Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / ScanQueryOperator.cs / 1305376 / ScanQueryOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // ScanQueryOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// A scan is just a simple operator that is positioned directly on top of some /// real data source. It's really just a place holder used during execution and /// analysis -- it should never actually get opened. /// ///internal sealed class ScanQueryOperator : QueryOperator { private readonly IEnumerable m_data; // The actual data source to scan. //------------------------------------------------------------------------------------ // Constructs a new scan on top of the target data source. // internal ScanQueryOperator(IEnumerable data) : base(Scheduling.DefaultPreserveOrder, QuerySettings.Empty) { Contract.Assert(data != null); ParallelEnumerableWrapper wrapper = data as ParallelEnumerableWrapper ; if (wrapper != null) { data = wrapper.WrappedEnumerable; } m_data = data; } //----------------------------------------------------------------------------------- // Accesses the underlying data source. // public IEnumerable Data { get { return m_data; } } //----------------------------------------------------------------------------------- // Override of the query operator base class's Open method. It creates a partitioned // stream that reads scans the data source. // internal override QueryResults Open(QuerySettings settings, bool preferStriping) { Contract.Assert(settings.DegreeOfParallelism.HasValue); IList dataAsList = m_data as IList ; if (dataAsList != null) { return new ListQueryResults (dataAsList, settings.DegreeOfParallelism.GetValueOrDefault(), preferStriping); } else { return new ScanEnumerableQueryOperatorResults(m_data, settings); } } //----------------------------------------------------------------------------------- // IEnumerable data source represented as QueryResults . Typically, we would not // use ScanEnumerableQueryOperatorResults if the data source implements IList . // internal override IEnumerator GetEnumerator(ParallelMergeOptions? mergeOptions, bool suppressOrderPreservation) { return m_data.GetEnumerator(); } //---------------------------------------------------------------------------------------- // Returns an enumerable that represents the query executing sequentially. // internal override IEnumerable AsSequentialQuery(CancellationToken token) { return m_data; } //--------------------------------------------------------------------------------------- // The state of the order index of the results returned by this operator. // internal override OrdinalIndexState OrdinalIndexState { get { return m_data is IList ? OrdinalIndexState.Indexible : OrdinalIndexState.Correct; } } //---------------------------------------------------------------------------------------- // Whether this operator performs a premature merge. // internal override bool LimitsParallelism { get { return false; } } private class ScanEnumerableQueryOperatorResults : QueryResults { private IEnumerable m_data; // The data source for the query private QuerySettings m_settings; // Settings collected from the query internal ScanEnumerableQueryOperatorResults(IEnumerable data, QuerySettings settings) { m_data = data; m_settings = settings; } internal override void GivePartitionedStream(IPartitionedStreamRecipient recipient) { // Since we are not using m_data as an IList, we can pass useStriping = false. PartitionedStream partitionedStream = ExchangeUtilities.PartitionDataSource( m_data, m_settings.DegreeOfParallelism.Value, false); recipient.Receive (partitionedStream); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
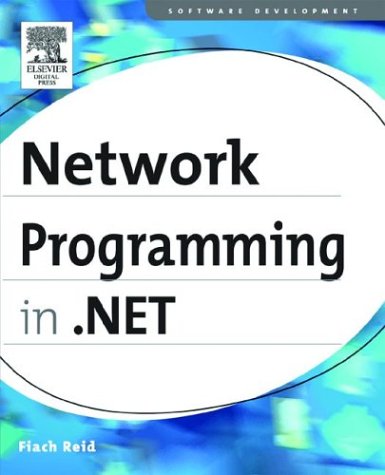
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Vertex.cs
- TypeLoadException.cs
- CapabilitiesPattern.cs
- Typography.cs
- InternalSafeNativeMethods.cs
- UriTemplateHelpers.cs
- SerializationFieldInfo.cs
- MatrixTransform3D.cs
- ColumnResult.cs
- HTTPNotFoundHandler.cs
- DependencyObjectPropertyDescriptor.cs
- Helpers.cs
- peersecuritysettings.cs
- StrokeNode.cs
- QueryTreeBuilder.cs
- DetailsViewInsertEventArgs.cs
- XslNumber.cs
- ConnectionManagementElement.cs
- ContextMenuStrip.cs
- RunInstallerAttribute.cs
- SystemIcmpV4Statistics.cs
- ContextMenuService.cs
- ExecutionProperties.cs
- StateMachineDesignerPaint.cs
- Operand.cs
- DrawingImage.cs
- PerformanceCounterManager.cs
- WebHttpElement.cs
- Decoder.cs
- XmlUTF8TextWriter.cs
- EnumerableRowCollection.cs
- UInt16.cs
- RunClient.cs
- WebConfigurationManager.cs
- RoleGroupCollection.cs
- DesignerSerializationVisibilityAttribute.cs
- Buffer.cs
- LogLogRecord.cs
- CompiledQueryCacheEntry.cs
- StandardBindingOptionalReliableSessionElement.cs
- TypeSource.cs
- AssertFilter.cs
- Normalization.cs
- OleDbFactory.cs
- ByteKeyFrameCollection.cs
- AttributeCollection.cs
- FieldInfo.cs
- SegmentInfo.cs
- StyleXamlParser.cs
- CachedCompositeFamily.cs
- DataReaderContainer.cs
- TemplateComponentConnector.cs
- VisualProxy.cs
- DateTimeFormat.cs
- Int32Rect.cs
- WorkflowOperationAsyncResult.cs
- FusionWrap.cs
- AbandonedMutexException.cs
- PerfCounters.cs
- Subtree.cs
- SqlFacetAttribute.cs
- StorageBasedPackageProperties.cs
- Splitter.cs
- DescendantBaseQuery.cs
- RuleValidation.cs
- MetadataPropertyCollection.cs
- XmlException.cs
- XamlPathDataSerializer.cs
- SynthesizerStateChangedEventArgs.cs
- CodeThrowExceptionStatement.cs
- ObjectManager.cs
- SimpleType.cs
- SQLConvert.cs
- PlainXmlDeserializer.cs
- SelectionRange.cs
- LayoutUtils.cs
- ImageSource.cs
- AddInContractAttribute.cs
- SqlConnectionManager.cs
- BaseProcessor.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- Splitter.cs
- XhtmlTextWriter.cs
- ProjectionCamera.cs
- EntityTemplateFactory.cs
- AbsoluteQuery.cs
- HorizontalAlignConverter.cs
- RadioButtonFlatAdapter.cs
- LinkLabel.cs
- RefType.cs
- TokenBasedSet.cs
- TextBox.cs
- DeploymentExceptionMapper.cs
- ProfileEventArgs.cs
- CodeDOMUtility.cs
- LoginName.cs
- FolderBrowserDialogDesigner.cs
- EventEntry.cs
- TypeValidationEventArgs.cs
- ToolStripLabel.cs