Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Automation / Peers / UIElement3DAutomationPeer.cs / 1 / UIElement3DAutomationPeer.cs
using System; using System.Security; using System.Windows; using System.Windows.Input; using System.Windows.Interop; using System.Windows.Media; using System.Collections.Generic; using MS.Internal; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Automation.Peers { /// public class UIElement3DAutomationPeer: AutomationPeer { /// public UIElement3DAutomationPeer(UIElement3D owner) { if(owner == null) { throw new ArgumentNullException("owner"); } _owner = owner; } /// public UIElement3D Owner { get { return _owner; } } ////// This static helper creates an AutomationPeer for the specified element and /// caches it - that means the created peer is going to live long and shadow the /// element for its lifetime. The peer will be used by Automation to proxy the element, and /// to fire events to the Automation when something happens with the element. /// The created peer is returned from this method and also from subsequent calls to this method /// and public static AutomationPeer CreatePeerForElement(UIElement3D element) { if(element == null) { throw new ArgumentNullException("element"); } return element.CreateAutomationPeer(); } /// public static AutomationPeer FromElement(UIElement3D element) { if(element == null) { throw new ArgumentNullException("element"); } return element.GetAutomationPeer(); } /// override protected List. The type of the peer is determined by the /// virtual callback. If UIElement3D does not /// implement the callback, there will be no peer and this method will return 'null' (in other /// words, there is no such thing as a 'default peer'). /// GetChildrenCore() { List children = null; iterate(_owner, (IteratorCallback)delegate(AutomationPeer peer) { if (children == null) children = new List (); children.Add(peer); return (false); }); return children; } private delegate bool IteratorCallback(AutomationPeer peer); // private static bool iterate(DependencyObject parent, IteratorCallback callback) { bool done = false; if(parent != null) { AutomationPeer peer = null; int count = VisualTreeHelper.GetChildrenCount(parent); for (int i = 0; i < count && !done; i++) { DependencyObject child = VisualTreeHelper.GetChild(parent, i); if( child != null && child is UIElement && (peer = UIElementAutomationPeer.CreatePeerForElement((UIElement)child)) != null ) { done = callback(peer); } else if ( child != null && child is UIElement3D && (peer = CreatePeerForElement(((UIElement3D)child))) != null ) { done = callback(peer); } else { done = iterate(child, callback); } } } return done; } /// override public object GetPattern(PatternInterface patternInterface) { return null; } // // P R O P E R T I E S // /// protected override AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Custom; } /// protected override string GetAutomationIdCore() { return (AutomationProperties.GetAutomationId(_owner)); } /// protected override string GetNameCore() { return (AutomationProperties.GetName(_owner)); } /// protected override string GetHelpTextCore() { return (AutomationProperties.GetHelpText(_owner)); } /// /// override protected Rect GetBoundingRectangleCore() { Rect rectScreen; if (!ComputeBoundingRectangle(out rectScreen)) { rectScreen = Rect.Empty; } return rectScreen; } /// ////// /// Critical - Calls PresentationSource.CriticalFromVisual to get the source for this visual /// TreatAsSafe - The returned PresenationSource object is not exposed and is only used for converting /// co-ordinates to screen space. /// [SecurityCritical, SecurityTreatAsSafe] private bool ComputeBoundingRectangle(out Rect rect) { rect = Rect.Empty; PresentationSource presentationSource = PresentationSource.CriticalFromVisual(_owner); // If there's no source, the element is not visible, return empty rect if(presentationSource == null) return false; HwndSource hwndSource = presentationSource as HwndSource; // If the source isn't an HwndSource, there's not much we can do, return empty rect if(hwndSource == null) return false; Rect rectElement = _owner.Visual2DContentBounds; // we use VisualTreeHelper.GetContainingVisual2D to transform from the containing Viewport3DVisual Rect rectRoot = PointUtil.ElementToRoot(rectElement, VisualTreeHelper.GetContainingVisual2D(_owner), presentationSource); Rect rectClient = PointUtil.RootToClient(rectRoot, presentationSource); rect = PointUtil.ClientToScreen(rectClient, hwndSource); return true; } /// override protected bool IsOffscreenCore() { return !_owner.IsVisible; } /// override protected AutomationOrientation GetOrientationCore() { return (AutomationOrientation.None); } /// override protected string GetItemTypeCore() { return AutomationProperties.GetItemType(_owner); } /// override protected string GetClassNameCore() { return string.Empty; } /// override protected string GetItemStatusCore() { return AutomationProperties.GetItemStatus(_owner); } /// override protected bool IsRequiredForFormCore() { return AutomationProperties.GetIsRequiredForForm(_owner); } /// override protected bool IsKeyboardFocusableCore() { return Keyboard.IsFocusable(_owner); } /// override protected bool HasKeyboardFocusCore() { return _owner.IsKeyboardFocused; } /// override protected bool IsEnabledCore() { return _owner.IsEnabled; } /// override protected bool IsPasswordCore() { return false; } /// override protected bool IsContentElementCore() { return true; } /// override protected bool IsControlElementCore() { return true; } /// override protected AutomationPeer GetLabeledByCore() { UIElement element = AutomationProperties.GetLabeledBy(_owner); if (element != null) return element.GetAutomationPeer(); return null; } /// override protected string GetAcceleratorKeyCore() { return AutomationProperties.GetAcceleratorKey(_owner); } /// override protected string GetAccessKeyCore() { string result = AutomationProperties.GetAccessKey(_owner); if (string.IsNullOrEmpty(result)) return AccessKeyManager.InternalGetAccessKeyCharacter(_owner); return string.Empty; } // // M E T H O D S // ////// override protected Point GetClickablePointCore() { Rect rectScreen; Point pt = new Point(double.NaN, double.NaN); if (ComputeBoundingRectangle(out rectScreen)) { pt = new Point(rectScreen.Left + rectScreen.Width * 0.5, rectScreen.Top + rectScreen.Height * 0.5); } return pt; } /// override protected void SetFocusCore() { if (!_owner.Focus()) throw new InvalidOperationException(SR.Get(SRID.SetFocusFailed)); } private UIElement3D _owner; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
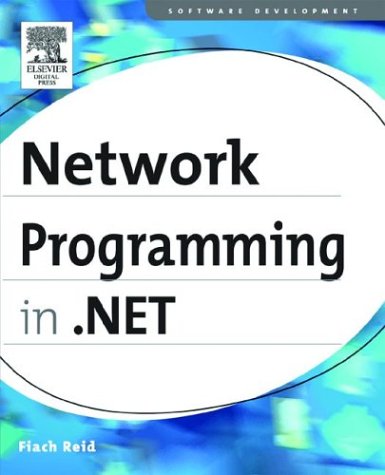
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectDataSourceEventArgs.cs
- CheckableControlBaseAdapter.cs
- SqlMethodCallConverter.cs
- MessageDescription.cs
- SafeNativeMethods.cs
- _NtlmClient.cs
- HtmlInputCheckBox.cs
- NativeObjectSecurity.cs
- PersistenceTypeAttribute.cs
- Queue.cs
- AdapterUtil.cs
- FormClosingEvent.cs
- HttpProfileBase.cs
- TableProviderWrapper.cs
- ContextMenuStripActionList.cs
- BaseParagraph.cs
- DbFunctionCommandTree.cs
- SystemWebExtensionsSectionGroup.cs
- DirectionalLight.cs
- SafePointer.cs
- ProfilePropertySettingsCollection.cs
- PolygonHotSpot.cs
- CLRBindingWorker.cs
- TextEditorLists.cs
- PopupControlService.cs
- CapabilitiesUse.cs
- KeyBinding.cs
- IntSecurity.cs
- EntityDataSourceEntityTypeFilterConverter.cs
- SQLBinary.cs
- ContainerSelectorActiveEvent.cs
- TextElement.cs
- CommandLineParser.cs
- Pool.cs
- NavigationProperty.cs
- CharStorage.cs
- GradientStop.cs
- TextElementAutomationPeer.cs
- Latin1Encoding.cs
- Subset.cs
- VirtualPathUtility.cs
- returneventsaver.cs
- PropertyExpression.cs
- HandlerBase.cs
- TrustLevelCollection.cs
- TextFormatterContext.cs
- ApplicationFileCodeDomTreeGenerator.cs
- HtmlElementErrorEventArgs.cs
- GradientStopCollection.cs
- PopupRoot.cs
- PermissionSetEnumerator.cs
- CmsInterop.cs
- GPRECT.cs
- SelectorAutomationPeer.cs
- TextCompositionEventArgs.cs
- RadioButtonPopupAdapter.cs
- XmlSchemaComplexContentRestriction.cs
- Route.cs
- Effect.cs
- SqlParameter.cs
- DateTimeConstantAttribute.cs
- InstancePersistenceException.cs
- AutoGeneratedField.cs
- ReadOnlyDataSourceView.cs
- ParsedAttributeCollection.cs
- CodeMethodReturnStatement.cs
- EventOpcode.cs
- PropertyReferenceSerializer.cs
- SqlUserDefinedAggregateAttribute.cs
- NumericPagerField.cs
- Vars.cs
- UnwrappedTypesXmlSerializerManager.cs
- PointConverter.cs
- XmlSchemaInfo.cs
- BinaryObjectReader.cs
- XhtmlBasicTextViewAdapter.cs
- XmlSerializationWriter.cs
- TextSelectionHighlightLayer.cs
- userdatakeys.cs
- UniqueID.cs
- TTSEvent.cs
- AutomationElement.cs
- Directory.cs
- BamlTreeUpdater.cs
- Matrix.cs
- _BaseOverlappedAsyncResult.cs
- EncryptedData.cs
- NaturalLanguageHyphenator.cs
- BamlWriter.cs
- AmbiguousMatchException.cs
- ManipulationVelocities.cs
- SqlBuilder.cs
- OutputScopeManager.cs
- ImportCatalogPart.cs
- Object.cs
- CacheAxisQuery.cs
- EventLogEntryCollection.cs
- TrackingMemoryStream.cs
- XmlSchemaInfo.cs
- TextEditor.cs