Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ContextMenuStrip.cs / 1305376 / ContextMenuStrip.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.ComponentModel; using System.Drawing; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; ///this class is just a conceptual wrapper around ToolStripDropDownMenu. [ ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), DefaultEvent("Opening"), SRDescription(SR.DescriptionContextMenuStrip) ] public class ContextMenuStrip : ToolStripDropDownMenu { /// /// Summary of ContextMenuStrip. /// public ContextMenuStrip(IContainer container) : base() { // this constructor ensures ContextMenuStrip is disposed properly since its not parented to the form. if (container == null) { throw new ArgumentNullException("container"); } container.Add(this); } public ContextMenuStrip(){ } protected override void Dispose(bool disposing) { base.Dispose(disposing); } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ContextMenuStripSourceControlDescr) ] public Control SourceControl { [UIPermission(SecurityAction.Demand, Window=UIPermissionWindow.AllWindows)] get { return SourceControlInternal; } } // minimal Clone implementation for DGV support only. internal ContextMenuStrip Clone() { // VERY limited support for cloning. ContextMenuStrip contextMenuStrip = new ContextMenuStrip(); // copy over events contextMenuStrip.Events.AddHandlers(this.Events); contextMenuStrip.AutoClose = AutoClose; contextMenuStrip.AutoSize = AutoSize; contextMenuStrip.Bounds = Bounds; contextMenuStrip.ImageList = ImageList; contextMenuStrip.ShowCheckMargin = ShowCheckMargin; contextMenuStrip.ShowImageMargin = ShowImageMargin; // copy over relevant properties for (int i = 0; i < Items.Count; i++) { ToolStripItem item = Items[i]; if (item is ToolStripSeparator) { contextMenuStrip.Items.Add(new ToolStripSeparator()); } else if (item is ToolStripMenuItem) { ToolStripMenuItem menuItem = item as ToolStripMenuItem; contextMenuStrip.Items.Add(menuItem.Clone()); } } return contextMenuStrip; } // internal overload so we know whether or not to show mnemonics. internal void ShowInternal(Control source, Point location, bool isKeyboardActivated) { Show(source, location); // if we were activated by keyboard - show mnemonics. if (isKeyboardActivated) { ToolStripManager.ModalMenuFilter.Instance.ShowUnderlines = true; } } internal void ShowInTaskbar(int x, int y) { // we need to make ourselves a topmost window WorkingAreaConstrained = false; Rectangle bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.AboveLeft); Rectangle screenBounds = Screen.FromRectangle(bounds).Bounds; if (bounds.Y < screenBounds.Y) { bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.BelowLeft); } else if (bounds.X < screenBounds.X) { bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.AboveRight); } bounds = WindowsFormsUtils.ConstrainToBounds(screenBounds, bounds); Show(bounds.X, bounds.Y); } protected override void SetVisibleCore(bool visible) { if (!visible) { WorkingAreaConstrained = true; } base.SetVisibleCore(visible); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.ComponentModel; using System.Drawing; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; ///this class is just a conceptual wrapper around ToolStripDropDownMenu. [ ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), DefaultEvent("Opening"), SRDescription(SR.DescriptionContextMenuStrip) ] public class ContextMenuStrip : ToolStripDropDownMenu { /// /// Summary of ContextMenuStrip. /// public ContextMenuStrip(IContainer container) : base() { // this constructor ensures ContextMenuStrip is disposed properly since its not parented to the form. if (container == null) { throw new ArgumentNullException("container"); } container.Add(this); } public ContextMenuStrip(){ } protected override void Dispose(bool disposing) { base.Dispose(disposing); } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ContextMenuStripSourceControlDescr) ] public Control SourceControl { [UIPermission(SecurityAction.Demand, Window=UIPermissionWindow.AllWindows)] get { return SourceControlInternal; } } // minimal Clone implementation for DGV support only. internal ContextMenuStrip Clone() { // VERY limited support for cloning. ContextMenuStrip contextMenuStrip = new ContextMenuStrip(); // copy over events contextMenuStrip.Events.AddHandlers(this.Events); contextMenuStrip.AutoClose = AutoClose; contextMenuStrip.AutoSize = AutoSize; contextMenuStrip.Bounds = Bounds; contextMenuStrip.ImageList = ImageList; contextMenuStrip.ShowCheckMargin = ShowCheckMargin; contextMenuStrip.ShowImageMargin = ShowImageMargin; // copy over relevant properties for (int i = 0; i < Items.Count; i++) { ToolStripItem item = Items[i]; if (item is ToolStripSeparator) { contextMenuStrip.Items.Add(new ToolStripSeparator()); } else if (item is ToolStripMenuItem) { ToolStripMenuItem menuItem = item as ToolStripMenuItem; contextMenuStrip.Items.Add(menuItem.Clone()); } } return contextMenuStrip; } // internal overload so we know whether or not to show mnemonics. internal void ShowInternal(Control source, Point location, bool isKeyboardActivated) { Show(source, location); // if we were activated by keyboard - show mnemonics. if (isKeyboardActivated) { ToolStripManager.ModalMenuFilter.Instance.ShowUnderlines = true; } } internal void ShowInTaskbar(int x, int y) { // we need to make ourselves a topmost window WorkingAreaConstrained = false; Rectangle bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.AboveLeft); Rectangle screenBounds = Screen.FromRectangle(bounds).Bounds; if (bounds.Y < screenBounds.Y) { bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.BelowLeft); } else if (bounds.X < screenBounds.X) { bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.AboveRight); } bounds = WindowsFormsUtils.ConstrainToBounds(screenBounds, bounds); Show(bounds.X, bounds.Y); } protected override void SetVisibleCore(bool visible) { if (!visible) { WorkingAreaConstrained = true; } base.SetVisibleCore(visible); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
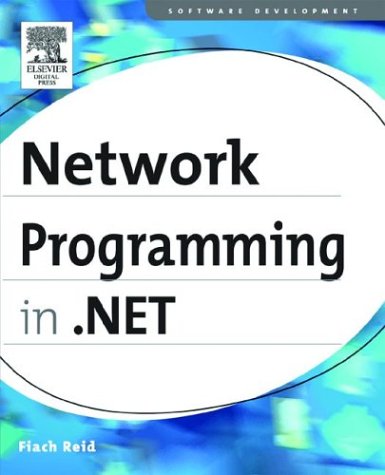
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CorrelationManager.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- XmlWellformedWriter.cs
- RelatedCurrencyManager.cs
- SqlCommandBuilder.cs
- CodeIterationStatement.cs
- MSHTMLHost.cs
- IdentityManager.cs
- HTMLTextWriter.cs
- NetNamedPipeSecurity.cs
- _Connection.cs
- OdbcConnectionFactory.cs
- SQLInt64Storage.cs
- WebPartConnection.cs
- MediaPlayer.cs
- WebResponse.cs
- TemplateContentLoader.cs
- ObjectSecurity.cs
- DataListGeneralPage.cs
- ExtractorMetadata.cs
- Profiler.cs
- PropertyMapper.cs
- ProgressBar.cs
- CommandHelper.cs
- PreProcessInputEventArgs.cs
- DesignerCategoryAttribute.cs
- SystemIPGlobalProperties.cs
- QilValidationVisitor.cs
- SoundPlayerAction.cs
- SqlAggregateChecker.cs
- KeyPullup.cs
- NativeRecognizer.cs
- IndentTextWriter.cs
- XmlTextAttribute.cs
- CancelEventArgs.cs
- PropertyTabAttribute.cs
- MediaPlayerState.cs
- SecurityResources.cs
- XhtmlBasicControlAdapter.cs
- XmlNamespaceManager.cs
- HtmlPanelAdapter.cs
- MessageLoggingElement.cs
- StdValidatorsAndConverters.cs
- GraphicsContainer.cs
- PolicyUnit.cs
- InputLanguageSource.cs
- Formatter.cs
- RegistryKey.cs
- ConfigurationSchemaErrors.cs
- KeyFrames.cs
- TdsParser.cs
- ExpressionSelection.cs
- BasicDesignerLoader.cs
- _TimerThread.cs
- StoreItemCollection.cs
- HtmlControlPersistable.cs
- EdmConstants.cs
- ButtonChrome.cs
- KeyTime.cs
- ResourcePermissionBaseEntry.cs
- DoubleAnimationUsingKeyFrames.cs
- LinkConverter.cs
- BamlResourceSerializer.cs
- BridgeDataRecord.cs
- Thickness.cs
- PermissionAttributes.cs
- WindowPatternIdentifiers.cs
- BaseProcessProtocolHandler.cs
- HelpProvider.cs
- TypeConverter.cs
- ConfigurationSection.cs
- XsdDateTime.cs
- XsltArgumentList.cs
- DataServiceQueryContinuation.cs
- PropertyChangedEventManager.cs
- XPathSingletonIterator.cs
- Accessible.cs
- KnownTypesHelper.cs
- MenuItemBinding.cs
- PermissionAttributes.cs
- ToolBarButton.cs
- ImageDrawing.cs
- DoubleCollectionConverter.cs
- AsyncResult.cs
- TypeExtension.cs
- EnvironmentPermission.cs
- TaiwanCalendar.cs
- SynchronizationValidator.cs
- LineServicesRun.cs
- ParameterBuilder.cs
- RuntimeEnvironment.cs
- XmlTextWriter.cs
- StickyNoteContentControl.cs
- SafeNativeMethods.cs
- TextHidden.cs
- RadioButtonAutomationPeer.cs
- ApplicationGesture.cs
- Binding.cs
- ScriptingProfileServiceSection.cs
- TokenBasedSet.cs