Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / HtmlWindowCollection.cs / 1 / HtmlWindowCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.IO; using System.Drawing; using System.Drawing.Printing; using System.Windows.Forms; using System.Security.Permissions; using System.Security; using System.Runtime.InteropServices; using System.Net; using System.Collections; namespace System.Windows.Forms { ////// /// public class HtmlWindowCollection : ICollection { private UnsafeNativeMethods.IHTMLFramesCollection2 htmlFramesCollection2; private HtmlShimManager shimManager; internal HtmlWindowCollection(HtmlShimManager shimManager, UnsafeNativeMethods.IHTMLFramesCollection2 collection) { this.htmlFramesCollection2 = collection; this.shimManager = shimManager; Debug.Assert(this.NativeHTMLFramesCollection2 != null, "The window collection object should implement IHTMLFramesCollection2"); } private UnsafeNativeMethods.IHTMLFramesCollection2 NativeHTMLFramesCollection2 { get { return this.htmlFramesCollection2; } } ///[To be supplied.] ////// /// public HtmlWindow this[int index] { get { if (index < 0 || index >= this.Count) { throw new ArgumentOutOfRangeException("index", SR.GetString(SR.InvalidBoundArgument, "index", index, 0, this.Count - 1)); } object oIndex = (object)index; UnsafeNativeMethods.IHTMLWindow2 htmlWindow2 = this.NativeHTMLFramesCollection2.Item(ref oIndex) as UnsafeNativeMethods.IHTMLWindow2; return (htmlWindow2 != null) ? new HtmlWindow(shimManager, htmlWindow2) : null; } } ///[To be supplied.] ////// /// public HtmlWindow this[string windowId] { get { object oWindowId = (object)windowId; UnsafeNativeMethods.IHTMLWindow2 htmlWindow2 = null; try { htmlWindow2 = this.htmlFramesCollection2.Item(ref oWindowId) as UnsafeNativeMethods.IHTMLWindow2; } catch (COMException) { throw new ArgumentException(SR.GetString(SR.InvalidArgument, "windowId", windowId)); } return (htmlWindow2 != null) ? new HtmlWindow(shimManager, htmlWindow2) : null; } } ///[To be supplied.] ////// /// Returns the total number of elements in the collection. /// public int Count { get { return this.NativeHTMLFramesCollection2.GetLength(); } } ////// bool ICollection.IsSynchronized { get { return false; } } /// /// object ICollection.SyncRoot { get { return this; } } /// /// void ICollection.CopyTo(Array dest, int index) { int count = this.Count; for (int i = 0; i < count; i++) { dest.SetValue(this[i], index++); } } /// /// public IEnumerator GetEnumerator() { HtmlWindow[] htmlWindows = new HtmlWindow[this.Count]; ((ICollection)this).CopyTo(htmlWindows, 0); return htmlWindows.GetEnumerator(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.IO; using System.Drawing; using System.Drawing.Printing; using System.Windows.Forms; using System.Security.Permissions; using System.Security; using System.Runtime.InteropServices; using System.Net; using System.Collections; namespace System.Windows.Forms { ////// /// public class HtmlWindowCollection : ICollection { private UnsafeNativeMethods.IHTMLFramesCollection2 htmlFramesCollection2; private HtmlShimManager shimManager; internal HtmlWindowCollection(HtmlShimManager shimManager, UnsafeNativeMethods.IHTMLFramesCollection2 collection) { this.htmlFramesCollection2 = collection; this.shimManager = shimManager; Debug.Assert(this.NativeHTMLFramesCollection2 != null, "The window collection object should implement IHTMLFramesCollection2"); } private UnsafeNativeMethods.IHTMLFramesCollection2 NativeHTMLFramesCollection2 { get { return this.htmlFramesCollection2; } } ///[To be supplied.] ////// /// public HtmlWindow this[int index] { get { if (index < 0 || index >= this.Count) { throw new ArgumentOutOfRangeException("index", SR.GetString(SR.InvalidBoundArgument, "index", index, 0, this.Count - 1)); } object oIndex = (object)index; UnsafeNativeMethods.IHTMLWindow2 htmlWindow2 = this.NativeHTMLFramesCollection2.Item(ref oIndex) as UnsafeNativeMethods.IHTMLWindow2; return (htmlWindow2 != null) ? new HtmlWindow(shimManager, htmlWindow2) : null; } } ///[To be supplied.] ////// /// public HtmlWindow this[string windowId] { get { object oWindowId = (object)windowId; UnsafeNativeMethods.IHTMLWindow2 htmlWindow2 = null; try { htmlWindow2 = this.htmlFramesCollection2.Item(ref oWindowId) as UnsafeNativeMethods.IHTMLWindow2; } catch (COMException) { throw new ArgumentException(SR.GetString(SR.InvalidArgument, "windowId", windowId)); } return (htmlWindow2 != null) ? new HtmlWindow(shimManager, htmlWindow2) : null; } } ///[To be supplied.] ////// /// Returns the total number of elements in the collection. /// public int Count { get { return this.NativeHTMLFramesCollection2.GetLength(); } } ////// bool ICollection.IsSynchronized { get { return false; } } /// /// object ICollection.SyncRoot { get { return this; } } /// /// void ICollection.CopyTo(Array dest, int index) { int count = this.Count; for (int i = 0; i < count; i++) { dest.SetValue(this[i], index++); } } /// /// public IEnumerator GetEnumerator() { HtmlWindow[] htmlWindows = new HtmlWindow[this.Count]; ((ICollection)this).CopyTo(htmlWindows, 0); return htmlWindows.GetEnumerator(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
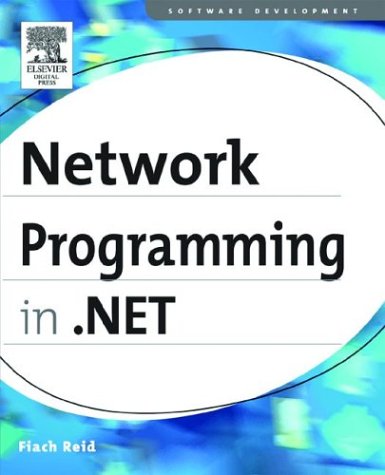
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SymmetricCryptoHandle.cs
- XomlSerializationHelpers.cs
- CommandTreeTypeHelper.cs
- AffineTransform3D.cs
- Style.cs
- TreeViewHitTestInfo.cs
- InstallerTypeAttribute.cs
- WebPartMenuStyle.cs
- XmlRootAttribute.cs
- ErrorHandler.cs
- EllipseGeometry.cs
- DocumentApplication.cs
- UrlPropertyAttribute.cs
- ListViewCommandEventArgs.cs
- __ComObject.cs
- Menu.cs
- StrokeNodeData.cs
- CodeSnippetExpression.cs
- DictionaryBase.cs
- _OSSOCK.cs
- MulticastDelegate.cs
- Visitor.cs
- VerificationException.cs
- MetadataItemEmitter.cs
- SqlServer2KCompatibilityCheck.cs
- DiscriminatorMap.cs
- XmlWriter.cs
- SettingsPropertyValue.cs
- DebugController.cs
- CommandEventArgs.cs
- KnowledgeBase.cs
- CodeDomSerializationProvider.cs
- IPHostEntry.cs
- DesignerEventService.cs
- TargetControlTypeCache.cs
- ResourceAssociationSetEnd.cs
- MatrixKeyFrameCollection.cs
- GCHandleCookieTable.cs
- TriggerAction.cs
- LinearKeyFrames.cs
- KoreanCalendar.cs
- HybridDictionary.cs
- WizardForm.cs
- CompositeDataBoundControl.cs
- LocationReference.cs
- ElementAtQueryOperator.cs
- OdbcPermission.cs
- XmlAttributes.cs
- QuaternionAnimation.cs
- MailWebEventProvider.cs
- DynamicField.cs
- LoadGrammarCompletedEventArgs.cs
- HttpHandlersSection.cs
- InheritablePropertyChangeInfo.cs
- WinInetCache.cs
- CharacterString.cs
- FormViewModeEventArgs.cs
- HtmlAnchor.cs
- AppSettingsReader.cs
- GeometryGroup.cs
- SpinWait.cs
- DebugController.cs
- CodeCompileUnit.cs
- WindowsGraphics.cs
- BasicHttpBinding.cs
- SoapObjectInfo.cs
- DataGridViewAdvancedBorderStyle.cs
- ConnectionsZone.cs
- ListViewHitTestInfo.cs
- StubHelpers.cs
- ConnectionProviderAttribute.cs
- DataTableTypeConverter.cs
- LambdaCompiler.Logical.cs
- FunctionParameter.cs
- StrongNameUtility.cs
- ToolStripContentPanelRenderEventArgs.cs
- ColumnHeaderConverter.cs
- Assert.cs
- DataGridState.cs
- SystemInfo.cs
- DecimalConstantAttribute.cs
- SpellCheck.cs
- ScrollViewer.cs
- PermissionAttributes.cs
- ReplyChannelAcceptor.cs
- HotSpotCollection.cs
- Run.cs
- ProtocolImporter.cs
- ObjectDataSourceDisposingEventArgs.cs
- QilList.cs
- webeventbuffer.cs
- Transform3DGroup.cs
- Timer.cs
- GetResponse.cs
- StreamResourceInfo.cs
- LoginUtil.cs
- FlowLayoutSettings.cs
- XpsThumbnail.cs
- SharedPersonalizationStateInfo.cs
- QueryTaskGroupState.cs