Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / xsp / System / Web / Extensions / ui / webcontrols / ListViewUpdateEventArgs.cs / 1 / ListViewUpdateEventArgs.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections.Specialized; using System.ComponentModel; namespace System.Web.UI.WebControls { [AspNetHostingPermission(System.Security.Permissions.SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(System.Security.Permissions.SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class ListViewUpdateEventArgs : CancelEventArgs { private int _itemIndex; private OrderedDictionary _values; private OrderedDictionary _keys; private OrderedDictionary _oldValues; public ListViewUpdateEventArgs(int itemIndex) { _itemIndex = itemIndex; } ////// public int ItemIndex { get { return _itemIndex; } } ///Gets the int argument to the command posted to the ///. This property is read-only. /// public IOrderedDictionary Keys { get { if (_keys == null) { _keys = new OrderedDictionary(); } return _keys; } } ///Gets a keyed list to populate with updated row values. This property is read-only. ////// public IOrderedDictionary NewValues { get { if (_values == null) { _values = new OrderedDictionary(); } return _values; } } ///Gets a OrderedDictionary to populate with updated row values. This property is read-only. ////// public IOrderedDictionary OldValues { get { if (_oldValues == null) { _oldValues = new OrderedDictionary(); } return _oldValues; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Gets a OrderedDictionary to populate with pre-edit row values. This property is read-only. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections.Specialized; using System.ComponentModel; namespace System.Web.UI.WebControls { [AspNetHostingPermission(System.Security.Permissions.SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(System.Security.Permissions.SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class ListViewUpdateEventArgs : CancelEventArgs { private int _itemIndex; private OrderedDictionary _values; private OrderedDictionary _keys; private OrderedDictionary _oldValues; public ListViewUpdateEventArgs(int itemIndex) { _itemIndex = itemIndex; } ////// public int ItemIndex { get { return _itemIndex; } } ///Gets the int argument to the command posted to the ///. This property is read-only. /// public IOrderedDictionary Keys { get { if (_keys == null) { _keys = new OrderedDictionary(); } return _keys; } } ///Gets a keyed list to populate with updated row values. This property is read-only. ////// public IOrderedDictionary NewValues { get { if (_values == null) { _values = new OrderedDictionary(); } return _values; } } ///Gets a OrderedDictionary to populate with updated row values. This property is read-only. ////// public IOrderedDictionary OldValues { get { if (_oldValues == null) { _oldValues = new OrderedDictionary(); } return _oldValues; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Gets a OrderedDictionary to populate with pre-edit row values. This property is read-only. ///
Link Menu
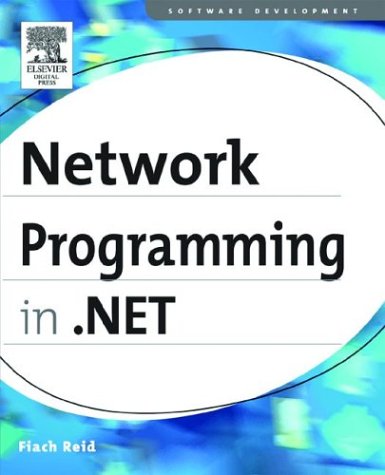
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ControlOperationInvoker.cs
- RemotingConfiguration.cs
- XmlCollation.cs
- ClassDataContract.cs
- BrowsableAttribute.cs
- OleDbParameter.cs
- WebBrowserHelper.cs
- HandoffBehavior.cs
- GeometryDrawing.cs
- HashAlgorithm.cs
- ToolConsole.cs
- PageCatalogPart.cs
- DesignerActionMethodItem.cs
- BatchServiceHost.cs
- SQLSingleStorage.cs
- JobDuplex.cs
- COM2ComponentEditor.cs
- SystemIPGlobalProperties.cs
- DecimalAnimation.cs
- AudioDeviceOut.cs
- NumberFunctions.cs
- _AutoWebProxyScriptWrapper.cs
- HttpResponseBase.cs
- MarkedHighlightComponent.cs
- ChangeBlockUndoRecord.cs
- ToolStripTemplateNode.cs
- PagedDataSource.cs
- CompilerTypeWithParams.cs
- IgnoreFileBuildProvider.cs
- DictionarySectionHandler.cs
- ClientSession.cs
- LiteralText.cs
- NavigationEventArgs.cs
- IdentityModelStringsVersion1.cs
- TimeSpanParse.cs
- Span.cs
- TextBox.cs
- WebEncodingValidator.cs
- ComplexBindingPropertiesAttribute.cs
- Propagator.JoinPropagator.cs
- HttpModuleAction.cs
- ObjectSet.cs
- TextTrailingCharacterEllipsis.cs
- RealizationDrawingContextWalker.cs
- PartialArray.cs
- ServiceRouteHandler.cs
- ValueQuery.cs
- DataGridViewElement.cs
- TemplateComponentConnector.cs
- ScaleTransform3D.cs
- WebPartCatalogAddVerb.cs
- CustomErrorCollection.cs
- JsonDeserializer.cs
- Utils.cs
- MultipleViewPattern.cs
- WebPartZoneBaseDesigner.cs
- AddInContractAttribute.cs
- Point.cs
- controlskin.cs
- HttpCapabilitiesEvaluator.cs
- LayoutUtils.cs
- EmbeddedObject.cs
- ProfileGroupSettings.cs
- GAC.cs
- ThemeConfigurationDialog.cs
- DiscoveryDocumentLinksPattern.cs
- InterleavedZipPartStream.cs
- TextBox.cs
- SqlCacheDependencyDatabase.cs
- UpdatePanelControlTrigger.cs
- TextBox.cs
- ScriptReferenceBase.cs
- FormViewUpdatedEventArgs.cs
- SessionStateModule.cs
- PersianCalendar.cs
- DataServiceEntityAttribute.cs
- WarningException.cs
- AdornerPresentationContext.cs
- DetailsViewInsertEventArgs.cs
- RegexMatchCollection.cs
- QuaternionAnimation.cs
- ArraySegment.cs
- NavigationProperty.cs
- MdbDataFileEditor.cs
- CodeNamespace.cs
- Group.cs
- ColumnReorderedEventArgs.cs
- ProcessProtocolHandler.cs
- MobilePage.cs
- DataServiceClientException.cs
- PkcsMisc.cs
- XmlSchemaObjectTable.cs
- TargetControlTypeAttribute.cs
- SqlComparer.cs
- ConfigurationElement.cs
- StdValidatorsAndConverters.cs
- LocalClientSecuritySettings.cs
- DataGridViewCellStyleConverter.cs
- TaskResultSetter.cs
- AssemblyLoader.cs