Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / PanelDesigner.cs / 1 / PanelDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System.ComponentModel; using System.Diagnostics; using System; using System.Drawing; using System.Drawing.Drawing2D; using System.Windows.Forms; using Microsoft.Win32; using System.Windows.Forms.Design.Behavior; ////// /// This class handles all design time behavior for the panel class. This /// draws a visible border on the panel if it doesn't have a border so the /// user knows where the boundaries of the panel lie. /// internal class PanelDesigner : ScrollableControlDesigner { public PanelDesigner() { AutoResizeHandles = true; } ////// /// This draws a nice border around our panel. We need /// this because the panel can have no border and you can't /// tell where it is. /// ///protected virtual void DrawBorder(Graphics graphics) { Panel panel = (Panel)Component; // if the panel is invisible, bail now if(panel == null || !panel.Visible) { return; } Pen pen = BorderPen; Rectangle rc = Control.ClientRectangle; rc.Width --; rc.Height--; graphics.DrawRectangle(pen, rc); pen.Dispose(); } /// /// /// Overrides our base class. Here we check to see if there /// is no border on the panel. If not, we draw one so that /// the panel shape is visible at design time. /// protected override void OnPaintAdornments(PaintEventArgs pe) { Panel panel = (Panel)Component; if (panel.BorderStyle == BorderStyle.None) { DrawBorder(pe.Graphics); } base.OnPaintAdornments(pe); } ////// Creates a Dashed-Pen of appropriate color. /// protected Pen BorderPen { get { Color penColor = Control.BackColor.GetBrightness() < .5 ? ControlPaint.Light(Control.BackColor) : ControlPaint.Dark(Control.BackColor); Pen pen = new Pen(penColor); pen.DashStyle = DashStyle.Dash; return pen; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
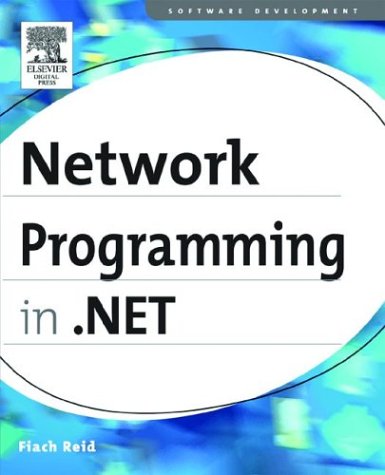
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AppDomainEvidenceFactory.cs
- OpenTypeLayoutCache.cs
- HelloMessage11.cs
- ExpressionBuilderCollection.cs
- MenuEventArgs.cs
- DataGridViewHeaderCell.cs
- TextBounds.cs
- Rule.cs
- DateTimeFormatInfo.cs
- Trace.cs
- UserMapPath.cs
- SetterBaseCollection.cs
- TextPointer.cs
- BrowserCapabilitiesFactoryBase.cs
- NamespaceEmitter.cs
- ZipArchive.cs
- QueryStringParameter.cs
- XsdDuration.cs
- SortFieldComparer.cs
- LookupBindingPropertiesAttribute.cs
- SecureStringHasher.cs
- SafeMarshalContext.cs
- IdentityHolder.cs
- GeometryGroup.cs
- HttpCookieCollection.cs
- NamespaceInfo.cs
- BCLDebug.cs
- AuthenticationSchemesHelper.cs
- RNGCryptoServiceProvider.cs
- Timer.cs
- MenuItemStyleCollection.cs
- EdmValidator.cs
- DataGridCell.cs
- DataGridPagerStyle.cs
- DataTemplateKey.cs
- PathGeometry.cs
- PageEventArgs.cs
- InvokeProviderWrapper.cs
- TextSchema.cs
- DateTimePickerDesigner.cs
- PanelStyle.cs
- DataGridViewRowPostPaintEventArgs.cs
- ReflectionPermission.cs
- RelationshipManager.cs
- DeflateEmulationStream.cs
- Int32Animation.cs
- DefaultBindingPropertyAttribute.cs
- Filter.cs
- ParenthesizePropertyNameAttribute.cs
- DataGridToolTip.cs
- Size.cs
- ExpandSegmentCollection.cs
- Filter.cs
- RootBuilder.cs
- CalendarAutomationPeer.cs
- ReadOnlyCollectionBase.cs
- WmlValidationSummaryAdapter.cs
- ListBindingConverter.cs
- FieldMetadata.cs
- _NegoStream.cs
- SetterTriggerConditionValueConverter.cs
- Span.cs
- InfoCardServiceInstallComponent.cs
- OracleInternalConnection.cs
- BaseCAMarshaler.cs
- Hashtable.cs
- SqlDependencyListener.cs
- SelectionHighlightInfo.cs
- CheckBox.cs
- Config.cs
- NetCodeGroup.cs
- LabelEditEvent.cs
- KeyEvent.cs
- EnvelopedPkcs7.cs
- EntityReference.cs
- HandleDictionary.cs
- XsdBuilder.cs
- XmlMemberMapping.cs
- ResourceManager.cs
- DiscardableAttribute.cs
- PowerStatus.cs
- ComponentResourceKey.cs
- XPathParser.cs
- ToolStrip.cs
- XPathException.cs
- Win32SafeHandles.cs
- RewritingValidator.cs
- ScriptReference.cs
- DataColumnPropertyDescriptor.cs
- ToolStripComboBox.cs
- XmlAttributeProperties.cs
- AuthorizationPolicyTypeElementCollection.cs
- SQLDecimalStorage.cs
- DataColumnPropertyDescriptor.cs
- LogWriteRestartAreaAsyncResult.cs
- StylusPointCollection.cs
- ReflectionTypeLoadException.cs
- XPathChildIterator.cs
- LocalsItemDescription.cs
- SqlFunctionAttribute.cs