Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CommonUI / System / Drawing / Advanced / FontCollection.cs / 1 / FontCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Text { using System.Diagnostics; using System; using System.Drawing; using System.Drawing.Internal; using System.Runtime.InteropServices; using System.ComponentModel; using Microsoft.Win32; ////// /// When inherited, enumerates the FontFamily /// objects in a collection of fonts. /// public abstract class FontCollection : IDisposable { internal IntPtr nativeFontCollection; internal FontCollection() { nativeFontCollection = IntPtr.Zero; } ////// /// Disposes of this public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// protected virtual void Dispose(bool disposing) { // nothing... } /// /// /// public FontFamily[] Families { get { int numSought = 0; int status = SafeNativeMethods.Gdip.GdipGetFontCollectionFamilyCount(new HandleRef(this, nativeFontCollection), out numSought); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); IntPtr[] gpfamilies = new IntPtr[numSought]; int numFound = 0; status = SafeNativeMethods.Gdip.GdipGetFontCollectionFamilyList(new HandleRef(this, nativeFontCollection), numSought, gpfamilies, out numFound); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); Debug.Assert(numSought == numFound, "GDI+ can't give a straight answer about how many fonts there are"); FontFamily[] families = new FontFamily[numFound]; for (int f = 0; f < numFound; f++) { IntPtr native; SafeNativeMethods.Gdip.GdipCloneFontFamily(new HandleRef(null, (IntPtr)gpfamilies[f]), out native); families[f] = new FontFamily(native); } return families; } } /** * Object cleanup */ ////// Gets the array of ////// objects associated with this . /// /// /// ~FontCollection() { Dispose(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Allows an object to free resources before the object is /// reclaimed by the Garbage Collector ( ///). /// // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Text { using System.Diagnostics; using System; using System.Drawing; using System.Drawing.Internal; using System.Runtime.InteropServices; using System.ComponentModel; using Microsoft.Win32; ////// /// When inherited, enumerates the FontFamily /// objects in a collection of fonts. /// public abstract class FontCollection : IDisposable { internal IntPtr nativeFontCollection; internal FontCollection() { nativeFontCollection = IntPtr.Zero; } ////// /// Disposes of this public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// protected virtual void Dispose(bool disposing) { // nothing... } /// /// /// public FontFamily[] Families { get { int numSought = 0; int status = SafeNativeMethods.Gdip.GdipGetFontCollectionFamilyCount(new HandleRef(this, nativeFontCollection), out numSought); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); IntPtr[] gpfamilies = new IntPtr[numSought]; int numFound = 0; status = SafeNativeMethods.Gdip.GdipGetFontCollectionFamilyList(new HandleRef(this, nativeFontCollection), numSought, gpfamilies, out numFound); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); Debug.Assert(numSought == numFound, "GDI+ can't give a straight answer about how many fonts there are"); FontFamily[] families = new FontFamily[numFound]; for (int f = 0; f < numFound; f++) { IntPtr native; SafeNativeMethods.Gdip.GdipCloneFontFamily(new HandleRef(null, (IntPtr)gpfamilies[f]), out native); families[f] = new FontFamily(native); } return families; } } /** * Object cleanup */ ////// Gets the array of ////// objects associated with this . /// /// /// ~FontCollection() { Dispose(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Allows an object to free resources before the object is /// reclaimed by the Garbage Collector ( ///). ///
Link Menu
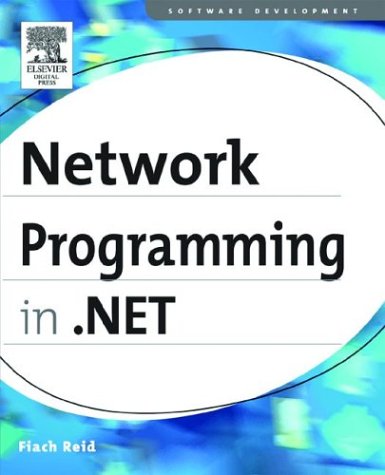
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SchemaTableOptionalColumn.cs
- BinaryParser.cs
- Translator.cs
- SqlParameter.cs
- MarkupCompilePass1.cs
- SuppressMessageAttribute.cs
- PasswordPropertyTextAttribute.cs
- WebServiceResponse.cs
- GenerateTemporaryAssemblyTask.cs
- DynamicEndpointElement.cs
- RoutedEvent.cs
- ToolTipAutomationPeer.cs
- ImportException.cs
- MarshalByValueComponent.cs
- StyleBamlRecordReader.cs
- ImpersonationContext.cs
- GlyphManager.cs
- EntityDataSourceSelectedEventArgs.cs
- ListViewHitTestInfo.cs
- MimeFormatter.cs
- DatasetMethodGenerator.cs
- QueryLifecycle.cs
- DesignerTextViewAdapter.cs
- ResponseBodyWriter.cs
- FrugalMap.cs
- DefaultCommandConverter.cs
- IntranetCredentialPolicy.cs
- CodeAccessSecurityEngine.cs
- UrlAuthorizationModule.cs
- TypeBuilderInstantiation.cs
- FunctionNode.cs
- ColorBlend.cs
- StyleCollection.cs
- Knowncolors.cs
- ImageFormat.cs
- UdpReplyToBehavior.cs
- WebDisplayNameAttribute.cs
- WorkflowIdleBehavior.cs
- Parameter.cs
- CollectionConverter.cs
- PowerStatus.cs
- ClockController.cs
- SamlSerializer.cs
- SoapAttributes.cs
- FormViewAutoFormat.cs
- DataRelationCollection.cs
- GreenMethods.cs
- TreeNodeBindingCollection.cs
- TreeNodeStyleCollection.cs
- InvalidOleVariantTypeException.cs
- ServiceDefaults.cs
- SendAgentStatusRequest.cs
- Translator.cs
- FontStretchConverter.cs
- IconHelper.cs
- EntityViewGenerator.cs
- XmlNamespaceManager.cs
- AddInServer.cs
- PointIndependentAnimationStorage.cs
- PanelDesigner.cs
- EntityReference.cs
- DataServiceResponse.cs
- StrokeNodeData.cs
- QueryResponse.cs
- Clause.cs
- Attribute.cs
- Imaging.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- FrameworkTemplate.cs
- ElementProxy.cs
- EmbeddedMailObject.cs
- WSHttpBindingCollectionElement.cs
- RoleServiceManager.cs
- CaseInsensitiveHashCodeProvider.cs
- Filter.cs
- RewritingSimplifier.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- EventTrigger.cs
- XmlSchemaCollection.cs
- ObjectAssociationEndMapping.cs
- SessionEndedEventArgs.cs
- WindowsFormsSectionHandler.cs
- BitmapPalettes.cs
- CharacterString.cs
- RadialGradientBrush.cs
- BrowsableAttribute.cs
- SortKey.cs
- SubpageParagraph.cs
- CryptoKeySecurity.cs
- GridSplitterAutomationPeer.cs
- SafePipeHandle.cs
- RowToFieldTransformer.cs
- ListViewHitTestInfo.cs
- WorkflowInstanceExtensionCollection.cs
- ListCollectionView.cs
- ContentTextAutomationPeer.cs
- AppliesToBehaviorDecisionTable.cs
- TransactionInterop.cs
- EpmTargetPathSegment.cs
- Zone.cs