Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Tokens / SamlNameIdentifierClaimResource.cs / 1 / SamlNameIdentifierClaimResource.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Runtime.Serialization; namespace System.IdentityModel.Tokens { [DataContract] public class SamlNameIdentifierClaimResource { [DataMember] string nameQualifier; [DataMember] string format; [DataMember] string name; [OnDeserialized] void OnDeserialized(StreamingContext ctx) { if (String.IsNullOrEmpty(this.name)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("name"); } public SamlNameIdentifierClaimResource(string name, string nameQualifier, string format) { if (String.IsNullOrEmpty(name)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("name"); this.name = name; this.nameQualifier = nameQualifier; this.format = format; } public string NameQualifier { get { return this.nameQualifier; } } public string Format { get { return this.format; } } public string Name { get { return this.name; } } public override bool Equals(object obj) { if (obj == null) return false; if (ReferenceEquals(this, obj)) return true; SamlNameIdentifierClaimResource rhs = obj as SamlNameIdentifierClaimResource; if (rhs == null) return false; return ((this.nameQualifier == rhs.nameQualifier) && (this.format == rhs.format) && (this.name == rhs.name)); } public override int GetHashCode() { return this.name.GetHashCode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
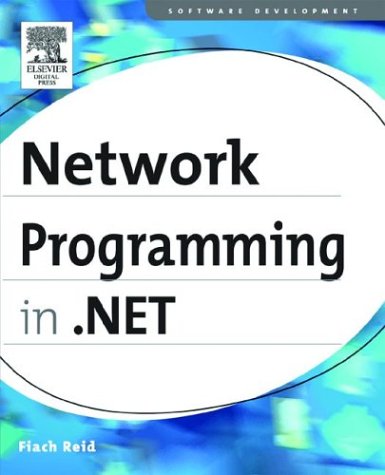
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Configuration.cs
- Documentation.cs
- WebPartConnection.cs
- InputLangChangeEvent.cs
- CryptoApi.cs
- messageonlyhwndwrapper.cs
- HtmlLiteralTextAdapter.cs
- JournalEntryStack.cs
- ResXFileRef.cs
- ObjectDisposedException.cs
- SoapSchemaMember.cs
- DirectionalLight.cs
- ToolStripHighContrastRenderer.cs
- PartialCachingControl.cs
- SetIterators.cs
- baseaxisquery.cs
- TypeLibConverter.cs
- ToolbarAUtomationPeer.cs
- EncodingInfo.cs
- RootBrowserWindow.cs
- TextRunTypographyProperties.cs
- PenContexts.cs
- OutputCacheSettingsSection.cs
- SetStoryboardSpeedRatio.cs
- TrackingMemoryStream.cs
- Configuration.cs
- ApplicationDirectory.cs
- DotNetATv1WindowsLogEntrySerializer.cs
- FileDialogCustomPlacesCollection.cs
- WindowInteropHelper.cs
- WebServiceFault.cs
- EmptyEnumerable.cs
- NumberFormatter.cs
- DataGridViewButtonColumn.cs
- BrowserCapabilitiesCompiler.cs
- unsafenativemethodstextservices.cs
- ClientSession.cs
- ArglessEventHandlerProxy.cs
- PersistenceMetadataNamespace.cs
- SerializerWriterEventHandlers.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- Authorization.cs
- RegexBoyerMoore.cs
- StateItem.cs
- QilFactory.cs
- UrlRoutingModule.cs
- CatalogZone.cs
- HotSpotCollection.cs
- AssociationTypeEmitter.cs
- XmlSchemaSimpleContentExtension.cs
- LoginUtil.cs
- BookmarkNameHelper.cs
- RemotingConfigParser.cs
- RPIdentityRequirement.cs
- CellLabel.cs
- ComboBox.cs
- BlurBitmapEffect.cs
- CodeCompileUnit.cs
- TypeDefinition.cs
- EmptyCollection.cs
- IntSecurity.cs
- BindingList.cs
- RectangleGeometry.cs
- TreePrinter.cs
- FormsIdentity.cs
- SafeNativeMemoryHandle.cs
- JobPageOrder.cs
- EndSelectCardRequest.cs
- RedirectionProxy.cs
- Code.cs
- MediaElement.cs
- HMACSHA1.cs
- HttpApplicationFactory.cs
- WpfKnownType.cs
- MatrixUtil.cs
- SecurityRuntime.cs
- AssemblySettingAttributes.cs
- CharacterMetricsDictionary.cs
- DesignerEditorPartChrome.cs
- IIS7WorkerRequest.cs
- EnvironmentPermission.cs
- HttpVersion.cs
- IisTraceListener.cs
- Queue.cs
- ProxyAssemblyNotLoadedException.cs
- NetTcpBindingCollectionElement.cs
- ToolStripCustomTypeDescriptor.cs
- ToolStripStatusLabel.cs
- mediapermission.cs
- LayoutEngine.cs
- VariantWrapper.cs
- InternalsVisibleToAttribute.cs
- Vector3DAnimationBase.cs
- SelectionItemProviderWrapper.cs
- SEHException.cs
- LocatorManager.cs
- UnknownBitmapDecoder.cs
- SmtpLoginAuthenticationModule.cs
- DesignTimeXamlWriter.cs
- FigureHelper.cs