Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / PermissionSetEnumerator.cs / 1305376 / PermissionSetEnumerator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // namespace System.Security { //PermissionSetEnumerator.cs using System; using System.Collections; using TokenBasedSetEnumerator = System.Security.Util.TokenBasedSetEnumerator; using TokenBasedSet = System.Security.Util.TokenBasedSet; internal class PermissionSetEnumerator : IEnumerator { PermissionSetEnumeratorInternal enm; public Object Current { get { return enm.Current; } } public bool MoveNext() { return enm.MoveNext(); } public void Reset() { enm.Reset(); } internal PermissionSetEnumerator(PermissionSet permSet) { enm = new PermissionSetEnumeratorInternal(permSet); } } internal struct PermissionSetEnumeratorInternal { private PermissionSet m_permSet; private TokenBasedSetEnumerator enm; public Object Current { get { return enm.Current; } } internal PermissionSetEnumeratorInternal(PermissionSet permSet) { m_permSet = permSet; enm = new TokenBasedSetEnumerator(permSet.m_permSet); } public int GetCurrentIndex() { return enm.Index; } public void Reset() { enm.Reset(); } public bool MoveNext() { while (enm.MoveNext()) { Object obj = enm.Current; IPermission perm = obj as IPermission; if (perm != null) { enm.Current = perm; return true; } #if FEATURE_CAS_POLICY SecurityElement elem = obj as SecurityElement; if (elem != null) { perm = m_permSet.CreatePermission(elem, enm.Index); if (perm != null) { enm.Current = perm; return true; } } #endif // FEATURE_CAS_POLICY } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // namespace System.Security { //PermissionSetEnumerator.cs using System; using System.Collections; using TokenBasedSetEnumerator = System.Security.Util.TokenBasedSetEnumerator; using TokenBasedSet = System.Security.Util.TokenBasedSet; internal class PermissionSetEnumerator : IEnumerator { PermissionSetEnumeratorInternal enm; public Object Current { get { return enm.Current; } } public bool MoveNext() { return enm.MoveNext(); } public void Reset() { enm.Reset(); } internal PermissionSetEnumerator(PermissionSet permSet) { enm = new PermissionSetEnumeratorInternal(permSet); } } internal struct PermissionSetEnumeratorInternal { private PermissionSet m_permSet; private TokenBasedSetEnumerator enm; public Object Current { get { return enm.Current; } } internal PermissionSetEnumeratorInternal(PermissionSet permSet) { m_permSet = permSet; enm = new TokenBasedSetEnumerator(permSet.m_permSet); } public int GetCurrentIndex() { return enm.Index; } public void Reset() { enm.Reset(); } public bool MoveNext() { while (enm.MoveNext()) { Object obj = enm.Current; IPermission perm = obj as IPermission; if (perm != null) { enm.Current = perm; return true; } #if FEATURE_CAS_POLICY SecurityElement elem = obj as SecurityElement; if (elem != null) { perm = m_permSet.CreatePermission(elem, enm.Index); if (perm != null) { enm.Current = perm; return true; } } #endif // FEATURE_CAS_POLICY } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
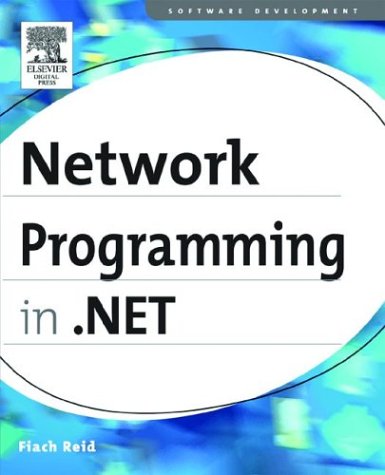
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CollectionContainer.cs
- HelpFileFileNameEditor.cs
- InteropBitmapSource.cs
- XmlSchemaAll.cs
- GridViewCommandEventArgs.cs
- NetTcpSecurity.cs
- XmlResolver.cs
- MatrixTransform.cs
- FreeIndexList.cs
- DataGridTable.cs
- InputGestureCollection.cs
- Events.cs
- SetStateDesigner.cs
- EvidenceTypeDescriptor.cs
- XmlSchemaComplexType.cs
- SchemaObjectWriter.cs
- ProvideValueServiceProvider.cs
- HtmlImageAdapter.cs
- ScriptReference.cs
- securitycriticaldata.cs
- TextViewBase.cs
- ObjectTypeMapping.cs
- WindowsHyperlink.cs
- MaterialGroup.cs
- SqlBuilder.cs
- WindowsRichEditRange.cs
- SynchronizingStream.cs
- AxParameterData.cs
- XmlStringTable.cs
- IdentityNotMappedException.cs
- DataGridViewAdvancedBorderStyle.cs
- Page.cs
- TreeViewBindingsEditor.cs
- AncestorChangedEventArgs.cs
- StreamReader.cs
- StyleXamlTreeBuilder.cs
- PermissionAttributes.cs
- ColorAnimationUsingKeyFrames.cs
- CompositeTypefaceMetrics.cs
- SafeSecurityHandles.cs
- StorageEntityContainerMapping.cs
- SpotLight.cs
- DataGridItemCollection.cs
- Part.cs
- FontFamily.cs
- DataGridViewCellCancelEventArgs.cs
- AppDomainShutdownMonitor.cs
- TextElementEnumerator.cs
- IdentityReference.cs
- EntityDataSourceReferenceGroup.cs
- IgnoreFileBuildProvider.cs
- ProcessThreadCollection.cs
- RequestValidator.cs
- RichTextBox.cs
- webbrowsersite.cs
- XsdBuildProvider.cs
- NamespaceCollection.cs
- EditorZone.cs
- PermissionSet.cs
- _AcceptOverlappedAsyncResult.cs
- UnsafeNativeMethodsTablet.cs
- LineServices.cs
- TextMessageEncoder.cs
- UnsafeNativeMethods.cs
- FixedPageStructure.cs
- Quad.cs
- OlePropertyStructs.cs
- AttributeProviderAttribute.cs
- MaxSessionCountExceededException.cs
- Enum.cs
- GroupBox.cs
- HostSecurityManager.cs
- SurrogateSelector.cs
- CommandField.cs
- GridViewPageEventArgs.cs
- SamlAssertionKeyIdentifierClause.cs
- ParserStreamGeometryContext.cs
- Int32.cs
- ClosableStream.cs
- NetStream.cs
- CqlBlock.cs
- RemoteCryptoSignHashRequest.cs
- IntPtr.cs
- MethodImplAttribute.cs
- DbConnectionClosed.cs
- ErrorProvider.cs
- MachineKeyValidationConverter.cs
- AnnotationStore.cs
- WindowHideOrCloseTracker.cs
- XmlBoundElement.cs
- SBCSCodePageEncoding.cs
- UInt64.cs
- CoreSwitches.cs
- FixedSOMPageConstructor.cs
- FormViewModeEventArgs.cs
- EntityDataSourceWizardForm.cs
- MarkupProperty.cs
- ToolStripOverflowButton.cs
- BufferedGraphicsContext.cs
- TabletDeviceInfo.cs