantComparer : IEqualityComparer { public bool Equals(CellConstant left, CellConstant right) { // Quick check with references if (object.ReferenceEquals(left, right)) { // Gets the Null and Undefined case as well return true; } // One of them is non-null at least. So if the other one is // null, we cannot be equal if (left == null || right == null) { return false; } // Both are non-null at this point return left.IsEqualTo(right); } public int GetHashCode(CellConstant key) { EntityUtil.CheckArgumentNull(key, "key"); return key.GetHash(); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
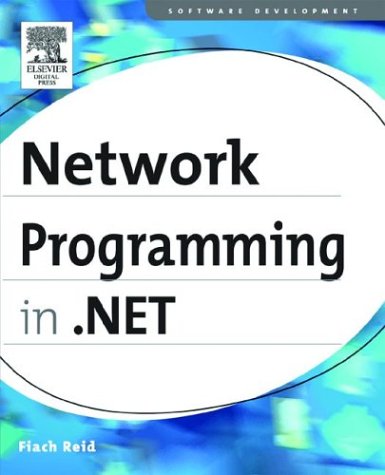
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VirtualPathUtility.cs
- XmlAnyAttributeAttribute.cs
- NeutralResourcesLanguageAttribute.cs
- AllowedAudienceUriElement.cs
- WrappedReader.cs
- DbConnectionStringCommon.cs
- CallContext.cs
- GridEntry.cs
- CertificateElement.cs
- ITextView.cs
- DecoratedNameAttribute.cs
- XmlSerializableWriter.cs
- _Events.cs
- Quaternion.cs
- XmlSchemaObjectCollection.cs
- GeneralTransform3DCollection.cs
- RoleGroupCollection.cs
- WebBrowserNavigatedEventHandler.cs
- SqlFlattener.cs
- AccessibilityHelperForVista.cs
- SqlBuilder.cs
- InputBinder.cs
- AnnotationResourceCollection.cs
- StaticTextPointer.cs
- DataGridAutoFormat.cs
- DbConnectionInternal.cs
- XmlDownloadManager.cs
- XdrBuilder.cs
- VarRemapper.cs
- HttpCookie.cs
- SqlXmlStorage.cs
- ProcessModelInfo.cs
- XmlWriter.cs
- HatchBrush.cs
- TimeIntervalCollection.cs
- SimpleNameService.cs
- XLinq.cs
- QilNode.cs
- DesignTableCollection.cs
- TransactionFlowBindingElementImporter.cs
- shaper.cs
- GeneralTransform.cs
- TextureBrush.cs
- MediaElementAutomationPeer.cs
- BitmapEffect.cs
- ActivityTypeDesigner.xaml.cs
- listitem.cs
- PreviewKeyDownEventArgs.cs
- TreeViewItem.cs
- ToolboxItemFilterAttribute.cs
- TileBrush.cs
- CookieParameter.cs
- ProcessStartInfo.cs
- XmlEventCache.cs
- _emptywebproxy.cs
- StorageFunctionMapping.cs
- XmlSchemaDocumentation.cs
- PaginationProgressEventArgs.cs
- TrustManagerPromptUI.cs
- PolicyDesigner.cs
- PropertyEmitterBase.cs
- CommentEmitter.cs
- AlternationConverter.cs
- WebHttpEndpointElement.cs
- ResourceDictionaryCollection.cs
- BitStream.cs
- RepeatButton.cs
- StateItem.cs
- Policy.cs
- StylusPointCollection.cs
- DaylightTime.cs
- SqlGatherConsumedAliases.cs
- DynamicResourceExtensionConverter.cs
- SecUtil.cs
- TreeViewCancelEvent.cs
- MappingSource.cs
- RulePatternOps.cs
- SiteMapNodeItemEventArgs.cs
- ComMethodElement.cs
- TemplatePartAttribute.cs
- Solver.cs
- ProcessRequestArgs.cs
- X509Chain.cs
- SimpleTypesSurrogate.cs
- TimelineGroup.cs
- MetadataHelper.cs
- NetworkAddressChange.cs
- SiteMapPathDesigner.cs
- BitmapScalingModeValidation.cs
- TeredoHelper.cs
- DeflateEmulationStream.cs
- DataBoundLiteralControl.cs
- XmlSchemaDocumentation.cs
- XmlILStorageConverter.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- Number.cs
- WindowsListViewItemStartMenu.cs
- StyleModeStack.cs
- DataSourceCacheDurationConverter.cs
- RichTextBoxAutomationPeer.cs