Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / MS / Internal / IO / Packaging / TrackingMemoryStream.cs / 1305600 / TrackingMemoryStream.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This is a stream that is capable of reporting data usage up to the registered // owner // // History: // 05/24/2005: IgorBel: Initial creation. // 11/08/2005: BruceMac: Change namespace // //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.IO; namespace MS.Internal.IO.Packaging { // making this class sealed as it is taking advantage of some Virtual methods // in MemoryStream(Capacity); therefore, there is a danger of subclass overriding those and unexpected // behavior changes. Consider calls from Constructor->ReportIfNecessary->Capacity // prior to unsealing this class (they would be marked as FxCop violations) internal sealed class TrackingMemoryStream : MemoryStream { // other constructors can be added later, as we need them, for now we only use the following 2 internal TrackingMemoryStream(ITrackingMemoryStreamFactory memoryStreamFactory): base() { // although we could have implemented this constructor in terms of the other constructor; we shouldn't. // It seems safer to always call the equivalent base class constructor, as we might be ignorant about // some minor differences between various MemoryStream constructors Debug.Assert(memoryStreamFactory != null); _memoryStreamFactory = memoryStreamFactory; ReportIfNeccessary(); } internal TrackingMemoryStream (ITrackingMemoryStreamFactory memoryStreamFactory, Int32 capacity) : base(capacity) { Debug.Assert(memoryStreamFactory != null); _memoryStreamFactory = memoryStreamFactory; ReportIfNeccessary(); } // Here are the overrides for members that could possible result in changes in the allocated memory public override int Read(byte[] buffer, int offset, int count) { int result = base.Read(buffer, offset, count); ReportIfNeccessary(); return result; } public override void Write(byte[] buffer, int offset, int count) { base.Write(buffer, offset, count); ReportIfNeccessary(); } public override void SetLength(long value) { base.SetLength(value); ReportIfNeccessary(); } protected override void Dispose(bool disposing) { try { if (disposing) { if (_memoryStreamFactory != null) { // release all the memory, and report it to the TrackingMemoryStreamFactory SetLength(0); Capacity = 0; ReportIfNeccessary(); _memoryStreamFactory = null; } } } finally { base.Dispose(disposing); } } private void ReportIfNeccessary () { if (this.Capacity !=_lastReportedHighWaterMark) { // we need to report the new memory being allocated as a part of the constructor _memoryStreamFactory.ReportMemoryUsageDelta(checked(this.Capacity - _lastReportedHighWaterMark)); _lastReportedHighWaterMark = this.Capacity; } } private ITrackingMemoryStreamFactory _memoryStreamFactory; private int _lastReportedHighWaterMark; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This is a stream that is capable of reporting data usage up to the registered // owner // // History: // 05/24/2005: IgorBel: Initial creation. // 11/08/2005: BruceMac: Change namespace // //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.IO; namespace MS.Internal.IO.Packaging { // making this class sealed as it is taking advantage of some Virtual methods // in MemoryStream(Capacity); therefore, there is a danger of subclass overriding those and unexpected // behavior changes. Consider calls from Constructor->ReportIfNecessary->Capacity // prior to unsealing this class (they would be marked as FxCop violations) internal sealed class TrackingMemoryStream : MemoryStream { // other constructors can be added later, as we need them, for now we only use the following 2 internal TrackingMemoryStream(ITrackingMemoryStreamFactory memoryStreamFactory): base() { // although we could have implemented this constructor in terms of the other constructor; we shouldn't. // It seems safer to always call the equivalent base class constructor, as we might be ignorant about // some minor differences between various MemoryStream constructors Debug.Assert(memoryStreamFactory != null); _memoryStreamFactory = memoryStreamFactory; ReportIfNeccessary(); } internal TrackingMemoryStream (ITrackingMemoryStreamFactory memoryStreamFactory, Int32 capacity) : base(capacity) { Debug.Assert(memoryStreamFactory != null); _memoryStreamFactory = memoryStreamFactory; ReportIfNeccessary(); } // Here are the overrides for members that could possible result in changes in the allocated memory public override int Read(byte[] buffer, int offset, int count) { int result = base.Read(buffer, offset, count); ReportIfNeccessary(); return result; } public override void Write(byte[] buffer, int offset, int count) { base.Write(buffer, offset, count); ReportIfNeccessary(); } public override void SetLength(long value) { base.SetLength(value); ReportIfNeccessary(); } protected override void Dispose(bool disposing) { try { if (disposing) { if (_memoryStreamFactory != null) { // release all the memory, and report it to the TrackingMemoryStreamFactory SetLength(0); Capacity = 0; ReportIfNeccessary(); _memoryStreamFactory = null; } } } finally { base.Dispose(disposing); } } private void ReportIfNeccessary () { if (this.Capacity !=_lastReportedHighWaterMark) { // we need to report the new memory being allocated as a part of the constructor _memoryStreamFactory.ReportMemoryUsageDelta(checked(this.Capacity - _lastReportedHighWaterMark)); _lastReportedHighWaterMark = this.Capacity; } } private ITrackingMemoryStreamFactory _memoryStreamFactory; private int _lastReportedHighWaterMark; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
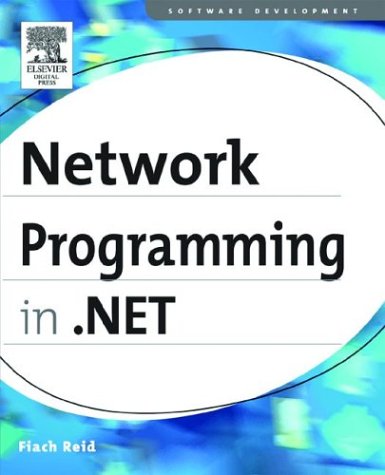
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataExpression.cs
- SymbolPair.cs
- SortQueryOperator.cs
- DataChangedEventManager.cs
- ErasingStroke.cs
- HttpWriter.cs
- WhiteSpaceTrimStringConverter.cs
- CodeBlockBuilder.cs
- IntPtr.cs
- TagMapInfo.cs
- TakeQueryOptionExpression.cs
- ConnectionPoolRegistry.cs
- ListViewDesigner.cs
- IncomingWebRequestContext.cs
- _NTAuthentication.cs
- BitmapMetadataBlob.cs
- Track.cs
- TextElementCollectionHelper.cs
- FtpRequestCacheValidator.cs
- CustomAttributeBuilder.cs
- HTMLTagNameToTypeMapper.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- CachingHintValidation.cs
- Connection.cs
- Visitor.cs
- SqlMethods.cs
- QueryContext.cs
- SqlParameterizer.cs
- ArgumentException.cs
- RequestCachePolicyConverter.cs
- SqlCacheDependency.cs
- UnsupportedPolicyOptionsException.cs
- SortedList.cs
- PropertyIDSet.cs
- SqlConnectionPoolGroupProviderInfo.cs
- WebUtility.cs
- TemplateXamlParser.cs
- ListViewInsertedEventArgs.cs
- HandlerFactoryWrapper.cs
- System.Data_BID.cs
- BooleanKeyFrameCollection.cs
- NotifyParentPropertyAttribute.cs
- TextSegment.cs
- SqlBooleanMismatchVisitor.cs
- SaveFileDialog.cs
- DrawingBrush.cs
- XamlFilter.cs
- ProcessInfo.cs
- WebPartCancelEventArgs.cs
- ListControl.cs
- HMACSHA256.cs
- WindowClosedEventArgs.cs
- MaterialGroup.cs
- RoleGroupCollection.cs
- BindingWorker.cs
- ParentQuery.cs
- DataColumn.cs
- PrintController.cs
- EdmItemCollection.cs
- MatrixTransform3D.cs
- Int64AnimationUsingKeyFrames.cs
- SynchronizationLockException.cs
- PrimarySelectionAdorner.cs
- TransformerInfo.cs
- FormatException.cs
- EntityException.cs
- RelationshipConverter.cs
- DataGridPageChangedEventArgs.cs
- OrderedDictionaryStateHelper.cs
- FtpWebRequest.cs
- TextRenderer.cs
- SspiNegotiationTokenProvider.cs
- RowVisual.cs
- ExpressionServices.cs
- RenderData.cs
- TypeExtensions.cs
- TextParagraphView.cs
- X509SecurityTokenProvider.cs
- ScrollProviderWrapper.cs
- HandlerWithFactory.cs
- CalendarItem.cs
- GeneratedCodeAttribute.cs
- DataBoundLiteralControl.cs
- DeviceContext2.cs
- DataGridAutoFormatDialog.cs
- WindowsListViewItemStartMenu.cs
- CapabilitiesUse.cs
- DateTimePicker.cs
- ExpressionLexer.cs
- ToolStripItemEventArgs.cs
- CatalogPartCollection.cs
- SchemaImporter.cs
- dsa.cs
- WindowsFormsHostAutomationPeer.cs
- MULTI_QI.cs
- _FtpDataStream.cs
- dtdvalidator.cs
- ServiceDesigner.cs
- ColumnReorderedEventArgs.cs
- ToolStripDesignerUtils.cs